Boost React Performance with Debouncing and Throttling

Table of contents
- Introduction
- Basics of Debouncing and Throttling
- Practical Applications in React
- Implementing Debouncing in React
- Implementing Throttling in React
- Case Studies and Real-World Examples
- Handling Edge Cases
- Best Practices
- Performance Monitoring and Testing
- Common Pitfalls and How to Avoid Them
- Advanced Techniques
- Tools and Libraries
- Debugging and Profiling
- Conclusion
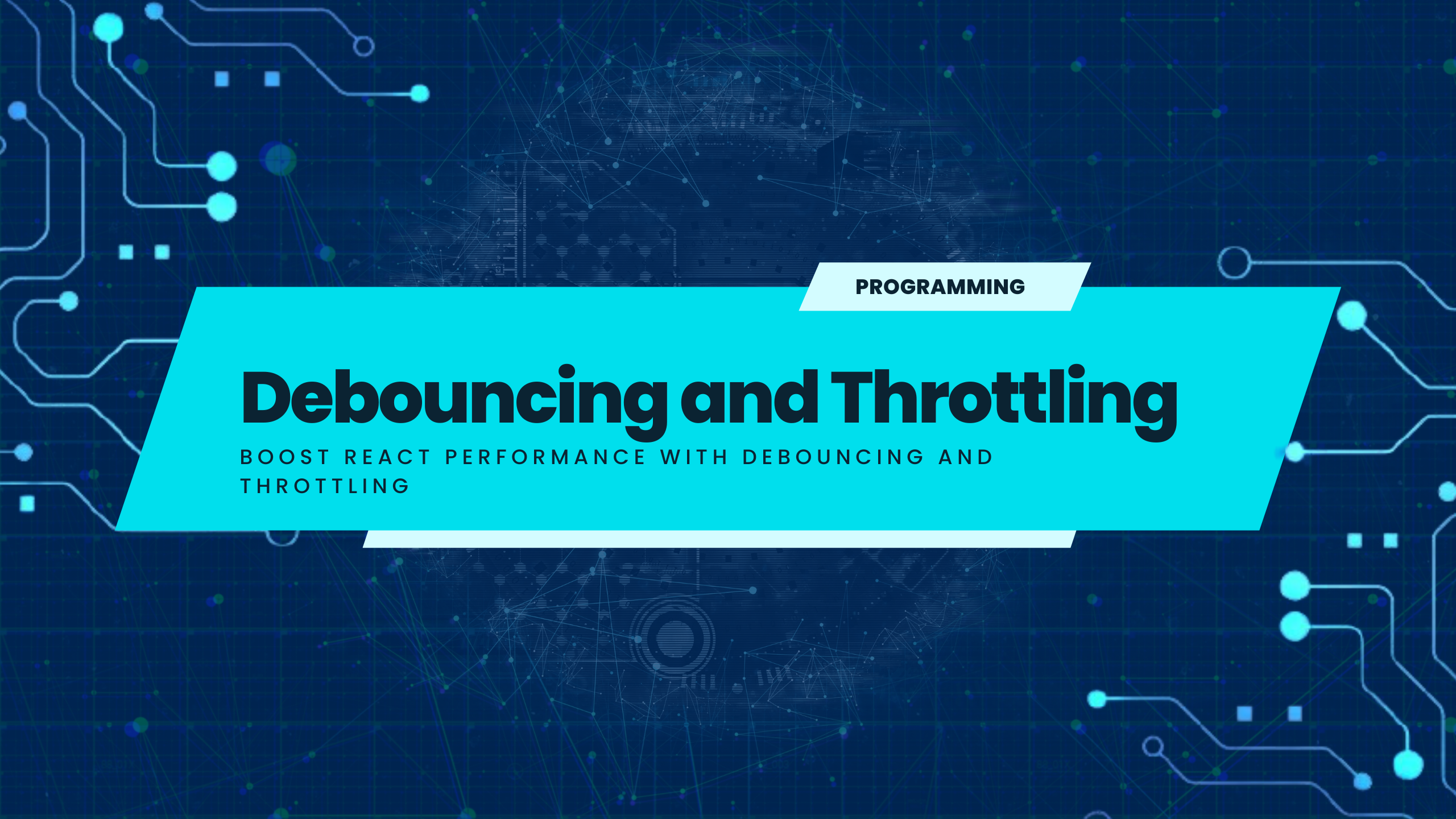
Introduction
In the realm of web development, particularly with React, performance optimization is paramount. Applications that respond sluggishly or fail to handle user interactions efficiently can lead to a poor user experience, ultimately affecting user retention and satisfaction. React, with its robust component-based architecture, offers various strategies for enhancing performance. Among these strategies, debouncing and throttling stand out as crucial techniques for managing function execution frequency. These methods are particularly beneficial when dealing with high-frequency events such as keystrokes, window resizing, or scrolling.
Understanding React Performance Bottlenecks
Performance bottlenecks in React applications often arise from excessive re-renders, unoptimized state management, and heavy computations. High-frequency events like typing in a search box or scrolling a long list can trigger a cascade of renders, leading to noticeable lag. Addressing these bottlenecks requires a deep understanding of how and when React updates the DOM, and how to minimize unnecessary updates.
Why Debouncing and Throttling Are Important
Debouncing and throttling are essential because they help control the rate at which functions are executed. By managing this execution, developers can ensure that their applications remain responsive and efficient, even under heavy user interaction. These techniques are especially important in modern single-page applications where real-time feedback and interaction are crucial.
Basics of Debouncing and Throttling
What is Debouncing?
Debouncing is a programming practice used to ensure that time-consuming tasks do not fire so often. It consolidates multiple successive calls into a single call. For instance, in the context of a search input field, debouncing can be used to delay the execution of the search function until the user has stopped typing for a certain period. This avoids sending a search request for every keystroke, thus reducing unnecessary server calls and improving application performance.
What is Throttling?
Throttling, on the other hand, ensures that a function is called at most once in a specified period. Unlike debouncing, which only executes a function after a series of rapid events have ceased, throttling executes the function at regular intervals. This is particularly useful for actions like window resizing or scrolling, where you want to ensure a function executes regularly but not too frequently.
Key Differences Between Debouncing and Throttling
The primary difference between debouncing and throttling lies in their approach to handling rapid events. Debouncing delays the execution until the event flow has paused, while throttling guarantees regular execution at specified intervals, regardless of how many times the event is triggered.
Practical Applications in React
When to Use Debouncing in React
Debouncing is ideal for scenarios where you want to limit the rate of function execution based on a series of rapid events. This includes:
Search Inputs: Debouncing a search input function to avoid sending a request for each keystroke.
Form Validations: Delaying form validation logic until the user has stopped typing.
When to Use Throttling in React
Throttling is better suited for situations where you need regular updates but want to limit the frequency of these updates. This includes:
Scroll Events: Throttling a scroll event listener to improve performance.
Window Resizing: Ensuring a resize handler does not execute too frequently.
Implementing Debouncing in React
Basic Debouncing Example in React
A basic debouncing example in React involves using a timeout function to delay the execution of a handler. For instance, in a search component, you can use setTimeout
to postpone the execution of the search function until the user stops typing.
function SearchComponent() {
const [query, setQuery] = useState('');
const handleSearch = (event) => {
const value = event.target.value;
clearTimeout(debounceTimeout);
const debounceTimeout = setTimeout(() => {
setQuery(value);
}, 300);
};
return <input type="text" onChange={handleSearch} />;
}
Using Lodash for Debouncing
Lodash, a popular utility library, provides a debounce
function that simplifies debouncing in React. By using Lodash, you can create a debounced version of a function without manually managing timeouts.
import { debounce } from 'lodash';
function SearchComponent() {
const [query, setQuery] = useState('');
const handleSearch = debounce((event) => {
setQuery(event.target.value);
}, 300);
return <input type="text" onChange={handleSearch} />;
}
Custom Debounce Hook: Step-by-Step Guide
Creating a custom debounce hook in React enhances code reuse and modularity. This hook encapsulates the debouncing logic, allowing you to easily apply it to various components.
import { useState, useEffect } from 'react';
function useDebounce(value, delay) {
const [debouncedValue, setDebouncedValue] = useState(value);
useEffect(() => {
const handler = setTimeout(() => {
setDebouncedValue(value);
}, delay);
return () => {
clearTimeout(handler);
};
}, [value, delay]);
return debouncedValue;
}
Implementing Throttling in React
Basic Throttling Example in React
Implementing throttling in React involves using a flag or a timestamp to control function execution frequency. For example, in a scroll event handler, you can use a timestamp to ensure the function executes at most once per specified interval.
function ScrollComponent() {
const [scrollPosition, setScrollPosition] = useState(0);
const handleScroll = () => {
const currentTime = Date.now();
if (currentTime - lastExecutionTime >= 200) {
setScrollPosition(window.scrollY);
lastExecutionTime = currentTime;
}
};
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => {
window.removeEventListener('scroll', handleScroll);
};
}, []);
return <div>Scroll Position: {scrollPosition}</div>;
}
Using Lodash for Throttling
Lodash also provides a throttle
function that simplifies throttling in React. This function creates a throttled version of a function that only executes at most once per specified interval.
import { throttle } from 'lodash';
function ScrollComponent() {
const [scrollPosition, setScrollPosition] = useState(0);
const handleScroll = throttle(() => {
setScrollPosition(window.scrollY);
}, 200);
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => {
window.removeEventListener('scroll', handleScroll);
};
}, []);
return <div>Scroll Position: {scrollPosition}</div>;
}
Custom Throttle Hook: Step-by-Step Guide
Creating a custom throttle hook allows for consistent throttling across different components. This hook encapsulates the throttling logic, improving code maintainability.
import { useRef } from 'react';
function useThrottle(callback, delay) {
const lastExecutionTime = useRef(Date.now());
return (...args) => {
const currentTime = Date.now();
if (currentTime - lastExecutionTime.current >= delay) {
callback(...args);
lastExecutionTime.current = currentTime;
}
};
}
Case Studies and Real-World Examples
Debouncing in Search Input Fields
In search input fields, debouncing prevents excessive API calls by delaying the search function until the user has stopped typing. This improves both performance and user experience by reducing server load and providing more relevant search results.
Throttling in Scroll Events
Throttling scroll events ensures that heavy computations triggered by scrolling, such as infinite scrolling or parallax effects, do not degrade performance. This maintains smooth scrolling and responsive UI.
Combining Debouncing and Throttling for Optimal Performance
In complex applications, combining debouncing and throttling can yield optimal performance. For example, debouncing user input while throttling window resize events ensures efficient function execution without overwhelming the browser.
Handling Edge Cases
Debouncing with Immediate Execution
In some scenarios, you may need to execute a debounced function immediately on the first call and then delay subsequent calls. This approach is useful for providing instant feedback while still limiting the frequency of function execution.
import { debounce } from 'lodash';
const debouncedFunction = debounce((input) => {
console.log(input);
}, 300, { leading: true, trailing: false });
Throttling with Leading and Trailing Options
Throttling with leading and trailing options ensures that a function executes at both the start and end of a throttling interval. This is beneficial for scenarios where immediate response and final state update are both important.
import { throttle } from 'lodash';
const throttledFunction = throttle((input) => {
console.log(input);
}, 200, { leading: true, trailing: true });
Best Practices
Choosing Between Debouncing and Throttling
Choosing between debouncing and throttling depends on the specific use case. Debouncing is ideal for scenarios where the final action is more important than the intermediate actions, such as form submission. Throttling is better suited for continuous actions like scrolling or resizing.
Balancing Performance and User Experience
Balancing performance and user experience requires careful consideration of delay intervals and function execution frequency. Setting appropriate delays ensures that applications remain responsive without sacrificing performance.
Performance Monitoring and Testing
Measuring React Performance Before and After
To measure the impact of debouncing and throttling, compare application performance before and after implementation. Use metrics such as render times, API call frequency, and user interaction latency to quantify improvements.
Using React DevTools for Performance Analysis
React DevTools provides insights into component rendering and performance. Use it to identify unnecessary re-renders and measure the effectiveness of debouncing and throttling in optimizing performance.
Common Pitfalls and How to Avoid Them
Overusing Debouncing and Throttling
Overusing debouncing and throttling can lead to delayed user interactions and a sluggish application. Apply these techniques judiciously to avoid compromising user experience.
Incorrect Implementation and Its Consequences
Incorrect implementation of debouncing and throttling can result in missed events or excessive function executions. Carefully test and validate implementations to ensure desired behavior.
Advanced Techniques
Combining Debouncing, Throttling, and RequestAnimationFrame
Combining debouncing, throttling, and requestAnimationFrame
can further optimize performance for animations and complex interactions. This approach ensures smooth animations and efficient function execution.
Optimizing React Performance with useMemo and useCallback
In addition to debouncing and throttling, use useMemo
and useCallback
to optimize React performance. These hooks memoize expensive computations and function references, reducing unnecessary re-renders.
Tools and Libraries
Overview of Lodash Functions for Debouncing and Throttling
Lodash provides robust debouncing and throttling functions that simplify implementation. Explore Lodash's debounce
and throttle
functions to leverage these techniques in your React applications.
Exploring Other Libraries: Underscore, RxJS
Other libraries like Underscore and RxJS also offer debouncing and throttling utilities. Evaluate these alternatives to find the best fit for your project requirements.
Debugging and Profiling
Debugging Debounce and Throttle Functions
Debugging debounced and throttled functions involves understanding their execution flow and timing. Use console logs and breakpoints to trace function calls and ensure correct behavior.
Profiling Performance with Chrome DevTools
Chrome DevTools provides comprehensive profiling tools to analyze application performance. Use the Performance panel to profile JavaScript execution and validate the impact of debouncing and throttling.
Conclusion
Recap of Key Concepts
In summary, debouncing and throttling are powerful techniques for optimizing React application performance. By controlling function execution frequency, these methods enhance responsiveness and efficiency.
The Future of Performance Optimization in React
As React continues to evolve, new optimization techniques and best practices will emerge. Stay informed about the latest advancements to maintain high-performing React applications.
Further Reading and Resources for React Developers
For further reading, explore resources such as the React documentation, performance optimization guides, and articles on advanced React techniques. These materials provide deeper insights into debouncing, throttling, and other performance optimization strategies.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
๐ Hi there! I'm Manvendra, a tech enthusiast with a knack for turning ideas into reality. I love making cool stuff that makes life easier and a lot more fun. ๐ง My toolbox is packed with skills in WordPress, and Iโm always on the lookout for the next exciting project to dive into. I thrive in environments where innovation meets practicality, and I enjoy collaborating with like-minded techies who share my passion for creativity. ๐ Apart from that you can find me exploring new places, soaking in different cultures, and satisfying my inner foodie. From street food stalls in Delhi to fine dining in Bengaluru, Iโm on a never-ending quest for the next great culinary adventure. Letโs connect and chat about tech, travel, or the best pizza spots in town! ๐๐โ๏ธ