How to fix : Bearer error="invalid_token", error_description="The issuer 'https://dev-[DOMAIN].auth0.com/' is invalid"
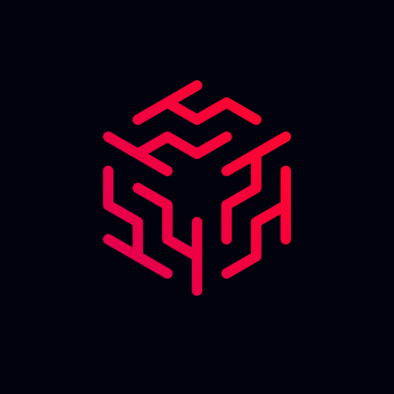
Before we start
This guide assumes:
You are using a recent version of .NET (.NET 6+) and that you are using JWT authentication with Auth0.
You have already followed an Auth0 guide to setup API authentication for ASP.NET but cannot fix the error mentioned in the title
You understand how Auth0 works and what domains, bearer tokens, JWTs, authentication, and authorization are. If not, I recommend reading something like this.
What does this error mean?
Bearer error="invalid_token", error_description="The issuer '
https://dev-[DOMAIN].[AREA].auth0.com/
' is invalid"
This error indicates that the JWT authentication module is unable to verify your token because the token's issuer and the ValidIssuer
you specified in your configuration do not match.
How to solve the issue
I ran into this problem while writing a Minimal Web Api. This solution should work for traditional controller-based projects as well but YMMV.
0. Verify your token is valid
Take the token you got from API Settings -> Test and paste it into jwt.io.
It will show you if it is valid. If it is, it will also show the domain and audience this token is associated with. Make sure those match with the values for your current API application.
Note that the issuer field shown on jwt.io will include a trailing slash at the end. This is important for later.
1. Make sure your values in appsettings.json
are correct.
It should look something like this:
"Auth0": {
"Domain": "dev-[yourdomain].[area].auth0.com",
"Audience": "https://[audience].com"
}
Audience
refers to theIdentifier
parameter in the APIs settings page.
Make sure that the Domain
does not start with 'https'.
Audience
should start with 'https' however.
I originally had this error because my domain was prefixed with 'https'.
2. Properly configure authentication in your Program.cs
As shown in this guide you need to setup JWT authentication for your API. I will not be covering how to set this up, refer to the guide for more info.
Once you have that set up your Program.cs
should look something like this:
var builder = WebApplication.CreateBuilder(args);
// other stuff...
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(JwtBearerDefaults.AuthenticationScheme, c =>
{
c.Authority = $"https://{builder.Configuration["Auth0:Domain"]}";
c.TokenValidationParameters = new Microsoft.IdentityModel.Tokens.TokenValidationParameters
{
ValidAudience = builder.Configuration["Auth0:Audience"],
ValidIssuer = $"https://{builder.Configuration["Auth0:Domain"]}",
};
});
builder.Services.AddAuthorization();
// adding other services...
var app = builder.Build();
app.UseAuthentication();
app.UseAuthorization();
// other stuff..
app.Run();
It was easier for me to extract the domain value as it was used twice and we need to modify it.
My code looks like this:
var domain = $"https://{builder.Configuration["Auth0:Domain"]}/";
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(JwtBearerDefaults.AuthenticationScheme, c =>
{
c.Authority = domain;
c.TokenValidationParameters = new Microsoft.IdentityModel.Tokens.TokenValidationParameters
{
ValidAudience = builder.Configuration["Auth0:Audience"],
ValidIssuer = domain,
};
});
This trailing slash is important as it matches the issuer field of the token as we saw earlier
Your [Authorized]
endpoints should now accept the valid token.
If this solution did not work for you, debug your Program.cs
and step through setup until you can read the values of ValidAudience
and ValidIssuer
. Make sure those two values match those found on jwt.io exactly!
Also, check out the code of this sample project it helped me finally solve this.
Acknowledgements
Helpful resources used:
https://auth0.com/blog/securing-blazor-webassembly-apps/#Securing-the-API-with-Auth0
https://github.com/auth0-blog/secure-blazor-wasm-quiz-manager
https://auth0.com/blog/aspnet-web-api-authorization/#Testing-the-Secure-Web-API
https://auth0.com/docs/quickstart/backend/aspnet-core-webapi/01-authorization
https://auth0.com/blog/securing-aspnet-minimal-webapis-with-auth0/
https://auth0.com/docs/quickstart/backend/aspnet-core-webapi/03-troubleshooting
Hope this helps!!
Tags:
- .NET
- ASP.NET Core
- Minimal Web Api
- Auth0
- JWT authentication
- api authentication
Subscribe to my newsletter
Read articles from Musa Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
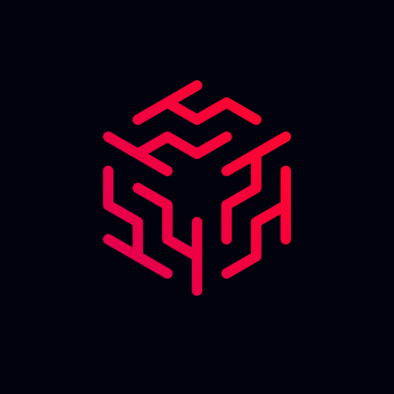