Java's Two Input Methods: Scanner and BufferedReader

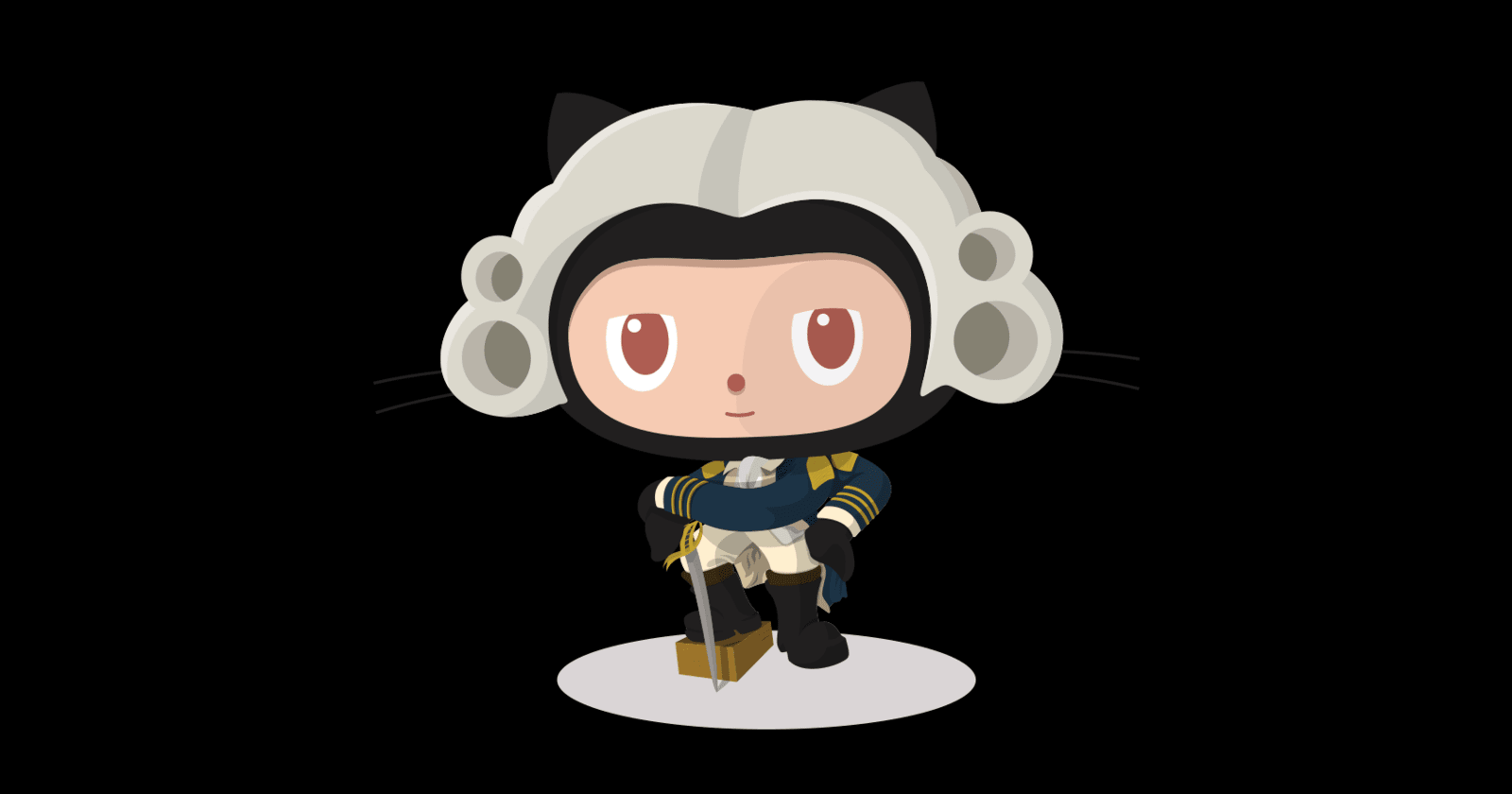
Introduction: Scanner
Scanner class is widely used in java because of it's easy code implementation . Input allows programs to obtain data from various sources, such as users, files, or external devices.
This data can be used for processing, analysis, or storage
Scanner makes console input easy for common tasks, with simple methods for different data types. It handles user input, allowing you to effortlessly read data from the keyboard or other sources.
java.util package includes Scanner class
Provides methods for parsing different data types (e.g.,
nextInt()
,nextLine()
).Simple, Slowerfor large inputs, Higher memory consumption.
import java.util.Scanner; public class ScannerExample { public static void main(String[] args) { Scanner scanner = new Scanner(SJavaystem.in); System.out.println("Enter your name:"); String name = scanner.nextLine(); System.out.println("Enter your age:"); int age = scanner.nextInt(); System.out.println("Name: " + name + ", Age: " + age); scanner.close(); } }
Introduction: BufferedReader
BufferedReader gives more control and efficiency, making it better for complex input and large data, like reading from files or network streams.
It reads input as a stream of characters and is useful for handling text-based data, parsing lines, or processing data incrementally. BufferedReader offers better control over input, allowing for graceful error handling. It's often used when performance and robust error handling are important.
- To use BufferedReader, first, we create an object of the InputStreamReader class. This object specifies where the input originates from
InputStreamReader is = new InputStreamReader(System.in)
Initializing BufferedReader: Create a BufferedReader object and pass the InputStreamReader object into it. This BufferedReader will be responsible for reading and processing the input.
InputStreamReader is = new InputStreamReader(System.in); BufferedReader bf = new BufferedReader(in);
Here, the InputStreamReader class is used to convert theraw byte-based inputstream (System.in) into a character-based input stream. This is necessary to read text input conveniently.
Can be used as:
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
The BufferedReader is used to buffer the input stream, making it more efficient to read lines of text. It provides methods like readLine() to read complete lines of text, which is very useful for user input.
Reading Numerical Input
To read numerical input from the user, we use the readLine() method. However, this method returns a string, so you'll need to convert it to an integer using Integer.parseInt().
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class BufferedReaderExample {
public static void main(String[] args) {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
try {
System.out.println("Enter your name:");
String name = reader.readLine();
System.out.println("Enter your age:");
int age = Integer.parseInt(reader.readLine());
System.out.println("Name: " + name + ", Age: " + age);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Conclusion:
Scanner and BufferedReader are very effective classes in java for taking inputs and are used as per the requirement.
Subscribe to my newsletter
Read articles from Harsh Pareek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
