Optimizing Lambda Performance: Advanced Techniques for Reducing Cold Starts and Enhancing Execution Efficiency
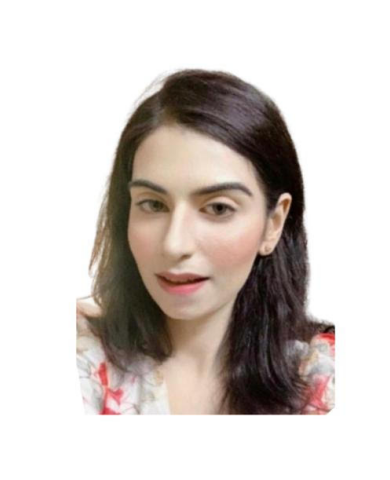
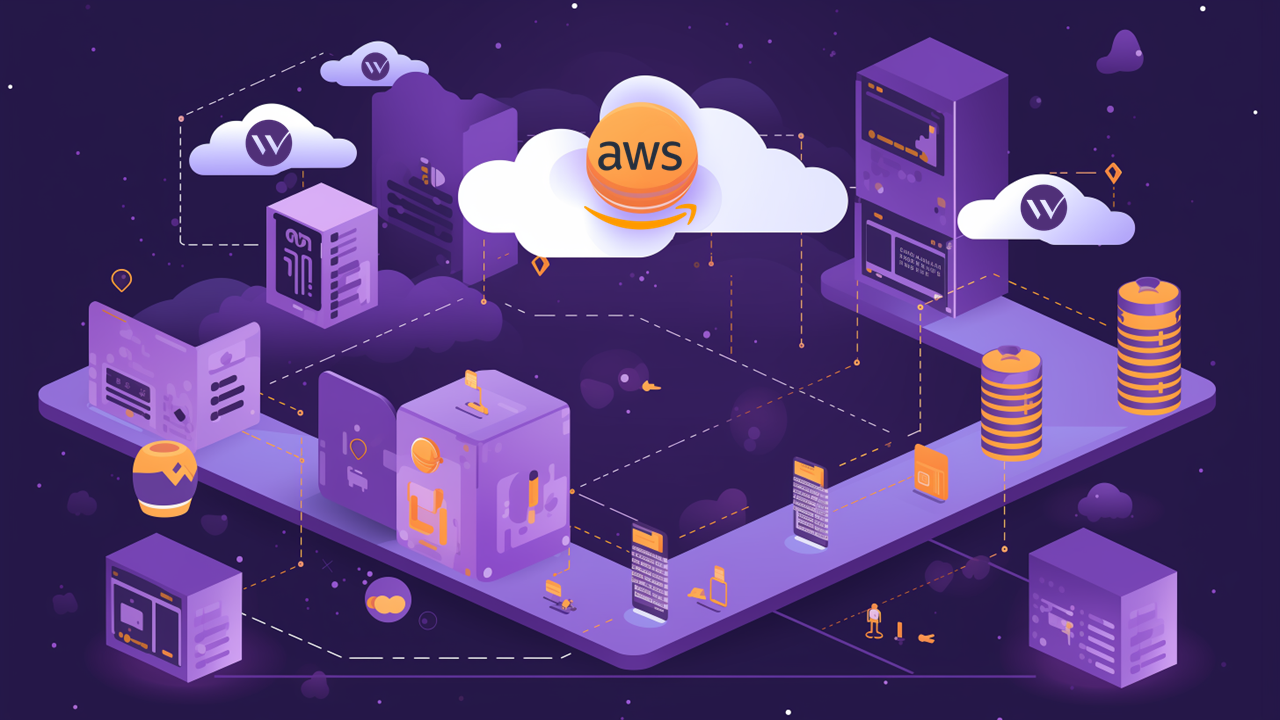
Hello there!
Lambda has been an absolute game changer for serverless architecture, but optimizing its performance is crucial for applications requiring high responsiveness and low latency.
In this post, I'll share advanced techniques for reducing cold starts and enhancing execution efficiency, drawing from my experiences and a fascinating case study on how Amazon Prime Video optimizes Lambda for their streaming services.
Understanding Cold Starts
A cold start occurs when a Lambda function is invoked after being inactive, leading to additional latency as AWS provisions a new container and initializes the runtime environment. This can significantly impact performance, particularly for latency-sensitive applications.
Techniques to Reduce Cold Starts
Provisioned Concurrency
Keeps a specified number of instances warm and ready to handle requests. Allocate provisioned concurrency for critical Lambda functions to ensure they are always warm.
Benefits: Significantly reduces latency by eliminating cold starts.
aws_lambda_function:
reserved_concurrent_executions: 10
Lambda Warmer
A custom solution that periodically invokes Lambda functions to keep them warm. Create a Lambda function that triggers other functions at regular intervals.
Benefits: Cost-effective way to reduce cold starts without using provisioned concurrency.
import boto3
client = boto3.client('lambda')
def lambda_warmer(event, context):
response = client.invoke(
FunctionName='your-lambda-function-name',
InvocationType='RequestResponse'
)
Optimizing Package Size
Remove unnecessary dependencies, use Lambda layers, and minimize the codebase.
Benefits: Faster cold start times as smaller packages are quicker to load and initialize.
pip install -t ./package boto3
cd package
zip -r ../deployment-package.zip .
cd ..
zip -g deployment-package.zip lambda_function.py
Environment Variables and Initialization Code
Use environment variables for configuration and minimize the code executed during initialization.
Benefits: Reduces the time taken for the function to become ready to handle requests.
import os
db_host = os.getenv('DB_HOST')
db_user = os.getenv('DB_USER')
Enhancing Execution Efficiency
Memory Allocation
Allocating appropriate memory to Lambda functions. Adjust memory settings based on the function's needs. Higher memory allocations provide more CPU power.
Benefits: Faster execution times and potentially lower costs due to reduced execution duration.
aws_lambda_function:
memory_size: 1024
Optimizing Code Performance
Writing efficient and optimized code. Use asynchronous processing, avoid blocking operations, and leverage efficient data structures.
Benefits: Faster execution and reduced resource consumption.
async def handler(event, context):
response = await some_async_function()
return response
Using AWS Lambda Power Tuning
A tool to determine the optimal memory configuration. Use the AWS Lambda Power Tuning tool to benchmark different memory configurations and choose the best one.
Benefits: Optimal balance between performance and cost.
./power-tuning.sh --region us-west-2 --function my-lambda-function --payload '{"key": "value"}'
Case Study: Amazon Prime Video
Background
Amazon Prime Video is a popular streaming service that leverages AWS Lambda to handle various backend processes. Ensuring a seamless streaming experience requires high performance and low latency.
Challenges
High traffic during peak times.
Need for low latency in streaming services.
Efficiently handling a large number of requests.
Strategies Employed
Provisioned Concurrency
Prime Video allocates provisioned concurrency for critical Lambda functions, ensuring they are always ready to handle requests without delay.
Efficient Code Optimization
The engineering team focuses on optimizing the codebase, using efficient algorithms, and minimizing the size of deployment packages to enhance performance.
Asynchronous Processing
Leveraging asynchronous processing wherever possible to handle requests more efficiently and improve the overall responsiveness of the service.
Advanced Monitoring and Logging
Implementing detailed monitoring and logging to quickly identify and address performance bottlenecks.
Utilizing Lambda Layers
By leveraging Lambda layers, Prime Video separates common dependencies into reusable layers, reducing the overall size of individual function deployments and improving load times.
Optimizing Initialization Code
Prime Video minimizes the initialization code executed during function startup, ensuring that functions become ready to handle requests more quickly.
Warm Starts Through Traffic Shaping
Implementing traffic shaping to ensure that critical functions receive steady, low levels of traffic, keeping them warm without overloading the system.
Outcomes
Significant reduction in cold start latency.
Enhanced execution efficiency leading to a smoother streaming experience.
Improved ability to handle high traffic volumes during peak times.
Increased overall system reliability and performance.
Trade-offs and Nuances
Trade-offs in Optimization Strategies
While optimizing AWS Lambda performance, it’s crucial to balance various trade-offs.
Cost vs. Performance
Provisioned Concurrency: Ensures low latency but incurs additional costs.
Lambda Warmer: Cost-effective but may not be as reliable as provisioned concurrency.
Simplicity vs. Control
- Managed Services: Simplify operations but may offer less control compared to custom solutions.
Further Optimizations
Minimize Dependencies
Only include essential dependencies in your Lambda package to reduce the deployment size.
Optimize Cold Start Times
Use minimal initialization code and leverage AWS SDK's async capabilities.
Effective Caching
Use caching mechanisms like Amazon ElastiCache to reduce repetitive data fetching operations.
Network Optimization
Use AWS VPC endpoints to reduce latency and improve data transfer rates.
Sample Snippet for Performance Optimization
import boto3
import os
import json
dynamodb = boto3.resource('dynamodb', region_name='us-west-2')
table = dynamodb.Table(os.environ['DYNAMODB_TABLE'])
def lambda_handler(event, context):
# Optimize initialization code
if 'httpMethod' not in event:
return {"statusCode": 400, "body": "Invalid Request"}
# Efficient data handling
response = table.scan()
data = response['Items']
return {
"statusCode": 200,
"body": json.dumps(data)
}
Note: The above code demonstrates efficient data handling and optimized initialization for reduced cold start times.
Conclusion
Optimizing AWS Lambda performance is crucial for applications that demand high responsiveness and low latency. By employing techniques such as provisioned concurrency, lambda warming, optimizing package sizes, and efficient code practices, developers can significantly reduce cold starts and enhance execution efficiency. The case study of Amazon Prime Video illustrates how these strategies can be successfully implemented to achieve optimal performance in a real-world scenario.
Further Reading and Resources
Call to Action
Stay tuned for more in-depth articles and case studies on optimizing cloud infrastructure, improving system performance, and ensuring the highest levels of security. Subscribe to Cloud Design Diaries for the latest updates and insights from the world of cloud computing.
Feel free to connect atLinkedIn Profile
Subscribe to my newsletter
Read articles from Tanishka Marrott directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
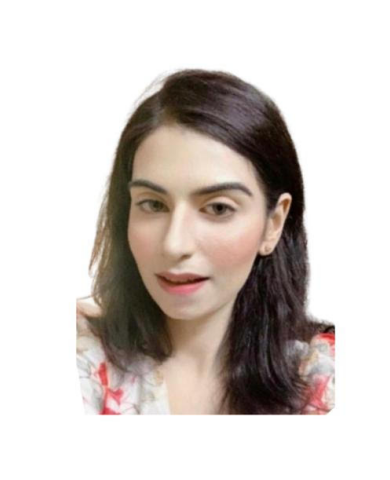
Tanishka Marrott
Tanishka Marrott
I'm a results-oriented cloud architect passionate about designing resilient cloud solutions. I specialize in building scalable architectures that meet business needs and are agile. With a strong focus on scalability, performance, and security, I ensure solutions are adaptable. My DevSecOps foundation allows me to embed security into CI/CD pipelines, optimizing deployments for security and efficiency. At Quantiphi, I led security initiatives, boosting compliance from 65% to 90%. Expertise in data engineering, system design, serverless solutions, and real-time data analytics drives my enthusiasm for transforming ideas into impactful solutions. I'm dedicated to refining cloud infrastructures and continuously improving designs. If our goals align, feel free to message me. I'd be happy to connect!