How to Use Realtime Database in Firebase

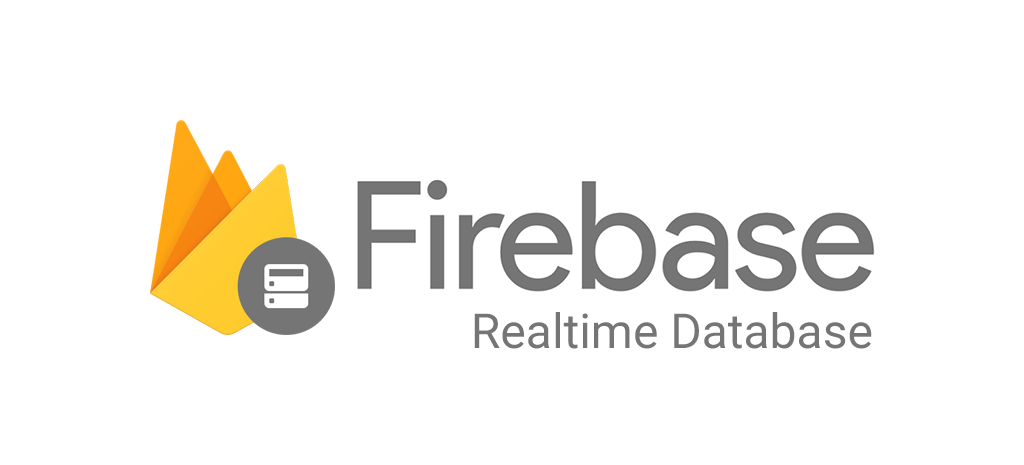
Firebase Realtime Database is a cloud-hosted NoSQL database that lets you store and sync data between your users in real-time. Whether you're developing a chat application, a collaborative tool, or an IoT application, Firebase Realtime Database provides you with a fast, efficient, and scalable solution to handle real-time data. In this blog, we'll go through the basics of how to use Firebase Realtime Database in your projects.
Setting Up Firebase
Create a Firebase Project
Go to the Firebase Console.
Click on "Add project" and follow the on-screen instructions to create a new project.
Add Firebase to Your App
Click on the web icon (</>) to set up Firebase for web applications.
Follow the instructions to register your app and add Firebase SDK to your project.
Initializing Firebase in Your Project
In your HTML file, include the Firebase SDK scripts:
<!-- Firebase App (the core Firebase SDK) is always required and must be listed first -->
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-app.js"></script>
<!-- Add the Firebase products that you want to use -->
<script src="https://www.gstatic.com/firebasejs/9.0.0/firebase-database.js"></script>
Next, initialize Firebase in your JavaScript file:
// Your web app's Firebase configuration
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
databaseURL: "https://YOUR_PROJECT_ID.firebaseio.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
// Initialize Firebase
firebase.initializeApp(firebaseConfig);
// Get a reference to the database service
const database = firebase.database();
Writing Data to Realtime Database
To write data to Firebase Realtime Database, you can use the set
method to create or overwrite data at a specified reference. For example:
// Reference to the database location
const reference = database.ref('users/' + userId);
// Set data at the reference
reference.set({
username: "JohnDoe",
email: "johndoe@example.com",
profile_picture: "https://example.com/johndoe/profile.jpg"
});
Reading Data from Realtime Database
To read data, you can use the once
method to read data once or the on
method to listen for changes in real-time:
// Read data once
reference.once('value').then((snapshot) => {
const data = snapshot.val();
console.log(data);
});
// Listen for data changes
reference.on('value', (snapshot) => {
const data = snapshot.val();
console.log(data);
});
Updating Data in Realtime Database
To update specific fields in your data without overwriting the entire data, you can use the update
method:
// Update specific fields
reference.update({
username: "JaneDoe",
email: "janedoe@example.com"
});
Deleting Data from Realtime Database
To delete data, you can use the remove
method:
// Remove data at the reference
reference.remove()
.then(() => {
console.log("Data removed successfully");
})
.catch((error) => {
console.error("Error removing data: ", error);
});
Securing Your Database
Firebase Realtime Database provides security rules that allow you to specify who has read and write access to your data. You can configure these rules in the Firebase Console under the "Database" section.
Here’s a basic example of a security rule:
{
"rules": {
".read": "auth != null",
".write": "auth != null"
}
}
This rule ensures that only authenticated users can read and write data.
Conclusion
Firebase Realtime Database is a powerful tool for building real-time applications. By following the steps outlined in this blog, you can easily set up and start using Firebase Realtime Database in your projects. Remember to explore more features and best practices in the official Firebase documentation to make the most out of this service.
Happy coding!
Subscribe to my newsletter
Read articles from Rajiv theju directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
