Chapter 11: Testing
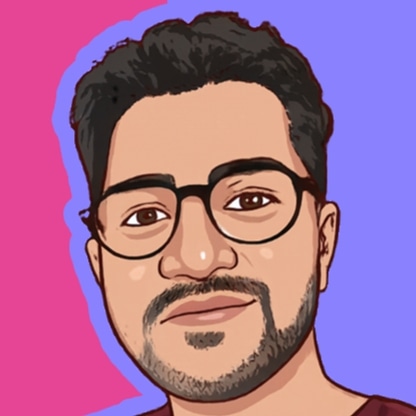
Welcome back to "React Revolution"! In this chapter, we’re going to talk about something every developer needs to know. Testing might sound intimidating, but it’s an essential part of developing reliable and maintainable applications. Think of it as a way to ensure your code works as expected before it reaches your users.
11.1 Why Testing Matters
Testing helps you catch bugs early, ensures your code does what it's supposed to do, and makes your codebase more maintainable. With a good set of tests, you can refactor or add new features with confidence, knowing that you’re not breaking existing functionality.
11.2 Types of Tests
There are several types of tests you can write, but for simplicity, we'll focus on three main types:
Unit Tests: Test individual components or functions in isolation.
Integration Tests: Test how different parts of your application work together.
End-to-End (E2E) Tests: Test your application as a whole, simulating user interactions.
11.3 Setting Up Testing in React
React has great support for testing through tools like Jest and React Testing Library. Let’s set up a basic testing environment.
Step 1: Install Jest and React Testing Library
First, install the necessary packages:
npm install --save-dev jest @testing-library/react @testing-library/jest-dom
Step 2: Configure Jest
Jest works out of the box with Create React App, so if you’re using it, you’re good to go. Otherwise, add the following script to your package.json
:
"scripts": {
"test": "jest"
}
11.4 Writing Your First Test
Let's write a simple unit test for a React component. Consider a Button
component:
// Button.js
import React from 'react';
const Button = ({ onClick, children }) => (
<button onClick={onClick}>
{children}
</button>
);
export default Button;
Now, let’s write a test for this component:
// Button.test.js
import React from 'react';
import { render, screen, fireEvent } from '@testing-library/react';
import Button from './Button';
test('renders button with text', () => {
render(<Button>Click Me</Button>);
const buttonElement = screen.getByText(/Click Me/i);
expect(buttonElement).toBeInTheDocument();
});
test('calls onClick when clicked', () => {
const handleClick = jest.fn();
render(<Button onClick={handleClick}>Click Me</Button>);
const buttonElement = screen.getByText(/Click Me/i);
fireEvent.click(buttonElement);
expect(handleClick).toHaveBeenCalledTimes(1);
});
In the first test, we check if the button renders with the correct text. In the second test, we ensure the onClick
function is called when the button is clicked.
11.5 Integration Testing
Integration tests check how different parts of your application work together. Let’s write an integration test for a simple Form
component:
// Form.js
import React, { useState } from 'react';
const Form = ({ onSubmit }) => {
const [value, setValue] = useState('');
const handleSubmit = (event) => {
event.preventDefault();
onSubmit(value);
};
return (
<form onSubmit={handleSubmit}>
<input
type="text"
value={value}
onChange={(e) => setValue(e.target.value)}
/>
<button type="submit">Submit</button>
</form>
);
};
export default Form;
Now, the integration test:
// Form.test.js
import React from 'react';
import { render, screen, fireEvent } from '@testing-library/react';
import Form from './Form';
test('submits form with input value', () => {
const handleSubmit = jest.fn();
render(<Form onSubmit={handleSubmit} />);
const inputElement = screen.getByRole('textbox');
const buttonElement = screen.getByRole('button', { name: /submit/i });
fireEvent.change(inputElement, { target: { value: 'test input' } });
fireEvent.click(buttonElement);
expect(handleSubmit).toHaveBeenCalledWith('test input');
});
Here, we check if the form submits the correct value when the submit button is clicked.
11.6 End-to-End (E2E) Testing
E2E tests simulate user interactions and test the entire application. Tools like Cypress or Selenium are commonly used for E2E testing. Setting up E2E tests is more complex, so we’ll keep it simple here. For a full setup, refer to the Cypress documentation.
Testing might seem daunting at first, but it’s a crucial part of software development. By starting with simple tests, you can gradually build confidence and ensure your application works as intended.
Happy Testing!
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
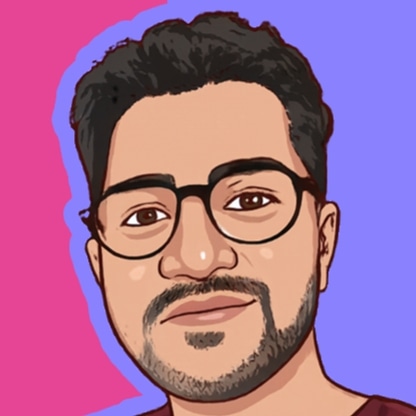
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠