Goodbye Zone.js: What's new in Angular 18?
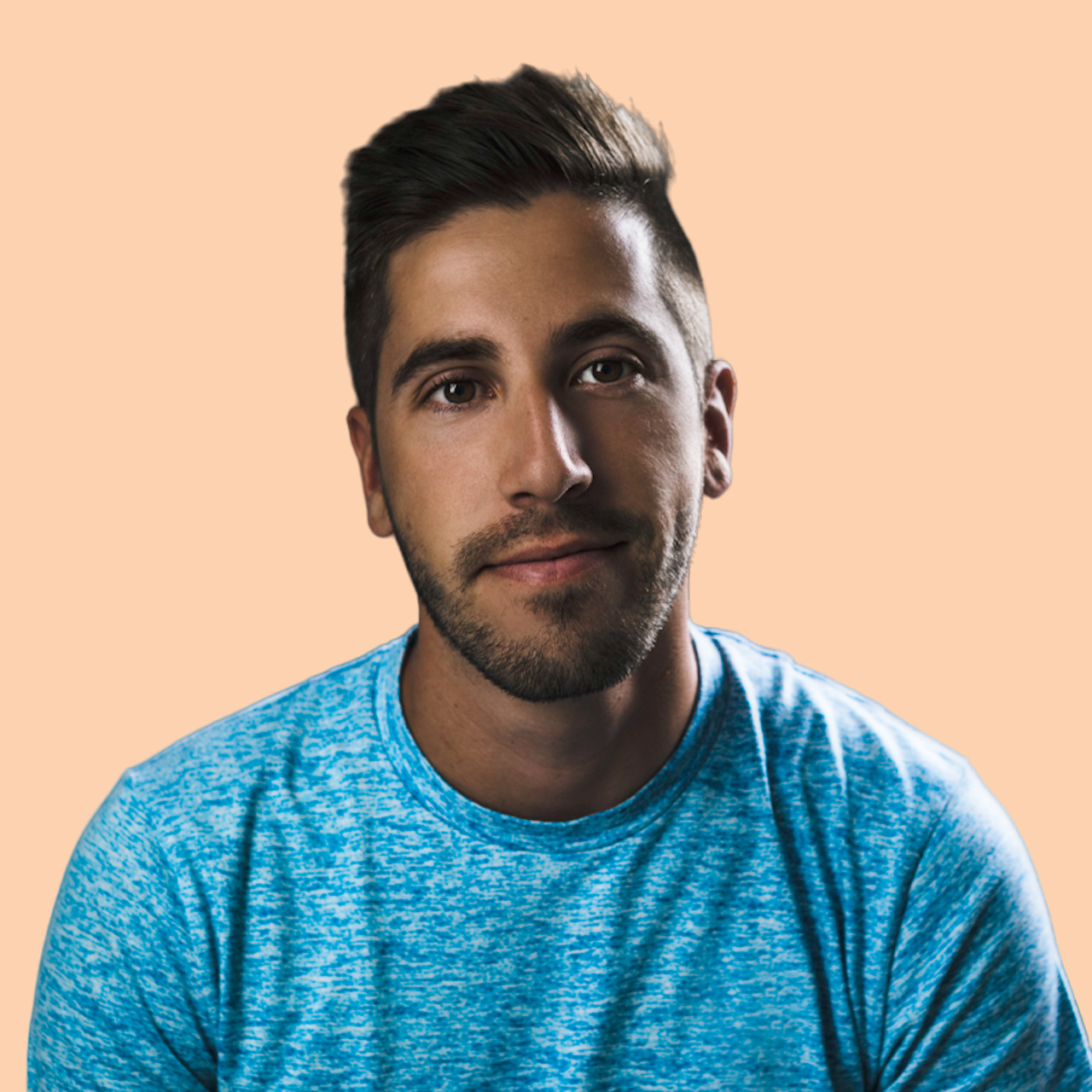
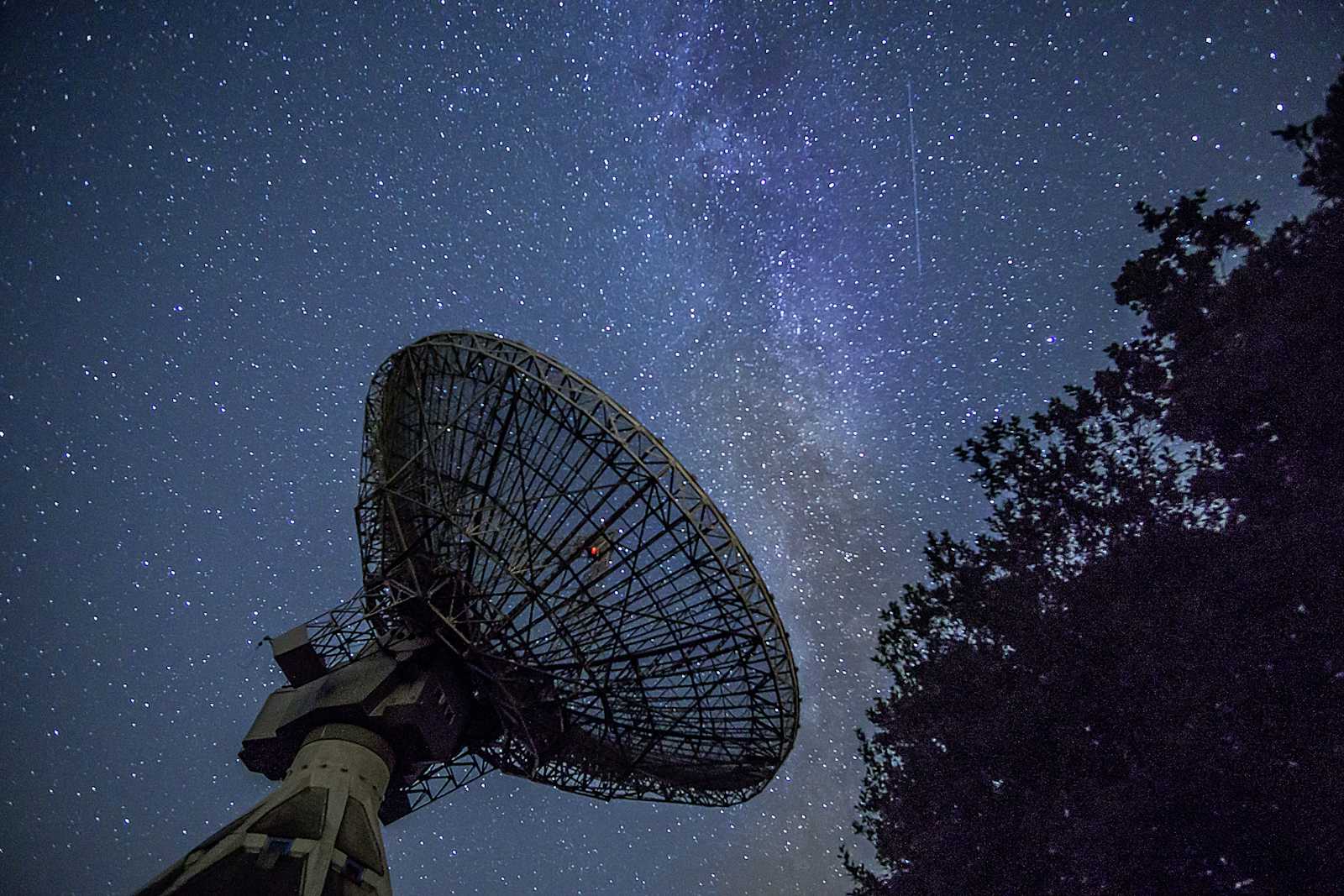
Angular 18 is revolutionizing the way change detection is handled, removing the dependency on Zone.js. This change began in Angular 17 with the introduction of Signals. Now, instead of depending only on Signal components, the new hybrid detection system will automatically trigger change detection for any invoker of markForCheck
, whether they are inside or outside of Zone.js.
What is Zone.js?
Zone.js acts as a helper to check whether each component in a large application needs to be updated. Each time you use a component, Zone.js ensures that any change is correctly detected and updated.
Signals: The introduction in Angular 17
Angular 17 introduced a new concept called signals. Signals allow for more efficient interactions with components, reducing the dependency on Zone.js. Signals allow developers to handle state changes more explicitly.
The revolution of Angular 18: Hybrid system
Angular 18 takes this a step further with a hybrid change detection system. Now, any invoker of markForCheck
will automatically trigger change detection, regardless of whether it is inside or outside of Zone.js.
Here is an example to illustrate this:
import { Component, ChangeDetectorRef, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `<div>{{ mySignal }}</div>`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
mySignal = 'Initial Value';
constructor(private cdr: ChangeDetectorRef) {}
ngOnInit() {
setTimeout(() => {
this.mySignal = 'Updated Value';
this.cdr.markForCheck();
}, 3000);
}
}
In this example, we manually inject ChangeDetectorRef
and invoke markForCheck
after updating the component's state.
Using Signals for simplicity
With the new Signals feature in Angular 18, you can simplify this process:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `{{ mySignal() }}`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class MyComponent {
mySignal = signal(0);
ngOnInit() {
setTimeout(() => this.mySignal.set(1), 3000);
}
}
In this improved version, we use a signal, and the view updates automatically without needing to manually call markForCheck
.
Conclusion
The main benefit of this new system is the reduction in dependency on Zone.js, which can improve performance and code clarity. However, it is important for developers to understand how Signals and the hybrid system work in order to make the most of these improvements. Angular 18 is a revolution in change detection, making applications more efficient and easier to maintain. Removing the dependency on Zone.js, along with the introduction of Signals and the hybrid system, is a significant step forward for Angular developers.
Subscribe to my newsletter
Read articles from Rubén Peregrina directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
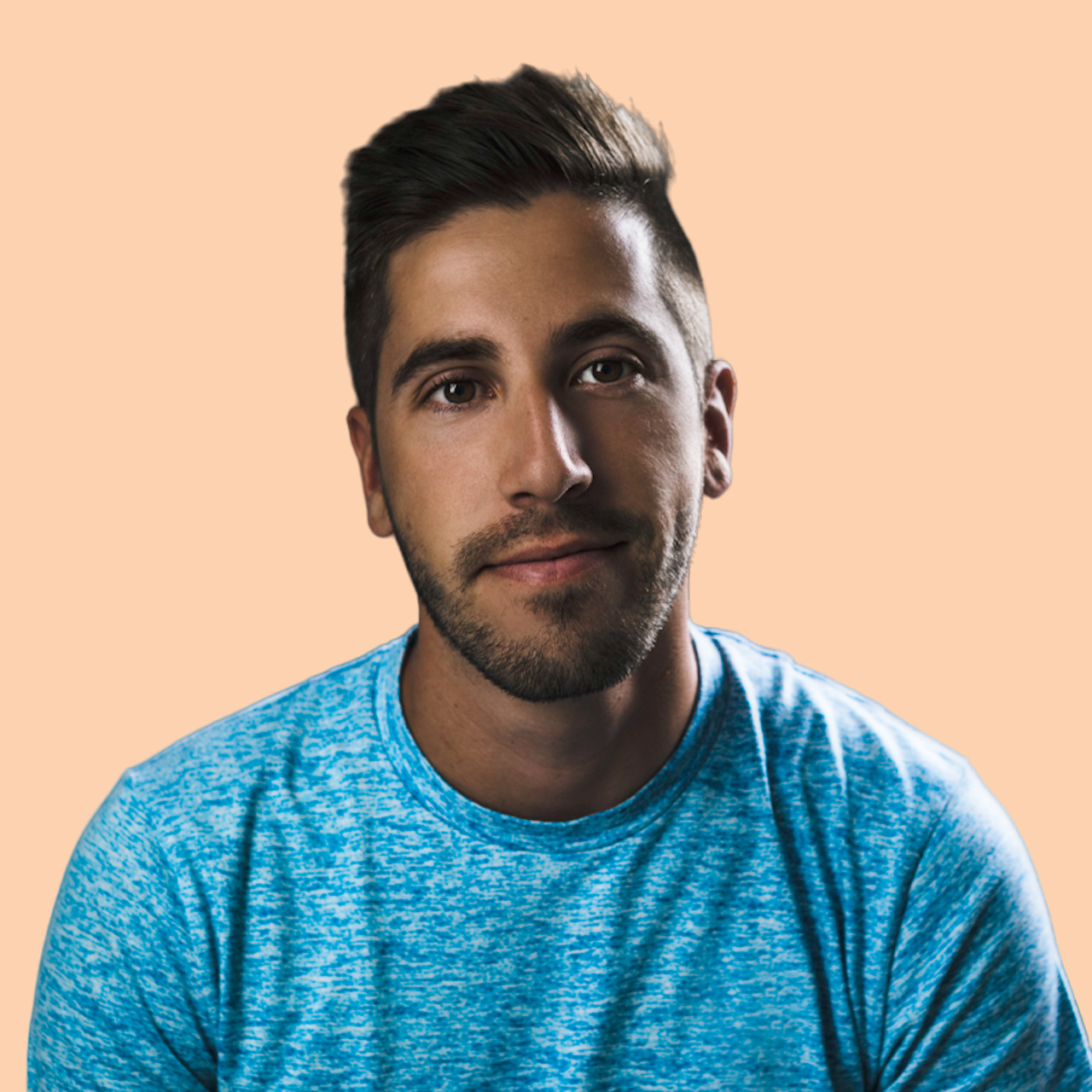
Rubén Peregrina
Rubén Peregrina
Front-end software engineer and I specialise in Angular🤖. I've been working with it since Angular JS and I like to keep up with new technologies. I love to write about Angular, TypeScript, JavaScript and more 📰. My hobbies are travelling around Southeast Asia ✈️, cars and technology.