Mastering Version Control: From Centralized Systems to Distributed Git Workflows
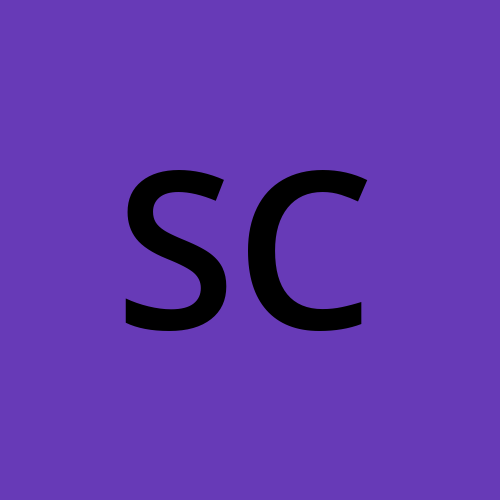
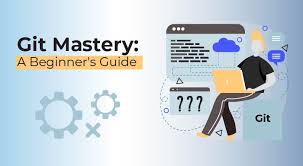
Version control systems (VCS) are essential tools for developers, providing mechanisms for sharing and versioning code. This blog will explore the differences between centralized and distributed VCS, key concepts like forking, and practical Git commands.
Centralized vs Distributed Version Control Systems
Centralized Version Control System (CVCS)
In a centralized VCS, such as Subversion (SVN), the entire codebase is stored on a central server. Developers access the code by checking it out from this central repository.
Pros:
Simple and straightforward setup.
Easier to understand and use for small teams.
Cons:
If the central server goes down, no one can access the code or communicate changes.
Single point of failure for the entire project.
Distributed Version Control System (DVCS)
In a distributed VCS, such as Git, each developer has a full copy of the repository, including its history. This setup allows for greater flexibility and robustness.
Pros:
Each developer works independently with a full repository.
Enhanced collaboration; changes can be shared directly between developers.
No single point of failure; if one copy goes down, others are still available.
Cons:
Can be more complex to set up and manage.
Requires understanding of more advanced concepts for effective use.
What is a Fork?
A fork in GitHub refers to creating an entire copy of the original repository. This allows you to experiment with changes without affecting the main project. Forks are crucial for contributing to open-source projects, enabling developers to propose changes via pull requests.
Detailed Example:
Scenario: You find an open-source project on GitHub that you want to contribute to.
Step 1: Fork the repository to create a copy in your own GitHub account.
Step 2: Clone the forked repository to your local machine.
git clone "forked-repository-url"
Step 3: Make changes to the codebase.
Step 4: Push your changes to your forked repository.
git push origin branch-name
Step 5: Create a pull request to propose your changes to the original repository.
Git vs GitHub
Git: An open-source, distributed version control system that organizations can download and implement independently. Git manages the history of changes and facilitates collaboration.
GitHub/Bitbucket/GitLab: Platforms built around Git, offering enhanced usability, code commenting, reviewing, and project management features. They add a layer of user-friendly interfaces and additional features like pull requests, issue tracking, and continuous integration.
Detailed Comparison:
Git:
Command-line tool.
Manages repositories locally.
Free and open-source.
GitHub/Bitbucket/GitLab:
Web-based platforms.
Host repositories online.
Provide collaboration tools like code reviews, issue tracking, and project boards.
Essential Git Commands
Installation
apt-get install git
Initialize an Empty Repository
git init
Show Hidden Folders
ls -la
This reveals the .git directory, which contains all the information about the repository.
Check Repository Status
git status
Add a File to the Repository
git add filename
View Changes Made
git diff
Commit Changes
git commit
Commit with Message
git commit -m "Your commit message"
Revert to a Previous Commit
git reset --hard "commit-id"
Push Changes to Remote Repository
git push
Sometimes git push
doesn't work because you aren't having any remote references, so you can add using git remote add
.
Add Remote Location
git remote add origin "repository-path"
Clone a Repository
git clone "repository-path"
Create a Branch
git branch branch-name
Switch Branches
git checkout branch-name
Merge Branches
git merge branch-name
Merge creates comments on top of branches, i.e., after the main commit is done.
Rebase Branches
git rebase branch-name
Rebase merges branches in a linear fashion, showing all changes before the main commit takes place. It's good practice to use git rebase if you want to track changes linearly.
Simplify Git Log
git log --oneline
If you want to avoid lengthy logs.
Git Workflow in an Organization
The typical workflow involves adding changes, committing with a message, and pushing to the remote repository:
git add .
git commit -m "Your commit message"
git push
Git Branching Strategy
Branching is a powerful feature of Git, allowing for isolated development and safer code integration.
Main Branch (Master Branch)
The primary working branch, always up-to-date and stable.
Feature Branch
Used to develop new features or changes. Once tested, it can be merged back into the main branch.
Release Branch
Created from the main branch to prepare for a new release. It ensures that no new changes interfere with the release process.
Hotfix Branch
Used to quickly address critical issues in the production code. Changes in this branch are merged back into the main, feature, and release branches to keep all versions up-to-date.
Example Workflow
Create a feature branch:
git checkout -b feature-branch
Work on the feature and commit changes:
git add . git commit -m "Developed new feature"
Merge the feature branch into the main branch:
git checkout main git merge feature-branch
Conclusion
By understanding these concepts and commands, you can effectively manage code versions, collaborate with team members, and maintain a clean, organized project history. Git and its related platforms provide robust solutions for modern software development, enhancing productivity and collaboration across teams.
Additional Tips:
Always Pull Before Pushing: Ensure you have the latest changes from the remote repository before you push your own changes.
git pull origin main git push origin main
Regularly Commit: Make small, frequent commits with clear messages to keep your project history clean and manageable.
Use Branches Wisely: Create branches for each new feature or bug fix to keep your main branch stable.
With these practices, you'll be well-equipped to handle any version control challenges in your development projects.
Subscribe to my newsletter
Read articles from Snigdha Chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
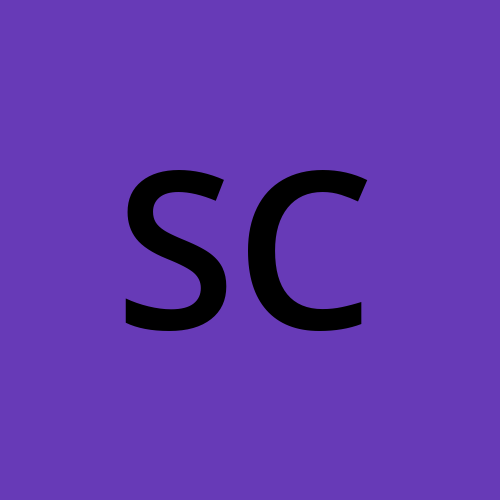