Implementing Access Control in Yii2

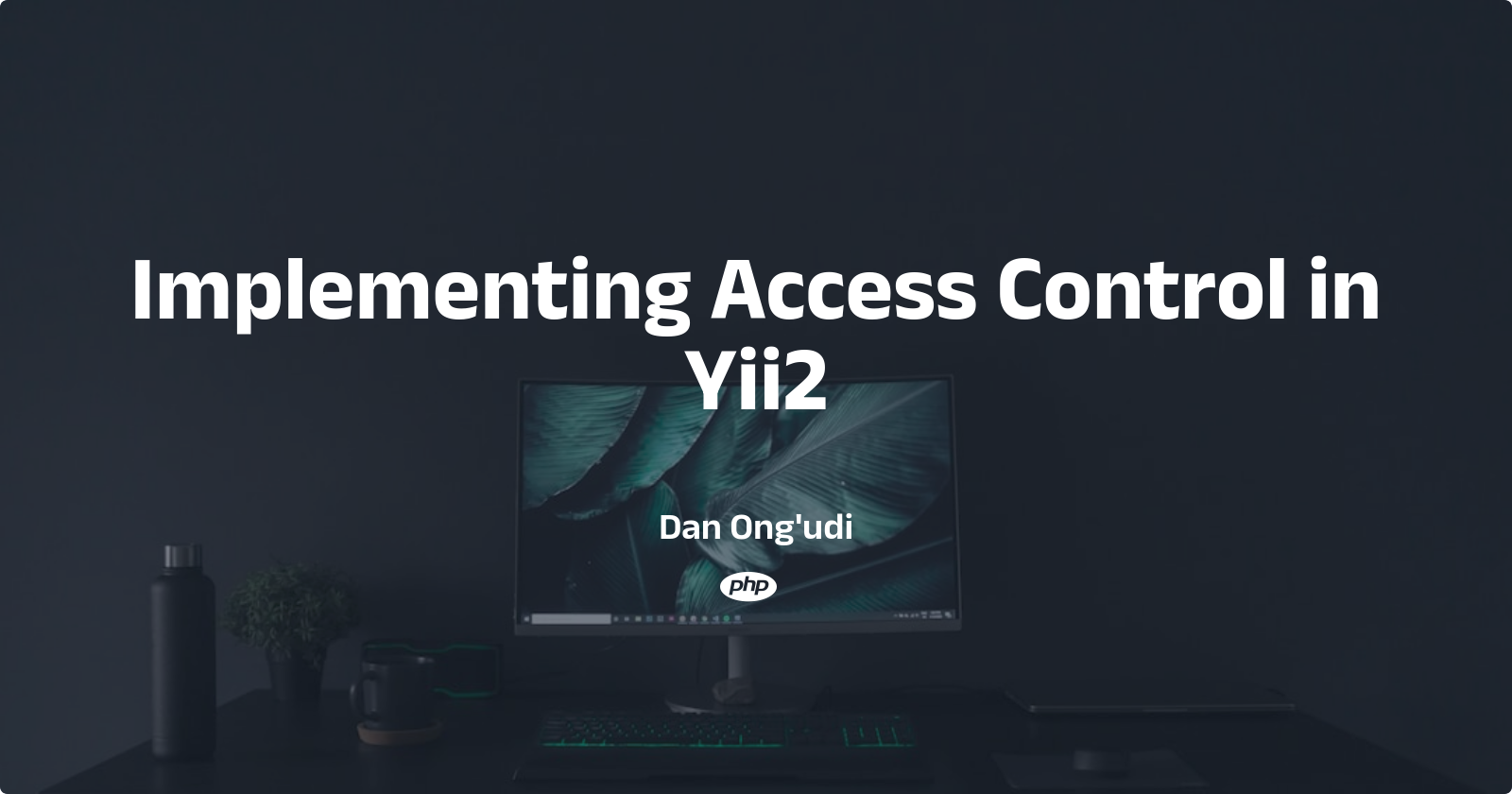
To restrict access to certain actions, such as create, update, and delete, you need to modify the controller's behaviors
function. Here's a step-by-step guide using the CompaniesController.php
as an example:
Step 1: Modify the behaviors
Function
In your CompaniesController.php
file, update the behaviors
function to include access control and verb filtering:
public function behaviors()
{
return array_merge(
parent::behaviors(),
[
'access' => [
'class' => AccessControl::classname(),
'only' => ['create', 'update', 'delete'], // Specify the actions that need access control
'rules' => [
[
'allow' => true, // Allow access to these actions
'roles' => ['@'], // Restrict access to authenticated users only
],
],
],
'verbs' => [
'class' => VerbFilter::className(),
'actions' => [
'delete' => ['POST'], // Restrict the delete action to only allow POST requests
],
],
]
);
}
Explanation:
Access Control (
AccessControl
):Class: Specifies that
AccessControl
is used for managing access rules.Only: Lists the actions (
create
,update
,delete
) that require access control.Rules: Defines the rules for access:
Allow: Set to
true
, meaning access is allowed for these actions.Roles: Restricts access to users who are authenticated (denoted by
@
).
Verb Filter (
VerbFilter
):Class: Specifies that
VerbFilter
is used for managing HTTP verb filtering.Actions: Specifies that the
delete
action should only be accessible via POST requests, adding an extra layer of security.
Result:
With this configuration, only authenticated users can access the create
, update
, and delete
actions in the CompaniesController
. Additionally, the delete
action is restricted to POST requests, preventing accidental or unauthorized deletions via other HTTP methods.
By following these steps, you can implement access control in your Yii2 application to secure specific actions and ensure they are only accessible to authorized users.
Subscribe to my newsletter
Read articles from Dan Ongudi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
