🚀Day 17/180 1752. Check if Array Is Sorted and Rotated(Leetcode)
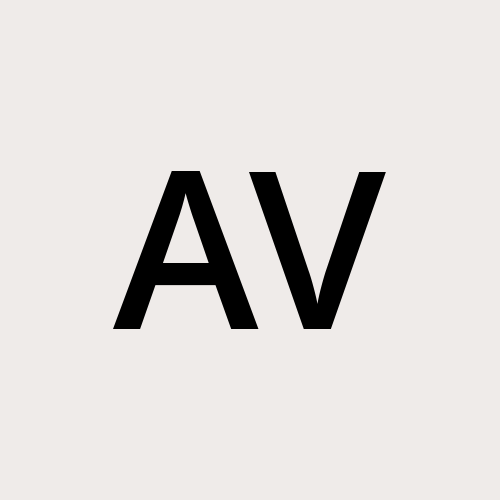
1752. Check if Array Is Sorted and Rotated
class Solution {
public boolean check(int[] nums) {
int spike=0;
for(int i=0;i<nums.length-1;i++){
if(nums[i]>nums[i+1]) spike++;
}
if(nums[nums.length-1]>nums[0]) spike++;
if(spike>1) return false;
return true;
}
}
DRY :
Dry run of the given solution with an example. We'll use the array [3, 4, 5, 1, 2]
to demonstrate the dry run.
Array: [3, 4, 5, 1, 2]
Initial Setup
spike = 0
Loop Through the Array
Iteration 1 (
i = 0
):nums[0] = 3
nums[1] = 4
3 <= 4
(No spike)spike = 0
Iteration 2 (
i = 1
):nums[1] = 4
nums[2] = 5
4 <= 5
(No spike)spike = 0
Iteration 3 (
i = 2
):nums[2] = 5
nums[3] = 1
5 > 1
(Spike detected)spike = 1
Iteration 4 (
i = 3
):nums[3] = 1
nums[4] = 2
1 <= 2
(No spike)spike = 1
Final Comparison
Compare the last element with the first element:
nums[4] = 2
nums[0] = 3
2 < 3
(No spike here)spike = 1
Final Spike Check
spike = 1
, which is not greater than 1.
Result
- Since
spike <= 1
, the function returnstrue
.
Conclusion
The array [3, 4, 5, 1, 2]
is determined to be sorted and rotated.
Here's the step-by-step dry run in code format:
class Solution {
public boolean check(int[] nums) {
int spike = 0;
for (int i = 0; i < nums.length - 1; i++) {
if (nums[i] > nums[i + 1]) {
spike++;
}
}
if (nums[nums.length - 1] > nums[0]) {
spike++;
}
return spike <= 1;
}
}
// Dry run example
Solution solution = new Solution();
int[] nums = {3, 4, 5, 1, 2};
boolean result = solution.check(nums);
// result should be true
This dry run confirms that the solution correctly identifies the array [3, 4, 5, 1, 2]
as sorted and rotated.
Subscribe to my newsletter
Read articles from Aniket Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
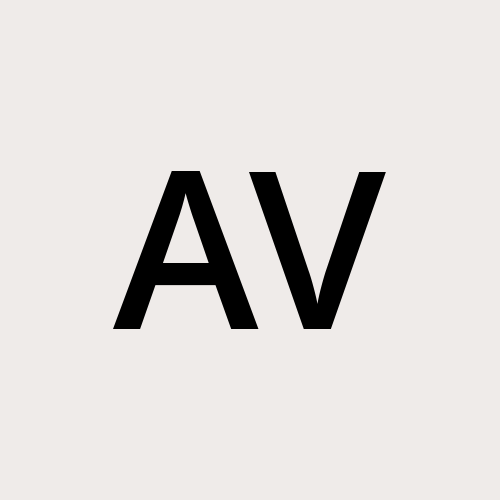