Exploring Depth-First Search (DFS) and Breadth-First Search (BFS) Algorithms with JAVA
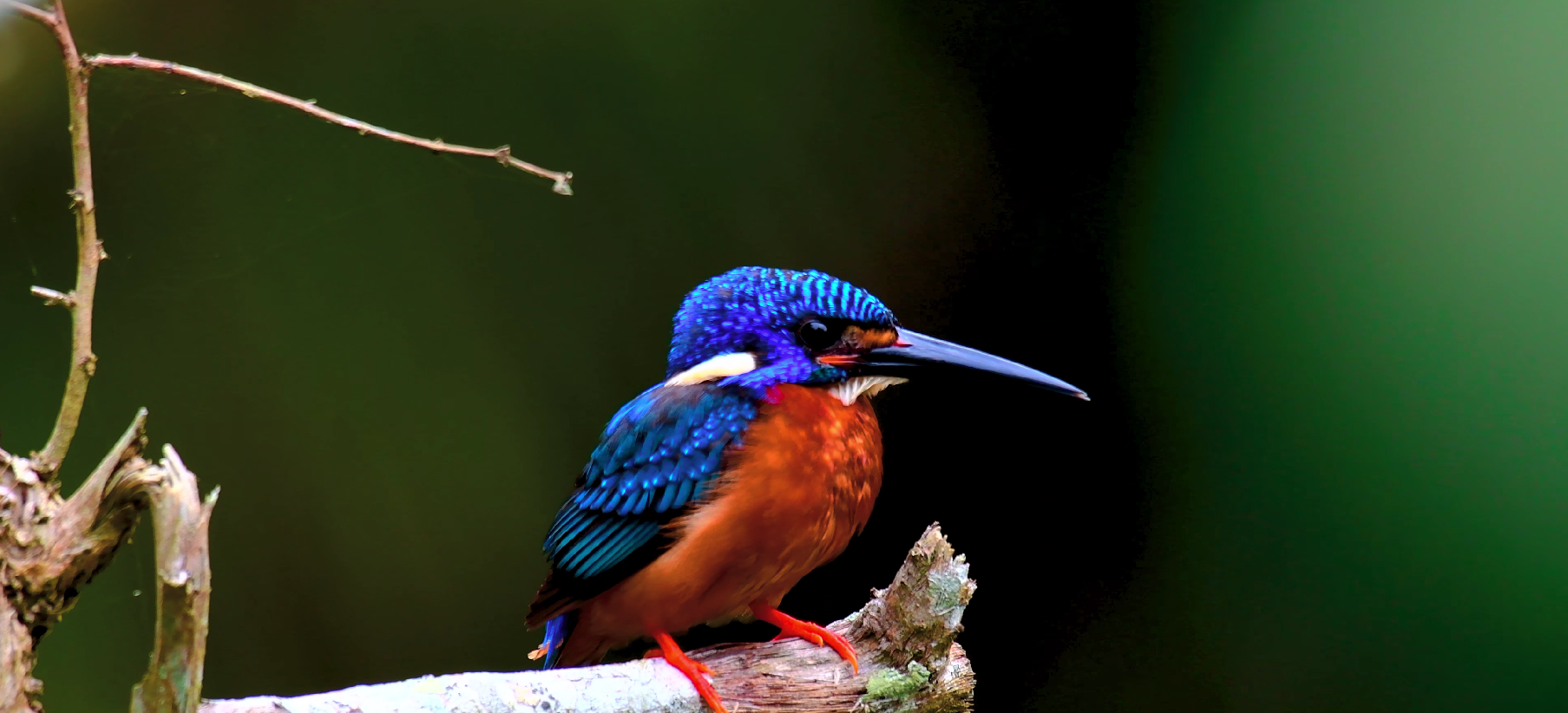
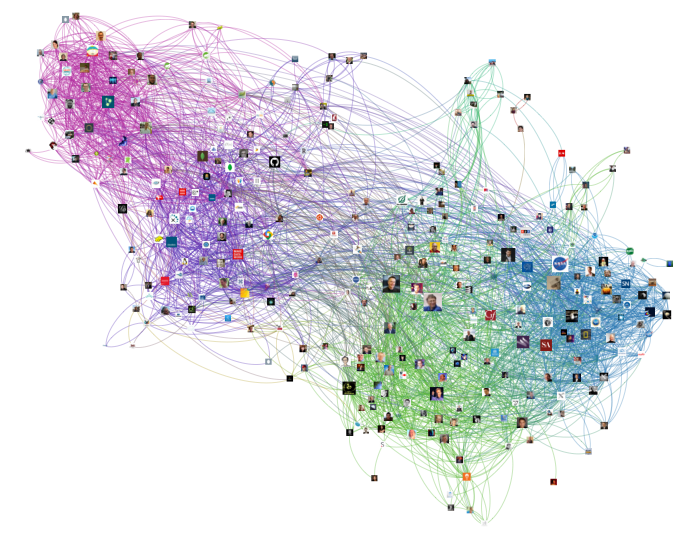
When it comes to traversing or searching through data structures like trees and graphs, two of the most fundamental algorithms are Depth-First Search (DFS) and Breadth-First Search (BFS). These algorithms are foundational in computer science and have a wide range of applications, from pathfinding in games to web crawling and more. In this blog post, we will delve into the details of DFS and BFS, explore their implementations, and discuss their use cases.
Depth-First Search (DFS)
The algorithm starts at the root node and explores as far as possible along each branch before backtracking.
Key Characteristics of DFS:
LIFO Structure: DFS uses a stack data structure (Last In, First Out) either explicitly or through recursion.
Path Discovery: DFS is useful for pathfinding and connectivity in graphs.
Memory Usage: DFS is memory efficient as it needs to store only the nodes on the current path.
DFS Implementation:
DFS can be implemented using either recursion or an explicit stack.
Recursive Implementation:
import java.util.*;
public class DFSRecursive {
private Map<String, List<String>> graph;
private Set<String> visited;
public DFSRecursive(Map<String, List<String>> graph) {
this.graph = graph;
this.visited = new HashSet<>();
}
public void dfs(String node) {
if (!visited.contains(node)) {
System.out.print(node + " ");
visited.add(node);
for (String neighbor : graph.get(node)) {
dfs(neighbor);
}
}
}
public static void main(String[] args) {
Map<String, List<String>> graph = new HashMap<>();
graph.put("A", Arrays.asList("B", "C"));
graph.put("B", Arrays.asList("D", "E"));
graph.put("C", Arrays.asList("F"));
graph.put("D", Collections.emptyList());
graph.put("E", Arrays.asList("F"));
graph.put("F", Collections.emptyList());
DFSRecursive dfsRecursive = new DFSRecursive(graph);
dfsRecursive.dfs("A");
}
}
Iterative Implementation:
import java.util.*;
public class DFSIterative {
private Map<String, List<String>> graph;
public DFSIterative(Map<String, List<String>> graph) {
this.graph = graph;
}
public void dfs(String start) {
Set<String> visited = new HashSet<>();
Stack<String> stack = new Stack<>();
stack.push(start);
while (!stack.isEmpty()) {
String node = stack.pop();
if (!visited.contains(node)) {
System.out.print(node + " ");
visited.add(node);
List<String> neighbors = graph.get(node);
Collections.reverse(neighbors);
for (String neighbor : neighbors) {
stack.push(neighbor);
}
}
}
}
public static void main(String[] args) {
Map<String, List<String>> graph = new HashMap<>();
graph.put("A", Arrays.asList("B", "C"));
graph.put("B", Arrays.asList("D", "E"));
graph.put("C", Arrays.asList("F"));
graph.put("D", Collections.emptyList());
graph.put("E", Arrays.asList("F"));
graph.put("F", Collections.emptyList());
DFSIterative dfsIterative = new DFSIterative(graph);
dfsIterative.dfs("A");
}
}
Applications of DFS:
Finding connected components in a graph.
Topological sorting.
Solving puzzles with only one solution, such as mazes.
Pathfinding in games.
Breadth-First Search (BFS)
Breadth-First Search (BFS) is an algorithm for traversing or searching tree or graph data structures. It starts at the root node and explores all neighbor nodes at the present depth level before moving on to nodes at the next depth level.
Key Characteristics of BFS:
FIFO Structure: BFS uses a queue data structure (First In, First Out).
Level Order Traversal: BFS processes nodes level by level.
Shortest Path: BFS is ideal for finding the shortest path in unweighted graphs.
BFS Implementation:
BFS is typically implemented using a queue.
import java.util.*;
public class BFS {
private Map<String, List<String>> graph;
public BFS(Map<String, List<String>> graph) {
this.graph = graph;
}
public void bfs(String start) {
Set<String> visited = new HashSet<>();
Queue<String> queue = new LinkedList<>();
queue.add(start);
while (!queue.isEmpty()) {
String node = queue.poll();
if (!visited.contains(node)) {
System.out.print(node + " ");
visited.add(node);
queue.addAll(graph.get(node));
}
}
}
public static void main(String[] args) {
Map<String, List<String>> graph = new HashMap<>();
graph.put("A", Arrays.asList("B", "C"));
graph.put("B", Arrays.asList("D", "E"));
graph.put("C", Arrays.asList("F"));
graph.put("D", Collections.emptyList());
graph.put("E", Arrays.asList("F"));
graph.put("F", Collections.emptyList());
BFS bfs = new BFS(graph);
bfs.bfs("A");
}
}
Applications of BFS:
Finding the shortest path in an unweighted graph.
Web crawling.
Peer-to-peer networks.
Broadcasting in network routers.
Comparing DFS and BFS
Performance:
Time Complexity: Both DFS and BFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges.
Space Complexity: DFS has a space complexity of O(V) due to the stack (or recursion call stack), while BFS also has a space complexity of O(V) due to the queue.
Use Cases:
DFS: More suitable for problems involving exhaustive search, like solving puzzles or checking connectivity.
BFS: More suitable for finding the shortest path or levels in an unweighted graph.
Conclusion
Understanding DFS and BFS is crucial for anyone looking to master data structures and algorithms. These algorithms not only form the basis of many complex algorithms but also have numerous practical applications. Whether you're preparing for a technical interview or working on a project that involves complex data structures, having a solid grasp of DFS and BFS will undoubtedly be beneficial.
Feel free to leave any questions or comments below. Happy coding!
Subscribe to my newsletter
Read articles from SANTOSH SINGH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
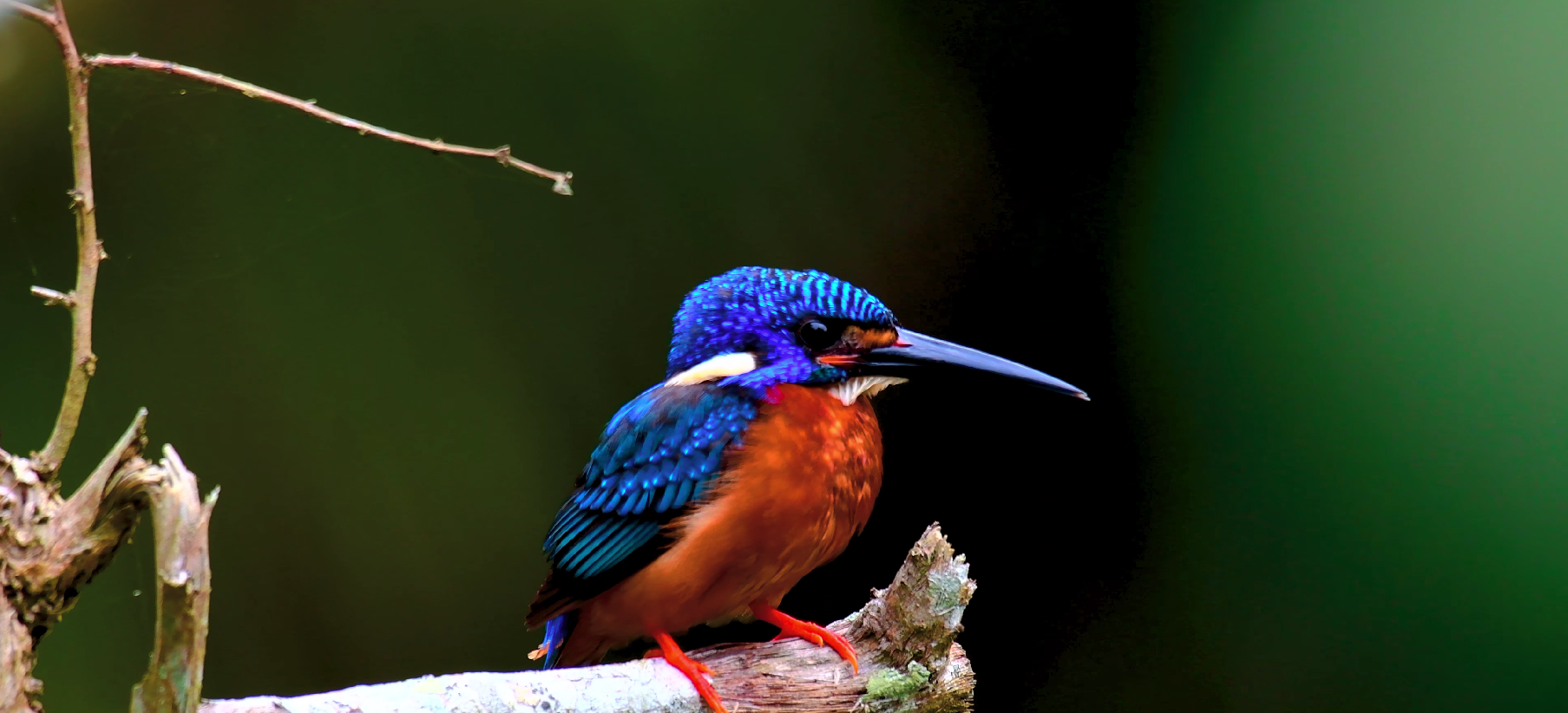