SQL Made Easy: Step-by-Step Guide from Basics to Advanced
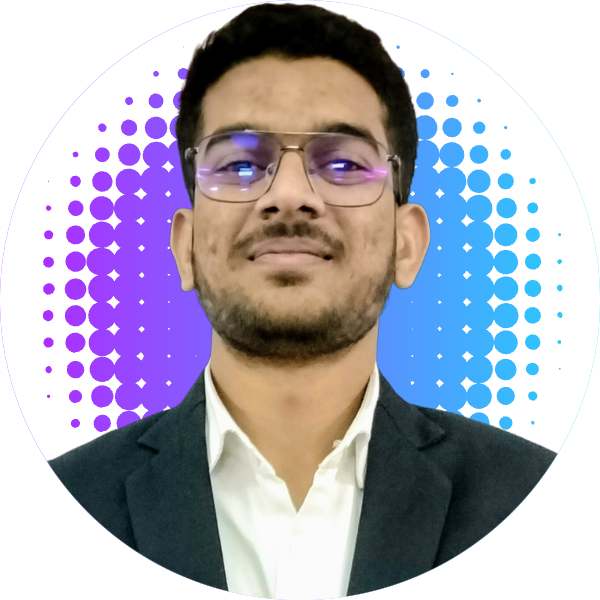
Table of contents
- 1. Introduction to SQL
- 2. SQL Basics
- 3. Advanced SQL Queries
- 4. SQL Performance Tuning
- 5. Common SQL Functions
- 6. SQL Joins Explained
- 7. SQL for Data Analysis
- 8. Working with SQL Databases
- 9. SQL Best Practices
- 10. SQL and Security
- 11. Case Studies and Real-World Applications
- 12. SQL vs NoSQL
- 13. SQL on Cloud Platforms
- 14. SQL in Data Science
- 15. Introduction to SQL Window Functions
- Conclusion
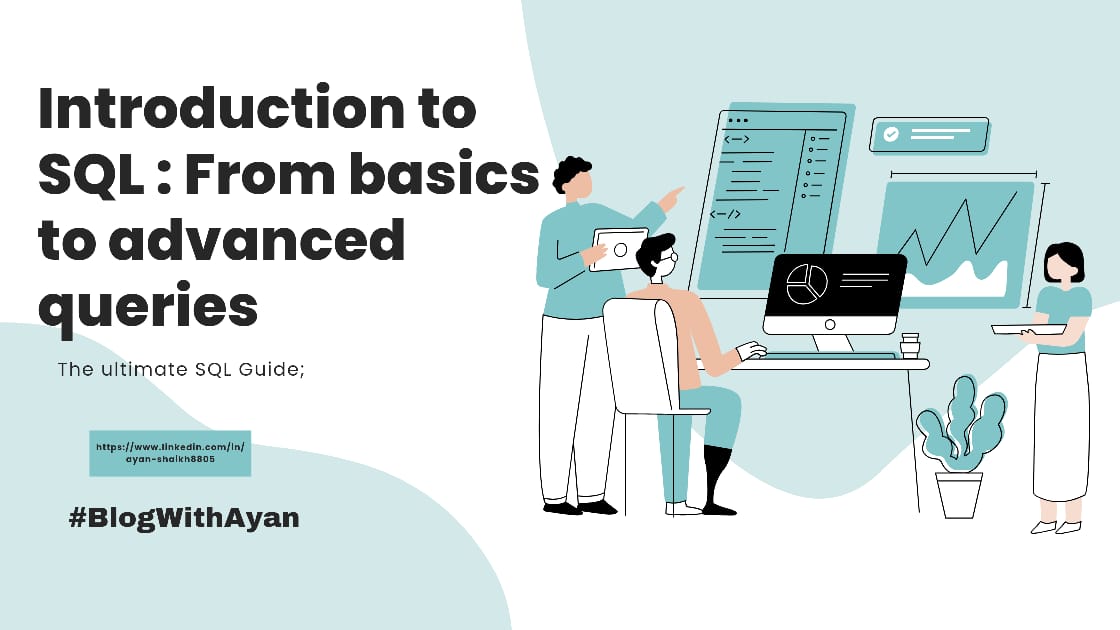
In today's data-driven world, understanding and utilizing databases is paramount for businesses, developers, and data analysts. At the heart of these databases lies SQL, or Structured Query Language. SQL is a powerful tool used to interact with and manipulate relational databases. Whether you're new to SQL or looking to sharpen your skills, this article will guide you through the essentials and advanced aspects of SQL, showcasing why it remains a cornerstone of data management.
1. Introduction to SQL
SQL stands for Structured Query Language, a standard language for managing and manipulating relational databases. It was initially developed in the 1970s by IBM and has since become the standard for database management systems (DBMS). SQL allows users to create, read, update, and delete data within a database, making it an essential tool for data professionals.
2. SQL Basics
Before diving into advanced topics, it's important to understand the basics of SQL:
a) SELECT Statements: Retrieve data from one or more tables
SELECT * FROM employees;
b) WHERE Clauses: Filter results based on specific conditions
SELECT * FROM employees WHERE department = 'Sales';
c) ORDER BY: Sort results
SELECT * FROM employees ORDER BY last_name ASC;
d) LIMIT: Restrict the number of rows returned.
SELECT * FROM employees LIMIT 10;
e) INSERT, UPDATE, DELETE: Modify data within tables.
INSERT INTO employees (first_name, last_name, department) VALUES ('John', 'Doe', 'Marketing');
UPDATE employees SET department = 'Marketing' WHERE employee_id = 5;
DELETE FROM employees WHERE employee_id = 5;
3. Advanced SQL Queries
As you become more comfortable with SQL, you can explore more complex queries:
a) JOINs: Combine rows from two or more tables based on a related column.
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
b) Subqueries: Nested queries that can be used within SELECT, INSERT, UPDATE, or DELETE statements.
SELECT first_name, last_name
FROM employees
WHERE department_id = (
SELECT department_id
FROM departments
WHERE department_name = 'HR'
);
c) Nested Queries: Queries within queries to perform more complex operations.
SELECT * FROM (
SELECT first_name, last_name
FROM employees
) AS emp
WHERE emp.last_name LIKE 'S%';
4. SQL Performance Tuning
Optimizing SQL queries is essential for improving performance:
a) Indexes: Create indexes on columns to speed up search queries.
CREATE INDEX idx_last_name ON employees(last_name);
b) Query Execution Plans: Analyze and understand the execution plan of a query to identify bottlenecks.
EXPLAIN SELECT * FROM employees WHERE last_name = 'Smith';
c) Avoiding N+1 Problems: Optimize queries to reduce the number of database hits.
SELECT employees.first_name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.department_id;
d) Efficient Use of JOINs and Subqueries: Write efficient JOINs and subqueries to minimize processing time.
SELECT first_name, last_name
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE location = 'New York');
5. Common SQL Functions
SQL provides various functions to perform operations on data:
a) Aggregate Functions: Perform calculations on a set of values (e.g., SUM, AVG, COUNT).
SELECT COUNT(*) FROM employees WHERE department = 'Sales';
SELECT AVG(salary) FROM employees;
b) String Functions: Manipulate string values (e.g., CONCAT, LENGTH, SUBSTRING.
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM employees;
SELECT LENGTH(first_name) FROM employees;
SELECT SUBSTRING(first_name, 1, 3) FROM employees;
c) Date Functions: Handle date and time values (e.g., NOW, DATEADD, DATEDIFINE)
SELECT NOW();
SELECT DATEADD(year, 1, hire_date) FROM employees;
SELECT DATEDIFF(CURDATE(), hire_date) FROM employees;
6. SQL Joins Explained
Understanding the different types of joins is crucial for combining data from multiple tables:
a) INNER JOIN: Returns rows that have matching values in both tables.
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
b) LEFT JOIN (or LEFT OUTER JOIN): Returns all rows from the left table, and the matched rows from the right table. Unmatched rows will show NULL values.
SELECT employees.first_name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.department_id;
c) RIGHT JOIN (or RIGHT OUTER JOIN): Returns all rows from the right table, and the matched rows from the left table. Unmatched rows will show NULL values.
SELECT employees.first_name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.department_id;
d) FULL JOIN (or FULL OUTER JOIN): Returns all rows when there is a match in either left or right table. Unmatched rows will show NULL values.
SELECT employees.first_name, departments.department_name
FROM employees
FULL JOIN departments ON employees.department_id = departments.department_id;
7. SQL for Data Analysis
SQL is a powerful tool for data analysis:
a) GROUP BY: Group rows that have the same values in specified columns.
SELECT department, COUNT(*)
FROM employees
GROUP BY department;
b) HAVING: Filter groups based on conditions.
SELECT department, COUNT(*)
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
c) Window Functions: Perform calculations across a set of table rows related to the current row.
SELECT first_name, last_name, salary,
RANK() OVER (PARTITION BY department ORDER BY salary DESC) AS rank
FROM employees;
8. Working with SQL Databases
Managing databases and tables is fundamental:
a) Creating Databases and Tables: Use CREATE DATABASE and CREATE TABLE statements.
CREATE DATABASE company;
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department VARCHAR(50),
hire_date DATE
);
b)Altering Tables: Modify the structure of existing tables with ALTER TABLE.
ALTER TABLE employees ADD COLUMN salary DECIMAL(10, 2);
c) Dropping Tables: Remove tables with DROP TABLE.
DROP TABLE employees;
9. SQL Best Practices
Writing clean and efficient SQL code is essential, listed below is some of the best practices;
a) Consistent Naming Conventions: Use clear and consistent names for tables and columns.
b) Comments: Document your code with comments.
c) Avoiding SQL Injection: Use parameterized queries to protect against SQL injection attacks.
cursor.execute("SELECT * FROM employees WHERE department = %s", (department_name,))
10. SQL and Security
Securing SQL databases is critical:
a) User Permissions: Grant appropriate permissions to users.
GRANT SELECT, INSERT ON employees TO 'user'@'localhost';
b) SQL Injection Prevention: Use prepared statements and parameterized queries.
cursor.execute("SELECT * FROM employees WHERE department = %s", (department_name,))
c) Encryption: Encrypt sensitive data.
11. Case Studies and Real-World Applications
Let us understand how SQL is used in various industries:
a) E-commerce: Managing product catalogs and customer orders.
SELECT products.product_name, orders.order_date
FROM products
JOIN orders ON products.product_id = orders.product_id
WHERE orders.customer_id = 123;
b) Finance: Handling transactions and financial reporting.
SELECT account_id, SUM(transaction_amount) AS total_transactions
FROM transactions
WHERE transaction_date BETWEEN '2023-01-01' AND '2023-12-31'
GROUP BY account_id;
c) Healthcare: Storing patient records and medical history.
SELECT patients.first_name, patients.last_name, visits.visit_date, visits.diagnosis
FROM patients
JOIN visits ON patients.patient_id = visits.patient_id
WHERE patients.patient_id = 456;
d) Social Media: Managing user data and activity logs.
SELECT users.username, posts.post_content
FROM users
JOIN posts ON users.user_id = posts.user_id
WHERE users.user_id = 789;
12. SQL vs NoSQL
Understand the differences between SQL and NoSQL databases:
a) SQL Databases: Structured data, ACID transactions, predefined schema (e.g., MySQL, PostgreSQL).
SELECT * FROM employees WHERE department = 'Sales';
b) NoSQL Databases: Unstructured data, scalability, flexible schema (e.g., MongoDB, Cassandra).
db.employees.find({ department: 'Sales' });
c) Use Cases: When to choose SQL or NoSQL based on project requirements.
SQL for structured data and complex queries
NoSQL for flexible schema and high scalability
13. SQL on Cloud Platforms
Learn how to use SQL with cloud-based databases:
a) AWS RDS: Amazon's managed relational database service.
b) Google Cloud SQL: Managed database service for MySQL, PostgreSQL, and SQL Server.
c) Azure SQL Database: Managed relational database service by Microsoft.
14. SQL in Data Science
SQL is a key tool for data scientists:
a) Data Preprocessing: Clean and prepare data for analysis.
DELETE FROM employees WHERE last_name IS NULL;
b) Data Exploration: Query and analyze data to gain insights.
SELECT department, AVG(salary) AS average_salary
FROM employees
GROUP BY department;
c) Integration with Data Science Tools: Use SQL with Python, R, and other tools.
import pandas as pd
import sqlalchemy
engine = sqlalchemy.create_engine('postgresql://user:password@localhost:5432/mydatabase')
df = pd.read_sql('SELECT * FROM employees', engine)
15. Introduction to SQL Window Functions
Window functions allow advanced data analysis:
a) ROW_NUMBER(): Assigns a unique sequential integer to rows within a partition.
SELECT first_name, last_name,
ROW_NUMBER() OVER (ORDER BY hire_date) AS row_num
FROM employees;
b) RANK(): Assigns a rank to each row within a partition.
SELECT first_name, last_name, salary,
RANK() OVER (ORDER BY salary DESC) AS rank
FROM employees;
c) LEAD() and LAG(): Access data from subsequent and preceding rows.
SELECT first_name, last_name, hire_date,
LEAD(hire_date, 1) OVER (ORDER BY hire_date) AS next_hire_date
FROM employees;
Conclusion
SQL is more than just a language; it's the backbone of modern data management system. Understanding the concepts of SQL in this data-driven world empowers you to grab the full potential of your data, starting from simple queries to complex data manipulations. As you delve deeper into SQL, you'll discover its vast capabilities and its critical role in shaping how we interact with data and its management system.
Are you ready to elevate your SQL skills and stay ahead of the curve? If yes, then Follow my blog for more insights, tutorials, and tips on SQL and other tech topics. Let’s understand this data journey together—subscribe now and never miss a beat in the world of data management!
Happy querying, and see you in the next post!
Subscribe to my newsletter
Read articles from Ayan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
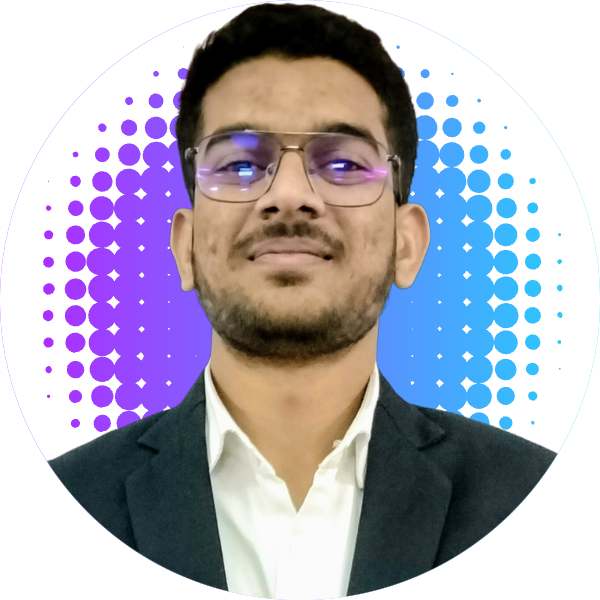
Ayan
Ayan
Hello! I'm Ayan Shaikh a passionate technologist with a diverse skill set in web applications, data science, machine learning, and cloud computing. I specialize in data science and Cloud computing. I also enjoy sharing my knowledge through technical writing, code reviews, and mentorship, helping developers improve their skills and practices. As an active member of Toastmasters International, I hone my public speaking skills and participate in tech conferences and webinars. Beyond tech, I'm a mental health advocate and peer counselor, supporting individuals with empathy and understanding. In my free time, I explore advancements in data science, machine learning, and cloud computing. Feel free to connect if you'd like to collaborate or chat about tech, mental health, or anything in between!