Mastering Cookies Management in API Testing
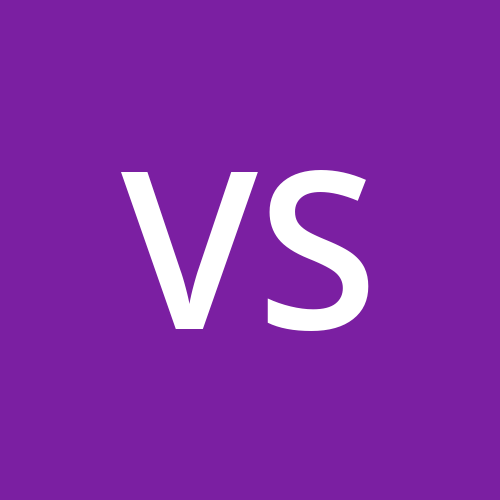
In API testing, managing cookies effectively is crucial for ensuring accurate session handling and security validations. Cookies play an essential role in maintaining stateful interactions between the client and the server, and their correct management can make or break your test outcomes. Here’s a closer look at why cookies are important and how to handle them in API testing:
🔍 Why Cookies Matter in API Testing?
Cookies are vital for:
Session Management: Keeping track of user sessions, ensuring that interactions are stateful.
Security Testing: Validating session expiration, secure cookie attributes, and session hijacking scenarios.
User-Specific Testing: Ensuring that personalized data is handled correctly across sessions.
Handling Cookies in API Testing with RestAssured
RestAssured is a powerful tool for API automation that makes cookie management straightforward and efficient.
1. Setting Cookies: Injecting cookies into your API requests is simple with RestAssured, allowing you to simulate various user scenarios.
RestAssured.given()
.cookie("sessionId", "abc123")
.when()
.get("/api/endpoint")
.then()
.statusCode(200);
2. Extracting Cookies: Capturing cookies from the server response helps in maintaining session continuity across multiple API calls.
response = RestAssured.get("/api/endpoint");
Map<String, String> cookies = response.getCookies();
for (Map.Entry<String, String> cookie : cookies.entrySet()) {
System.out.println(cookie.getKey() + " -> " + cookie.getValue());
}
3. Validating Cookies: Ensure that cookies meet security standards and behave as expected.
response = RestAssured.get("/api/endpoint");
String secureCookie = response.getCookie("secureToken");
assert secureCookie != null : "Secure cookie is missing!";
🌟 Best Practices for Cookie Management
Consistency: Maintain a consistent approach to capturing and setting cookies across your test cases.
Security: Regularly validate cookie attributes (e.g., HttpOnly, Secure) to ensure compliance with security best practices.
Modularity: Create reusable functions for cookie handling to simplify and streamline your test scripts.
By mastering cookie management in API testing, you can significantly enhance the reliability and accuracy of your automated tests. Proper handling ensures that your application’s stateful interactions are tested thoroughly, leading to higher-quality software and better user experiences.
#APITesting #CookiesManagement #AutomationTesting #RestAssured #QualityAssurance #SoftwareTesting #SessionManagement
Subscribe to my newsletter
Read articles from Vanshika Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
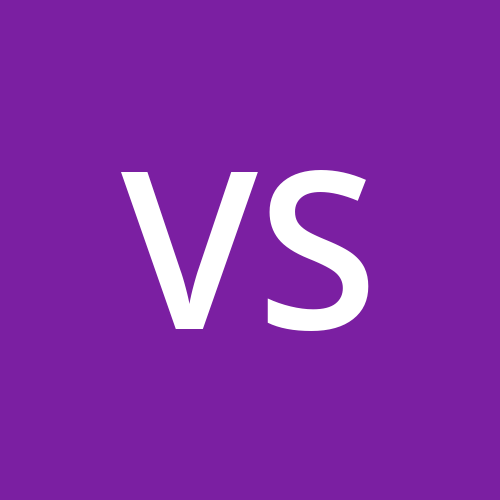