Creating a Floating Form (Modal) in Yii2

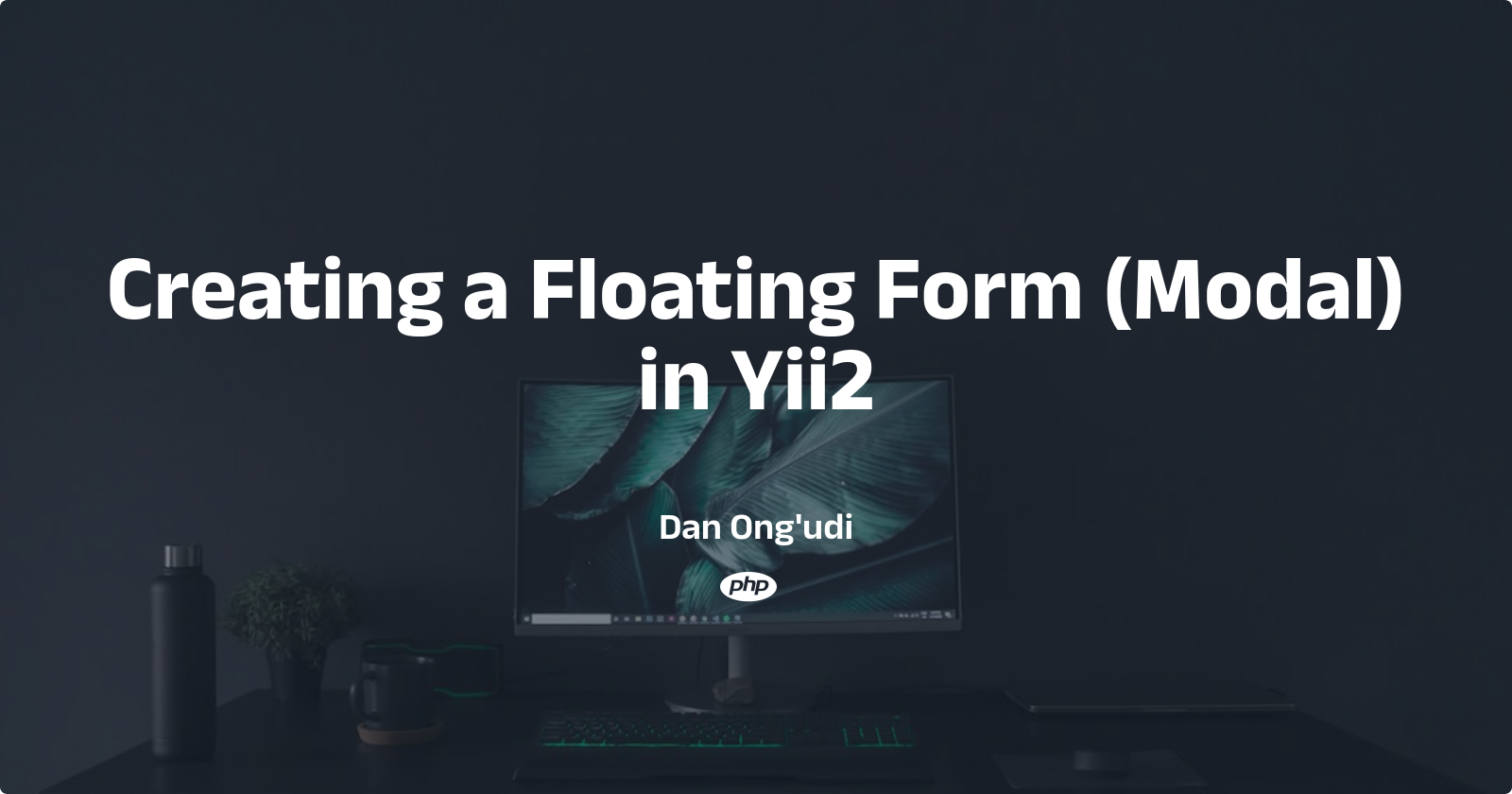
To create a floating form (modal) in Yii2, follow these detailed steps. This example will show how to create a modal for adding a new company on the company index page.
Step 1: Change the Anchor Tag to a Button
In the view file of your company index page, replace the anchor tag with a button that will trigger the modal. Add the following code:
use yii\helpers\Html;
use yii\helpers\Url;
// Add a button to trigger the modal
echo Html::button('Create Company', [
'value' => Url::to('index.php?r=company/create'),
'class' => 'btn btn-success',
'id' => 'modalButton'
]);
Step 2: Add Bootstrap Modal to the Page
Under the button, add the Bootstrap modal class to create the modal structure:
use yii\bootstrap4\Modal;
?>
<p>
<?= Html::button('Create Company', [
'value' => Url::to('index.php?r=company/create'),
'class' => 'btn btn-success',
'id' => 'modalButton'
]) ?>
</p>
<?php
Modal::begin([
'header' => '<h4>Companies</h4>',
'id' => 'modal',
'size' => 'modal-lg',
]);
echo "<div id='modalContent'></div>";
Modal::end();
?>
Step 3: Create a JavaScript File for the Modal
Create a JavaScript file (e.g., modal.js
) and include the following code. This script handles displaying the modal and loading its content:
$(function(){
$('#modalButton').click(function(){
$('#modal').modal('show')
.find('#modalContent')
.load($(this).attr('value'));
});
});
Include this JavaScript file in your view file, or register it in your view file's asset bundle:
$this->registerJsFile('@web/js/modal.js', ['depends' => [\yii\web\JqueryAsset::class]]);
Step 4: Update the CompanyController
In CompanyController.php
, modify the action responsible for rendering the create form to use AJAX rendering:
public function actionCreate()
{
$model = new Company();
if ($model->load(Yii::$app->request->post()) && $model->save()) {
return $this->redirect(['index']);
}
return $this->renderAjax('create', [
'model' => $model,
]);
}
Summary
With these steps, you have created a floating form (modal) in Yii2 for creating a new company.
Full Code Example
In the view file (index.php
):
<?php
use yii\helpers\Html;
use yii\helpers\Url;
use yii\bootstrap4\Modal;
?>
<p>
<?= Html::button('Create Company', [
'value' => Url::to('index.php?r=company/create'),
'class' => 'btn btn-success',
'id' => 'modalButton'
]) ?>
</p>
<?php
Modal::begin([
'header' => '<h4>Companies</h4>',
'id' => 'modal',
'size' => 'modal-lg',
]);
echo "<div id='modalContent'></div>";
Modal::end();
?>
<?php
$this->registerJsFile('@web/js/modal.js', ['depends' => [\yii\web\JqueryAsset::class]]);
?>
In the JavaScript file (modal.js
):
$(function(){
$('#modalButton').click(function(){
$('#modal').modal('show')
.find('#modalContent')
.load($(this).attr('value'));
});
});
In the controller (CompanyController.php
):
public function actionCreate()
{
$model = new Company();
if ($model->load(Yii::$app->request->post()) && $model->save()) {
return $this->redirect(['index']);
}
return $this->renderAjax('create', [
'model' => $model,
]);
}
By following these steps, you can create a modal form in Yii2, improving user experience by allowing data entry without leaving the current page.
Subscribe to my newsletter
Read articles from Dan Ongudi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
