Understanding DOM, Virtual DOM, and Shadow DOM
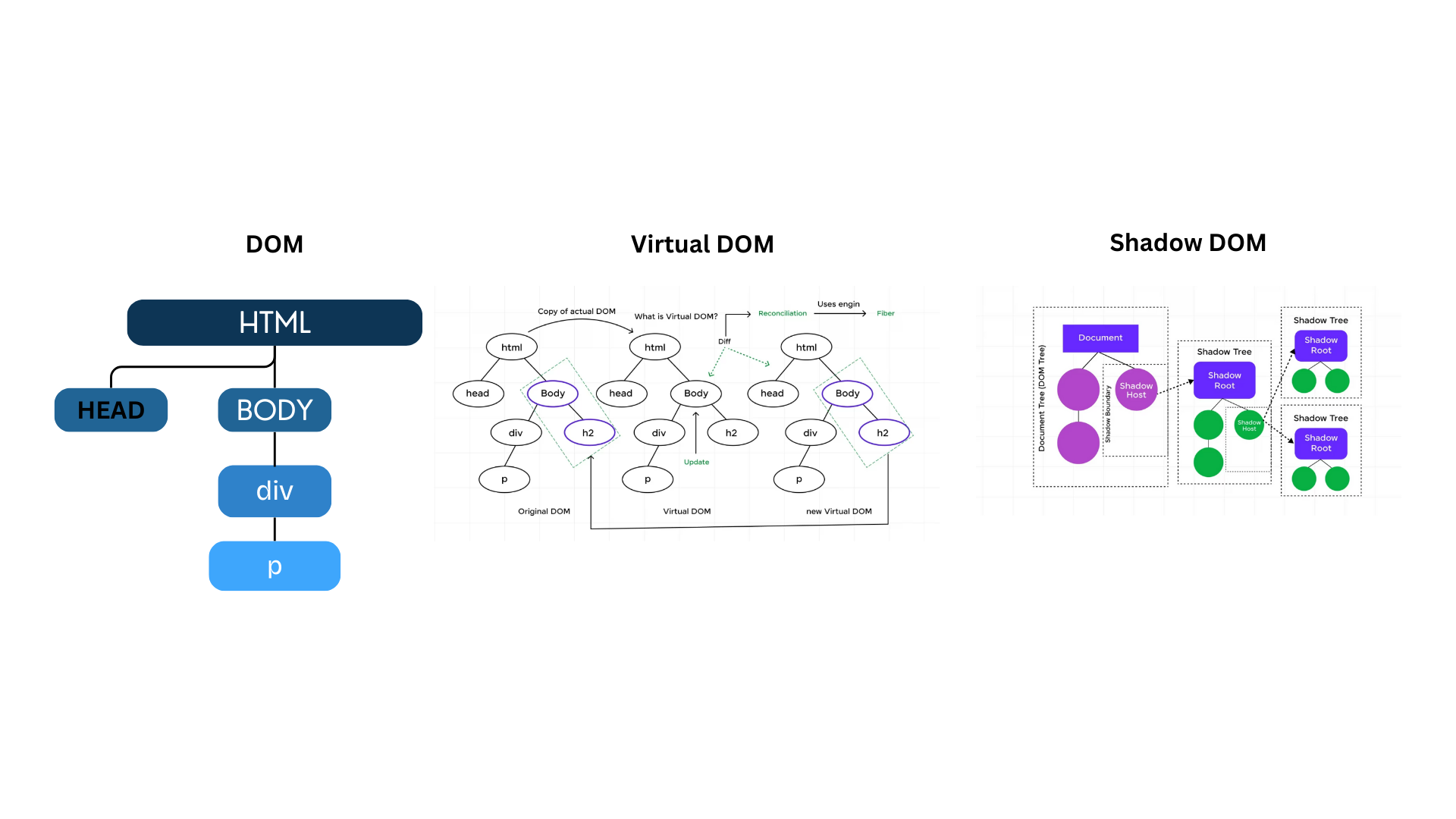
I've been working as a developer for around two years, and interestingly, I recently came across the term "Shadow DOM," which I wasn't aware of. So, I did some research, and it turned out to be quite fascinating.
The DOM itself is a very important topic, but understanding the other types of DOM is just as crucial because, as web development gets complex, handling the DOM becomes very important. This has led to the innovation of the Shadow DOM and Virtual DOM. Let's explore the traditional DOM, Virtual DOM, and Shadow DOM, understanding their uses, benefits, and typical scenarios for their application
Traditional DOM
What is DOM?
DOM stands for ‘Document Object Model.’ In simple terms, it is a structured representation of the HTML elements that are present in a webpage or web app. The DOM represents the entire UI of your application as a tree data structure, containing a node for each UI element in the web document. It is very useful as it allows web developers to modify content through JavaScript. Being in a structured format helps a lot because we can target specific elements, making the code much easier to work with.
Example
<!DOCTYPE html>
<html>
<head>
<title>Example</title>
</head>
<body>
<h1 id="heading">Hello, World!</h1>
<script>
const heading = document.getElementById('heading');
heading.textContent = 'Hello, DOM!';
</script>
</body>
</html>
In this example, JavaScript interacts with the DOM to change the text of the <h1>
element.
Why and When to Use It?
Direct Manipulation: Use the traditional DOM for straightforward, direct manipulation of the document structure.
Simple Applications: Ideal for static or simple web applications where performance is not a critical issue.
Disadvantages of Real DOM
Every time the DOM gets updated, the updated element and its children have to be rendered again to update the UI of our page. This means that each time there is a component update, the DOM needs to be updated, and the UI components have to be re-rendered, which can be inefficient.
Virtual DOM
What is Virtual DOM?
React uses the Virtual DOM, which is a lightweight copy of the actual DOM—a virtual representation of the DOM. So, for every object that exists in the original DOM, there is an object for that in the React Virtual DOM. It is exactly the same, but it does not have the power to directly change the layout of the document.
How Does the Virtual DOM Improve Performance?
Manipulating the DOM is slow, but manipulating the Virtual DOM is fast since nothing gets drawn on the screen. So, each time there is a change in the state of our application, the Virtual DOM gets updated first.
When anything new is added to the application, a Virtual DOM is created and represented as a tree. Each element in the application is a node in this tree.
Whenever there is a change in the state of any element, a new Virtual DOM tree is created.
This new Virtual DOM tree is then compared with the previous Virtual DOM tree, and the changes are noted.
The best possible ways to make these changes to the real DOM are determined, and only the updated elements get rendered on the page again.
Example
jsxCopy codeimport React, { useState } from 'react';
import ReactDOM from 'react-dom';
function App() {
const [count, setCount] = useState(0);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
Shadow DOM
What is Shadow DOM?
Shadow DOM is a term used to describe a limited or restricted view of the DOM tree. Unlike the Virtual DOM, which is a concept used to optimize performance, Shadow DOM refers to a specific structure within the DOM tree itself.
Example
htmlCopy code<!DOCTYPE html>
<html>
<body>
<my-element></my-element>
<script>
class MyElement extends HTMLElement {
constructor() {
super();
// Attach a shadow DOM to this element
const shadow = this.attachShadow({ mode: 'open' });
// Create and append a style element
const style = document.createElement('style');
style.textContent = `
h1 {
color: blue;
}
`;
shadow.appendChild(style);
// Create and append an h1 element
const h1 = document.createElement('h1');
h1.textContent = 'Hello from Shadow DOM!';
shadow.appendChild(h1);
}
}
// Define the new element
customElements.define('my-element', MyElement);
</script>
</body>
</html>
In this example:
Custom Element: We define a custom HTML element
<my-element>
.Shadow DOM Attachment: We attach a shadow DOM to this element using
this.attachShadow({ mode: 'open' })
.Scoped Styles: We create a style element inside the shadow DOM to style the content within the shadow tree.
Shadow Tree Content: We create and append an
<h1>
element to the shadow DOM.
The styles applied within the shadow DOM are scoped to the shadow host (<my-element>
) and do not affect any other part of the document.
Subscribe to my newsletter
Read articles from Vaishnavi Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by