Understanding Synchronous vs Asynchronous in Node.js: A Beginner's Guide
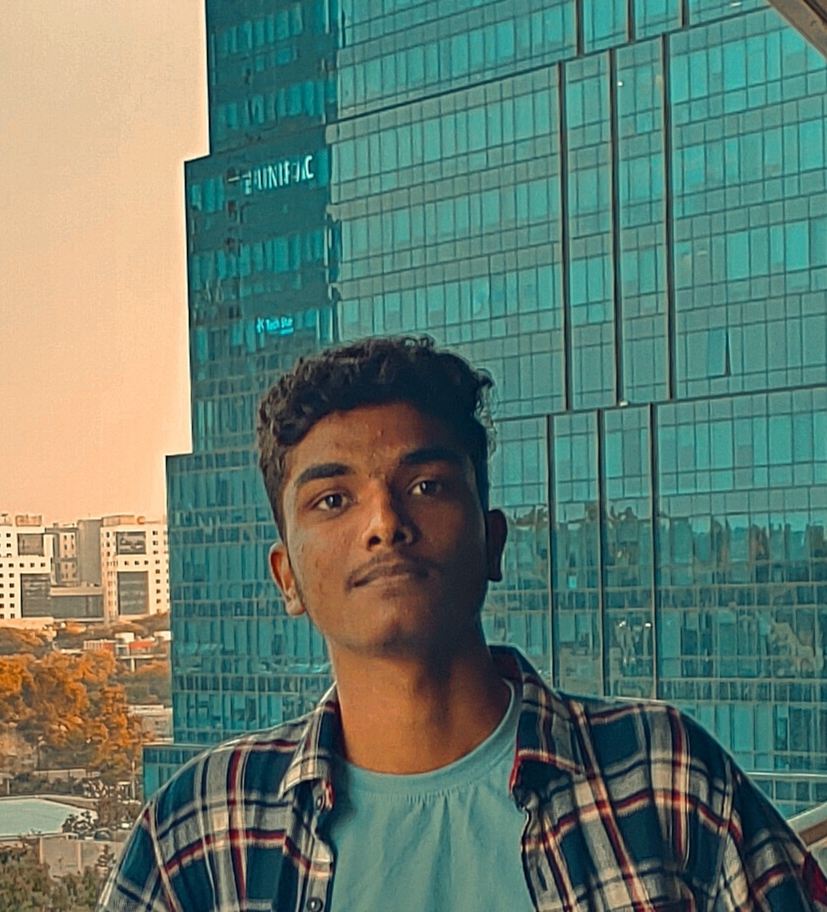
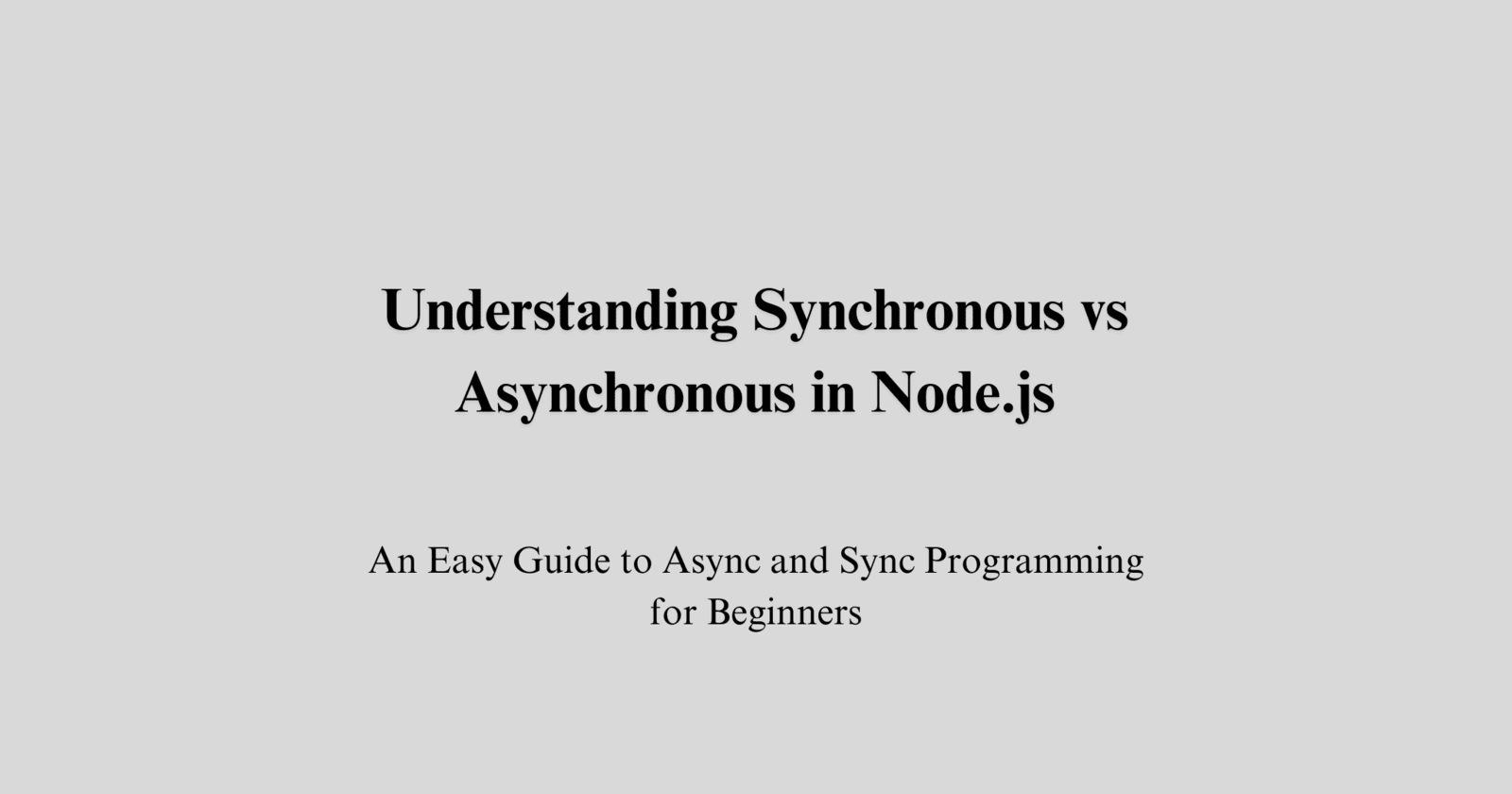
Node.js is a powerful and popular runtime environment that allows developers to run JavaScript on the server side. One of its key features is its ability to handle various asynchronous operations, which can greatly improve the performance and responsiveness of web applications.
In this blog, we'll explore the concepts of synchronous
and asynchronous
programming in Node.js, understand their differences, and see why asynchronous operations are so crucial.
Understanding Synchronous Operations
In synchronous programming
, tasks are executed one after another. Each operation waits for the previous one to complete before starting. This is straightforward and easy to understand but can lead to inefficiencies, especially in web applications that handle multiple requests or need to perform time-consuming operations.
Example of Synchronous Code:
function task1() {
console.log("Task 1: Starting");
for (let i = 0; i < 1e9; i++) {} // Simulating a time-consuming task
console.log("Task 1: Completed");
}
function task2() {
console.log("Task 2: Starting");
console.log("Task 2: Completed");
}
task1();
task2();
In this example, task2
will not start until task1
has finished, making the second task wait unnecessarily.
Understanding Asynchronous Operations
Asynchronous programming
allows tasks to run independently of the main program flow. Instead of waiting for a task to complete, the program can continue executing other tasks, making it much more efficient. This is especially useful for I/O operations, like reading from or writing to a database, making network requests, or handling user input.
Example of Asynchronous Code:
function task1() {
console.log("Task 1: Starting");
setTimeout(() => {
console.log("Task 1: Completed");
}, 1000); // Simulating a time-consuming task with a delay
}
function task2() {
console.log("Task 2: Starting");
console.log("Task 2: Completed");
}
task1();
task2();
In this example, task2
starts immediately after task1
starts, without waiting for the delay in task1
to finish. This non-blocking behavior makes asynchronous programming more efficient.
Callback Functions
One common way to handle asynchronous operations in Node.js is through callback functions. A callback function
is passed as an argument to another function and is executed after the completion of that function.
Example of Using Callbacks:
function task1(callback) {
console.log("Task 1: Starting");
setTimeout(() => {
console.log("Task 1: Completed");
callback(); // Call the callback function after task1 is done
}, 1000);
}
function task2() {
console.log("Task 2: Starting");
console.log("Task 2: Completed");
}
task1(task2);
Here, task2
is passed as a callback to task1
and will only run after task1
is completed.
Promises
Promises provide a cleaner way to handle asynchronous operations. A Promise
represents a value that may be available now, or in the future, or never. It can be in one of three states: pending, fulfilled, or rejected.
Example of Using Promises:
function task1() {
return new Promise((resolve) => {
console.log("Task 1: Starting");
setTimeout(() => {
console.log("Task 1: Completed");
resolve(); // Resolve the promise when task1 is done
}, 1000);
});
}
function task2() {
console.log("Task 2: Starting");
console.log("Task 2: Completed");
}
task1().then(task2);
In this example, task2
will run after the promise returned by task1
is resolved.
Async/Await
Async/await
is a modern syntax that makes working with Promises easier and more readable. It allows you to write asynchronous code as if it were synchronous.
Example of Using Async/Await:
async function main() {
console.log("Task 1: Starting");
await new Promise((resolve) => setTimeout(resolve, 1000));
console.log("Task 1: Completed");
console.log("Task 2: Starting");
console.log("Task 2: Completed");
}
main();
In this example, await
pauses the execution of main
until the promise is resolved, making the code look straightforward and easy to follow.
Conclusion
Understanding the difference between synchronous and asynchronous operations is essential for creating efficient Node.js applications. Synchronous operations are easy to use but can slow things down, whereas asynchronous operations—managed with callbacks, Promises, or async/await—can make your apps run faster and more smoothly. By learning these concepts, you'll be able to harness Node.js's full potential and build strong, scalable web applications.
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
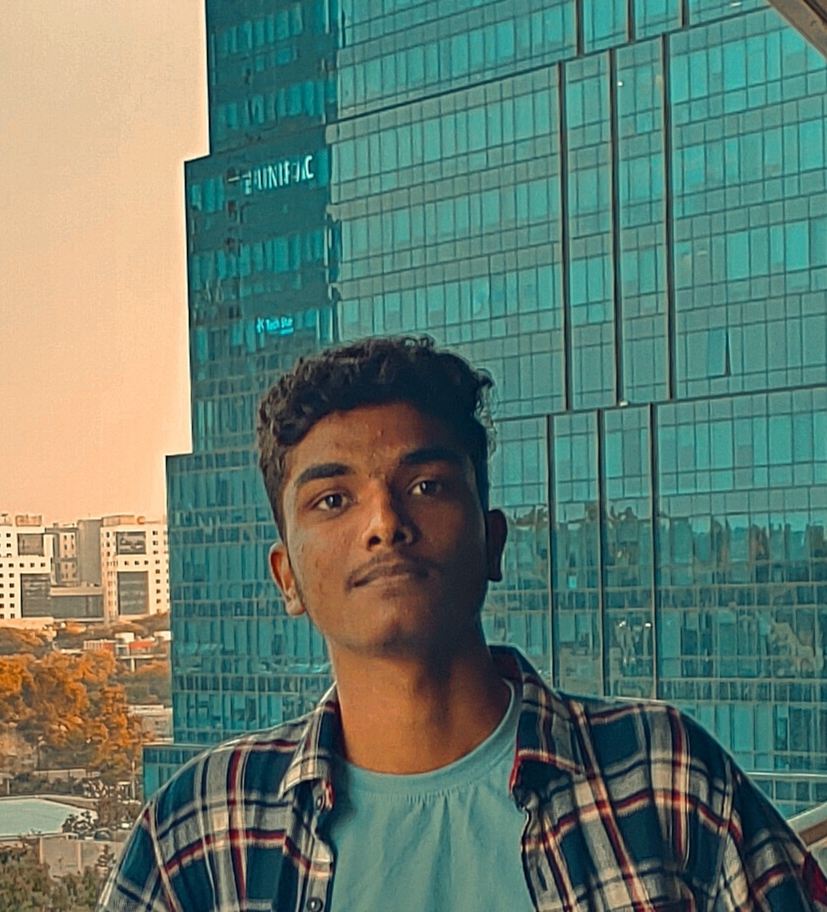
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.