Chapter 4: Lifecycle Methods and Hooks
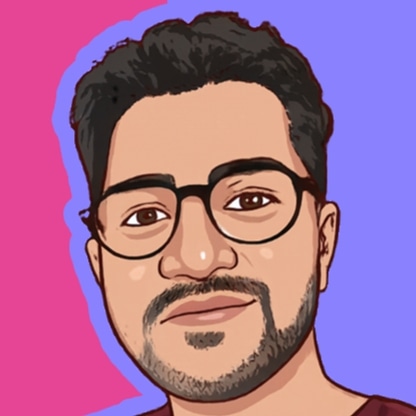
Welcome back to "React Revolution"! In the last chapter, we explored state management in React, a fundamental concept for creating interactive applications. Today, we're diving into another essential topic. These allow you to control what happens at different stages of a component's life, making your applications more robust and efficient.
4.1 What are Lifecycle Methods?
Lifecycle methods are special methods in React class components that get called at different stages of a component's existence. These stages include mounting, updating, and unmounting.
Here's a quick overview:
Mounting: When a component is being inserted into the DOM.
Updating: When a component is being re-rendered due to changes in props or state.
Unmounting: When a component is being removed from the DOM.
4.2 Lifecycle Methods in Class Components
Let's create a simple class component to illustrate some key lifecycle methods:
import React, { Component } from 'react';
class LifecycleDemo extends Component {
constructor(props) {
super(props);
console.log('Constructor: Component is being constructed.');
}
componentDidMount() {
console.log('componentDidMount: Component has been mounted.');
}
componentDidUpdate(prevProps, prevState) {
console.log('componentDidUpdate: Component has been updated.');
}
componentWillUnmount() {
console.log('componentWillUnmount: Component is about to be unmounted.');
}
render() {
return (
<div>
<h2>Check the console to see lifecycle methods in action.</h2>
</div>
);
}
}
export default LifecycleDemo;
In this example, we use four lifecycle methods:
constructor
: Initializes the component.componentDidMount
: Called once the component has been mounted.componentDidUpdate
: Called when the component updates.componentWillUnmount
: Called just before the component is removed from the DOM.
To see these methods in action, include the LifecycleDemo
component in your App
component and open the browser console:
import React from 'react';
import './App.css';
import LifecycleDemo from './LifecycleDemo';
function App() {
return (
<div className="App">
<header className="App-header">
<h1>React Revolution</h1>
<LifecycleDemo />
</header>
</div>
);
}
export default App;
When you refresh your browser, you'll see the console messages from each lifecycle method.
4.3 Hooks: The Modern Way to Handle Lifecycle
With the introduction of hooks in React 16.8, managing lifecycle events in functional components has become much more straightforward. The useEffect
hook is the most commonly used hook for this purpose.
Here's how you can use useEffect
to mimic the behavior of the lifecycle methods:
import React, { useEffect } from 'react';
function LifecycleDemo() {
useEffect(() => {
console.log('componentDidMount: Component has been mounted.');
return () => {
console.log('componentWillUnmount: Component is about to be unmounted.');
};
}, []);
useEffect(() => {
console.log('componentDidUpdate: Component has been updated.');
});
return (
<div>
<h2>Check the console to see lifecycle methods in action.</h2>
</div>
);
}
export default LifecycleDemo;
In this example:
The first
useEffect
hook with an empty dependency array[]
runs once, mimickingcomponentDidMount
. The cleanup function inside it mimicscomponentWillUnmount
.The second
useEffect
hook runs after every render, mimickingcomponentDidUpdate
.
4.4 Why Lifecycle Methods and Hooks are Important
Lifecycle methods and hooks allow you to control the behavior of your components at crucial points in their existence. This is essential for tasks like:
Fetching data from an API when a component mounts.
Cleaning up subscriptions or timers to avoid memory leaks.
Optimizing performance by preventing unnecessary updates.
Congratulations! You've just learned the basics of React lifecycle methods and hooks. We covered:
The different stages of a component's lifecycle.
How to use lifecycle methods in class components.
How to use the
useEffect
hook in functional components.
In the next chapter, we'll explore how to handle events in React. Understanding event handling is key to making your applications interactive and user-friendly.
Feel free to experiment with the code and create your components with lifecycle methods and hooks. The more you practice, the more comfortable you'll become with these concepts.
Happy Coding!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
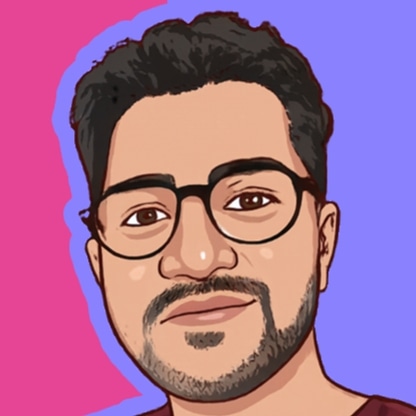
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code ๐ CS Grad at UTA | Full Stack Developer ๐ป | Problem Solver ๐ง