Power of Higher-Order Functions : Cornerstone of Modern JavaScript
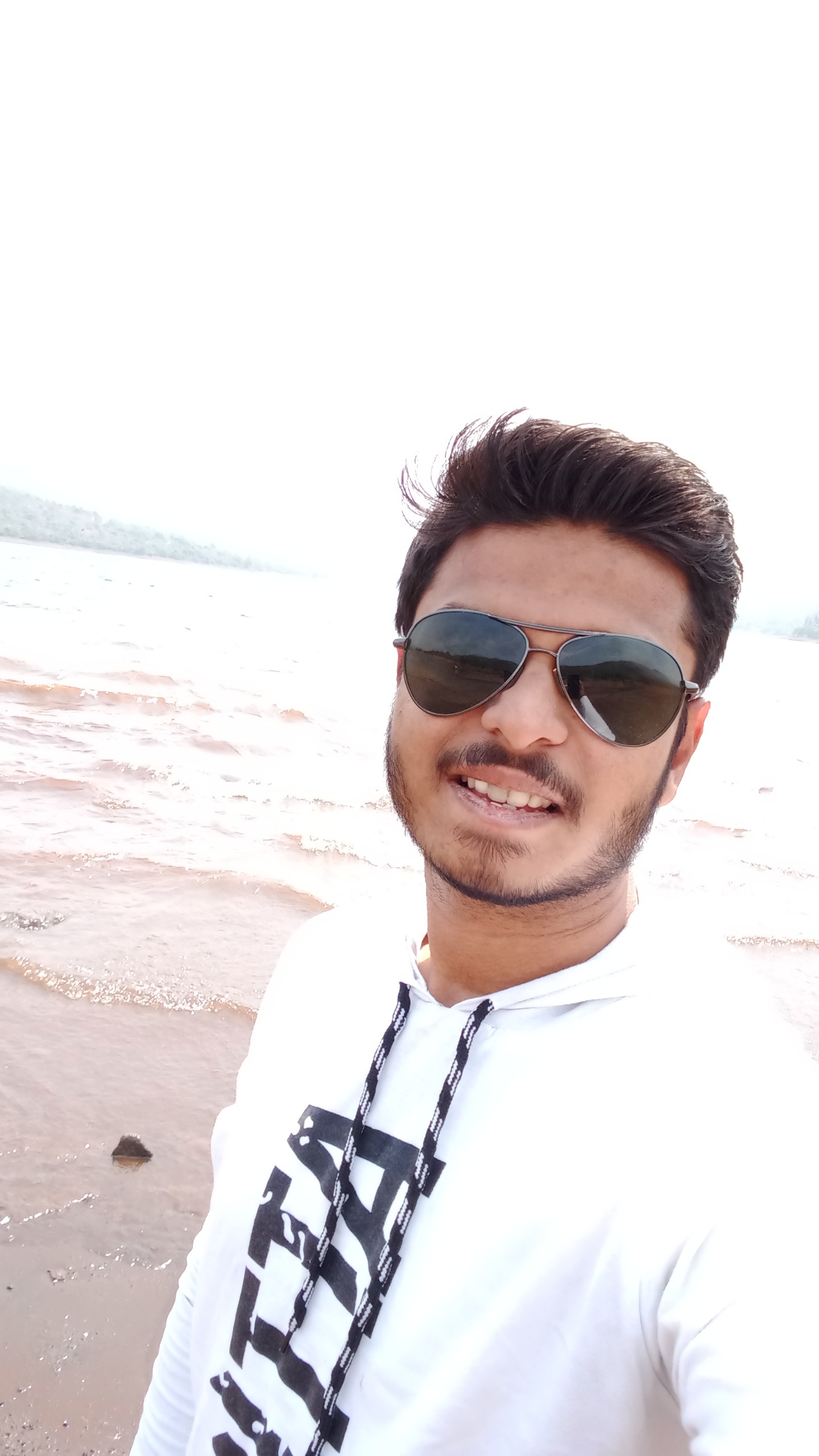
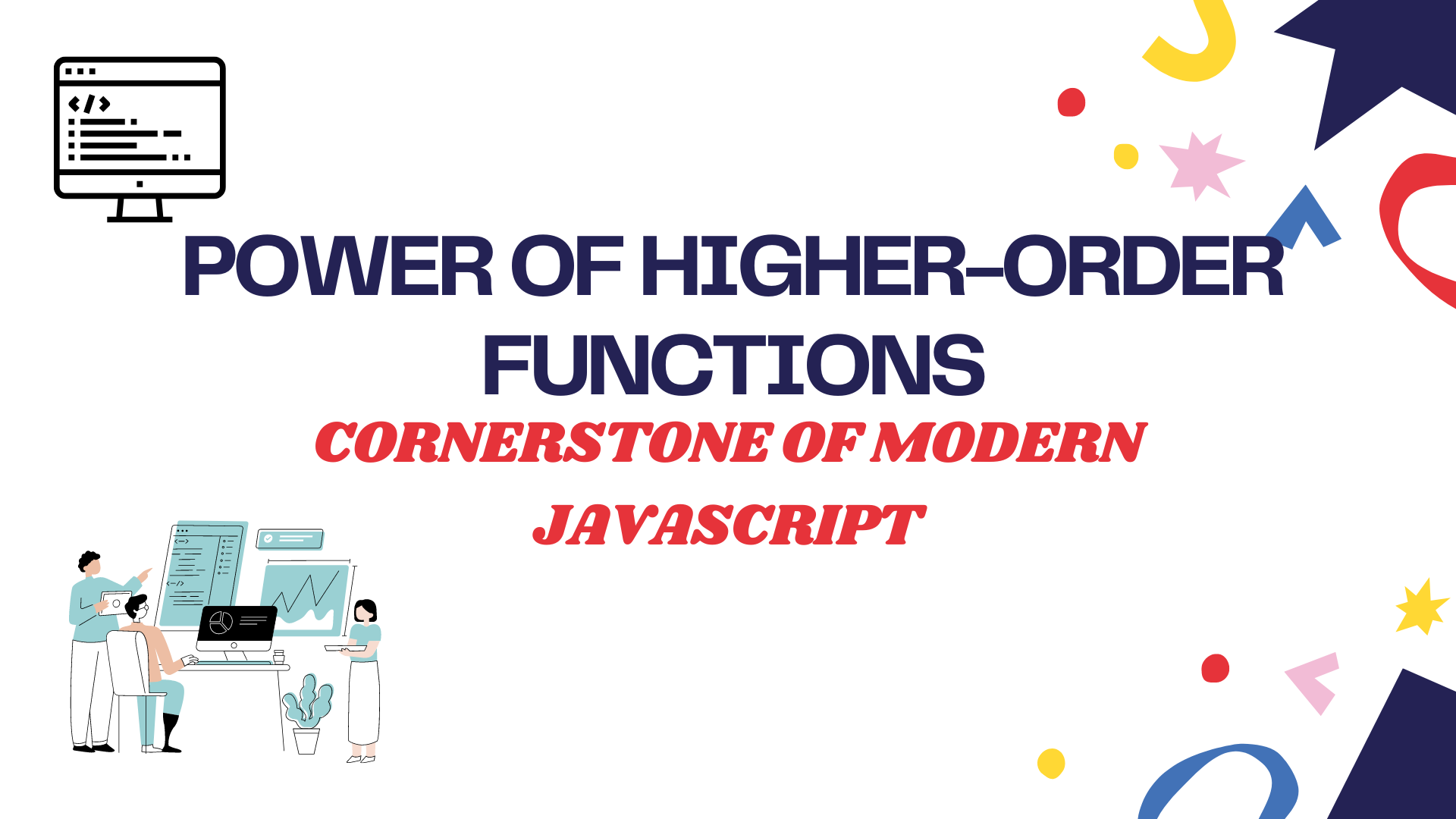
JavaScript, as one of the most versatile programming languages, offers numerous features that make it a favorite among developers. One such powerful feature is Higher-Order Functions (HOFs). In this blog, we'll explore what higher-order functions are, why they are beneficial, and how to use them effectively in your code.
What are Higher-Order Functions?
In JavaScript, functions are first-class citizens, meaning they can be treated like any other data type. Higher-order functions are functions that take other functions as arguments, return a function, or both. This allows for more abstract and flexible code.
Why Use Higher-Order Functions?
Code Reusability: Higher-order functions help in creating reusable and modular code.
Functional Programming: They are a core concept in functional programming, which leads to cleaner and more predictable code.
Abstraction: They allow for a higher level of abstraction, making complex operations easier to manage.
Common Higher-Order Functions
1. map()
The map() function takes a function as an argument and applies it to each element of an array, returning a new array with the results.
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
2. filter()
The filter() function takes a function as an argument and returns a new array containing only the elements that pass the test implemented by the provided function.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4]
3. reduce()
The reduce() function applies a function against an accumulator and each element in the array to reduce it to a single value.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // 15
Creating Your Own Higher-Order Functions
You can create your own higher-order functions to encapsulate common behaviors and improve code reuse.
function withLogging(fn) {
return function(...args) {
console.log(`Calling ${fn.name} with arguments: ${args}`);
const result = fn(...args);
console.log(`Result: ${result}`);
return result;
}
}
function add(a, b) {
return a + b;
}
const loggedAdd = withLogging(add);
loggedAdd(2, 3); // Logs: Calling add with arguments: 2,3 and Result: 5
Practical Use Cases
1. Event Handling
Higher-order functions are often used in event handling. For instance, attaching event listeners to elements dynamically.
function attachEventHandler(element, event, handler) {
element.addEventListener(event, handler);
}
const button = document.querySelector('button');
attachEventHandler(button, 'click', () => alert('Button clicked!'));
2. Middleware in Express.js
In Express.js, middleware functions are a perfect example of higher-order functions. They take req, res, and next as arguments and can perform operations before passing control to the next middleware.
function logger(req, res, next) {
console.log(`${req.method} ${req.url}`);
next();
}
app.use(logger);
Conclusion
Higher-order functions are a cornerstone of modern JavaScript development. They enable developers to write cleaner, more maintainable, and more abstract code. By understanding and utilizing higher-order functions, you can enhance your JavaScript skills and build more robust applications.
Subscribe to my newsletter
Read articles from Shreeyog Gaikwad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
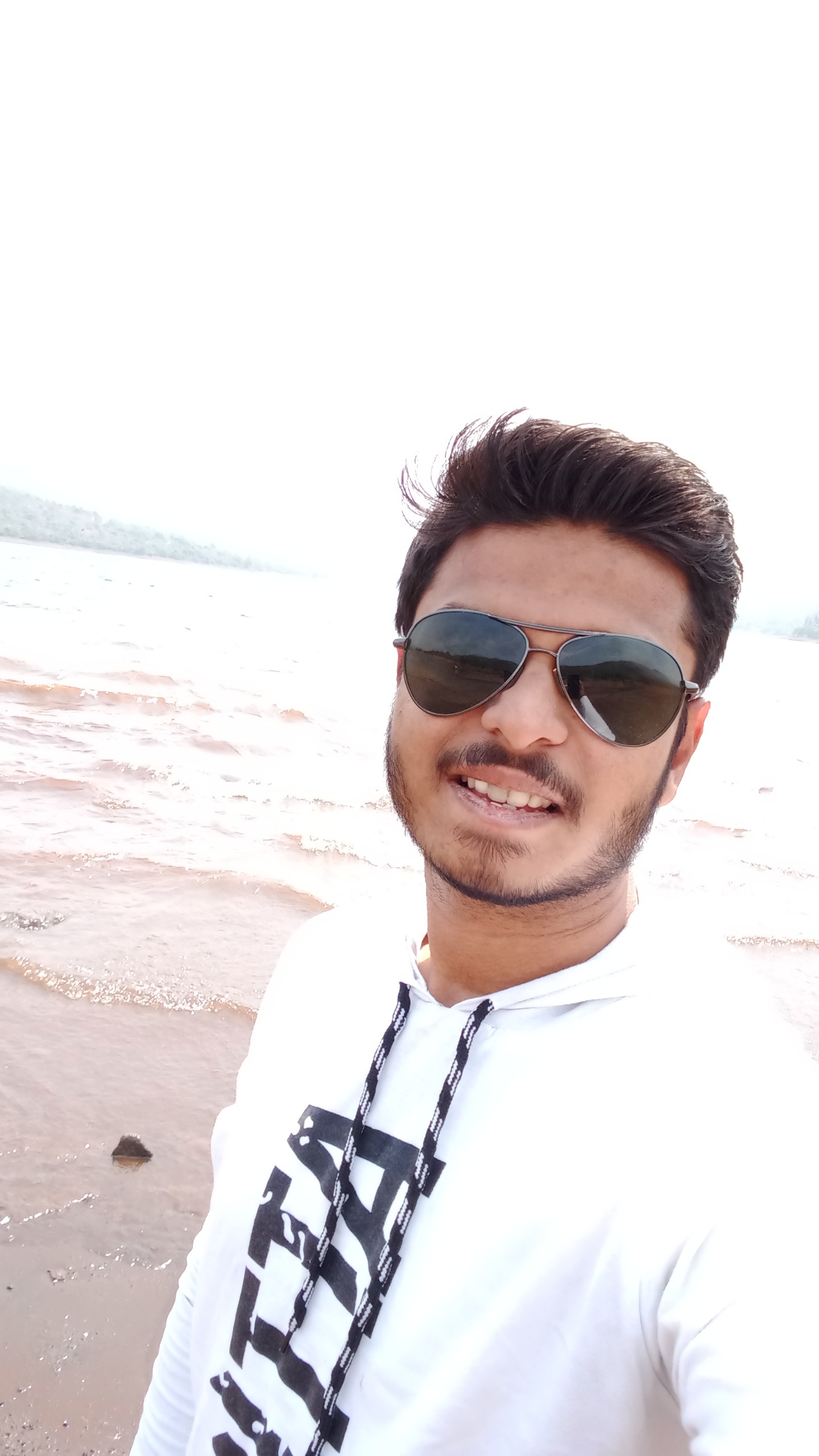
Shreeyog Gaikwad
Shreeyog Gaikwad
Passionate web developer with expertise in React, JavaScript and Node.js. Always eager to learn and share knowledge about modern web technologies. Join me on my journey of coding adventures and innovative web solutions!