Creating a Drop-Down List in Yii2

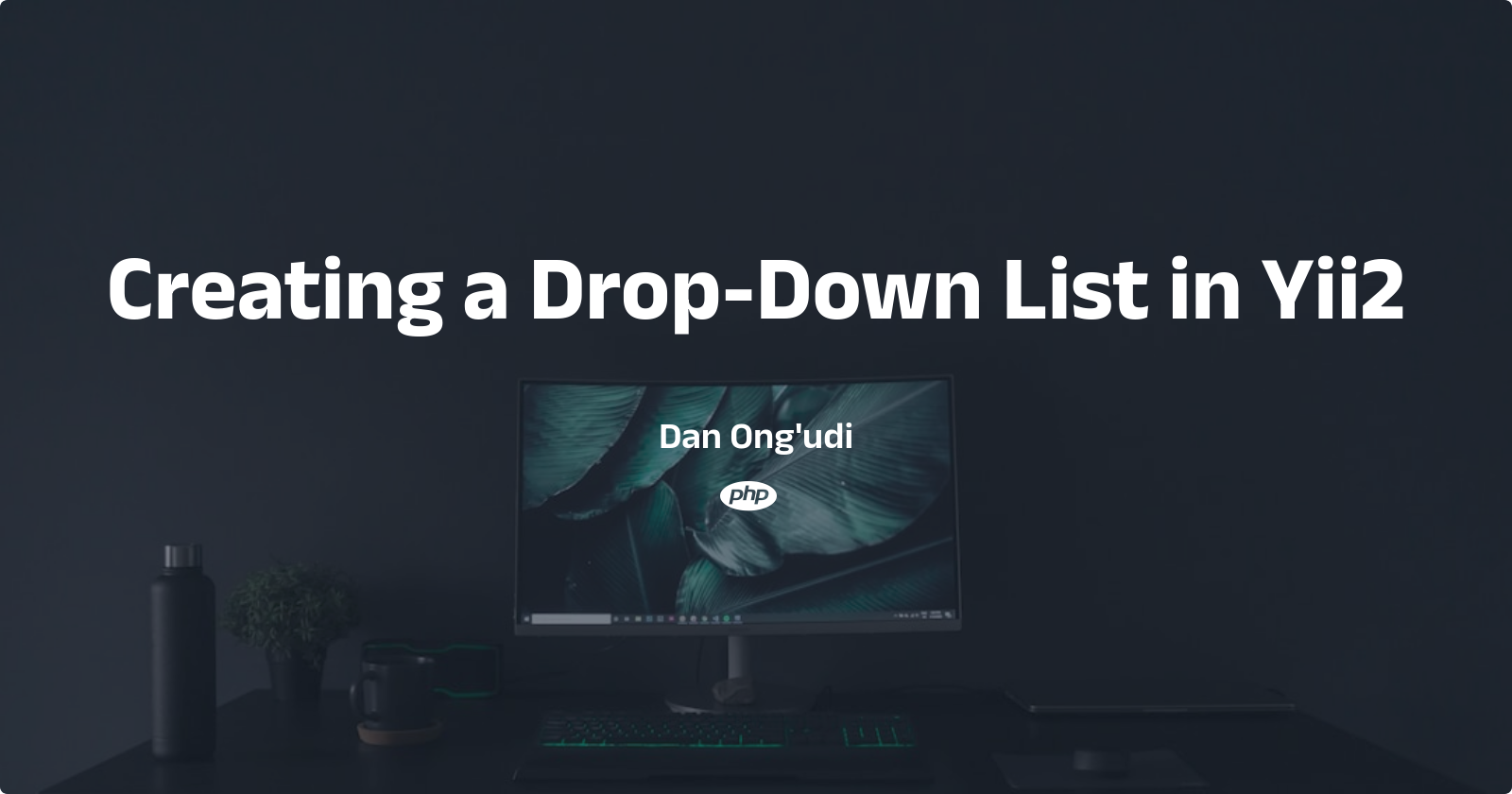
To create a drop-down list populated with data from the database, you can use the ArrayHelper
class. Here’s a detailed guide on how to create a drop-down list of companies in a form:
Step 1: Prepare the Form
In your form view file, add the following code to create a drop-down list:
<?= $form->field($model, 'companies_company_id')->dropDownList(
ArrayHelper::map(Companies::find()->all(), 'company_id', 'company_name'),
['prompt' => 'Select company']
) ?>
Explanation:
Retrieving Data:
Companies::find()->all()
retrieves all records from thecompanies
table in the database.
Mapping Data:
ArrayHelper::map(Companies::find()->all(), 'company_id', 'company_name')
converts the list of companies into an array suitable for a drop-down list.The first parameter is the data (all company records).
The second parameter is the key field (
company_id
), which will be used as the value in the drop-down options.The third parameter is the value field (
company_name
), which will be displayed in the drop-down options.
Prompt:
- The
['prompt' => 'Select company']
option adds a prompt at the top of the drop-down list to guide users to select a company.
- The
Step 2: Import Necessary Classes
To make the ArrayHelper
and Companies
functions work, you need to import them at the top of your form view file:
use yii\helpers\ArrayHelper;
use backend\models\Companies;
Step 3: Customize the Attribute Label
If you want to change the label displayed on top of the drop-down list from 'Company companies id' to a more user-friendly label like 'Company name', you need to update the attributeLabels
function in the Companies
model.
In backend\models\Companies.php
, update the attributeLabels
function:
public function attributeLabels()
{
return [
'companies_company_id' => 'Company name',
// other labels
];
}
Full Code Example
Here’s how your form view file might look with the drop-down list:
<?php
use yii\helpers\ArrayHelper;
use backend\models\Companies;
/* @var $form yii\widgets\ActiveForm */
/* @var $model backend\models\YourModel */
?>
<?= $form->field($model, 'companies_company_id')->dropDownList(
ArrayHelper::map(Companies::find()->all(), 'company_id', 'company_name'),
['prompt' => 'Select company']
) ?>
By following these steps, you will create a drop-down list in your Yii2 application that is populated with data from the companies
table. This allows users to select a company by name, with company_id
being used as the underlying value.
Subscribe to my newsletter
Read articles from Dan Ongudi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
