Simple Substitution Cipher Helper - codewars
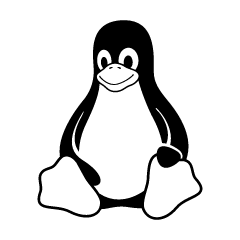
Table of contents
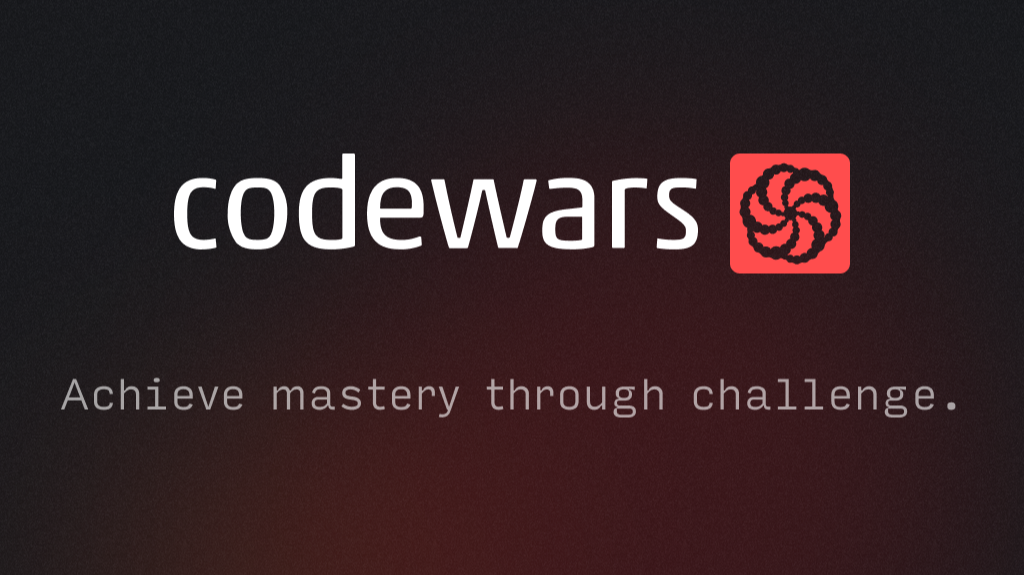
This problem is currently rated as a 6 kyu (ratings on codewars range from 8 (easiest) to 1 (hardest)) problem on codewars, this is its description :
“A simple substitution cipher replaces one character from an alphabet with a character from an alternate alphabet, where each character’s position in an alphabet is mapped to the alternate alphabet for encoding or decoding.”
My thoughts before solving :
“I can make translations for encoding and decoding respectively, then solve by returning the translation.”
My python solution:
class Cipher(object):
def __init__(self, map1, map2):
self.e = str.maketrans(map1,map2)
self.d = str.maketrans(map2,map1)
def encode(self, s):
return s.translate(self.e)
def decode(self, s):
return s.translate(self.d)
We start by assigning, e: dict, d: dict for our encode and decode translations. (We use str.maketrans to generate a dictionary which we can use for translation. It is equal to running the code below.)
self.e = {ord(x):ord(map2[y]) for y,x in enumerate(map1)}
self.d = {ord(x):ord(map1[y]) for y,x in enumerate(map2)}
For encoding we return the string translated with our earlier assigned e: dict using str.translate. We do the same for decoding.
(We use str.translate for replacing multiple characters in a string. It does this by using a dictionary full of key value pairs where the key is the character and the value is the replacement. You can get the ascii value of a specific character using the builtin ord() function. For getting a character from ascii value, you use chr() )
In conclusion
That is how I solved ‘Simple Substitution Cipher Helper’ on codewars, hopefully this helps you improve!
— 0xsweat 7/17/2024
My socials :
Subscribe to my newsletter
Read articles from 0xsweat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
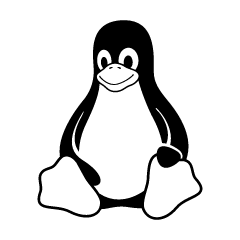
0xsweat
0xsweat
I am a machine that converts hours awake into problems solved at an exponential rate.