Building a Simple React Application

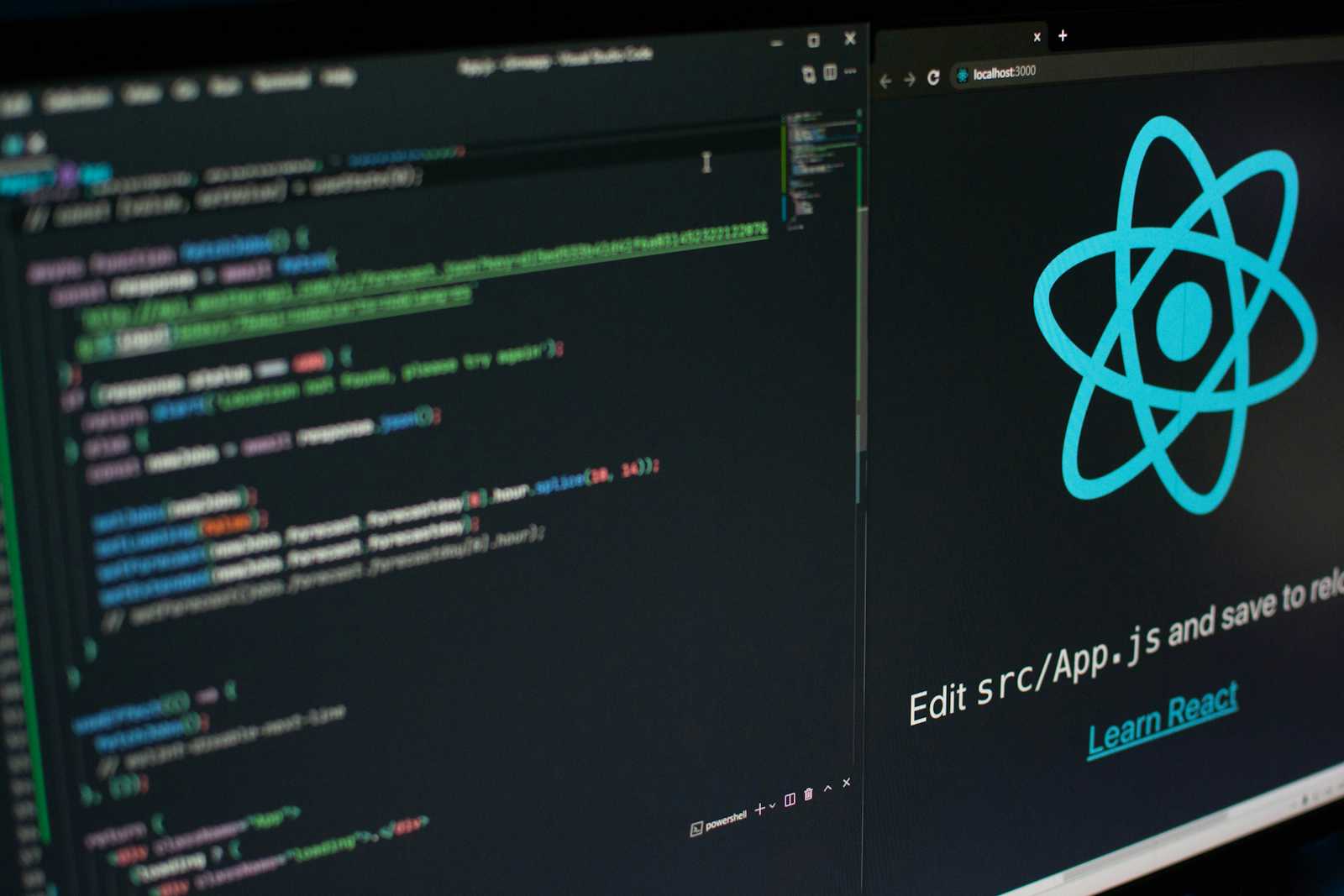
Getting Started
To begin, you'll need to have Node.js and npm (Node Package Manager) installed on your system. If you haven't already, you can download them from the official Node.js website (https://nodejs.org).
Once you have Node.js and npm set up, you can use the create-react-app tool to quickly bootstrap a new React project. Open your terminal or command prompt and run the following command:
npx create-react-app my-app
This will create a new directory called "my-app" and install all the necessary dependencies for a basic React application.
Exploring the Project Structure
After the installation is complete, navigate to the "my-app" directory and open the project in your preferred code editor. You'll see the following directory structure:
my-app/
├── node_modules/
├── public/
├── src/
├── .gitignore
├── package-json
├── package-lock.json
└── README.md
node_modules/
: This directory contains all the installed dependencies for your project.public/
: This directory holds the HTML file that will serve as the entry point for your application, as well as any other static assets (e.g., images, fonts).src/
: This is where you'll write your React components and application logic..gitignore
: This file specifies which files and directories should be ignored by Git.package.json
: This file contains metadata about your project, including dependencies and scripts.package-lock.json
: This file ensures that everyone working on the project has the same versions of dependencies installed.README.md
: This file contains information about your project, including instructions for setup and usage.
Creating a Simple Component
Now, let's create a simple React component. Open the src/App.js
file and replace its contents with the following code:
import React from 'react';
function App() {
return (
<div>
<h1>Hello, React!</h1>
<p>This is a simple React application.</p>
</div>
);
}
export default App;
In this example, we've created a functional component called App
that returns some JSX (JavaScript XML) code. The JSX code represents the structure of the component's user interface.
Running the Development Server
To start the development server and see your application in action, run the following command in your terminal:
npm start
This will start the development server and open your application in your default web browser. You should see the "Hello, React!" message displayed on the page.
Modifying the Application
Now that you have a basic React application running, you can start experimenting and adding more functionality. For example, you could create a new component, pass data between components, or add event handlers.
Here's an example of how you could create a simple counter component:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h2>Counter</h2>
<p>The current count is: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
}
export default Counter;
In this example, we've created a new component called Counter
that uses the useState
hook to manage the state of the counter. The component renders a heading, a paragraph displaying the current count, and two buttons to increment and decrement the count.
To use the Counter
component in your App
component, simply import it and add it to the JSX:
import React from 'react';
import Counter from './Counter';
function App() {
return (
<div>
<h1>Hello, React!</h1>
<p>This is a simple React application.</p>
<Counter />
</div>
);
}
export default App;
By following these steps, you've successfully created a simple React application and learned how to modify it to add more functionality. As you continue to explore and build with React, you'll discover the power and flexibility of this popular JavaScript library.
Subscribe to my newsletter
Read articles from Rajiv theju directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
