Day 17 Guide: Learning YAML and JSON
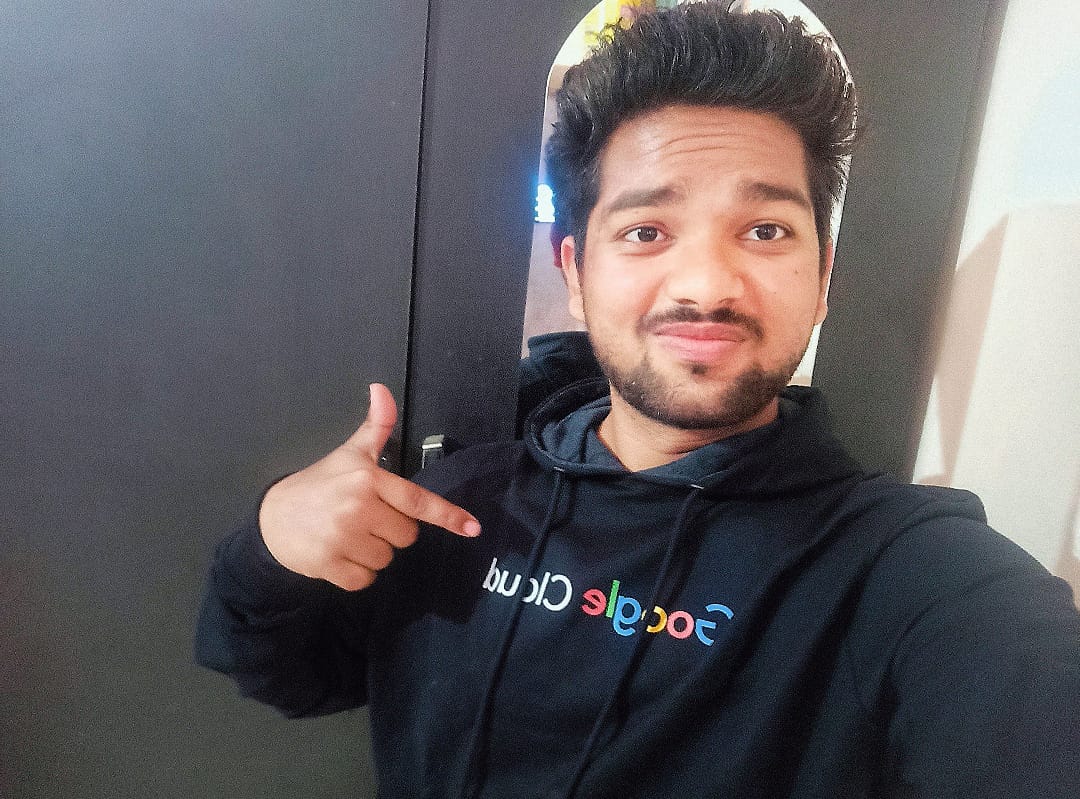
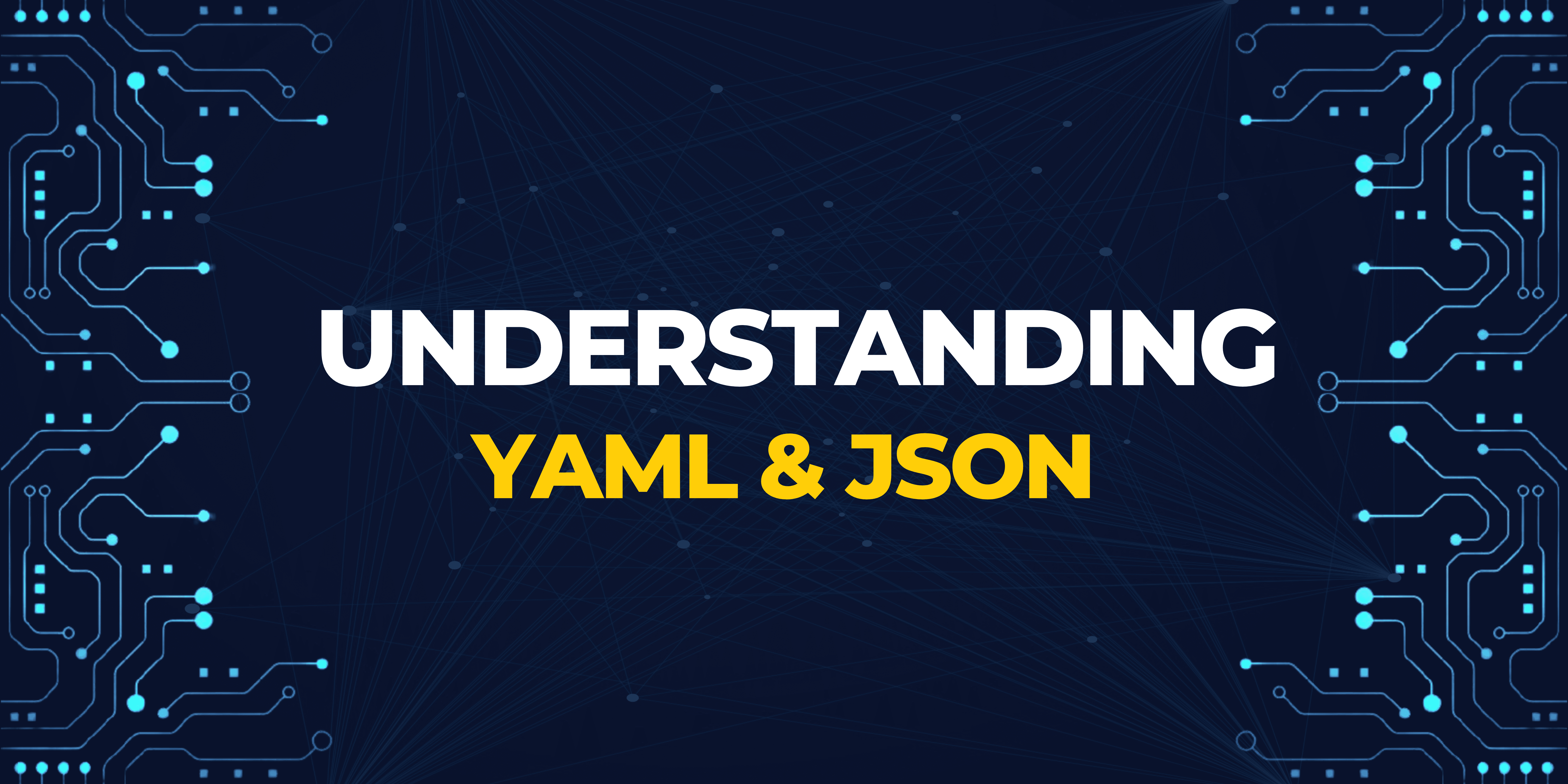
Today, we're diving into the practical side of Python for DevOps engineers. Python is not just a programming language but a powerful tool in the DevOps toolkit. Let's explore two essential tasks: reading JSON and YAML files.
Reading and Writing JSON Files in Python:
JSON (JavaScript Object Notation) is a popular format for data exchange between systems. As a DevOps engineer, you often need to parse and manipulate JSON data. Python provides a straightforward way to work with JSON through the json
module.
Task 1: Create a Dictionary and Write it to a JSON File
First, let's create a Python dictionary and write it into a JSON file. A dictionary in Python is a collection of key-value pairs enclosed in curly braces {}
.
import json
# Create a dictionary
data = {
"aws": "ec2",
"azure": "VM",
"gcp": "compute engine"
}
# Write dictionary to a JSON file
with open('services.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
In this example:
We import the
json
module.Define a dictionary
data
with cloud service providers and their respective services.Use
json.dump()
to write the dictionarydata
into a file namedservices.json
with an indentation of 4 spaces for readability.
Reading JSON Files
Now, let's read the services.json
file and print the service names for each cloud service provider.
# Read JSON file and print service names
with open('services.json', 'r') as json_file:
data = json.load(json_file)
for provider, service in data.items():
print(f"{provider}: {service}")
Output:
aws: ec2
azure: VM
gcp: compute engine
Reading YAML Files in Python
YAML (YAML Ain't Markup Language) is another human-readable data serialization standard. Python supports YAML through the PyYAML
library, which needs to be installed separately.
Task 2: Read a YAML File and Convert it to JSON
To work with YAML files, install the PyYAML
library using pip install pyyaml
. Then, let's read a YAML file (services.yaml
) and convert its contents to JSON.
import yaml
# Read YAML file and convert to JSON
with open('services.yaml', 'r') as yaml_file:
yaml_data = yaml.safe_load(yaml_file)
# Convert YAML data to JSON format
json_data = json.dumps(yaml_data, indent=4)
print(json_data)
In this task:
We import the
yaml
module.Open
services.yaml
file and load its contents usingyaml.safe
_load()
.Convert YAML data to JSON format using
json.dumps()
for easy manipulation.
When to Use JSON vs. YAML? ๐ค
JSON: Use JSON when you need a data format that's easy for machines to parse and commonly used in web applications and APIs.
YAML: Use YAML for configuration files and when you need a more human-readable format with support for comments.
Conclusion ๐
Understanding JSON and YAML is a must for any DevOps engineer. JSON is great for data exchange due to its lightweight and readable format, while YAML is perfect for configuration management with its human-readable and concise syntax. By mastering these formats and knowing how to use them in Python, you can make managing configurations and automating tasks a whole lot easier.
Feel free to reach out if you have any questions or want to explore more topics in DevOps! ๐
Subscribe to my newsletter
Read articles from Ritesh Dolare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
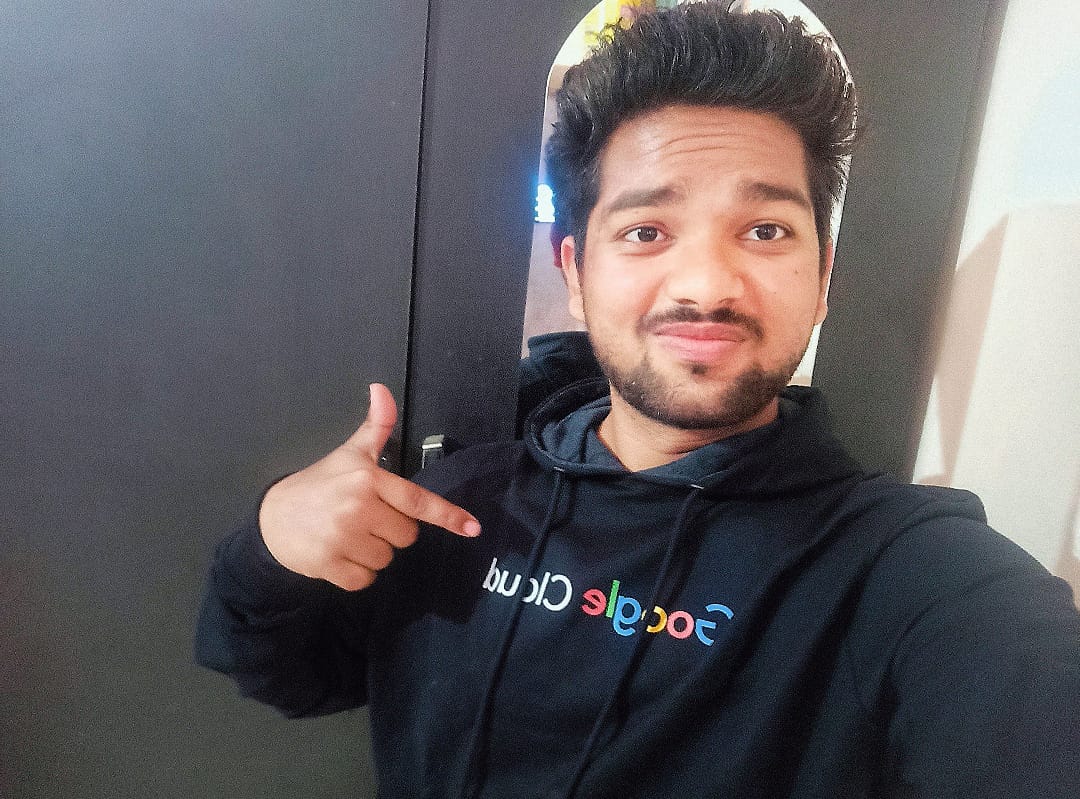
Ritesh Dolare
Ritesh Dolare
๐ Hi, I'm Ritesh Dolare, a DevOps enthusiast dedicated to mastering the art of DevOps.