Chapter 7: Styling
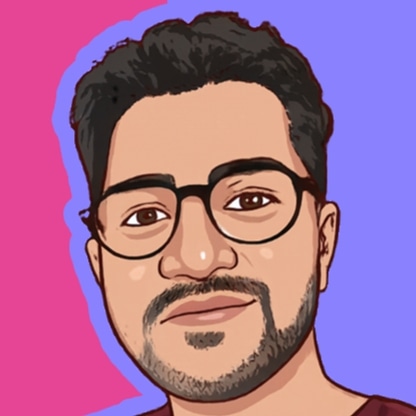
Welcome back to "React Revolution"! We've covered a lot so far, from the basics to conditional rendering. Now, it's time to make our applications look as good as they function. In this chapter, we'll explore different ways to style your React components, making your apps visually appealing and user-friendly.
7.1 Why Styling Matters
Styling is not just about making things look pretty; it's about enhancing user experience, accessibility, and overall usability. Good styling can make your application more intuitive and engaging.
7.2 Inline Styling
Inline styling is one of the simplest ways to style components in React. It allows you to add styles directly to elements using the style
attribute.
function InlineStyledComponent() {
return (
<div style={{ backgroundColor: 'lightblue', padding: '20px' }}>
<h2 style={{ color: 'darkblue' }}>Hello, Inline Styling!</h2>
</div>
);
}
export default InlineStyledComponent;
In this example:
We use the
style
attribute to apply styles directly to thediv
andh2
elements.Note that the styles are written in camelCase (e.g.,
backgroundColor
instead ofbackground-color
).
7.3 CSS Stylesheets
Using a separate CSS stylesheet is a common and organized way to style your React components. This method keeps your styles clean and maintainable.
First, create a CSS file (e.g., styles.css
):
/* styles.css */
.container {
background-color: lightblue;
padding: 20px;
}
.heading {
color: darkblue;
}
Then, import the CSS file in your component:
import './styles.css';
function StylesheetStyledComponent() {
return (
<div className="container">
<h2 className="heading">Hello, CSS Stylesheets!</h2>
</div>
);
}
export default StylesheetStyledComponent;
In this example:
We create a CSS file with styles for
.container
and.heading
.We import the CSS file in our component and use the
className
attribute to apply the styles.
7.4 CSS Modules
CSS Modules offer a way to scope CSS locally to the component, avoiding global namespace collisions. This is particularly useful in larger projects.
First, create a CSS module file (e.g., styles.module.css
):
/* styles.module.css */
.container {
background-color: lightblue;
padding: 20px;
}
.heading {
color: darkblue;
}
Then, import the module in your component:
import styles from './styles.module.css';
function CSSModuleStyledComponent() {
return (
<div className={styles.container}>
<h2 className={styles.heading}>Hello, CSS Modules!</h2>
</div>
);
}
export default CSSModuleStyledComponent;
In this example:
We create a CSS module file with styles.
We import the module and use the styles as properties of the
styles
object.
7.5 Styled Components
Styled-components is a popular library for styling React applications using tagged template literals. It allows you to write actual CSS code to style components.
First, install the library:
npm install styled-components
Then, use it in your component:
import styled from 'styled-components';
const Container = styled.div`
background-color: lightblue;
padding: 20px;
`;
const Heading = styled.h2`
color: darkblue;
`;
function StyledComponentsExample() {
return (
<Container>
<Heading>Hello, Styled Components!</Heading>
</Container>
);
}
export default StyledComponentsExample;
In this example:
We use
styled-components
to create styledContainer
andHeading
components.The styles are written as template literals, which makes them very readable and maintainable.
You've just learned various ways to style your React components! We covered:
Inline styling for quick and simple styles.
CSS stylesheets for organized and maintainable styles.
CSS Modules for scoped and conflict-free styles.
Styled components for writing actual CSS in JavaScript.
Styling is a crucial part of building user-friendly applications. Experiment with these methods to see which one fits your project best. In the next chapter, we'll dive into React Hooks, a powerful feature that allows you to use state and other React features without writing a class.
Happy Styling!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
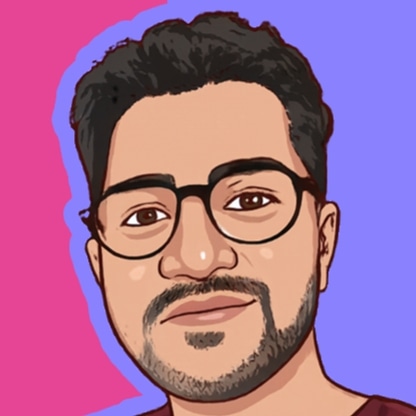
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code ๐ CS Grad at UTA | Full Stack Developer ๐ป | Problem Solver ๐ง