Top 10 Lodash Functions in my Everyday Coding
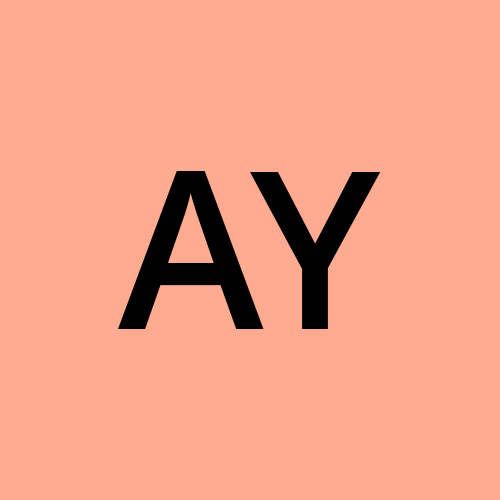
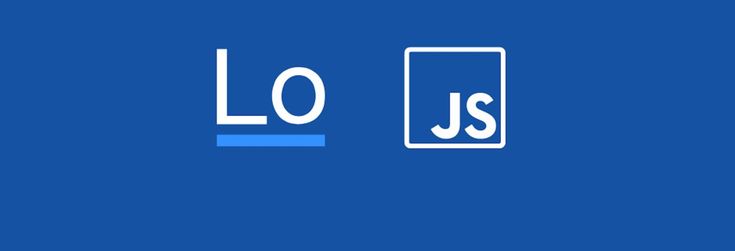
Efficiency is crucial nowadays. We often face repetitive tasks and boilerplate code. Lodash, a JavaScript utility library, simplifies these tasks with a rich set of functions for arrays, objects, strings, and more.
Some top lodash functions that I use to streamline my daily coding routine, making my code cleaner and more efficient.
You can install lodash in your javascript project by using the below commands
npm install lodash
Let's dive into the lodash functions, and let's take an example data
const allUsers = [
{ id: 101, name: 'Shreya', profession: 'Teacher' },
{ id: 102, name: 'Neetu', profession: 'Athelete' },
{ id: 103, name: 'Vidhi', profession: 'Teacher' },
{ id: 104, name: 'Sheetal', profession: 'Doctor' },
{ id: 105, name: '', profession: 'Athelete' },
];ร
const selectedUser = { id: 103, name: 'Vidhi' };
map
returns a new array containing all users Id's
map(allUsers, 'id'); //[101, 102, 103, 104]
find
find an element of the array which meets a specific condition
find(allUsers, { id: selectedUser.id }); // { id: 103, name: 'Vidhi', profession: 'Teacher' },
flattenDeep
recursively flattens a nested array.
const userIds = [101, 102, 103, 104,[105, [106, [107]]], [108, [109]]]; flattenDeep(userIds); //[101, 102, 103, 104, 105, 106, 107, 108, 109]
groupBy
groups array elements by the result of the iteratee function.
groupBy(allUsers, (user) => user.profession); { "Teacher" : [ { id: 101, name: 'Shreya', profession: 'Teacher' }, { id: 103, name: 'Vidhi', profession: 'Teacher' } ], "Athelete":[{ id: 102, name: 'Neetu', profession: 'Athelete' }, { id: 105, name: '', profession: 'Athelete' }, ], "Doctor":[{ id: 104, name: 'Sheetal', profession: 'Doctor' }] }
omit
creates an object excluding the specified keys.
omit(selectedUser, 'name') //{id: 103}
escape
converts characters "&", "<", ">", '"', and "'" in the string to their corresponding HTML entities.
escape('You are doing that & why'); //You are doing that & why
get
retrieves the value at the given path of an object.
get(selectedUser, 'name') //Vidhi
isNil
check if the value is null or undefined.
isNil(null) //true isNil(undefined) //true isNil('') // false
some
check if any element in the array meets the condition.
some(allUser, 'name'); //true some(allUser, 'id'); //true
every
check if every component of the array meets the condition.
every(allUser, 'name'); //false every(allUser, 'id'); //true
Do let me know in the comments, which lodash functions make your day-to-day coding easier.
Happy Coding ๐
Subscribe to my newsletter
Read articles from ankita yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
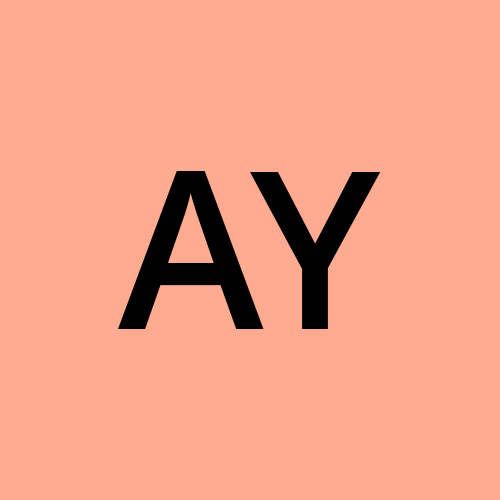