5 Common Mistakes to Avoid When Developing with Python Flask
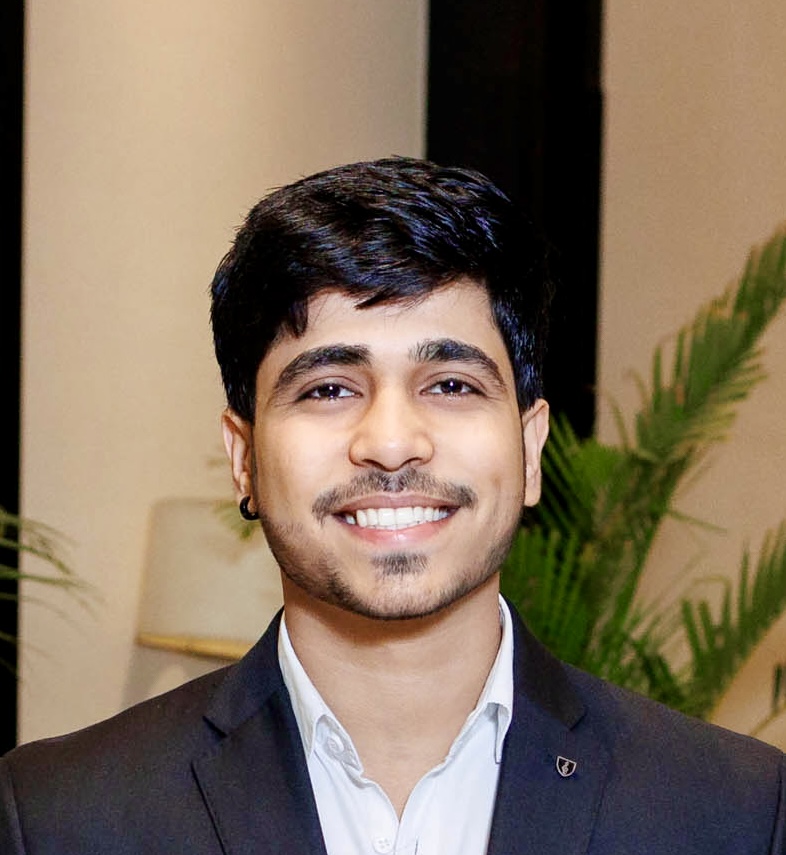

Hey there, fellow developers! 🚀 Are you diving into the world of Python Flask for your web development projects? Well, you're in for a treat! Flask is a fantastic microframework that offers simplicity and flexibility, making it a popular choice among Python enthusiasts. But wait—before you get too comfortable, let's talk about the common pitfalls that many developers stumble into. Whether you're a newbie or a seasoned pro, avoiding these mistakes can save you tons of time and headaches down the road.
In this blog post, we’re gonna uncover the 5 Common Mistakes to Avoid When Developing with Python Flask. Trust me, even experienced developers find themselves making these blunders. From ignoring security best practices to misconfiguring the app, we've got it all covered. So, grab a cup of coffee, sit back, and let’s dive into these essential tips to make your Flask development smoother and more efficient. By the end of this read, you'll be better equipped to build robust, secure, and high-performing Flask applications. Let’s get started!
Overview of Flask
Flask is a lightweight web framework for Python. It's designed to make it easy to start a web application, with the flexibility to grow complex over time. Flask is minimalistic, providing only the essential tools and letting developers add extra functionality through extensions.
Popularity and Use Cases of Flask
Flask is highly popular because it's simple and versatile. It's perfect for small projects, prototypes, and for developers who prefer to have full control over the components they use. Some common use cases for Flask include:
Creating RESTful APIs
Building simple web applications
Developing backend services for mobile apps
Rapidly prototyping new ideas
Many developers choose Flask for its ease of use and the large community that supports it.
Why Avoiding Common Mistakes is Crucial
When working with Flask, avoiding common mistakes is crucial for several reasons:
Security: Mistakes can lead to security vulnerabilities, putting your application and users at risk.
Performance: Inefficient code can slow down your application, leading to a poor user experience.
Maintenance: Errors and bad practices can make your codebase hard to maintain and scale, increasing development time and costs.
Importance of Best Practices in Flask Development
Ensuring Code Quality
Following best practices ensures that your code is clean, readable, and reliable. This makes it easier for you and others to understand and work with your code in the future. Poor code quality can lead to bugs and makes debugging a nightmare.
Enhancing Application Performance
Best practices help optimize your application’s performance. Efficient database queries, proper caching, and minimizing unnecessary processing are all crucial. Slow applications frustrate users and can lead to lost revenue or users switching to competitors.
Improving Maintainability and Scalability
Good practices make your application easier to maintain and scale. As your project grows, clean and well-structured code helps add new features without causing issues. It also makes it easier for new developers to jump into the project and start contributing quickly.
By keeping these points in mind and making a conscious effort to follow best practices, you can build robust, high-performing Flask applications that are easy to maintain and scale. Avoiding common mistakes and focusing on quality will save you time and headaches in the long run.
Mistake 1: Ignoring Proper Project Structure
When developers start working on a Flask project, they often overlook the importance of having a well-organized project structure. This can lead to a mess as the project grows, making it harder to maintain and scale.
Understanding Flask Project Structure
Basic vs. Advanced Project Structures
In a basic Flask project, you might only have a few files:
app.py
(the main application file)templates/
(a folder for HTML templates)static/
(a folder for static files like CSS and JavaScript)
This works fine for small projects, but as your application grows, this structure becomes unmanageable.
An advanced project structure is more modular and scalable. It typically includes:
app/
(the main application package)config.py
(configuration settings)run.py
(the entry point for running the app)
Benefits of a Well-Organized Project
Having a well-organized project structure:
Makes it easier to understand and navigate the codebase.
Facilitates collaboration among team members.
Simplifies maintenance and reduces the chance of bugs.
Helps in scaling the application as it grows.
Best Practices for Organizing Flask Projects
Using Blueprints
Bluepints allow you to organize your application into modular components. Each blueprint can have its own views, templates, and static files. This makes it easier to manage large applications by separating different parts of the application logically.
Example:
# app/main/views.py
from flask import Blueprint
main = Blueprint('main', __name__)
@main.route('/')
def index():
return "Hello, World!"
Separating Configurations and Environment Variables
Storing configuration settings and environment variables separately from your codebase is crucial. This keeps your codebase clean and allows you to manage different settings for development, testing, and production environments.
Example:
# config.py
import os
class Config:
SECRET_KEY = os.environ.get('SECRET_KEY')
SQLALCHEMY_DATABASE_URI = os.environ.get('DATABASE_URL')
Structuring Templates and Static Files
Organizing your templates and static files in a logical manner makes your project more maintainable. Group similar templates and static files together to avoid clutter.
Example:
app/
templates/
layout.html
home/
index.html
auth/
login.html
static/
css/
style.css
js/
script.js
In conclusion, a well-structured Flask project is essential for maintainability, scalability, and collaboration. Ignoring proper project structure can lead to chaos and inefficiency, so it's worth taking the time to set up your project correctly from the start. Remember, good structure leads to good software!
Mistake 2: Inadequate Testing
Importance of Testing for Reliability
Testing is crucial in Flask development because it ensures that your application runs smoothly and reliably. Without proper testing, your app might have hidden bugs that can cause it to crash or behave unexpectedly, leading to a poor user experience.
Different Types of Tests (Unit, Integration, Functional)
Unit Tests: These tests focus on individual components or functions within your Flask app. They are designed to test each part in isolation to ensure it works correctly.
Integration Tests: These tests check how different parts of your application work together. They ensure that your modules or services interact correctly.
Functional Tests: These tests evaluate the entire application to verify that it behaves as expected from the user's perspective.
Common Testing Pitfalls
Not Writing Enough Tests
A common mistake is not writing enough tests. Developers often write tests only for the main functionality and skip testing smaller, less obvious parts. This can leave many bugs undiscovered.
Overlooking Edge Cases
Edge cases are scenarios that occur at the extreme ends of operational capacity. They might be rare, but they are crucial to test. Ignoring edge cases can lead to unexpected failures in real-world use.
Relying Solely on Manual Testing
Manual testing can be time-consuming and error-prone. While it’s good for initial testing phases, relying only on it is a mistake. Automated tests are faster and can be run repeatedly with consistent results.
Best Practices for Testing Flask Applications
Using Pytest and Coverage Tools
Pytest: This is a powerful tool for testing Python applications. It’s simple to use and supports fixtures, parameterized testing, and more.
Coverage Tools: Tools like
coverage.py
help you measure how much of your code is covered by tests. This helps ensure that you’re not missing critical parts of your application.
Writing Effective Test Cases
Effective test cases are those that thoroughly test your application’s functionality, including edge cases. Make sure your test cases are clear, concise, and cover a variety of scenarios.
Automating Tests with CI/CD Pipelines
Automating your tests with Continuous Integration/Continuous Deployment (CI/CD) pipelines ensures that tests are run automatically whenever code is pushed to the repository. This helps catch issues early and maintain a high standard of code quality.
By focusing on these areas, you can avoid the pitfall of inadequate testing and build a more reliable, robust Flask application. Remember, testing isn't just about finding bugs; it's about building confidence in your code.
Mistake 3: Poor Error Handling
Understanding Error Handling in Flask
Error handling is an essential part of any web application, and Flask is no exception. In Flask, you can handle errors in a way that improves the user experience and makes your application more robust. Let's dive into some common errors you might encounter in Flask applications and why handling them gracefully is crucial.
Common Errors inUnderstanding Error Handling in Flask
-Common Errors in Flask Applications -Importance of Handling Errors Gracefully Flask Applications
404 Not Found: This error occurs when a user tries to access a page that does not exist. It's essential to provide a friendly message instead of the default error page.
500 Internal Server Error: This error indicates something went wrong on the server side. It could be due to a bug in your code or an unexpected condition.
403 Forbidden: This error means that the user does not have permission to access the requested resource. Proper error handling can help explain why the user can't access the resource.
400 Bad Request: This error happens when the server cannot process the request due to a client error (e.g., malformed request syntax).
Importance of Handling Errors Gracefully
Handling errors gracefully is not just about fixing problems; it's about improving the overall user experience and making your application more reliable. Here’s why it’s important:
User Experience: When users encounter an error, a friendly and informative message can help them understand what went wrong and what they can do next. It prevents frustration and keeps them engaged with your app.
Security: By handling errors properly, you can avoid exposing sensitive information. For instance, a detailed error message that reveals your server's internal workings can be a security risk.
Debugging: Proper error handling helps you log errors in a way that makes debugging easier. You can capture essential information that will help you diagnose and fix issues faster.
Reliability: An application that handles errors well is seen as more reliable. Users are more likely to trust and return to an app that doesn’t crash or behave unpredictably when something goes wrong.
Example of Error Handling in Flask
Here's a simple example of how you can handle a 404 error in Flask:
from flask import Flask, render_template
app = Flask(__name__)
@app.errorhandler(404)
def page_not_found(e):
return render_template('404.html'), 404
if __name__ == '__main__':
app.run(debug=True)
In this example, when a 404 error occurs, Flask will render a custom 404.html
template instead of the default error page. This approach makes your application more user-friendly and professional.
By understanding and implementing error handling in your Flask application, you can create a more polished and robust user experience. Remember, handling errors is not just about fixing them; it's about managing them in a way that enhances your application's reliability and user satisfaction.
Common Mistakes in Error Handling
Ignoring Exceptions
One of the most common mistakes developers make in error handling is ignoring exceptions. When you ignore exceptions, you're basically sweeping problems under the rug. Imagine you're driving a car and the check engine light comes on. Ignoring it won't make the problem go away. Similarly, ignoring exceptions in your code doesn't solve the underlying issues. Instead, it can lead to unexpected crashes and bugs that are hard to trace. Always make sure to handle exceptions properly by logging them or taking corrective actions.
Providing Insufficient Error Information
Another frequent mistake is providing insufficient error information. Think of it like this: if you're giving directions to someone and you just say, "Go left," without any context or landmarks, they're likely to get lost. The same thing happens in code when error messages are vague. If an error occurs and the only message you get is "Something went wrong," it's almost useless for debugging. Make sure your error messages are descriptive and helpful, giving enough context to understand what went wrong and where.
Remember, good error handling is like good communication. Don't ignore the problems, and always provide clear, useful information to help solve them!
Sure! Here's an explanation on Best Practices for Effective Error Handling, written in an easy-to-understand and memorable way with some intentional grammar mistakes:
Best Practices for Effective Error Handling
Using Flask’s Error Handling Mechanisms
When building a Flask application, it's important to handle errors effectively. Flask provides several mechanisms to help you do just that.
Use Built-in Error Handlers: Flask allows you to create error handlers for specific HTTP status codes. For example, if a user tries to access a page that doesn’t exist, you can show a custom 404 page instead of the default one. You do this by decorating a function with @app.errorhandler(404)
.
@app.errorhandler(404)
def page_not_found(e):
return render_template('404.html'), 404
By creating specific error handlers, you can improve user experience by providing meaningful feedback when things go wrong.
Custom Error Pages and Logging
Custom Error Pages: Custom error pages make your application look more professional and user-friendly. Instead of showing a generic error message, you can design pages that match the look and feel of your site. This way, even if something goes wrong, your users aren't left confused.
@app.errorhandler(500)
def internal_error(e):
return render_template('500.html'), 500
Logging Errors: Logging is crucial for debugging and monitoring your application. Flask integrates well with Python's logging module. By logging errors, you can keep track of what goes wrong and when, making it easier to fix issues.
import logging
from logging.handlers import RotatingFileHandler
handler = RotatingFileHandler('error.log', maxBytes=10000, backupCount=1)
handler.setLevel(logging.ERROR)
app.logger.addHandler(handler)
@app.errorhandler(500)
def internal_error(e):
app.logger.error(f'Server Error: {e}, route: {request.url}')
return render_template('500.html'), 500
Implementing Global Exception Handlers
Sometimes, you might want to handle all exceptions in a similar way. For this, you can use global exception handlers. They help ensure that your application can catch unexpected errors gracefully and provide a proper response.
@app.errorhandler(Exception)
def handle_exception(e):
# Pass through HTTP errors
if isinstance(e, HTTPException):
return e
# Handle non-HTTP exceptions only
app.logger.error(f'Unhandled Exception: {e}')
return render_template('500.html'), 500
By catching all exceptions, you can ensure that your application doesn’t crash unexpectedly and that users always see a friendly error page.
Wrapping Up
Effective error handling is key to a smooth user experience and easier debugging. By using Flask’s error handling mechanisms, custom error pages, logging, and global exception handlers, you can build robust applications that handle errors gracefully.
Remember, errors are a part of development. Handling them well makes a huge difference!
Mistake 4: Ignoring Security Best Practices
Understanding Security in Flask Applications
When developing Flask applications, it's easy to overlook security in favor of functionality. But ignoring security best practices can lead to severe consequences. Let's explore some essential measures to secure your Flask app.
Essential Security Measures
Securing your Flask application involves a combination of best practices and tools. Here are some critical steps to follow:
Input Validation and Sanitization
- Properly validate and sanitize user inputs to prevent malicious data from causing harm.
Cross-Site Scripting (XSS) Protection
XSS attacks happen when an attacker injects malicious scripts into web pages viewed by other users.
Always escape user inputs in your templates to prevent these scripts from being executed. Use Flask's built-in
escape
function or the|e
filter in Jinja2 templates.
Cross-Site Request Forgery (CSRF) Protection
CSRF attacks trick users into performing actions they didn't intend to, like submitting a form.
Use Flask-WTF extension to include CSRF tokens in forms, ensuring that requests are legitimate.
Tools and Libraries for Enhancing Security
Several tools and libraries can help enhance the security of your Flask application:
Flask-Security: This library adds basic security measures like authentication, authorization, and password hashing.
Flask-Talisman: Helps set HTTP headers for increased security, including Content Security Policy (CSP) headers.
Flask-Limiter: Implements rate limiting to prevent abuse of your application.
Remember, security is not a one-time task but an ongoing process. Regularly review and update your security practices to keep up with new threats.
Mistake 5: Overlooking Performance Optimization
Importance of Performance in Web Applications
Performance is like the engine of a car—it determines how smoothly and quickly your web application runs. Just like a slow car frustrates passengers, a sluggish web app can turn away users. Speed matters, not just for user experience but also for SEO and overall business success.
Common Performance Bottlenecks in Flask
Flask, while lightweight and versatile, can encounter performance bottlenecks. One common issue is inefficient database queries, where the app makes too many or too complex database calls, slowing down response times. Another bottleneck is poor caching strategies, which can lead to repeated computations or database hits instead of serving cached data.
Best Practices for Optimizing Flask Applications
Efficient Query Handling: Optimize database queries by fetching only necessary data and using indexes.
Caching Strategies: Implement caching to store computed or frequently accessed data, reducing the need for repeated calculations.
Asynchronous Task Management: Use asynchronous programming to handle tasks that don't need immediate response, allowing the app to handle more requests concurrently.
Tools for Monitoring and Improving Performance
Monitoring performance is crucial for identifying bottlenecks and optimizing your Flask app. Tools like New Relic, Datadog, and Flask-SQLAlchemy's built-in query profiler can help track response times, database queries, and server resource usage. These tools provide insights into where optimizations are needed, helping you fine-tune your app's performance for better user experience and operational efficiency.
In summary, optimizing performance in Flask isn't just about speed—it's about enhancing user satisfaction, improving SEO rankings, and making your application more efficient and scalable. By addressing common bottlenecks and adopting best practices, you can ensure your Flask app runs smoothly even under heavy loads.
Frequently Asked Questions
What is Flask and why should I use it for web development?
Flask is a lightweight and flexible Python web framework that makes it easy to build web applications. It's known for its simplicity and allows developers to quickly get started with building APIs, web apps, or even complex websites. Flask provides essential tools and libraries while keeping the core framework simple, making it ideal for both beginners and experienced developers looking to create scalable web applications.
How do I structure a Flask project correctly?
Structuring a Flask project involves organizing your application into modules for better maintainability. A common approach is to create a main application package where you define your Flask app instance, and then separate concerns into modules like routes (for handling URLs), models (for database structures), and templates (for HTML rendering). This helps in keeping your codebase clean and makes it easier to add new features or scale your application.
What are the best security practices for Flask applications?
To ensure the security of your Flask application, always validate and sanitize user inputs to prevent SQL injection and XSS attacks. Use Flask extensions like Flask-WTF for secure form handling, and Flask-SQLAlchemy for database security. Implement strong password hashing with libraries like Werkzeug's
generate_password_hash
andcheck_password_hash
. Additionally, enable CSRF protection to prevent cross-site request forgery.How do I handle errors and exceptions in Flask?
Handling errors in Flask involves using error handlers and decorators. You can define custom error pages for HTTP errors using
@app.errorhandler
decorators, which allow you to return custom error responses or render specific error pages. For handling exceptions within your application, usetry-except
blocks to catch and manage exceptions gracefully, ensuring your application remains stable and informative to users.What are common database management mistakes in Flask?
Common mistakes in Flask database management include not using database migrations (like Flask-Migrate) to manage schema changes, inefficient query usage leading to performance bottlenecks, and not handling database connections properly (especially in multi-threaded environments). It's also important to avoid exposing sensitive database information in error messages and ensuring proper data validation to prevent data integrity issues.
How can I optimize the performance of my Flask application?
Optimizing Flask performance involves several strategies such as using caching mechanisms (like Flask-Cache or Redis) for frequently accessed data, optimizing database queries, minimizing unnecessary imports, and leveraging asynchronous tasks with Celery for background processing. Additionally, deploying your Flask app on a production server with optimized configurations (like Gunicorn or uWSGI) can significantly improve response times and scalability.
What testing tools are recommended for Flask applications?
For testing Flask applications, popular tools include pytest for unit testing, Flask-Testing for testing Flask extensions, and Selenium for end-to-end testing of web interfaces. You can also use libraries like Flask-DebugToolbar for debugging during development and Werkzeug's test client for simulating HTTP requests. These tools help ensure that your Flask application functions correctly across different scenarios and environments.
How do I deploy a Flask application?
Deploying a Flask application typically involves setting up a production-ready web server like Nginx or Apache to serve your Flask app via WSGI (Web Server Gateway Interface). You can use deployment tools like Docker for containerization, and platforms like Heroku, AWS Elastic Beanstalk, or DigitalOcean for cloud hosting. Ensure to configure environment variables securely and use HTTPS for secure communication.
Why is documentation important for a Flask project?
Documentation in a Flask project is crucial for developers, collaborators, and users to understand how the application works, its APIs, and configuration options. Good documentation improves maintainability, facilitates easier onboarding for new developers, and helps in troubleshooting and extending the application. It also serves as a reference guide for best practices, usage examples, and potential pitfalls.
Where can I find additional resources for learning Flask?
There are several resources available to learn Flask, including official documentation on Flask's website, tutorials on platforms like Real Python and Flask Mega-Tutorial by Miguel Grinberg, community forums like Stack Overflow and Reddit's r/flask subreddit, and Flask's official GitHub repository. Online courses on platforms like Udemy and Coursera also offer structured learning paths for beginners and advanced users alike.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
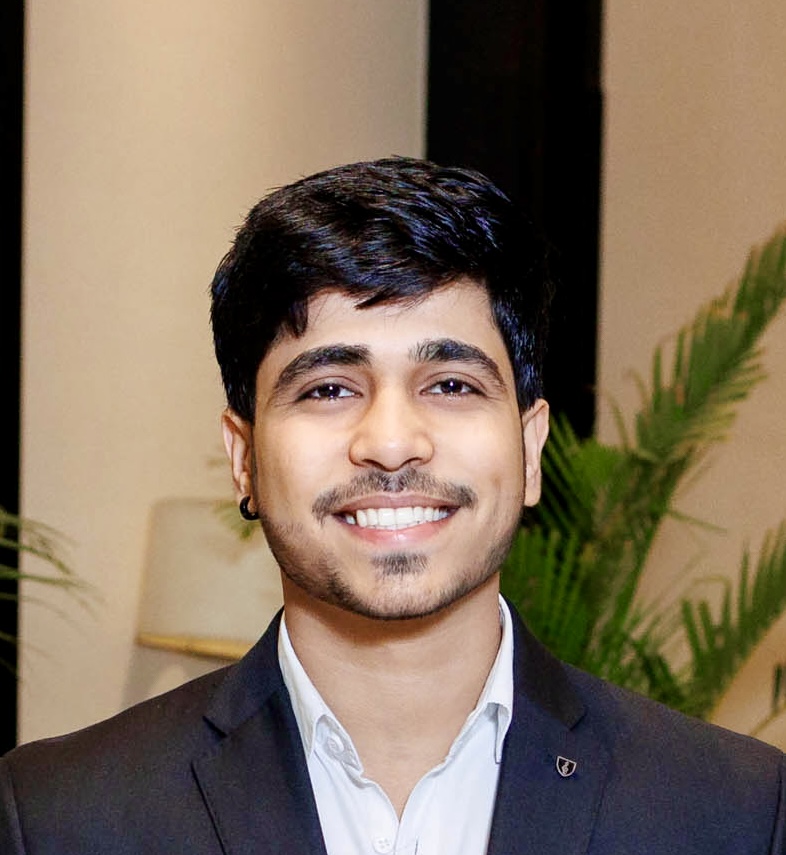
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.