10 Python Function Practice Exercises for Beginners
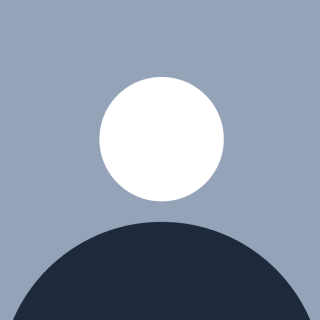
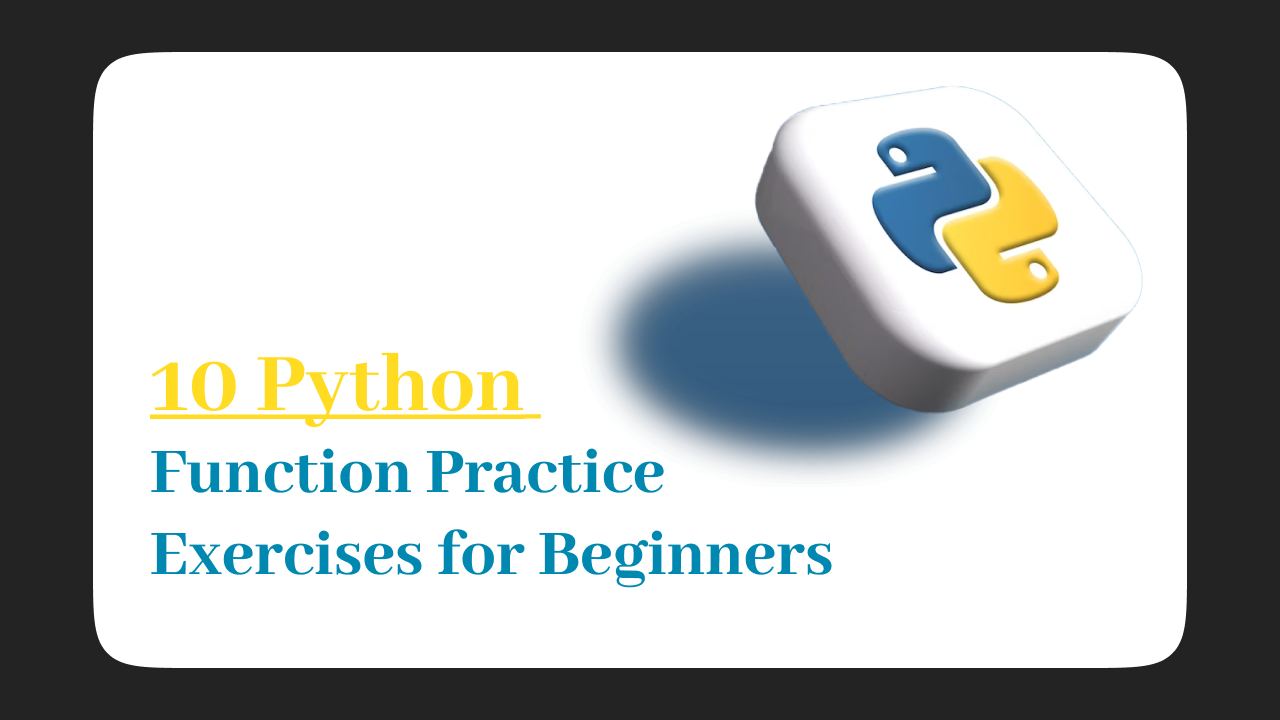
You are a new user of Python, wanting to make your grip tighter; practice in the field of functions. Ready to jack up that dial on your Python skills? Mastering Python can be achieved by working on its functions. These let you modularize your code into bits of calling and execution for repetitive actions so that you wouldn't have to rewrite them all over again.
Here are ten practice exercises that can help improve your skills in Python functions. If you need professional help or want to hire dedicated Python developers, outsource to a reputed Python development company in India for top-notch Python development services.
How Python Functions Work:
Before diving into the exercises, here's a quick refresher on defining a Python function:
Keyword def: This starts the function definition.
Function Name: Choose a descriptive name for your function.
Parameters: Place parameters inside parentheses (leave them empty if there are no parameters).
Colon (:): Indicates the start of the function body.
Indentation: Write the steps of the function indented under the definition line.
Return Value: Optionally, return a value using the return keyword.
def function_name(parameter1, parameter2):
Function body
return result
Exercise 1. Printing of a Sentence Multiple Times
Task: Create a Python function that will request the user to input a number and print "Hello, Python!" many times.
Solution:
def print_sentence_multiple_times():
num = int(input("Enter a number: "))
for _ in range(num):
print("Hello, Python!")
print_sentence_multiple_times()
Explanation: The following function takes a number from the user with the input(), it converts the provided number to an integer, and then uses a for loop to print out "Hello, Python!" as many times.
Exercise 2: Counting Upper and Lower Characters
Task: Write a Python function that takes a string as an argument and returns a dictionary with counts of uppercase, lowercase, and other characters.
Solution:
def count_upper_lower_chars(input_string):
char_count = {"uppercase": 0, "lowercase": 0, "other": 0}
for char in input_string:
if char.isupper():
char_count["uppercase"] += 1
elif char.islower():
char_count["lowercase"] += 1
else:
char_count["other"] += 1
return char_count
sentence = "Hello, Python! How are you?"
result = count_upper_lower_chars(sentence)
print(result)
Explanation: This function will take each character in the string, test if it is in uppercase, if not test whether it is in lowercase, and finally increment the count of the found character type in the dictionary.
Exercise 3. Find the Shortest and Longest Words
Write a Python function that accepts a list of strings and returns a tuple containing the shortest and longest word.
Solution:
def shortest_longest_words(words_list):
shortest_word = min(words_list, key=len)
longest_word = max(words_list, key=len)
return shortest_word, longest_word
words = ["apple", "banana", "kiwi", "grapefruit", "orange"]
result = shortest_longest_words(words)
print(result)
Explanation: A function was created that used the functions min and max with the key = len to receive the shortest and the longest words in the list.
Exercise 4: Check Before You Append
Task: Develop a Python function that takes a list and an element as an argument. The function should add the element to the list top if it doesn't exist there.
Solution:
def add_unique_element(input_list, element):
if element not in input_list:
input_list.append(element)
my_list = ["apple", "banana", "kiwi"]
add_unique_element(my_list, "banana")
add_unique_element(my_list, "orange")
print(my_list)
This function checks whether an element is in the list before it appends it and, therefore, does not allow for duplicates.
Exercise 5: Removing Duplicates and Sorting
Objective: Write a Python function that removes duplicates from a given list of strings and returns the sorted list of unique elements.
Solution:
def remove_duplicates_and_sort(input_list):
unique_sorted_list = sorted(set(input_list))
return unique_sorted_list
input_list = ["apple","banana","kiwi","banana","orange","apple"]
result = remove_duplicates_and_sort(input_list)
print(result)
This function converts the list to a set to remove all duplicate entries, sorts this set, and then returns it as a list.
Exercise 6: Second Occurrence
Problem: Write a Python function that takes a list and an element, and returns the 0-based index of the second element in the list. If not found, return -1.
Solution:
def second_occurrence_index(input_list, element):
occurrences = [index for index, value in enumerate(input_list) if value == element]
return occurrences[1] if len(occurrences) > 1 else -1
my_list = ["apple", "banana", "kiwi", "banana", "orange", "banana"]
element = "banana"
index = second_occurrence_index(my_list, element)
print(f"Index of the second occurrence of {element}: {index}")
Explanation: This function used list comprehension to find the 0-based index of the second element, or -1 if the element is not found.
Exercise 7: Non-Negative Integers
Task: Write a Python function returning a sorted list of the nonnegative numbers from the input list.
Solution:
def sort_non_negative_numbers(input_list):
non_negative_numbers = [num for num in input_list if num >= 0]
return sorted(non_negative_numbers)
numbers = [5, -3, 0, 9, -2, 7, -1, 4]
result = sort_non_negative_numbers(numbers)
print(result)
Explanation: This function filters out the negative numbers and sorts the remaining nonnegative numbers in ascending order.
Exercise 8: Word Value
Task: Write a Python function, which takes as an argument a word, and returns the value of that word according to the following point rules for consonants, vowels, and 'x'.
Solution:
def calculate_word_value(word):
value = 0
for char in word:
if char.lower() in 'aeiou':
value += 3
elif char.lower() == 'x':
value += 10
elif char.isalpha():
value += 1
return value
word = "Python"
result = calculate_word_value(word)
print(result)
Description: The function loops through each character in the word and therefore awards several points depending on the type of character and adds them to the total value.
Exercise 9. Shifting Alphabet Characters
Write a function in Python, which moves every character one position in an alphabet.
Solution:
def shift_characters(input_string):
alphabet = 'abcdefghijklmnopqrstuvwxyz'
shifted_string = ""
for char in input_string:
if char.isalpha():
shifted_char_idx = (alphabet.index(char.lower()) + 1) % 26
shifted_char = alphabet[shifted_char_idx]
shifted_string += shifted_char.upper() if char.isupper() else shifted_char
else:
shifted_string += char
return shifted_string
sentence = "Hello, Python!"
result = shift_characters(sentence)
print(result)
Description: The function shifts a string forward one position in the alphabet for every character while keeping the case for letters and other characters as they were.
Exercise 10: Caesar Cipher
Extend the previous function so that it accepts an arbitrary shift value. That is, create a Caesar cipher.
Solution:
def caesar_cipher(input_string, jump):
alphabet = 'abcdefghijklmnopqrstuvwxyz'
shifted_string = ""
for char in input_string:
if char.isalpha():
shifted_char_idx = (alphabet.index(char.lower()) + jump) % 26
shifted_char = alphabet[shifted_char_idx]
shifted_string += shifted_char.upper() if char.isupper() else shifted_char
else:
shifted_string += char
return shifted_string
input_str = "Hello, Python!"
jump = 3
result = caesar_cipher(input_str, jump)
print(result)
Description: Generalizing shifting logic so that any value of this argument will create a proper Caesar cipher.
Final words:
Practice of Python functions through practical exercises is an excellent means to confirm any of the learned material. Such practice exercises are given, which range from basic string manipulation to simple cryptographic manipulation with a Caesar cipher. This wide spectrum of exercises will thus test a fresh beginner who is looking to hold onto basic concepts—either students at the very beginning of their programming career or those seeking to improve their skills.
Enrich your skills in Python with our Python development services. Tuvoc Technologies – Learn more about. Hire Python development services to bring out the programmer within you by connecting with us today! Happy coding!
Subscribe to my newsletter
Read articles from Thomas Adman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Thomas Adman
Thomas Adman
Tuvoc Technologies began with a passion for growth, initially without a clear direction. However, leveraging our robust management background and an open mindset for exploration, we embarked on our journey in the B2B industry.