Beginner's Guide to API AND REST APIs
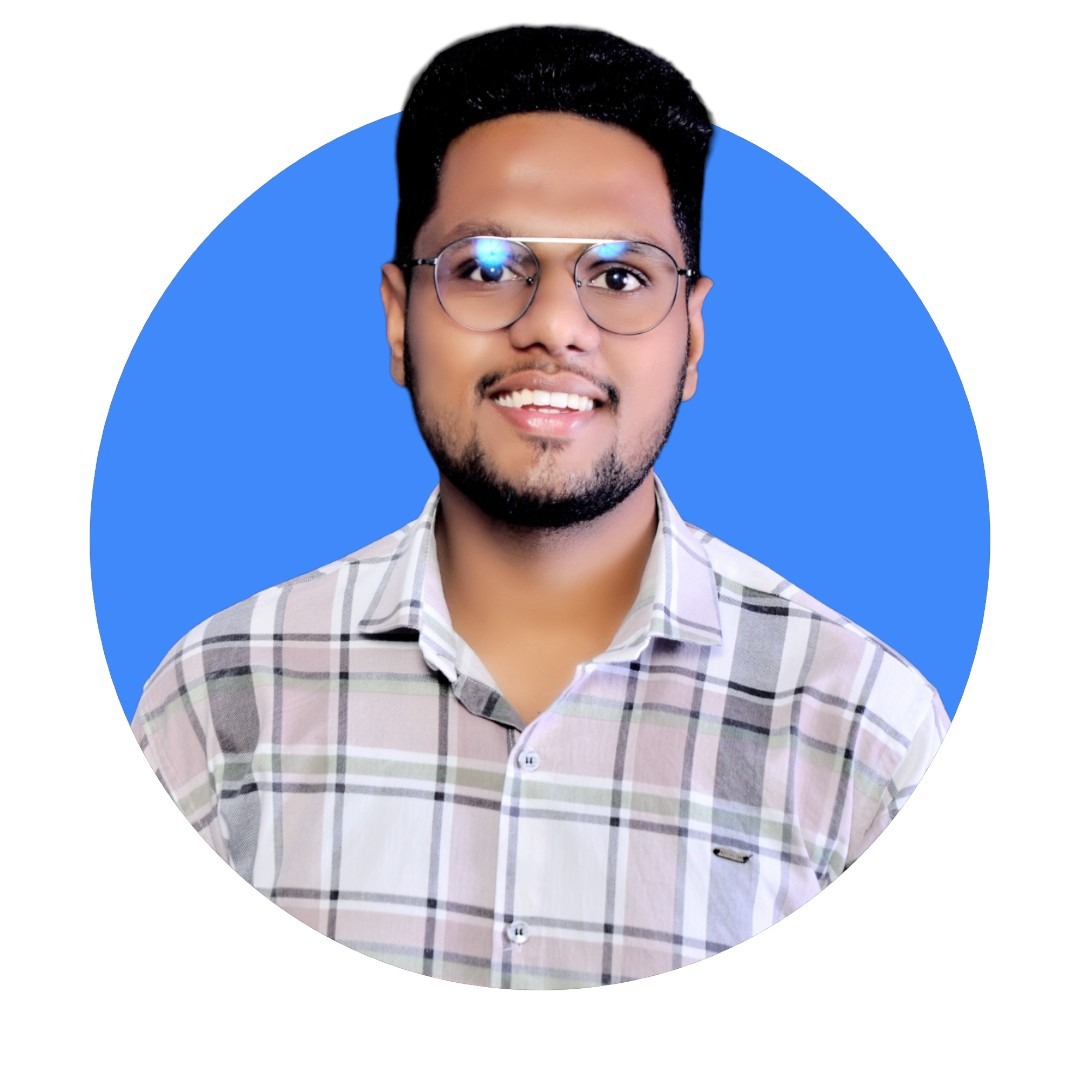
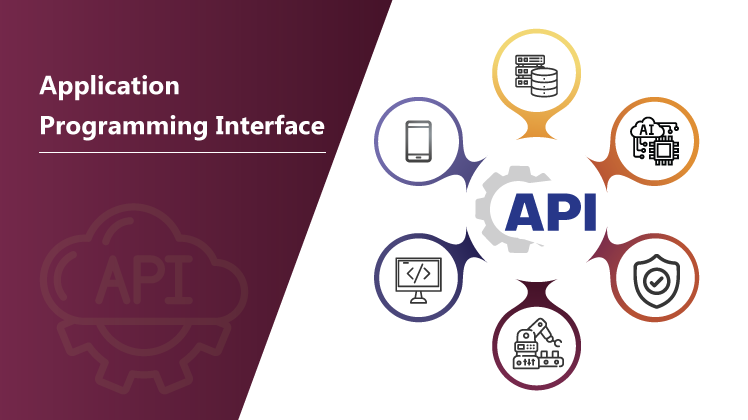
What is an API?
An API, which stands for application programming interface.
IT is a set of protocols that enable different software components to communicate and transfer data.
Developers use APIs to bridge the gaps between small, discrete chunks of code in order to create applications that are powerful, resilient, secure, and able to meet user needs.
Even though you can't see them, APIs are everywhere—working continuously in the background to power the digital experiences that are essential to our modern lives.
How do APIs work?
APIs work by sharing data between applications, systems, and devices. This happens through a request and response cycle. The request is sent to the API, which retrieves the data and returns it to the user. Here's a high-level overview of how that process works.
1. API client
The API client is responsible for starting the conversation by sending the request to the API server. The request can be triggered in many ways. For instance, a user might initiate an API request by entering a search term or clicking a button. API requests may also be triggered by external events, such as a notification from another application.
2. API request
An API request will look and behave differently depending on the type of API, but it will typically include the following components:
Endpoint: An API endpoint is a dedicated URL that provides access to a specific resource. For instance, the /articles endpoint in a blogging app would include the logic for processing all requests that are related to articles.
Method: The request's method indicates the type of operation the client would like to perform on a given resource. REST APIs are accessible through standard HTTP methods, which perform common actions like retrieving, creating, updating, and deleting data.
Parameters: Parameters are the variables that are passed to an API endpoint to provide specific instructions for the API to process. These parameters can be included in the API request as part of the URL, in the query string, or in the request body. For example, the /articles endpoint of a blogging API might accept a “topic” parameter, which it would use to access and return articles on a specific topic.
Request headers: Request headers are key-value pairs that provide extra details about the request, such as its content type or authentication credentials.
Request body: The body is the main part of the request, and it includes the actual data that is required to create, update, or delete a resource. For instance, if you were creating a new article in a blogging app, the request body would likely include the article's content, title, and author.
3. API server
The API client sends the request to the API server, which is responsible for handling authentication, validating input data, and retrieving or manipulating data.
4. API response
Finally, the API server sends a response to the client. The API response typically includes the following components:
Status code: HTTP status codes are three-digit codes that indicate the outcome of an API request. Some of the most common status codes include 200 OK, which indicates that the server successfully returned the requested data, 201 Created, which indicates the server successfully created a new resource, and 404 Not Found, which indicates that the server could not find the requested resource.
Response headers: HTTP response headers are very similar to request headers, except they are used to provide additional information about the server's response.
Response body: The response body includes the actual data or content the client asked for—or an error message if something went wrong.
In order to better understand this process, it can be useful to think of APIs like restaurants. In this metaphor, the customer is like the user, who tells the waiter what she wants. The waiter is like an API client, receiving the customer's order and translating it into easy-to-follow instructions for the kitchen—sometimes using specific codes or abbreviations that the kitchen staff will recognize. The kitchen staff is like the API server because it creates the order according to the customer's specifications and gives it to the waiter, who then delivers it to the customer.
What is REST API in Node.js ?
REST (Representational State Transfer) is an architectural style for designing networked applications.
An API is always needed to create mobile applications, single page applications, use AJAX calls and provide data to clients.
An popular architectural style of how to structure and name these APIs and the endpoints is called REST(Representational Transfer State).
A RESTful API is an API that adheres to the principles of REST, making it easy to interact with and understand.
In this article, we’ll explore what REST API is in the context of Node.js, its principles, and how to create one.
Understanding REST API
A RESTful API is a web service that follows the principles of REST architecture. It uses standard HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources, and data is typically transferred in JSON or XML format.
Principles of REST API
Client-Server Architecture: The client and server are separate entities that communicate via a stateless protocol (usually HTTP).
Statelessness: Each request from the client to the server must contain all the information necessary to understand and process the request. The server does not store any client state between requests.
Uniform Interface: Resources are identified by URIs (Uniform Resource Identifiers), and interactions with resources are performed using standard HTTP methods.
Cacheability: Responses must define whether they are cacheable or not to improve performance.
Layered System: The architecture can be composed of multiple layers, such as load balancers, proxies, and gateways, which can be used to improve scalability and security.
HTTP Methods Used in API
GET Method
Retrieves information from the system.
Used to fetch resources from a server specified by the given URI or URL.
POST Method
Creates new resources on the server.
Used for submitting data to be processed to a specified resource.
PUT Method
Updates the database with an entirely new entry or replaces the previous resource with a new one.
Used for creating a new resource or replacing an existing resource entirely.
PATCH Method
Partially updates an existing resource.
Used when only specific parts of a resource need to be modified, rather than replacing the entire resource.
DELETE Method
Removes an existing resource from the server.
Used to delete a resource identified by the given URI.
Understanding PUT and PATCH :
PUT Method Example
Imagine Amazon shipped you a bicycle that arrived broken.
Resolving the issue with a PUT method would involve replacing the entire bicycle, providing a new complete entity to replace the broken one.
PATCH Method Example:
- Continuing with the Amazon bicycle scenario, if only a part of the bicycle is broken, such as a wheel, the PATCH method would involve replacing only the broken part, leaving the rest of the bicycle intact.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
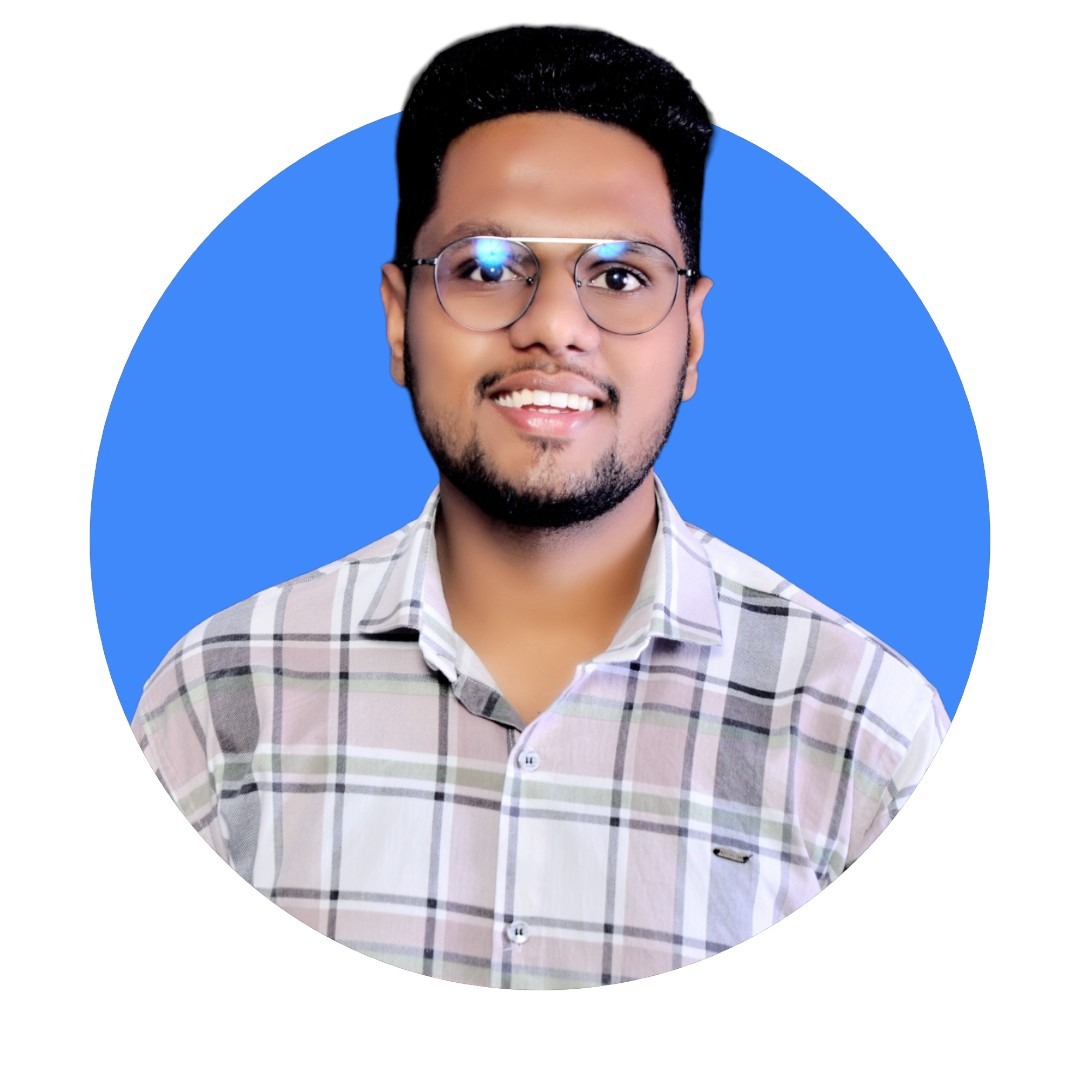
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.