Master Laravel Service Containers and Providers : Illustrated with Office Furniture Store
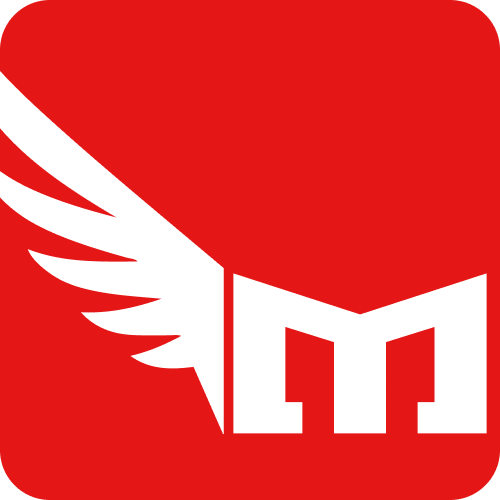
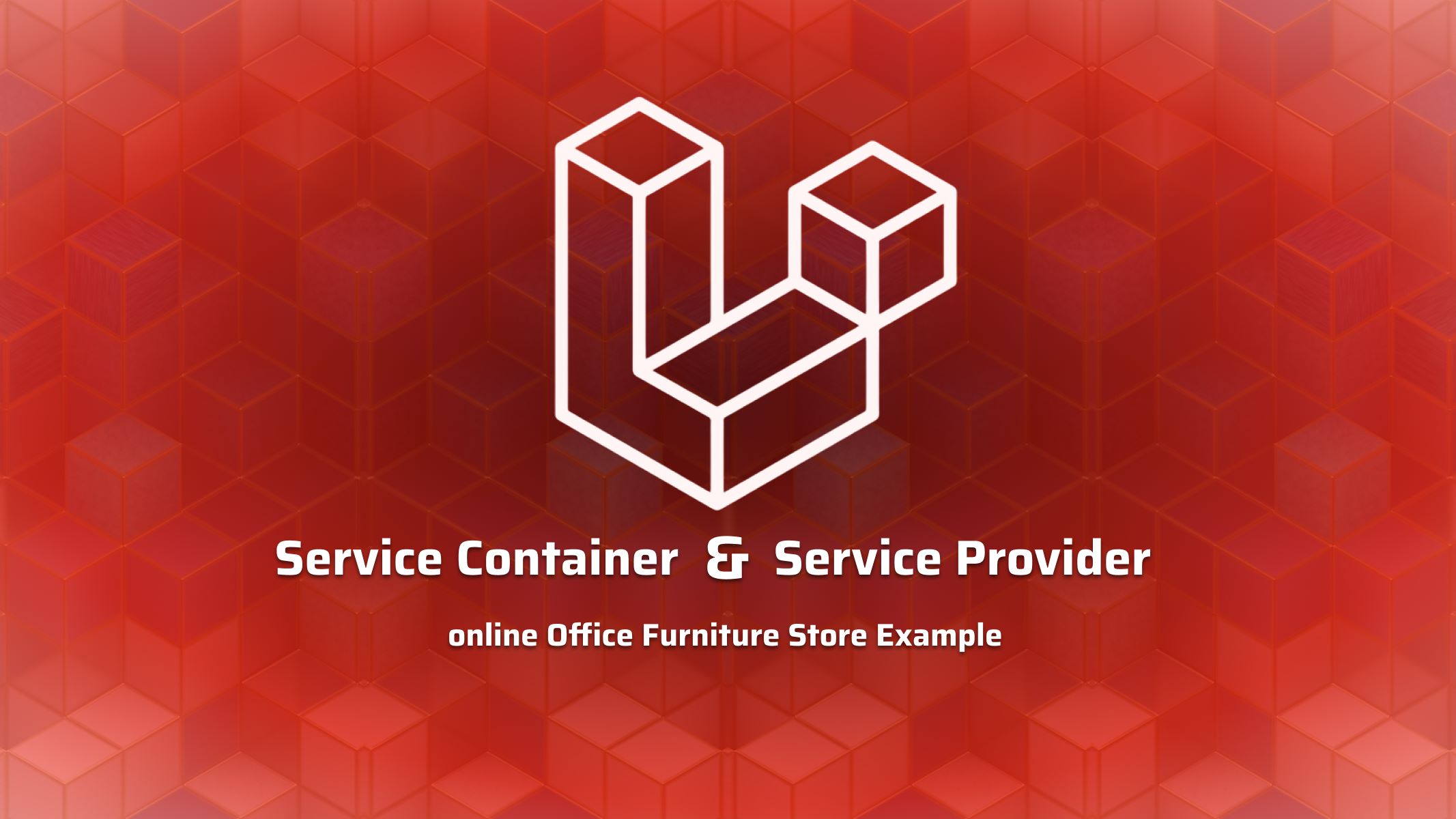
The service container in Laravel framework is a powerful tool yet not a simple paradigm to put out for someone novice with design patterns. Okay, this design pattern or architecture is for managing class dependencies and performing dependency injection. It's essentially a central registry where objects and their dependencies are stored and managed. The service container allows you to bind various classes and interfaces, making it easy to resolve and inject them wherever needed.
How It Works
Binding: You can bind classes or interfaces to the service container, telling Laravel how to instantiate them.
Resolving: When a class or interface is needed, the service container resolves it, creating an instance and injecting any dependencies it requires.
Service Provider in Laravel
Service providers are the central place to configure and bind classes into the service container. Each service provider contains a register
method to bind services to the container and a boot
method to perform actions after all services have been registered.
How It Works
Register Method: Used to bind services into the container.
Boot Method: Used to execute code after all services have been registered, ensuring that the necessary dependencies are available.
Illustrative Example: Online Office Furniture System
We take a case of an online modern office furniture store where we manage products (e.g., desks, chairs, workstations), customer orders, and inventory. We'll use Laravel's service container and service providers to manage these components.
Step-by-Step Implementation
Product Repository Interface and Implementation
Define an interface for product management and its implementation:
interface ProductRepositoryInterface { public function getAllProducts(); public function getProductById($id); } class ProductRepository implements ProductRepositoryInterface { public function getAllProducts() { // Fetch all products from the database } public function getProductById($id) { // Fetch a single product by ID from the database } }
Binding in a Service Provider
Create a service provider to bind the
ProductRepositoryInterface
to theProductRepository
implementation:namespace App\Providers; use Illuminate\Support\ServiceProvider; use App\Repositories\ProductRepositoryInterface; use App\Repositories\ProductRepository; class RepositoryServiceProvider extends ServiceProvider { public function register() { // Bind the interface to the implementation $this->app->bind(ProductRepositoryInterface::class, ProductRepository::class); } public function boot() { // Boot method if needed for additional configuration } }
Register this service provider in
config/app.php
under theproviders
array:'providers' => [ // Other Service Providers App\Providers\RepositoryServiceProvider::class, ],
Using the Service Container
In a controller, you can now type-hint the
ProductRepositoryInterface
, and Laravel will resolve it using the service container:namespace App\Http\Controllers; use App\Repositories\ProductRepositoryInterface; class ProductController extends Controller { protected $productRepository; public function __construct(ProductRepositoryInterface $productRepository) { $this->productRepository = $productRepository; } public function index() { $products = $this->productRepository->getAllProducts(); return view('products.index', compact('products')); } public function show($id) { $product = $this->productRepository->getProductById($id); return view('products.show', compact('product')); } }
Benefits in the Online Office Furniture Store System
Decoupling: The service container and service providers decouple the implementation details from the controllers, making the code more modular and testable.
Flexibility: If the product management logic changes (e.g., fetching products from an API instead of a database), you can create a new implementation of
ProductRepositoryInterface
and bind it in the service provider without changing the controllers.Ease of Testing: By binding interfaces to implementations, you can easily mock the dependencies in unit tests, making testing straightforward and isolated.
Adding a Layer of Inventory Management
Let's add another layer, such as inventory management, to further illustrate the use of the service container and providers.
Inventory Repository Interface and Implementation
interface InventoryRepositoryInterface { public function checkStock($productId); public function updateStock($productId, $quantity); } class InventoryRepository implements InventoryRepositoryInterface { public function checkStock($productId) { // Check stock for the given product ID } public function updateStock($productId, $quantity) { // Update stock for the given product ID } }
Binding in the Same Service Provider
Update the
RepositoryServiceProvider
to include the inventory repository bindings:class RepositoryServiceProvider extends ServiceProvider { public function register() { $this->app->bind(ProductRepositoryInterface::class, ProductRepository::class); $this->app->bind(InventoryRepositoryInterface::class, InventoryRepository::class); } public function boot() { // Boot method if needed for additional configuration } }
Using in a Controller
namespace App\Http\Controllers; use App\Repositories\ProductRepositoryInterface; use App\Repositories\InventoryRepositoryInterface; class InventoryController extends Controller { protected $productRepository; protected $inventoryRepository; public function __construct(ProductRepositoryInterface $productRepository, InventoryRepositoryInterface $inventoryRepository) { $this->productRepository = $productRepository; $this->inventoryRepository = $inventoryRepository; }
From now, you've become a professional Laravel framework as such functionality may only be applicable in large system than the basic ones. So, leverage Laravel's service container and service providers. You've seen how its implemented in an online office furniture system to make it highly modular, maintainable, and scalable. Here you can accommodate future changes while ensuring a clean, decoupled architecture.
Subscribe to my newsletter
Read articles from Mohammed Muwanga directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
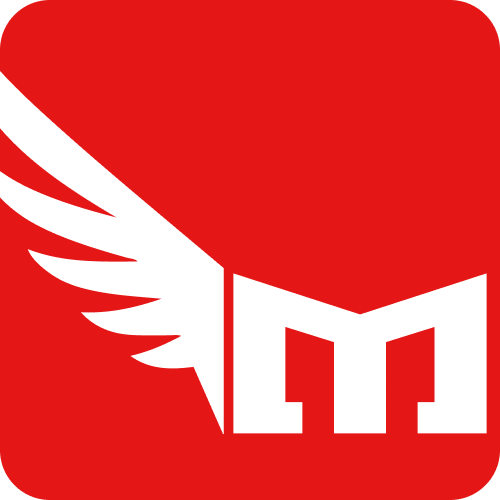
Mohammed Muwanga
Mohammed Muwanga
Wed design, Development and Workspace Ergonomics