React Class Components vs. Function Components: State Management
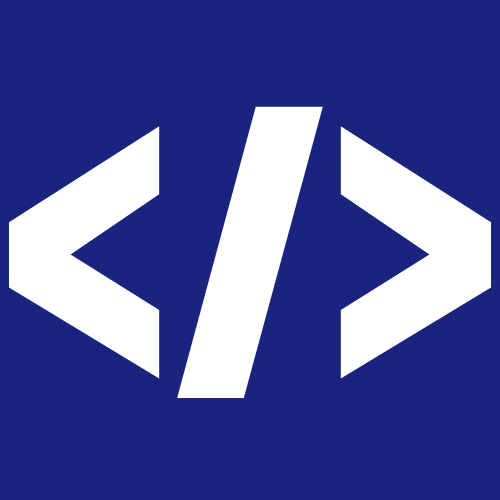
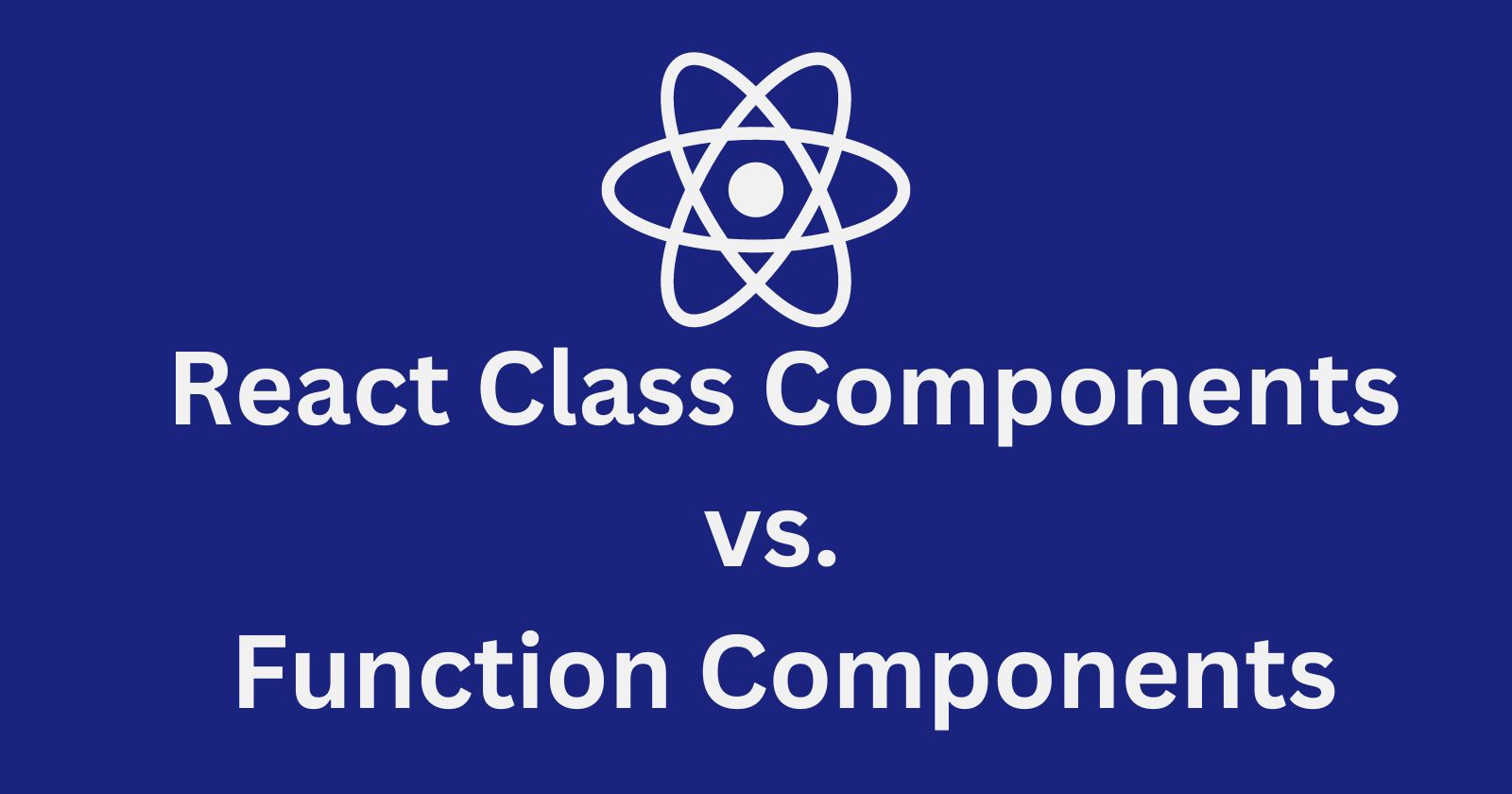
React has evolved significantly since its inception, with function components becoming increasingly popular due to the introduction of hooks. One of the critical aspects of this evolution is how state management differs between class components and function components. In this blog post, we'll explore these differences and discuss the advantages and disadvantages of each approach.
Class Components: State Management
Class components have been a fundamental part of React since its early days. They manage state using the this.state
object and the setState
method.
Example
Here’s an example of a simple counter implemented as a class component:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
this.state = { count: 0 }; // Initial state
// Binding methods
this.increment = this.increment.bind(this);
this.decrement = this.decrement.bind(this);
}
increment() {
this.setState(prevState => ({ count: prevState.count + 1 })); // Updating state
}
decrement() {
this.setState(prevState => ({ count: prevState.count - 1 })); // Updating state
}
render() {
return (
<div>
<h1>Count: {this.state.count}</h1>
<button onClick={this.increment}>Increment</button>
<button onClick={this.decrement}>Decrement</button>
</div>
);
}
}
export default Counter;
Advantages of Class Components
- Familiarity: Many developers are familiar with object-oriented programming, making it easier to understand and use class components.
- Lifecycle Methods: Class components provide built-in lifecycle methods like
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
, making it easier to manage side effects. - Single Place for State: State is centralized in one place (
this.state
), making it easy to understand the current state of the component.
Disadvantages of Class Components
- Verbosity: Class components can be verbose, requiring more boilerplate code (e.g., constructor, method binding).
- Complexity: Managing state and lifecycle methods can become complex, especially in large components.
- Performance: Class components can be less performant than function components due to the overhead of
this
binding and other object-oriented features.
Function Components: State Management with Hooks
Function components were initially stateless, but the introduction of hooks, particularly useState
and useEffect
, allowed them to manage state and side effects.
Example
Here’s the same counter example implemented as a function component:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0); // Initial state
const increment = () => {
setCount(prevCount => prevCount + 1); // Updating state
};
const decrement = () => {
setCount(prevCount => prevCount - 1); // Updating state
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
export default Counter;
Advantages of Function Components
- Simplicity: Function components are simpler and require less boilerplate code than class components.
- Hooks: Hooks provide a powerful and flexible way to manage state and side effects, promoting better code reuse and separation of concerns.
- Performance: Function components are generally more performant due to the lack of
this
binding and other object-oriented overhead. - Readability: The code is often more readable and easier to understand, especially for those familiar with functional programming concepts.
Disadvantages of Function Components
- Learning Curve: Developers need to learn and understand hooks, which can have a steeper learning curve for those new to React or functional programming.
- Complexity with Multiple Hooks: Managing complex state and side effects with multiple hooks can become challenging and sometimes lead to "hook hell."
Key Differences
State Initialization
- Class Components: State is initialized in the constructor using
this.state
. - Function Components: State is initialized using the
useState
hook directly in the function body.
State Updates
- Class Components: State updates are done using the
setState
method, which can merge state updates. - Function Components: State updates are done using the state setter function returned by
useState
, which replaces the state rather than merging.
Lifecycle Methods
- Class Components: Provide built-in lifecycle methods for managing side effects.
- Function Components: Use the
useEffect
hook to manage side effects, which can handle componentDidMount, componentDidUpdate, and componentWillUnmount in one function.
Boilerplate Code
- Class Components: Require more boilerplate, such as constructors and method binding.
- Function Components: Require less boilerplate, making the code cleaner and more concise.
Conclusion
Both class components and function components have their strengths and weaknesses when it comes to state management in React. Class components offer familiarity and built-in lifecycle methods, while function components provide simplicity, performance benefits, and the power of hooks.
In modern React development, function components with hooks are generally preferred due to their simplicity and flexibility. However, understanding both approaches is crucial for maintaining and upgrading existing React codebases and for making informed decisions in new projects.
Subscribe to my newsletter
Read articles from Nadim Anwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
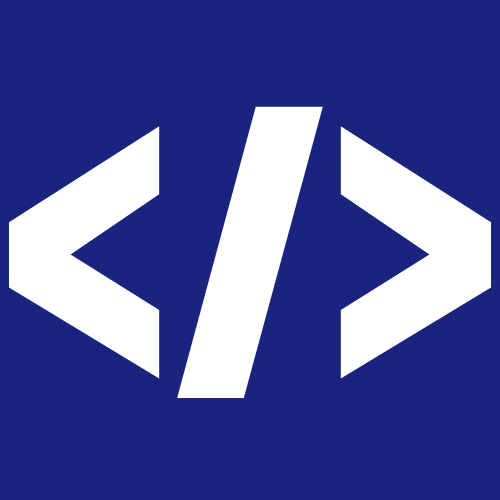
Nadim Anwar
Nadim Anwar
As an experienced front-end developer, I have expertise in building scalable and responsive web applications using modern technologies like ReactJS, Next.js, and TailwindCSS. With a solid understanding of JavaScript and a strong design sense, I excel at turning concepts into visually appealing and practical user interfaces. I am committed to continuously enhancing my skills and staying abreast of the latest industry trends to provide cutting-edge solutions.