Mastering the Two Pointers Technique
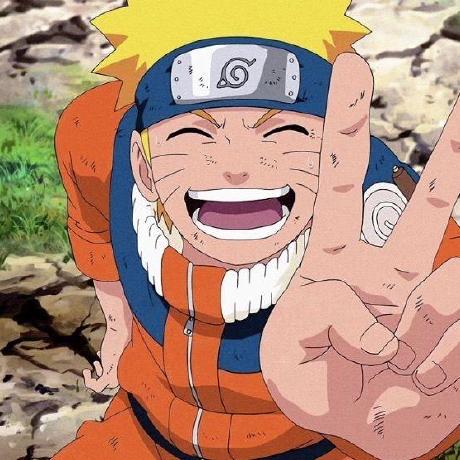
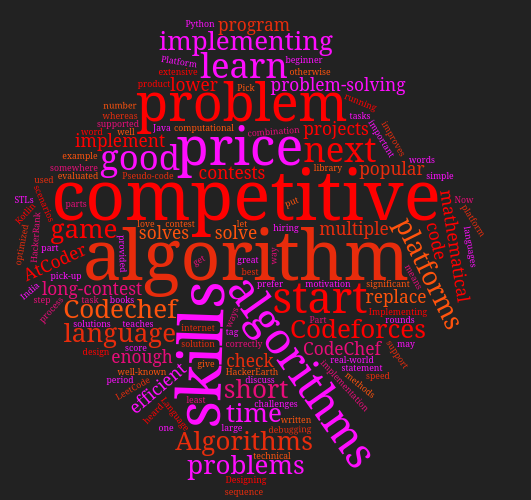
Introduction
The Two Pointers technique is a versatile and efficient method commonly used to solve various problems involving arrays, strings, and linked lists. This technique is particularly effective when dealing with pairs or sub-arrays that need to satisfy specific conditions or when searching for elements in sorted data structures. By strategically moving two pointers across the data structure, you can often reduce the time complexity of your solution.
When to Use Two Pointers
The Two Pointer technique is useful in scenerios such as:
Finding pairs or sub-arrays that meet certain citeria.
Searching for specific elements in a sorted array.
Optimizing problem where single pointer approach would be less efficient.
How It works
Typically, in the Two Pointers technique, you will use two pointers that start at opposite ends of the data structure and move towards each other with a fixed increment. This approach can be applied to various data structures like Array, String, and Linked Lists.
Sample Problems and Solutions
Find a Pair Of Numbers that Add Up to a Target Sum.
Input: [2,7,11,5] , Target Sum: 9
Output: [2,7]
Solution: Using two pointer starting from the beginning and the end of the sorted array. Adjust the pointers based on the sum compared to the target.
Find the Maximum Sum Sub-Array
Input: [-2,1,-3,4,-1,2,1,-5,4]
Output: [4,-1,2,1]
Solution: Utilize a variation of the Two Pointers technique, or more efficiently , Kadane's Algorithm for finding the maximum sum sub-array.
Find the Longest Palindrome Substring
Input: "babad"
Output: "bab"
Solution: Use two pointers to expand around each character (and pair of characters) to find the longest palindrome.
Conclusion
The Two Pointers technique is a powerful in your algorithm toolkit. By understand and apply this technique, you can solve a variety of problem more efficiently. Practice with different data structures and problem types to master this approach and improve your problem-solving skills.
Bad programmers worry about the code. Good progammers worry about data structures and their relationships.
Linus Torvalds
Subscribe to my newsletter
Read articles from ddhuu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
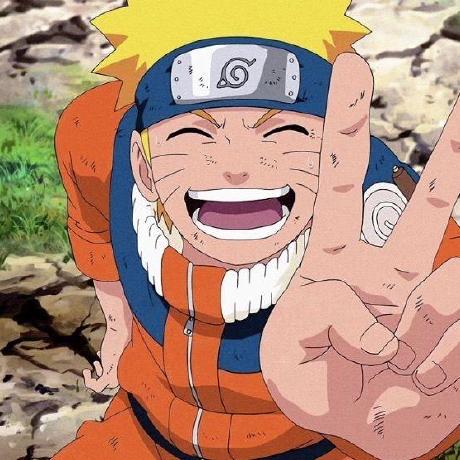