Keep it stupid simple (KISS) vs. Over-Engineering : Striking the Right Balance in Technical Implementations
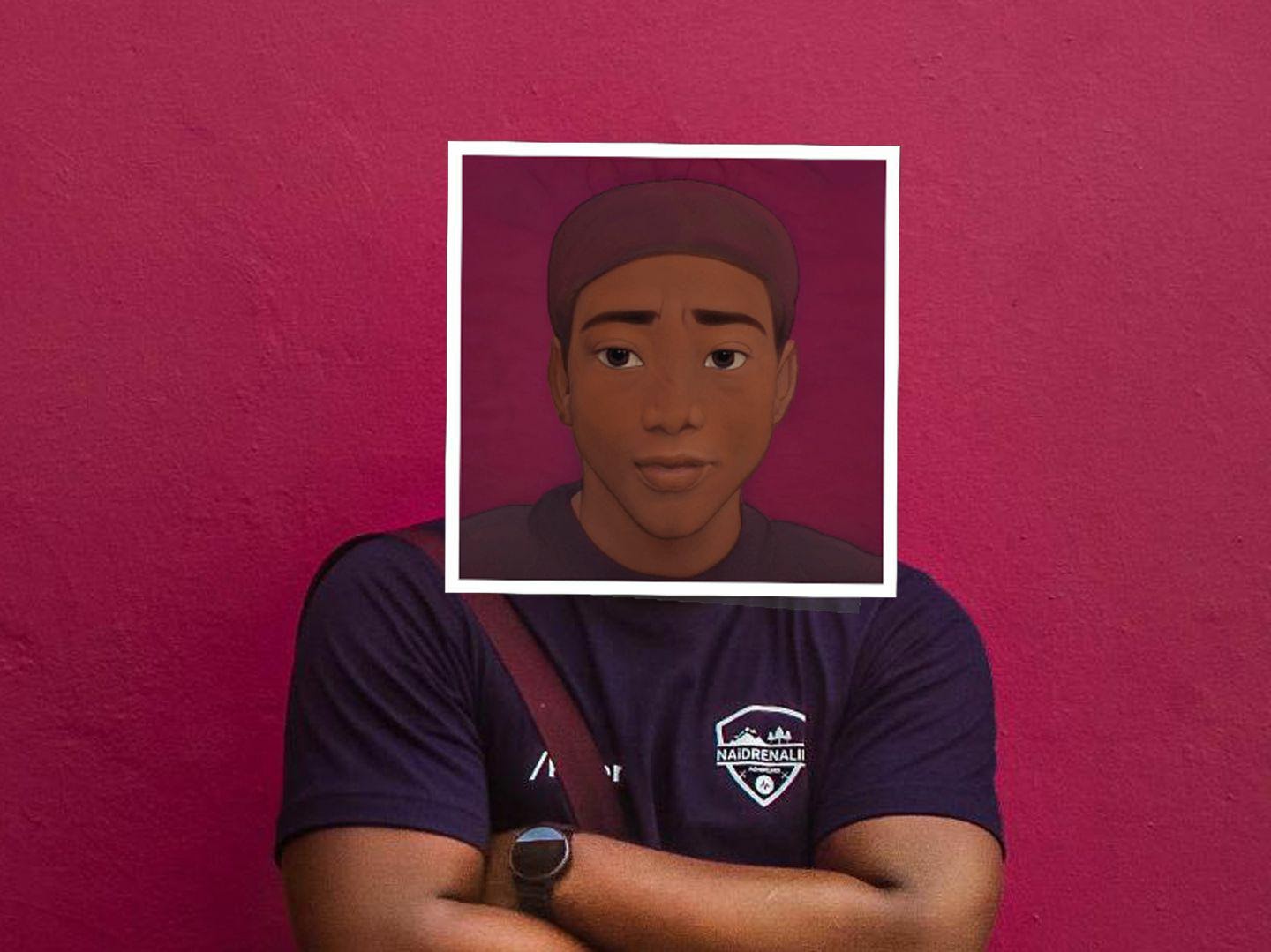
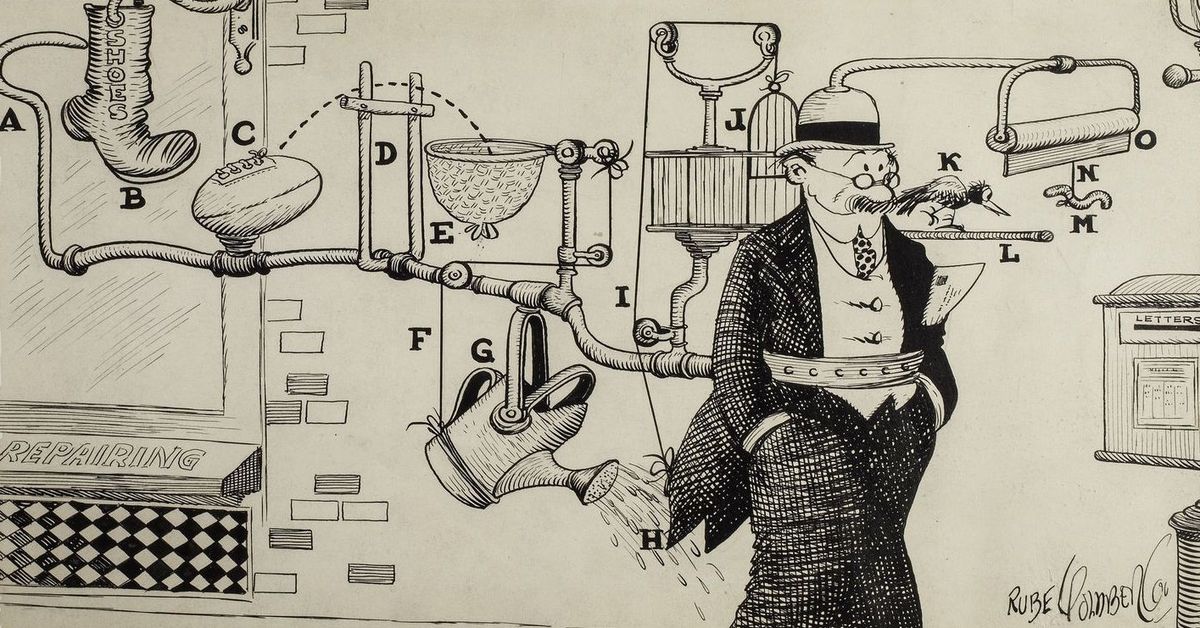
Introduction
As an engineer, you've likely received tasks from stakeholders that seem straightforward at first glance. Consider this common scenario:
Issue Type: Task
Summary: Implement Password Criteria for User Accounts
Description:
To improve security measures, implement password criteria for user accounts.
Requirements:
Password length must be a minimum of 8 characters.
Passwords must include a combination of capital letters, small letters, numerical characters, and special characters.
Acceptance Criteria:
User passwords are validated upon creation or modification to ensure they meet the minimum length requirement of 8 characters.
Passwords contain at least one capital letter, one small letter, one numeric character, and one special character.
Users attempting to create or update their passwords receive clear feedback if their chosen password does not meet the specified criteria.
The backend system enforces these criteria consistently across all user accounts.
Priority: High
Your client asks you to implement password criteria for user accounts to improve security. They outline basic requirements: a minimum length of 8 characters and a mix of uppercase, lowercase, and special characters. The acceptance criteria further specify the need for at least one of each character type and clear user feedback.
Now, you're faced with a classic engineering dilemma. Do you stick to these basic requirements, embracing the KISS principle (Keep It Simple, Stupid)? Or do you dive into complex engineering, considering every possible edge case and future expansion? This is where the art of balanced technical implementation comes into play. Let's explore how to navigate between simplicity and complexity, ensuring a solution that's both effective and maintainable.
Why KISS?
KISS, an acronym for "Keep It Simple, Stupid," is a guiding principle in software design. This concept emphasizes that the most effective designs, solutions, systems, and products are those that maintain simplicity at their core. The KISS principle advocates for straightforward approaches over intricate ones. It suggests that developers should strive to reduce complexity wherever possible, focusing on creating streamlined, easily understandable solutions. The underlying belief is that simplicity leads to more robust, maintainable, and user-friendly outcomes in software development.
Benefits of KISS in Code
Easier Long-term Maintenance: KISS principles lead to cleaner, simpler code that's easier to modify and update in the future. Less complexity means less time deciphering the logic and more time making improvements.
Enhanced Code Clarity: KISS promotes code that's easier to understand for both you and your fellow developers. Clear and concise code reduces the need for extensive comments and explanations, fostering better collaboration and knowledge sharing within the team.
More Effective Testing: Simpler code translates to easier testing. Unit tests, integration tests, and other automated testing methods become more efficient and reliable when dealing with code that's well-structured and avoids unnecessary complexity.
The Pitfalls of Over-Engineering
In simple words, over-engineering is writing code or designing a system that addresses potential problems you might encounter in the future, rather than focusing on the current requirements.
Over-engineering can be tempting, but it often leads to unintended consequences. Here are some key drawbacks to consider:
Extended Development Cycles: Overly complex solutions can take significantly longer to develop, delaying your project's launch and time to market.
Higher Costs: Complex systems require more resources to build and maintain, leading to increased development and maintenance costs.
Technical Debt: Overly complex code becomes "technical debt" that accumulates over time, making future modifications and updates more difficult and expensive.
Missed Market Fit: Excessive focus on engineering perfection may delay product launches and iterations, potentially hindering the ability to reach product-market fit in a timely manner.
Excessive Abstraction and Indirection: While abstraction can be valuable, over-engineering can lead to overly complex code that is difficult to understand and maintain. Strive for a balance between code that is clear, concise, and modular without sacrificing readability.
Finding the Right Balance
Achieving a balance between KISS and over-engineering requires careful planning and execution. Here are some key strategies:
Focusing on Core Needs:
Clear Project Definition: Define the project's purpose and goals to identify essential features (MVP). Prioritize these core functionalities and avoid feature creep.
Trading-off Complexity: Evaluate the time, resource, and complexity costs of additional features. Weigh these costs against potential benefits. Simple solutions can be surprisingly effective.
Prioritizing Practicality:
- Compromise for Success: Strive for a balance between a perfect solution and a functional one. Delivering "good enough" on time and within budget can be better than a missed deadline with a complex solution.
Simplicity and Maintainability:
- KISS (Keep It Simple, Stupid): Design solutions that are easy to understand and maintain. Simpler designs often demonstrate greater long-term robustness and adaptability.
Collaboration and Standards:
Peer Review: Seek feedback from other programmers to identify potential flaws or opportunities for simplification. Foster a culture of collaboration and knowledge sharing.
Adhere to industry and organizational standards. This promotes consistency and interoperability
Alignment with Business Goals:
- Business-Driven Development: Focus on features and improvements that directly contribute to the business objectives. Prioritize features and optimizations that directly contribute to business goals. Maintain open communication between engineering and business teams to ensure alignment.
Case Studies
Following the stakeholder's request to improve security through password criteria (introduced earlier), I've implemented and evaluated both a simple and a more complex approach to password validation.
Simple Approach
The simple approach uses a single ValidatePasswordRequirement
function. This function performs several checks:
Empty password check
Minimum length check
Checks for at least one of each character type (uppercase, lowercase, digit, and special character) using regular expressions
Pros:
Simpler implementation
Easier to understand for beginners
Cons:
Less flexible – adding new requirements would require modifying the function directly
Repetitive code for each character type check
Complex Approach
The second approach utilizes the Strategy pattern with several interfaces and classes:
IPasswordRequirementStrategy
: An interface defining a Validate method for each password requirement.Concrete strategy classes implementing
IPasswordRequirementStrategy
:MinimumLengthRequirementStrategy
: Checks for minimum password length.UppercaseRequirementStrategy
: Checks for at least one uppercase character. (Similar strategies for lowercase, digit, and non-alphanumeric characters)
PasswordRequirement
: A record holding configuration for password requirements (minimum length, uppercase requirement, etc.)PasswordValidationContext
: Class that manages a list of strategies and validates passwords.
Pros:
- More flexible – adding new requirements involves creating a new strategy class. Improved code organization and separation of concerns Easier to maintain and test
Cons:
- More complex implementation Requires understanding of interfaces and the Strategy pattern
Conclusion
In software development, striking a balance between simple and complex solutions is essential. Over-engineering can lead to slower development, long-term maintenance issues, and solutions that miss the mark on business needs.
To avoid these pitfalls, prioritize features aligned with business goals, embrace iterative development with an MVP, and continuously simplify. Foster open communication and emphasize delivering tangible value to users. By following these principles, development teams can create effective, maintainable solutions that meet user needs and business objectives. Remember, the focus is on solving problems, not showcasing technical skills at the expense of usability.
Reference
Subscribe to my newsletter
Read articles from Peter Makafan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
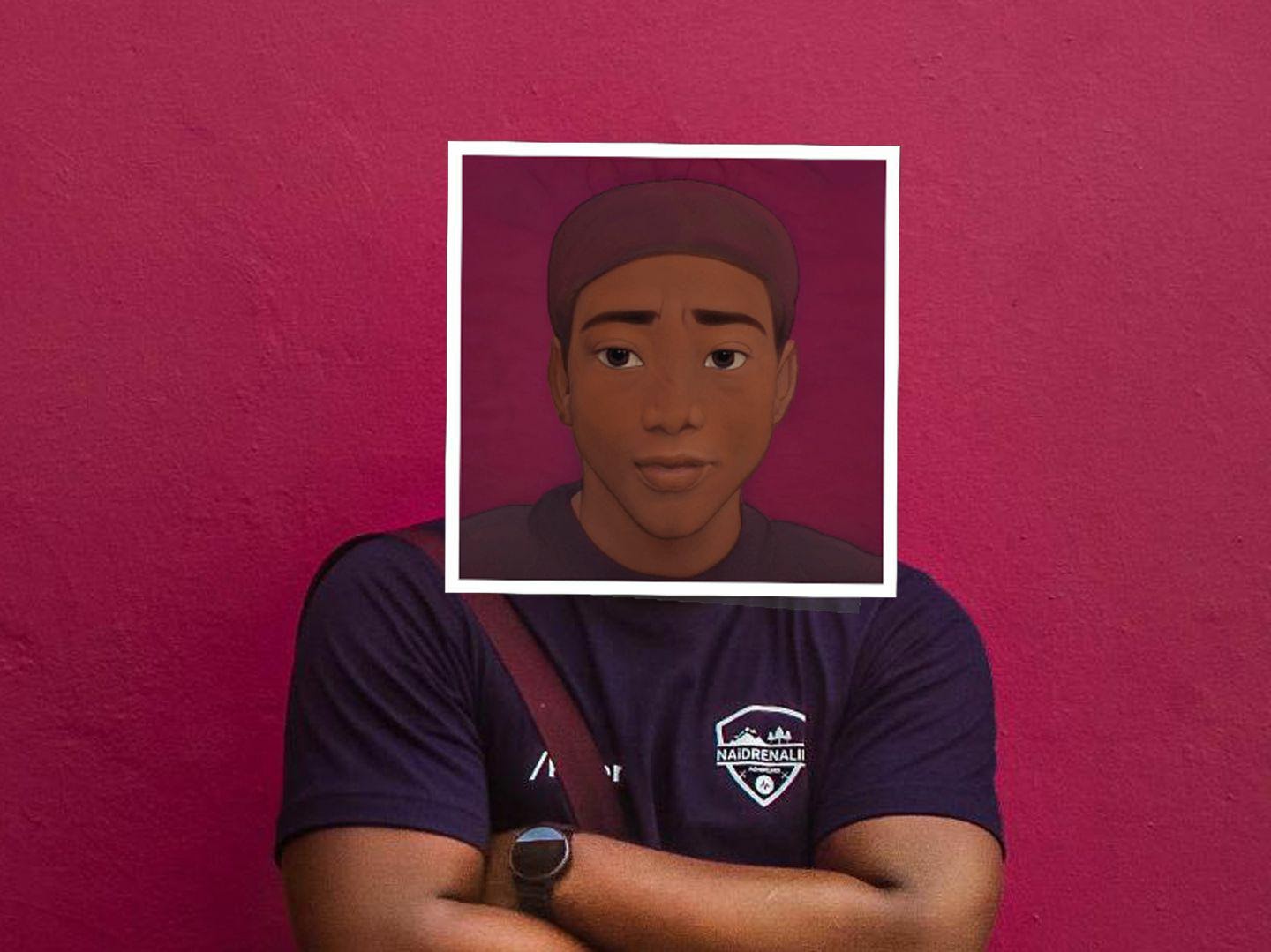
Peter Makafan
Peter Makafan
Hi, I'm Peter, a Senior Software Engineer and independent Technology consultant, specializes in building scalable, resilient, and distributed software systems. With over a decade of experience in fintech, ecommerce, and digital marketing, he brings a unique perspective to technology solutions. His academic background in Artificial Intelligence and Computer Science fuels his passion for cutting-edge technologies like financial technology, robotics, and cloud-native development.