🚀Day 16/180 125. Valid Palindrome || (Leetcode) 125. Valid Palindrome
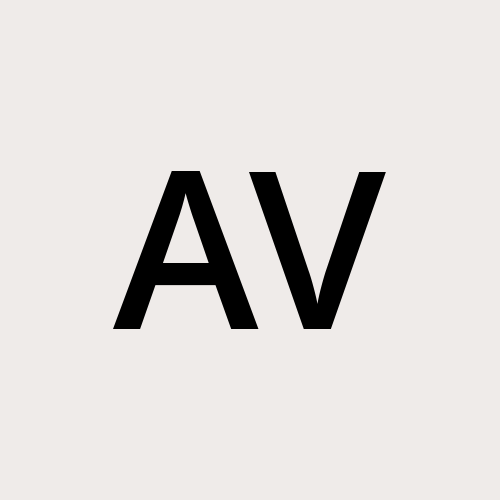
class Solution {
// This function compares the first and last characters of the string
private boolean checkPalindrome(int i, char[] ch, int n) {
if (i >= n / 2) // if the string is a palindrome, we only need to check up to half its length
return true;
if (ch[i] != ch[n - i - 1]) // if any character does not match its counterpart, return false
return false;
return checkPalindrome(i + 1, ch, n); // call the function for i + 1
}
public boolean isPalindrome(String s) {
List<Character> chList = new ArrayList<>(); // To store the characters of the string
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (Character.isLowerCase(c) || Character.isDigit(c)) {
chList.add(c); // Store the character in chList
} else if (Character.isUpperCase(c)) {
char lower = Character.toLowerCase(c); // Convert uppercase letter to lowercase
chList.add(lower); // Store the character in chList
}
}
int len = chList.size(); // This will store the size of chList, which will be used in the checkPalindrome function
char[] ch = new char[len];
for (int i = 0; i < len; i++) {
ch[i] = chList.get(i);
}
return checkPalindrome(0, ch, len); // If the function returns true, the string is a palindrome; otherwise, it's not
}
}
Let's dry run the provided Java code with an example input string "A man, a plan, a canal: Panama"
.
Dry Run:
Input: "A man, a plan, a canal: Panama"
Initial Setup:
s = "A man, a plan, a canal: Panama"
Preprocessing:
Initialize
chList
to store the characters after filtering and conversion.Loop through each character in the string:
'A'
(uppercase) -> converted to'a'
-> added tochList
.' '
(space) -> ignored.'m'
-> added tochList
.'a'
-> added tochList
.'n'
-> added tochList
.' '
(space) -> ignored.'a'
-> added tochList
.' '
(space) -> ignored.'p'
-> added tochList
.'l'
-> added tochList
.'a'
-> added tochList
.'n'
-> added tochList
.' '
(space) -> ignored.'a'
-> added tochList
.' '
(space) -> ignored.'c'
-> added tochList
.'a'
-> added tochList
.'n'
-> added tochList
.'a'
-> added tochList
.'l'
-> added tochList
.':'
(colon) -> ignored.' '
(space) -> ignored.'P'
(uppercase) -> converted to'p'
-> added tochList
.'a'
-> added tochList
.'n'
-> added tochList
.'a'
-> added tochList
.'m'
-> added tochList
.'a'
-> added tochList
.
Convert
chList
toch
array:chList = [a, m, a, n, a, p, l, a, n, a, c, a, n, a, l, a, p, a, n, a, m, a]
Convert
chList
tochar[] ch
.
Checking Palindrome:
- Call
checkPalindrome(0, ch, len)
wherelen = 21
.
- Call
Recursive Calls:
checkPalindrome(0, ch, 21)
: Comparech[0]
withch[20]
('a' == 'a'), proceed.checkPalindrome(1, ch, 21)
: Comparech[1]
withch[19]
('m' == 'm'), proceed.checkPalindrome(2, ch, 21)
: Comparech[2]
withch[18]
('a' == 'a'), proceed.checkPalindrome(3, ch, 21)
: Comparech[3]
withch[17]
('n' == 'n'), proceed.checkPalindrome(4, ch, 21)
: Comparech[4]
withch[16]
('a' == 'a'), proceed.checkPalindrome(5, ch, 21)
: Comparech[5]
withch[15]
('p' == 'p'), proceed.checkPalindrome(6, ch, 21)
: Comparech[6]
withch[14]
('l' == 'l'), proceed.checkPalindrome(7, ch, 21)
: Comparech[7]
withch[13]
('a' == 'a'), proceed.checkPalindrome(8, ch, 21)
: Comparech[8]
withch[12]
('n' == 'n'), proceed.checkPalindrome(9, ch, 21)
: Comparech[9]
withch[11]
('a' == 'a'), proceed.checkPalindrome(10, ch, 21)
: (Base case)i >= n/2
, returntrue
.
Since all recursive calls return true
, the final result is true
.
Conclusion:
The input string "A man, a plan, a canal: Panama"
is a palindrome, as confirmed by the dry run.
Subscribe to my newsletter
Read articles from Aniket Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
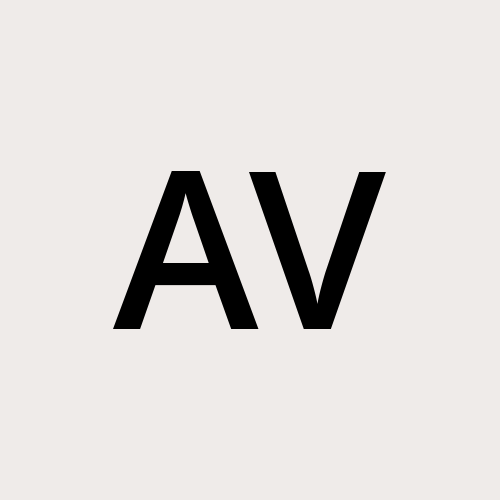