Closure in JS
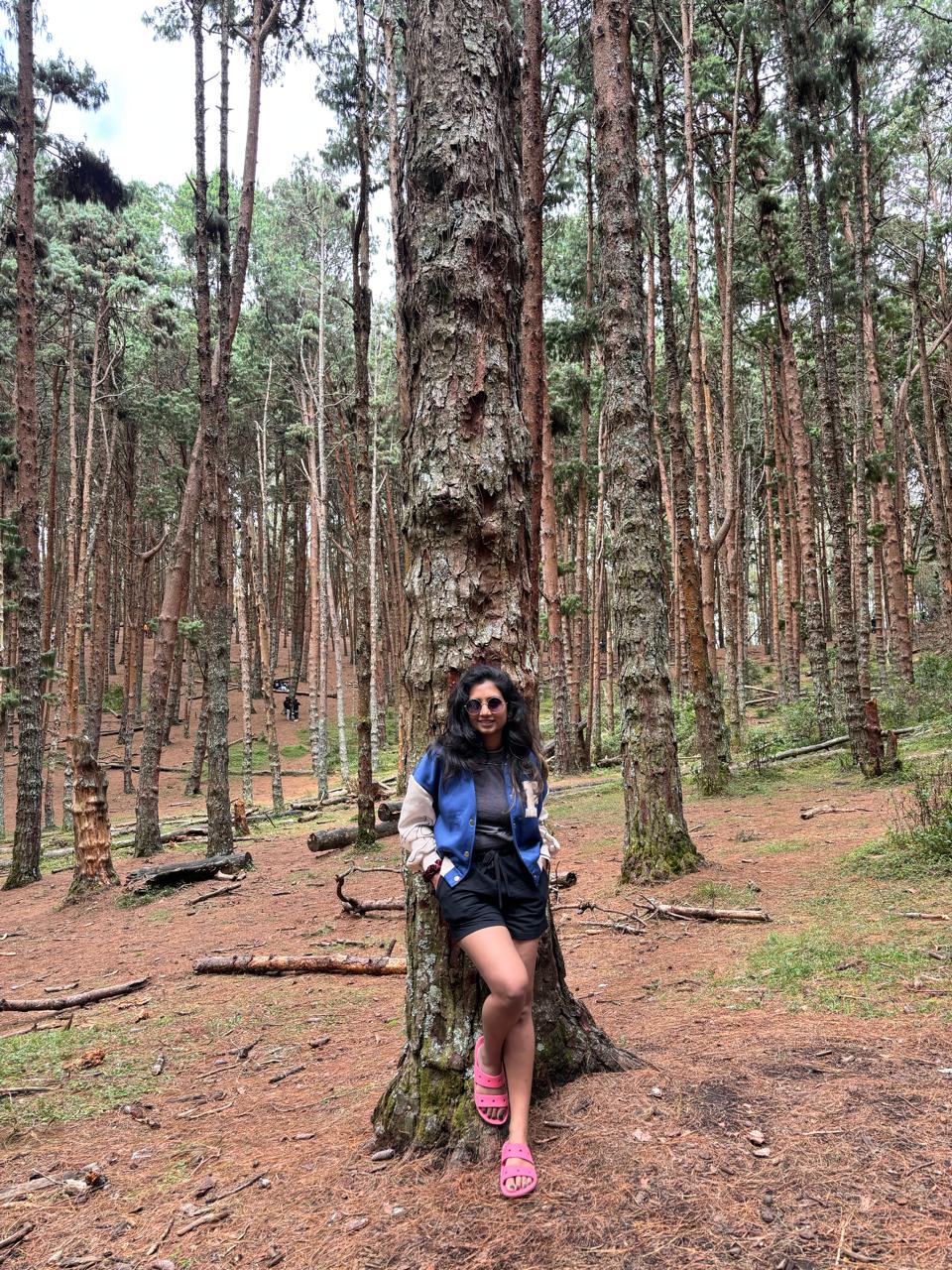
A function along with it's lexical scope inside a function is called closure.
example:
let's take a we want to increment a value by clicking a button, the basic way we can do it by is using a varible which will be incremented on every click.
var clickmeCount=0;
<button onclick="alert(++clickmeCount)">click me </button>
but the problem with this is, this is a global variable, without any wrapper, it can be tampered by any scripts, so
we can temprorialy fix this by using a variable inside of a function:
function x() {
var clickmeCount = 0;
return clickmeCount++;
}
<button onclick="alert(x())">click me </button>
But another problem we encounter here is on each click the clickmeCount is initialised to 0, which results in never changing the value of the variable,
so the concept of closures comes into picture,
Clousre is an inner function, which has access to the variables in it's lexical scope and it's parents's. aka it's own variables and variables of it's outer function
In other words, closure is a function inside of a function, these functions could be anonymous or named functions.
var inc = (function () {
var clickcount = 0;
return function () {
return clickcount++;
}
})();
here our outer function is executed just once since it is an IIFE, and rest of the times when we call the function inc, it will lead to incrementing the value of inner anonymous function.
the global variable cannot be tampered since it was an IIFE.
other usage of closures are:
Module Design Patterns
Currying
Functions that run once (IIFE)
Maintaining state in async world.
Iterators
setTimeOuts
etc.
Subscribe to my newsletter
Read articles from Preeti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
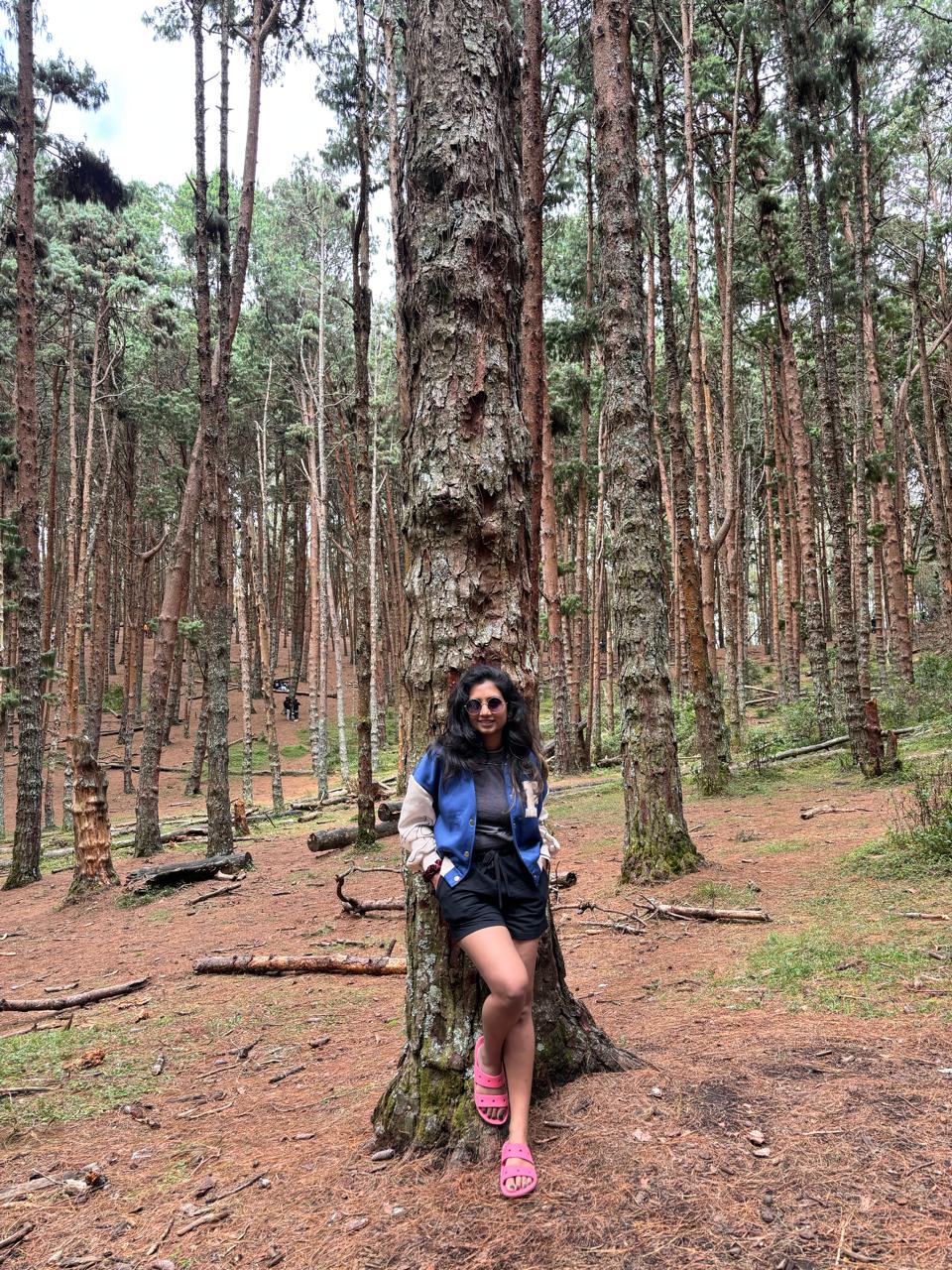