Day 15: Basics of Python for DevOps Engineers
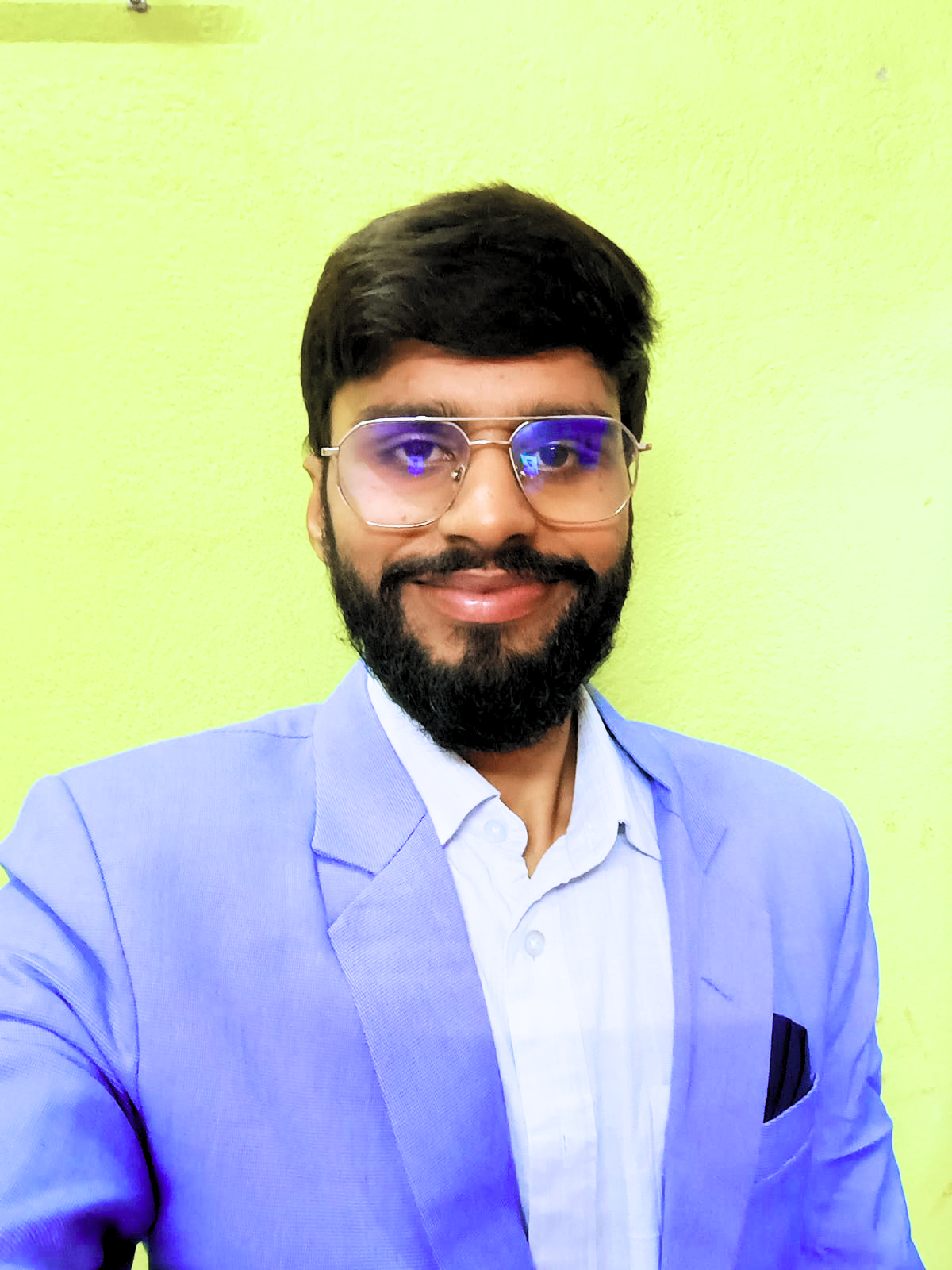

Hlo doston,
Let's start with the basics of Python, as this is also important for DevOps Engineers to build logic and programs.
What is Python?
Python is an open-source, general-purpose, high-level, and object-oriented programming language. It was created by Guido van Rossum. Python consists of vast libraries and various frameworks like Django, TensorFlow, Flask, Pandas, Keras, etc.
How to Install Python?
You can install Python on your system, whether it is Windows, macOS, Ubuntu, CentOS, etc. Below are the links for the installation:
Windows Installation: Python for Windows
Ubuntu Installation: Open your terminal and type:
bashCopy codesudo apt-get install python3.6
Tasks:
Task 1:
Install Python on your respective OS, and check the version.
Windows:
Download Python:
Open a web browser and go to the official Python website.
Select "Download Python 3.x.x" (where x.x is the latest version).
Run the Installer:
Locate the downloaded file (usually in the "Downloads" folder) and double-click to run the installer.
Important: Check the box that says "Add Python to PATH" at the bottom of the installer window.
Click "Install Now".
Installation Progress:
The installer will now install Python and all necessary files.
Once the installation is complete, click "Close".
Verify Installation:
Open Command Prompt by pressing
Win + R
, typingcmd
, and pressing Enter.Type
python --version
and press Enter.You should see the Python version number displayed, confirming the installation.
macOS:
Download Python:
Open a web browser and go to the official Python website.
Select "Download Python 3.x.x" (where x.x is the latest version) for macOS.
-
Locate the downloaded
.pkg
file (usually in the "Downloads" folder) and double-click to open it.Follow the installation prompts, clicking "Continue" and "Install" as needed.
-
Open Terminal by pressing
Cmd + Space
, typingTerminal
, and pressing Enter.Type
python3 --version
and press Enter.You should see the Python version number displayed, confirming the installation.
Linux (Ubuntu/Debian):
Update Package List:
Open Terminal by pressing
Ctrl
+ Alt + T
.
-
Run the command:
sudo ap
t install python3
and press Enter.
Verify Installation:
Type
python3 --version
and press Enter.You should see the Python version number displayed, confirming the installation.
Linux (CentOS/RHEL):
Update Package List:
Open Terminal.
Run the command:
sudo yum update
and press Enter.
-
Run the command:
sudo yu
m install python3
and press Enter.
Verify Installation:
Type
python3 --version
and press Enter.You should see the Python version number displayed, confirming the installation.
Read about different data types in Python.
Read about different data types in Python.
Python supports several built-in data types. Understanding these data types is essential for writing efficient and effective Python code. Here's a comprehensive overview of the different data types in Python:
1. Numeric Types
a. Integer (int)
Description: Represents whole numbers.
Example:
x = 10
b. Float (float)
Description: Represents floating-point numbers (numbers with a decimal point).
Example:
x = 10.5
c. Complex (complex)
Description: Represents complex numbers, which have a real and an imaginary part.
Example:
x = 3 + 5j
2. Sequence Types
a. String (str)
Description: Represents a sequence of characters.
Example:
x = "Hello, World!"
b. List (list)
Description: Ordered, mutable collection of items, which can be of different types.
Example:
x = [1, 2, 3, "apple", 4.5]
c. Tuple (tuple)
Description: Ordered, immutable collection of items.
Example:
x = (1, 2, 3, "apple", 4.5)
3. Set Types
a. Set (set)
Description: Unordered collection of unique items.
Example:
x = {1, 2, 3, "apple", 4.5}
b. Frozenset (frozenset)
Description: Immutable version of a set.
Example:
x = frozenset([1, 2, 3, "apple", 4.5])
4. Mapping Type
a. Dictionary (dict)
Description: Unordered collection of key-value pairs.
Example:
x = {"name": "Alice", "age": 25, "city": "New York"}
5. Boolean Type
a. Boolean (bool)
Description: Represents one of two values:
True
orFalse
.Example:
x = True
6. Binary Types
a. Bytes (bytes)
Description: Immutable sequence of bytes.
Example:
x = b"Hello"
b. Bytearray (bytearray)
Description: Mutable sequence of bytes.
Example:
x = bytearray(5)
c. Memoryview (memoryview)
Description: Provides memory-efficient slicing of binary data.
Example:
x = memoryview(b"Hello")
Examples of Usage
# Integer
a = 10
# Float
b = 20.5
# Complex
c = 1 + 2j
# String
d = "Hello, Python!"
# List
e = [1, 2, 3, "apple", 4.5]
# Tuple
f = (1, 2, 3, "apple", 4.5)
# Set
g = {1, 2, 3, "apple", 4.5}
# Frozenset
h = frozenset([1, 2, 3, "apple", 4.5])
# Dictionary
i = {"name": "Alice", "age": 25, "city": "New York"}
# Boolean
j = True
# Bytes
k = b"Hello"
# Bytearray
l = bytearray(5)
# Memoryview
m = memoryview(b"Hello")
Understanding these data types and their properties is crucial for effective programming in Python. Each type has its own methods and operations that can be performed, allowing you to manipulate and work with data efficiently.
Conclusion:
Python is a powerful language that can simplify many tasks in DevOps, from automation scripts to complex data analysis. Understanding the basics of Python will help you in writing efficient code and automating tasks effectively.
Real-life Example:
Imagine you have a task to monitor server logs for errors. You could write a Python script that reads the log file, searches for error patterns, and sends an email notification if any errors are found. This is just one of the many ways Python can make a DevOps engineer's life easier by automating routine tasks.
Keep learning and stay tuned for more updates on our 90-day DevOps journey!
Reference
For a visual guide, you can watch this YouTube video by Shubham Londhe Sir.
Connect and Follow Me on Socials
Subscribe to my newsletter
Read articles from priyadarshi ranjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
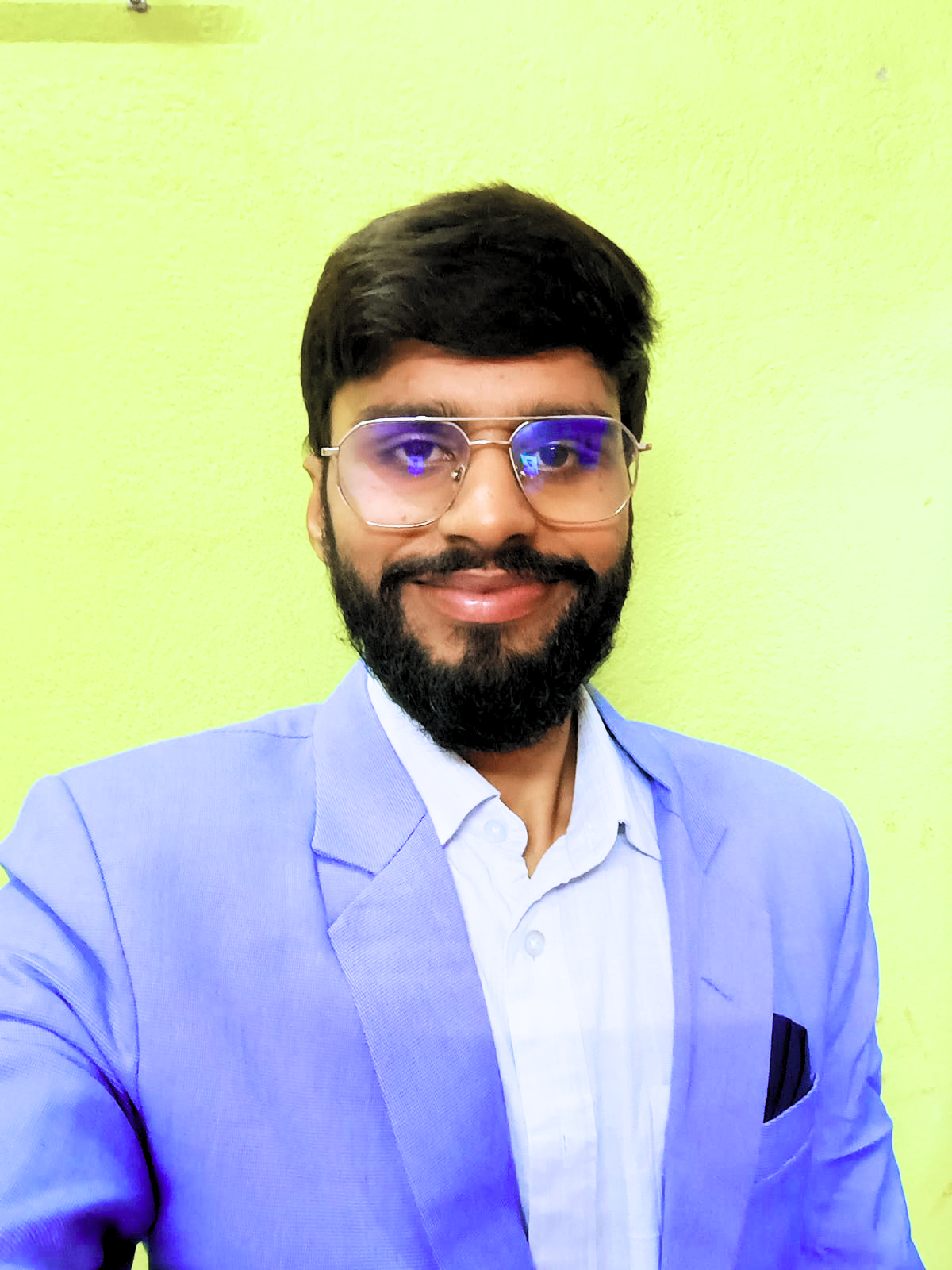
priyadarshi ranjan
priyadarshi ranjan
Greetings! ๐ I'm Priyadarshi Ranjan, a dedicated DevOps Engineer embarking on an enriching journey. Join me as I delve into the dynamic realms of cloud computing and DevOps through insightful blogs and updates. ๐ ๏ธ My focus? Harnessing AWS services, optimizing CI/CD pipelines, and mastering infrastructure as code. Whether you're peers, interns, or curious learners, let's thrive together in the vibrant DevOps ecosystem. ๐ Connect with me for engaging discussions, shared insights, and mutual growth opportunities. Let's embrace the learning curve and excel in the dynamic realm of AWS and DevOps technology!