Introduction to Core Concepts of Node.js
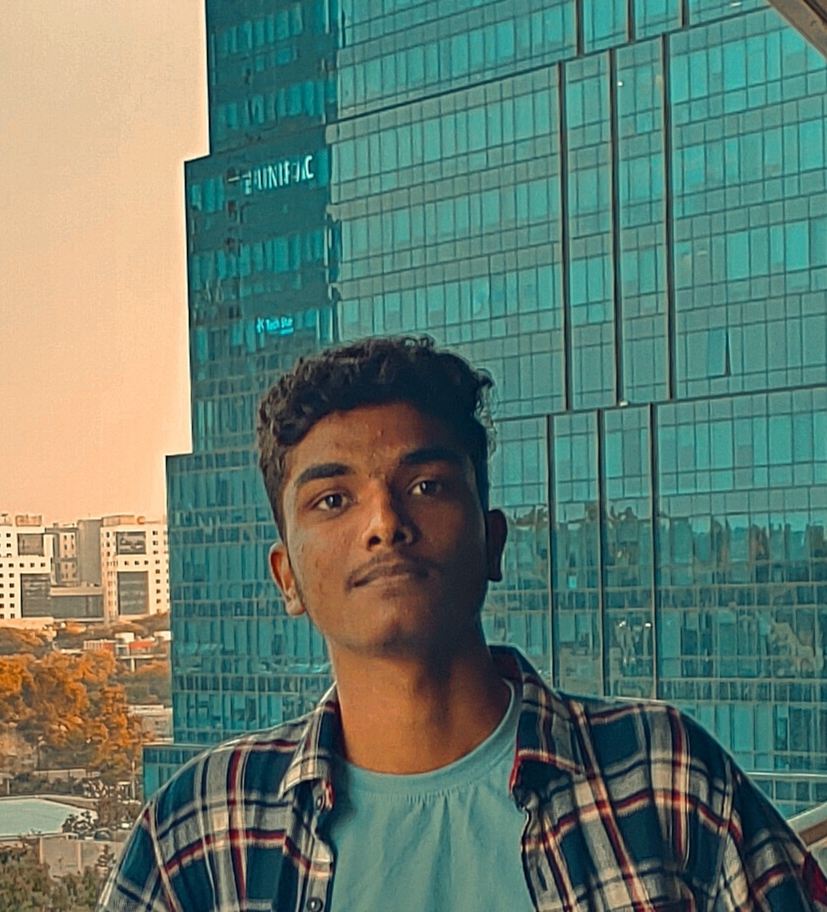
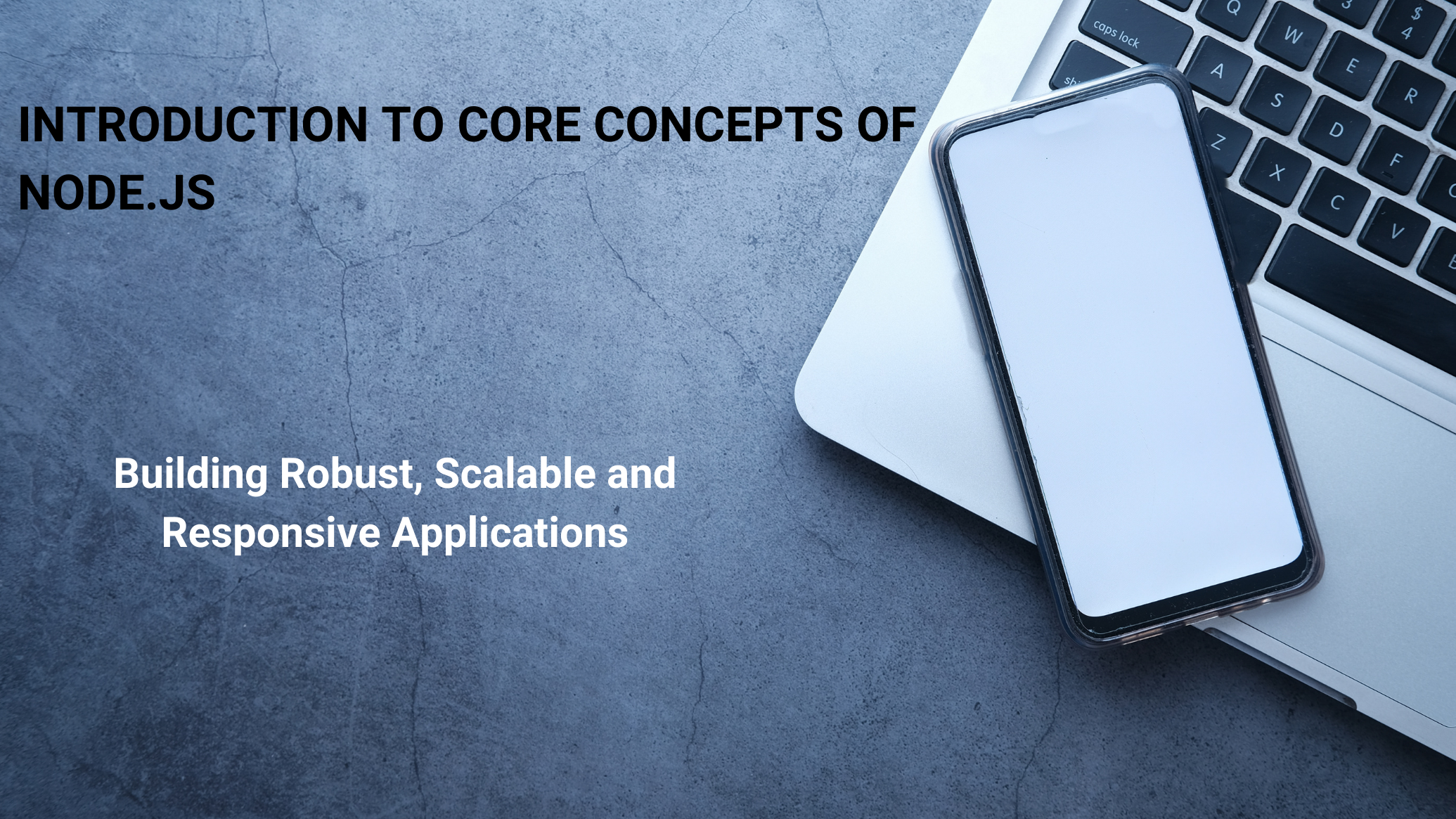
Intro
Node.js has revolutionized web development by engaging JavaScript to run on the server side. It's built on Chrome's V8 JavaScript runtime and gives a effective, lightweight environment for versatile server-side applications. To get a handle on its full potential, it's fundamental to get it its center concepts.
1. Event Loop: Enabling Asynchronous Efficiency
At the heart of Node.js lies its event loop, a fundamental mechanism that allows it to handle multiple tasks concurrently without blocking operations. This asynchronous nature is exemplified in scenarios like reading files:
const fs = require('fs');
// Initiating asynchronous file reading
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
console.log('Reading file asynchronously...');
Here, fs.readFile
initiates a non-blocking I/O operation, while Node.js continues executing subsequent code. When the file read completes, the callback function (err, data)
handles the result, showcasing Node.js's efficiency in handling I/O-bound tasks.
2. Modules: Encapsulation for Reusability
Node.js embraces modular programming with its CommonJS module system, enhancing code organization and reusability. Consider creating and using a module:
Creating a Module (math.js
):
// math.js
const add = (a, b) => a + b;
const subtract = (a, b) => a - b;
module.exports = { add, subtract };
Using the Module (app.js
):
// app.js
const { add, subtract } = require('./math');
console.log('Addition:', add(5, 3));
console.log('Subtraction:', subtract(5, 3));
This approach allows developers to encapsulate functionality into separate modules, promoting code maintainability and clarity.
3. Asynchronous Programming: Handling Concurrent Tasks
Node.js excels in asynchronous programming, crucial for handling concurrent operations such as HTTP requests:
const axios = require('axios');
// Asynchronous HTTP GET request
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log('Title:', response.data.title);
})
.catch(error => {
console.error('Error fetching data:', error);
});
Here, axios.get
asynchronously fetches data, utilizing promises (then
and catch
) to manage responses and errors effectively. This approach ensures responsiveness and scalability in applications.
4. Error Handling: Ensuring Robustness
Robust error handling is critical in Node.js applications to manage and respond to errors gracefully. Consider handling errors during file operations:
const fs = require('fs');
// Asynchronous file reading with error handling
fs.readFile('nonexistent-file.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err.message);
return;
}
console.log('File contents:', data);
});
By catching errors (err
) and providing meaningful feedback, Node.js applications can maintain stability and reliability, even in challenging scenarios.
5. Streams: Efficient Data Handling
Node.js streams are pivotal for handling large datasets or real-time data streams efficiently:
const fs = require('fs');
// Creating a readable stream from a file
const readStream = fs.createReadStream('large-file.txt', 'utf8');
// Handling stream events
readStream.on('data', chunk => {
console.log('Chunk received:', chunk);
});
readStream.on('end', () => {
console.log('Stream finished.');
});
readStream.on('error', err => {
console.error('Error reading file:', err);
});
Here, fs.createReadStream
enables streaming data from a file in manageable chunks (data
events), ensuring minimal memory usage and efficient processing of large files.
Real-World Application
Imagine developing a real-time chat application using Node.js:
Event Loop ensures the server handles multiple chat messages concurrently without blocking.
Modules organize features like user authentication and message handling into reusable components.
Asynchronous Programming enables concurrent handling of incoming messages and database queries.
Error Handling ensures graceful management of issues like database errors or message sending failures.
Streams efficiently manage real-time message streams or large file uploads, optimizing performance.
Understanding and mastering these core concepts is crucial for developers aiming to build robust, scalable, and responsive applications with Node.js. Each fundamental concept plays a pivotal role in enhancing performance and ensuring reliability in server-side development. By grasping these principles, developers can effectively optimize their applications for efficiency and maintainability, setting a solid foundation for successful Node.js projects.
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
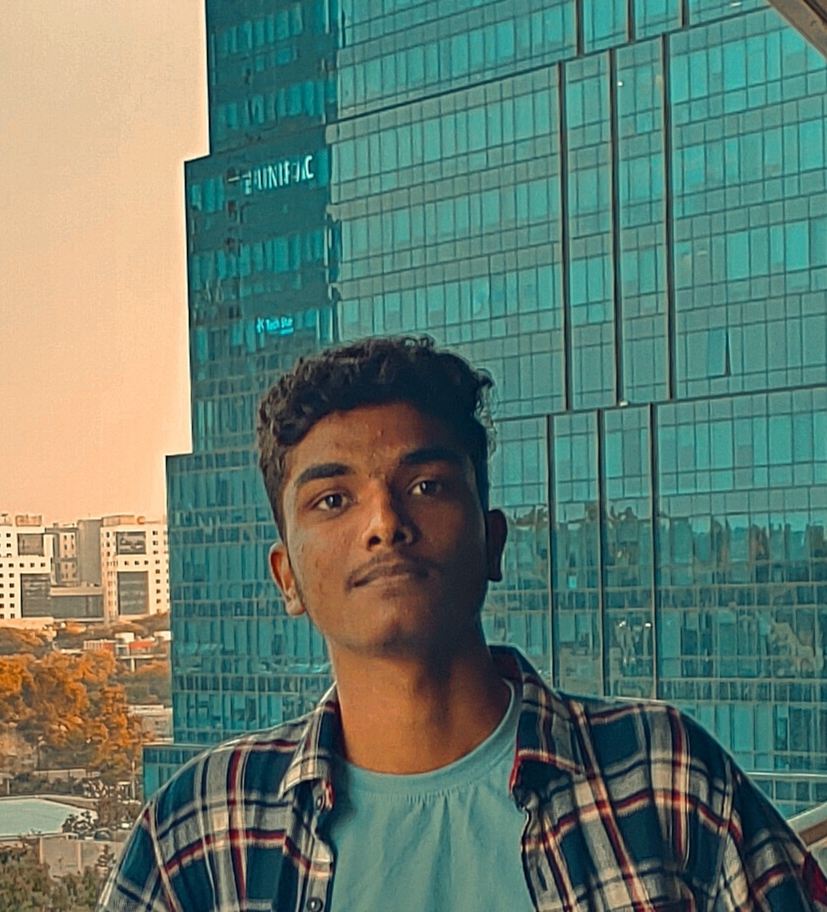
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.