Mastering Hypothesis Testing in Machine Learning Basics
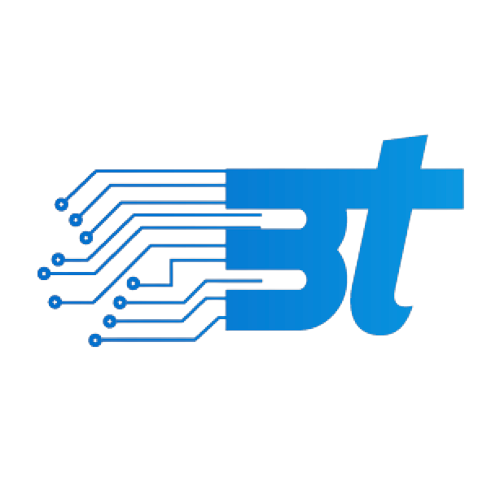
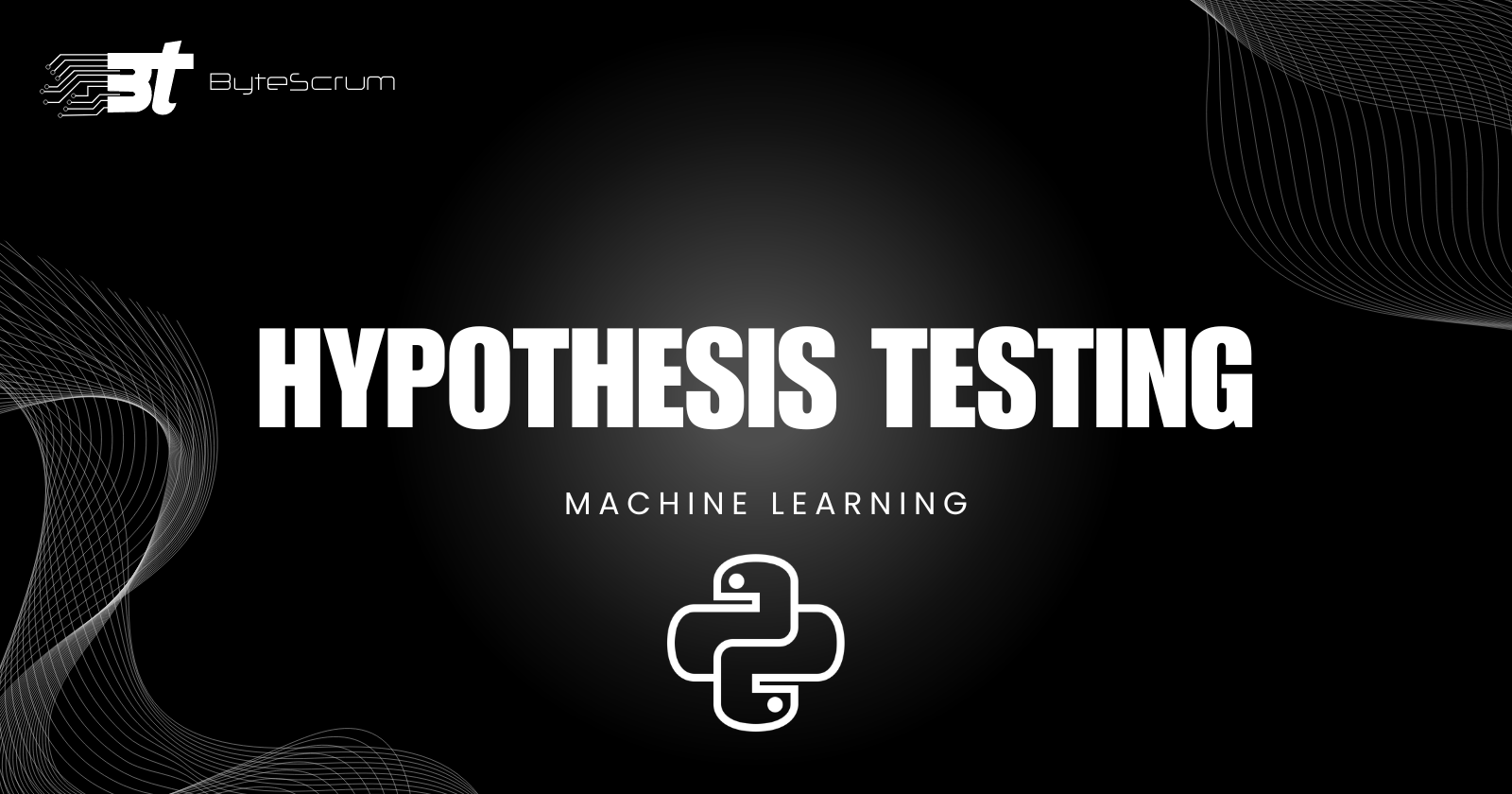
Hypothesis testing is a core concept in statistics that is crucial for machine learning. It allows us to make informed decisions based on sample data. This blog will explain the basics of hypothesis testing, its importance in machine learning, and how to perform common hypothesis tests with practical examples.
What is Hypothesis Testing?
Hypothesis testing is a method to decide whether there is enough evidence to reject a given hypothesis about a dataset. The steps involved are:
Formulate the Hypotheses:
Null Hypothesis (H₀): The default assumption (e.g., "The new treatment is not effective").
Alternative Hypothesis (H₁): What you aim to prove (e.g., "The new treatment is effective").
Select a Significance Level (α): The probability of rejecting the null hypothesis when it is true, commonly set at 0.05.
Collect Data and Compute a Test Statistic: Gather sample data and calculate a value (test statistic) that helps decide whether to reject H₀.
Make a Decision: If the p-value is less than α, reject H₀; otherwise, do not reject H₀.
Why is Hypothesis Testing Important in Machine Learning?
Hypothesis testing is vital for:
Model Validation: Ensuring improvements in model performance are statistically significant.
Feature Selection: Identifying significant features that improve model predictions.
Assumption Checking: Verifying statistical assumptions underlying machine learning algorithms.
Comparing Models: Determining if differences in model performance are statistically significant.
Common Hypothesis Tests in Machine Learning
1. t-Test
The t-test compares the means of two groups. Types include:
One-sample t-test: Tests if the mean of one sample is different from a known mean.
Two-sample t-test: Compares the means of two independent samples.
Paired t-test: Compares means from the same group at different times.
Example: One-Sample t-Test
Suppose we have a sample of exam scores from a class, and we want to know if the average score is significantly different from the passing score of 60.
import numpy as np
from scipy import stats
# Sample data
scores = [55, 65, 58, 62, 70, 68, 59, 63, 67, 61]
# Perform one-sample t-test
t_stat, p_value = stats.ttest_1samp(scores, 60)
print(f"t-statistic: {t_stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: The average score is significantly different from 60.")
else:
print("Fail to reject the null hypothesis: No significant difference from 60.")
Example: Two-sample t-test
We want to compare the test scores of two groups of students who used different study materials to prepare for an exam.
import numpy as np
from scipy import stats
# Sample data
group1 = [80, 85, 78, 90, 88]
group2 = [82, 79, 88, 85, 92]
# Perform two-sample t-test
t_stat, p_value = stats.ttest_ind(group1, group2)
print(f"t-statistic: {t_stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: The study materials have different effects on test scores.")
else:
print("Fail to reject the null hypothesis: No significant difference in test scores.")
Example: Paired t-Test
Suppose we want to determine if a new training program improves employee performance. We have performance scores before and after the training for the same group of employees.
import numpy as np
from scipy import stats
# Sample data
before_training = [70, 68, 75, 80, 72]
after_training = [75, 70, 78, 85, 76]
# Perform paired t-test
t_stat, p_value = stats.ttest_rel(before_training, after_training)
print(f"t-statistic: {t_stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: The training program significantly improved performance.")
else:
print("Fail to reject the null hypothesis: No significant improvement in performance.")
2. Chi-Square Test
The chi-square test evaluates if there is a significant association between categorical variables.
Example: Chi-Square Test
Imagine we want to know if there's an association between gender (male, female) and preference for a new product (like, dislike).
import pandas as pd
from scipy.stats import chi2_contingency
# Contingency table
data = pd.DataFrame({'Like': [30, 20], 'Dislike': [10, 40]}, index=['Male', 'Female'])
# Perform chi-square test
chi2, p, dof, expected = chi2_contingency(data)
print(f"Chi-square statistic: {chi2}, p-value: {p}")
alpha = 0.05
if p < alpha:
print("Reject the null hypothesis: There is an association between gender and product preference.")
else:
print("Fail to reject the null hypothesis: No association between gender and product preference.")
3. ANOVA (Analysis of Variance)
ANOVA compares the means of three or more groups to see if at least one group's mean is different.
Example: ANOVA
Let's compare the test scores of students using three different study methods.
from scipy import stats
# Sample data
method1 = [80, 85, 78, 90, 88]
method2 = [82, 79, 88, 85, 92]
method3 = [78, 83, 77, 84, 80]
# Perform ANOVA
f_stat, p_value = stats.f_oneway(method1, method2, method3)
print(f"F-statistic: {f_stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: At least one study method is different.")
else:
print("Fail to reject the null hypothesis: No significant difference among study methods.")
4. Wilcoxon Signed-Rank Test
The Wilcoxon signed-rank test is a non-parametric test used to compare two related samples. It is useful when the data is not normally distributed.
Example: Wilcoxon Signed-Rank Test
Suppose we have pre-test and post-test scores for the same group of students after a training program.
from scipy.stats import wilcoxon
# Sample data
pre_test = [70, 68, 75, 80, 72]
post_test = [75, 70, 78, 85, 76]
# Perform Wilcoxon signed-rank test
stat, p_value = wilcoxon(pre_test, post_test)
print(f"Test statistic: {stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: The training program had an effect.")
else:
print("Fail to reject the null hypothesis: No significant effect of the training program.")
5. Mann-Whitney U Test
The Mann-Whitney U test is a non-parametric test used to compare differences between two independent groups. It is an alternative to the two-sample t-test when the data is not normally distributed.
Example: Mann-Whitney U Test
We want to compare the effectiveness of two different diets on weight loss.
from scipy.stats import mannwhitneyu
# Sample data
diet1 = [5, 7, 8, 6, 9]
diet2 = [4, 6, 7, 5, 8]
# Perform Mann-Whitney U test
stat, p_value = mannwhitneyu(diet1, diet2)
print(f"U statistic: {stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: The diets have different effects on weight loss.")
else:
print("Fail to reject the null hypothesis: No significant difference in weight loss.")
6. Kruskal-Wallis H Test
The Kruskal-Wallis H test is a non-parametric alternative to ANOVA for comparing three or more groups.
Example: Kruskal-Wallis H Test
We want to compare the effectiveness of three different treatments on recovery time.
from scipy.stats import kruskal
# Sample data
treatment1 = [5, 7, 8, 6, 9]
treatment2 = [4, 6, 7, 5, 8]
treatment3 = [3, 5, 6, 4, 7]
# Perform Kruskal-Wallis H test
stat, p_value = kruskal(treatment1, treatment2, treatment3)
print(f"H statistic: {stat}, p-value: {p_value}")
alpha = 0.05
if p_value < alpha:
print("Reject the null hypothesis: At least one treatment is different.")
else:
print("Fail to reject the null hypothesis: No significant difference among treatments.")
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
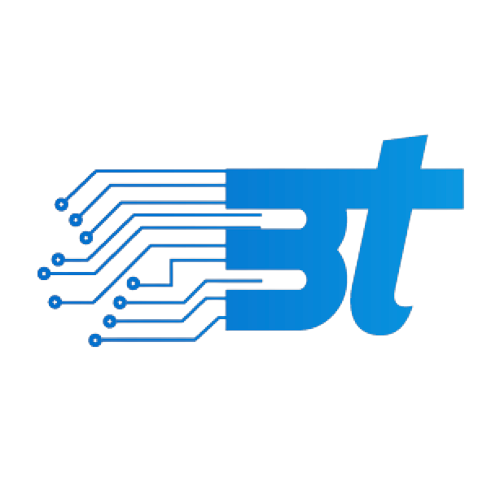
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.