Unlock the Full Potential of Flask: A Comprehensive Guide for Beginners
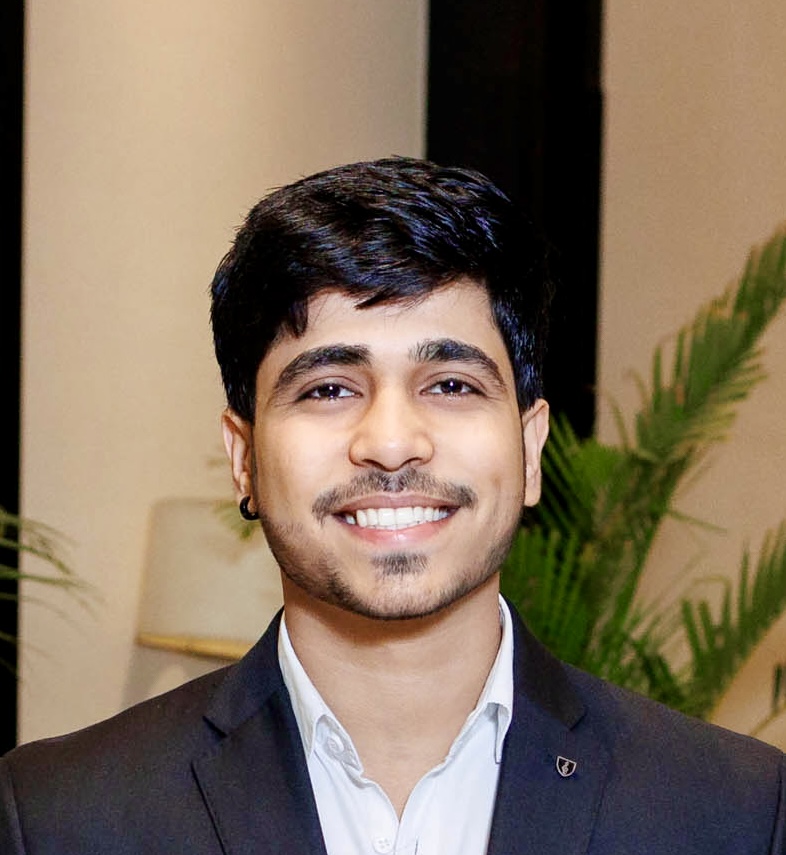
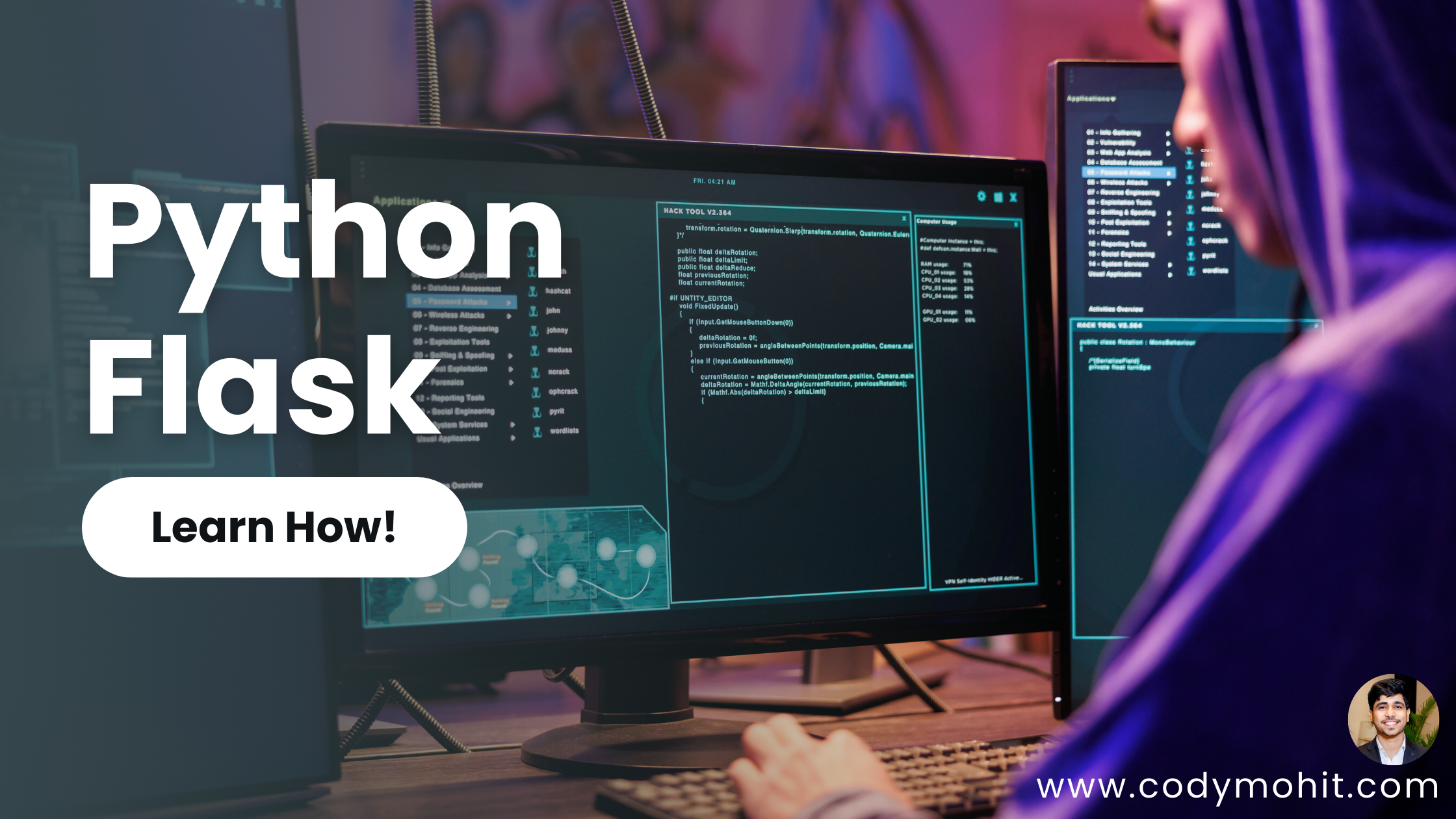
Have you ever wondered why Flask is the go-to framework for so many developers when it comes to web development? Well, you're in the right place! In this comprehensive guide, we're gonna dive deep into the world of Flask and uncover all its hidden gems. Whether you're a complete beginner or someone looking to brush up on their skills, this guide has got you covered. We'll walk you through the basics, show you how to set up your first Flask project, and even share some pro tips along the way. So, grab your favorite beverage, get comfy, and let's unlock the full potential of Flask together! You won't wanna miss a single bit of this. 🚀
Welcome to Flask
Brief Introduction to Flask
Flask is a lightweight web framework for Python. It's designed to be simple and easy to use, making it perfect for beginners and experienced developers alike. Unlike some other frameworks, Flask doesn’t come with a lot of built-in features. Instead, it gives you the essentials and lets you add what you need.
Importance of Flask in Web Development
Flask is important because it allows developers to create web applications quickly and easily. Its flexibility means you can start with a small project and scale it up as needed. Flask is also known for its simplicity and ease of use, which makes it a great choice for building prototypes or small to medium-sized web applications.
What This Guide Covers
In this guide, we will explore the basics of Flask, including how to set up a Flask project, create routes, and handle requests. We’ll also look at some of the most useful features of Flask and how you can use them in your projects.
Why Choose Flask?
Advantages of Using Flask
Simplicity: Flask is easy to learn and use. It doesn’t have a steep learning curve, making it ideal for beginners.
Flexibility: Since Flask is a micro-framework, you can build your app exactly the way you want. You're not forced to follow any specific patterns or conventions.
Extensibility: Flask can be easily extended with various extensions that add functionality to your application. This allows you to keep your codebase clean and modular.
Community Support: Flask has a large and active community. If you run into any issues, there are plenty of resources available to help you out.
Comparison with Other Web Frameworks
Django: Django is a high-level framework that comes with a lot of built-in features like authentication, ORM, and an admin interface. While it's great for large projects, it can be overkill for smaller applications. Flask, on the other hand, gives you more control and lets you add only what you need.
FastAPI: FastAPI is a newer framework that's designed for building APIs quickly and efficiently. It’s known for its speed and performance. While FastAPI is great for API development, Flask is more versatile and can be used for both web apps and APIs.
In conclusion, Flask is a powerful and flexible framework that’s perfect for developers who want to create web applications quickly and easily. Whether you’re just starting out or looking for a lightweight framework for your next project, Flask is an excellent choice.
Getting Started with Flask
Setting Up Your Development Environment
Installing Python
To get started with Flask, you first need to have Python installed on your machine. Flask works with Python 3, so make sure you're using the latest version of Python. You can download Python from the official website python.org. Follow the installation instructions for your operating system. Don't forget to check the option to add Python to your PATH during installation, this makes it easier to run Python from the command line.
Setting up a virtual environment
Before you install Flask, it's a good idea to set up a virtual environment. This keeps your project dependencies isolated and manageable. Open your command line interface (CLI) and navigate to the directory where you want to create your project. Run the following commands:
# On Windows
python -m venv venv
# On macOS/Linux
python3 -m venv venv
This creates a new directory called venv
containing your virtual environment. To activate the virtual environment, use these commands:
# On Windows
venv\Scripts\activate
# On macOS/Linux
source venv/bin/activate
You'll know the virtual environment is active when you see (venv)
at the beginning of your command prompt.
Installing Flask
With the virtual environment activated, you can now install Flask. Use the following command to install Flask via pip:
pip install flask
This command downloads and installs Flask and its dependencies in your virtual environment. You're now ready to create your first Flask app!
Your First Flask Application
Creating a basic Flask app
Let's create a simple Flask application. Open your favorite code editor and create a new file named app.py
in your project directory. Add the following code to app.py
:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run(debug=True)
This code creates a Flask application and defines a single route that returns "Hello, World!" when you visit the root URL.
Running the Flask development server
To run your Flask app, go back to your command line, make sure your virtual environment is still activated, and run the following command:
python app.py
You should see output indicating that the Flask development server is running. Open a web browser and go to http://127.0.0.1:5000/
to see your "Hello, World!" message.
Understanding the folder structure
As your Flask project grows, you'll need to organize your files properly. A common folder structure for a Flask project looks like this:
my_flask_app/
app.py
/static
/templates
/venv
config.py
requirements.txt
app.py
: Your main application file./static
: Folder for static files like CSS, JavaScript, and images./templates
: Folder for HTML templates.venv
: Your virtual environment directory.config.py
: Configuration file for your app.requirements.txt
: File listing your project dependencies.
By following this structure, you can keep your project organized and manageable.
Congratulations, you've created your first Flask app! As you continue to build your Flask projects, you'll learn more about the powerful features Flask has to offer. Happy coding!
Core Concepts of Flask
Flask is a micro web framework written in Python. It is known for being lightweight, flexible, and easy to use. Here are some of the core concepts:
Minimalism: Flask is minimalistic, meaning it comes with only the essentials. You can add more functionality as needed through extensions.
Routing: Routing is the process of matching a URL to a function that should handle it. Flask makes this easy with its @app.route decorator.
Templating: Flask uses Jinja2 as its templating engine, which allows you to generate HTML dynamically using Python code.
Blueprints: These are a way to organize your application into smaller, reusable modules.
Flask Routing
Flask routing is how you direct web requests to specific parts of your application. Let's dive into the key aspects of Flask routing.
Defining Routes
To define a route in Flask, you use the @app.route
decorator. This tells Flask which URL should trigger a particular function. Here’s an example:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, World!"
In this example, visiting the home page ("/") will trigger the home
function, which returns a simple "Hello, World!" message.
Handling Different HTTP Methods
HTTP methods like GET, POST, PUT, and DELETE are used to interact with resources on a server. In Flask, you can handle these methods using the methods
argument in the route decorator.
@app.route('/submit', methods=['GET', 'POST'])
def submit():
if request.method == 'POST':
return "Form submitted!"
return "Submit your form."
Here, the /submit
route can handle both GET and POST requests. If the method is POST, it returns "Form submitted!". Otherwise, it returns "Submit your form."
Route Parameters
Route parameters allow you to pass variables in the URL, making your routes more dynamic.
@app.route('/user/<username>')
def show_user(username):
return f"User: {username}"
In this example, <username>
is a route parameter. If you visit /user/john
, it will return "User: john".
Templates in Flask
Templates are a key part of web development with Flask, allowing you to separate the HTML structure of your web pages from your Python code. This makes your code cleaner, more maintainable, and more flexible. Flask uses Jinja2, a powerful templating engine, to help you create dynamic web pages.
Introduction to Jinja2 Templates
Jinja2 is a modern and designer-friendly templating engine for Python. It's easy to use and very powerful, allowing you to embed Python-like expressions directly into your HTML. This lets you create dynamic content that changes based on the data passed to the template. With Jinja2, you can use variables, control structures (like loops and conditionals), and even call functions within your templates.
Creating and Rendering Templates
Creating a template in Flask is straightforward. First, you need to create an HTML file and save it in a folder named templates
in your project directory. Here's a simple example:
<!-- templates/hello.html -->
<!DOCTYPE html>
<html>
<head>
<title>Hello</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
In this example, {{ name }}
is a placeholder for a variable that will be passed to the template. To render this template from your Flask application, you use the render_template
function.
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/hello/<name>')
def hello(name):
return render_template('hello.html', name=name)
if __name__ == '__main__':
app.run(debug=True)
When you visit /hello/YourName
, Flask will render the hello.html
template and replace {{ name }}
with the value of the name
variable, resulting in a personalized greeting.
Template Inheritance and Macros
Template inheritance is a powerful feature in Jinja2 that allows you to create a base template and extend it with child templates. This helps you avoid repetition and keep your templates organized. Here's how it works:
Base Template (base.html)
<!-- templates/base.html -->
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
</head>
<body>
<header>
<h1>My Website</h1>
</header>
<main>
{% block content %}{% endblock %}
</main>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
Child Template (index.html)
<!-- templates/index.html -->
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block content %}
<h2>Welcome to My Website</h2>
<p>This is the homepage.</p>
{% endblock %}
In this example, index.html
extends base.html
. It fills in the title
and content
blocks defined in the base template, creating a complete HTML page.
Macros
Macros in Jinja2 are like functions in Python. They allow you to define reusable snippets of template code. Here's an example:
<!-- templates/macros.html -->
{% macro render_user(user) %}
<div class="user">
<h2>{{ user.name }}</h2>
<p>Email: {{ user.email }}</p>
</div>
{% endmacro %}
You can then import and use this macro in other templates:
<!-- templates/users.html -->
{% from "macros.html" import render_user %}
<!DOCTYPE html>
<html>
<head>
<title>Users</title>
</head>
<body>
{% for user in users %}
{{ render_user(user) }}
{% endfor %}
</body>
</html>
In this example, render_user
is a macro that generates HTML for a user. The users.html
template imports and uses this macro to display a list of users.
Wrapping Up
Using Jinja2 templates in Flask lets you create dynamic and maintainable web pages with ease. By leveraging features like template inheritance and macros, you can keep your templates clean and DRY (Don't Repeat Yourself). Remember, separating your HTML from your Python code makes your web application more organized and easier to manage.
By understanding and utilizing these concepts, you'll be able to build robust and dynamic web applications with Flask. Happy coding!
Working with Forms in Flask: An Easy Guide
Forms are essential for web applications, allowing users to input data that your app can process. In Flask, handling forms is made easy with the Flask-WTF extension. Let's break it down step by step:
Handling Form Data with Flask-WTF
Flask-WTF integrates Flask with WTForms, making form handling smooth and simple. Here's how you can do it:
Install Flask-WTF: First, you'll need to install the extension using pip.
pip install Flask-WTF
Create a Form Class: Define a form class that inherits from
FlaskForm
. This class will include the form fields you want.from flask_wtf import FlaskForm from wtforms import StringField, PasswordField, SubmitField from wtforms.validators import DataRequired class LoginForm(FlaskForm): username = StringField('Username', validators=[DataRequired()]) password = PasswordField('Password', validators=[DataRequired()]) submit = SubmitField('Log In')
Use the Form in a Route: In your Flask routes, create an instance of the form and pass it to the template.
from flask import Flask, render_template, redirect, url_for from forms import LoginForm app = Flask(__name__) app.config['SECRET_KEY'] = 'yoursecretkey' @app.route('/login', methods=['GET', 'POST']) def login(): form = LoginForm() if form.validate_on_submit(): return redirect(url_for('success')) return render_template('login.html', form=form) @app.route('/success') def success(): return "Logged in successfully!"
Create the Template: In your HTML template, use the form instance to render the form.
<!doctype html> <html> <head> <title>Login</title> </head> <body> <form method="POST"> {{ form.hidden_tag() }} <p> {{ form.username.label }}<br> {{ form.username(size=32) }}<br> {% for error in form.username.errors %} <span style="color: red;">[{{ error }}]</span> {% endfor %} </p> <p> {{ form.password.label }}<br> {{ form.password(size=32) }}<br> {% for error in form.password.errors %} <span style="color: red;">[{{ error }}]</span> {% endfor %} </p> <p>{{ form.submit() }}</p> </form> </body> </html>
Validating Form Inputs
Validation ensures that the data submitted by the user is correct and secure. With Flask-WTF, you can add validators to your form fields easily.
DataRequired: Ensures that the field is not empty.
Email: Validates that the input is a valid email address.
Length: Ensures the input meets a minimum and maximum length requirement.
Here's how to add more validators to a form:
from wtforms.validators import Email, Length
class RegistrationForm(FlaskForm):
username = StringField('Username', validators=[DataRequired(), Length(min=4, max=25)])
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired(), Length(min=6)])
submit = SubmitField('Register')
Preventing CSRF Attacks
Cross-Site Request Forgery (CSRF) is a type of attack where malicious websites can trick users into performing actions on your site without their consent. Flask-WTF helps prevent this by generating a CSRF token for each form.
CSRF Protection: Flask-WTF automatically includes CSRF protection in your forms. When you use
form.hidden_tag()
, it adds a hidden field with a CSRF token.Configuration: Make sure you set a secret key in your Flask app to enable CSRF protection.
Here's an example of configuring CSRF protection:
app = Flask(__name__)
app.config['SECRET_KEY'] = 'yoursecretkey'
And in your form template:
<form method="POST">
{{ form.hidden_tag() }}
<!-- rest of your form fields -->
</form>
By following these steps, you can handle form data, validate inputs, and prevent CSRF attacks effectively in your Flask application.
Remember, keeping your forms secure and well-validated is crucial for a safe and user-friendly web app. Happy coding!
Database Integration with Flask
Setting up SQLite with Flask
Install Flask and SQLite: First, make sure you have Flask installed. SQLite comes pre-installed with Python.
pip install Flask
Create a Flask Application: Start by creating a simple Flask app.
from flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run(debug=True)
Configure SQLite: Set up the SQLite database by configuring the Flask app.
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
Using SQLAlchemy for ORM
Install SQLAlchemy: You'll need SQLAlchemy to handle ORM (Object-Relational Mapping).
pip install Flask-SQLAlchemy
Initialize SQLAlchemy: Initialize SQLAlchemy with your Flask app.
from flask_sqlalchemy import SQLAlchemy app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False db = SQLAlchemy(app)
Define a Model: Create a model to represent your database table.
class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(80), unique=True, nullable=False) email = db.Column(db.String(120), unique=True, nullable=False) def __repr__(self): return f'<User {self.username}>'
Performing CRUD Operations
Create the Database: Before performing operations, create the database.
with app.app_context(): db.create_all()
Create (Insert) a Record: Add a new record to the database.
@app.route('/add') def add_user(): new_user = User(username='john_doe', email='john@example.com') db.session.add(new_user) db.session.commit() return "User added!"
Read (Retrieve) Records: Fetch records from the database.
@app.route('/users') def get_users(): users = User.query.all() return ', '.join([user.username for user in users])
Update a Record: Update an existing record in the database.
@app.route('/update/<int:id>') def update_user(id): user = User.query.get(id) if user: user.email = 'newemail@example.com' db.session.commit() return "User updated!" return "User not found."
Delete a Record: Remove a record from the database.
@app.route('/delete/<int:id>') def delete_user(id): user = User.query.get(id) if user: db.session.delete(user) db.session.commit() return "User deleted!" return "User not found."
Summary
Setting up a database with Flask is super easy! Here's a quick recap:
Install Flask and SQLite: Use
pip
to install Flask, SQLite is already included with Python.Configure SQLite: Set the
SQLALCHEMY_DATABASE_URI
in your Flask app config.Use SQLAlchemy for ORM: Install and initialize SQLAlchemy, then define your models.
Perform CRUD Operations: Create, Read, Update, and Delete records using SQLAlchemy.
By following these steps, you'll have a fully functional database integrated with your Flask application. Remember, practice makes perfect! Happy coding!Setting up SQLite with Flask
Handling Static Files in Your Web Application
When building a web application, you'll often need to serve static files like CSS, JavaScript, and images. These files are essential for styling your site, adding interactivity, and displaying visuals. Let's break down how to handle these static files in a simple and memorable way.
Serving CSS, JavaScript, and Images
1. CSS (Cascading Style Sheets): CSS files are used to style your web pages. Think of them as the makeup and clothes of your website. They define how elements like text, buttons, and layout look. To include a CSS file in your HTML, you link to it using a <link>
tag in the <head>
section.
Example:
<link rel="stylesheet" href="/static/css/styles.css">
2. JavaScript: JavaScript files add interactivity to your site. They can be used to create animations, handle form submissions, and more. To include a JavaScript file, you use a <script>
tag, usually placed before the closing </body>
tag.
Example:
<script src="/static/js/scripts.js"></script>
3. Images: Images make your site visually appealing. To include images, you use the <img>
tag. The src
attribute specifies the path to your image file.
Example:
<img src="/static/images/logo.png" alt="Site Logo">
Configuring Static File Paths
To make sure your web server knows where to find these static files, you need to configure static file paths. Here’s how you do it:
1. Directory Structure: Organize your static files in a structured way. Commonly, you’ll have a static
or public
directory in your project. Inside this directory, you might have folders for CSS, JavaScript, and images.
Example:
/static
/css
styles.css
/js
scripts.js
/images
logo.png
2. Server Configuration: Depending on your web framework or server, you might need to configure it to serve static files correctly. For instance, in Flask (a popular Python framework), you use app.static_folder
to set the directory for static files.
Example:
app = Flask(__name__, static_folder='static')
In Django, you set the STATIC_URL
in your settings file to point to the URL for static files.
Example:
STATIC_URL = '/static/'
3. File Paths in HTML: When referencing static files in your HTML, use relative paths based on the static directory configuration.
For example, if your static directory is set up correctly and you want to reference styles.css
, you might use /static/css/styles.css
as shown above.
Remember to keep the paths relative to the root of your static directory, and make sure your web server is configured to serve files from this directory.
By organizing your static files and configuring your server properly, you’ll ensure that your web pages are styled, interactive, and visually appealing. This setup not only helps in keeping your project organized but also improves the performance and user experience of your web application.
Advanced Flask Features
Blueprints for Modular Applications
Introduction to Blueprints
Blueprints are a cool feature in Flask that help you split your app into smaller, more manageable pieces. Think of them as a way to organize your code so it’s easier to maintain and scale. Instead of having one giant app file, you can create multiple blueprints, each handling a specific part of your app, like user authentication or blog posts.
Organizing Your Application with Blueprints
Imagine you’re building a blog. You might have one blueprint for handling posts and another for user profiles. Here’s how you can set it up:
Create Blueprints: In your project, you can create a new file for each blueprint. For instance, you might have
posts.py
andusers.py
.Define Blueprints: In each file, define a blueprint using
Blueprint
class:from flask import Blueprint posts_bp = Blueprint('posts', __name__) @posts_bp.route('/posts') def show_posts(): return "Here are the blog posts!"
from flask import Blueprint users_bp = Blueprint('users', __name__) @users_bp.route('/users') def show_users(): return "Here are the users!"
Register Blueprints: In your main app file, register these blueprints:
from flask import Flask from posts import posts_bp from users import users_bp app = Flask(__name__) app.register_blueprint(posts_bp) app.register_blueprint(users_bp)
Using Blueprints for Better Code Management
By using blueprints, you keep related routes and logic in separate files. This makes your code easier to understand and manage. When your app grows, you can add more blueprints without making your main file messy.
Flask Extensions
Overview of Popular Flask Extensions
Flask extensions add extra features to your Flask app. They are like plugins that provide functionalities such as email support, user authentication, and database migrations.
Flask-Mail, Flask-Login, Flask-Migrate
Flask-Mail: This extension makes it easy to send emails. Here’s a basic setup:
from flask_mail import Mail, Message app = Flask(__name__) app.config['MAIL_SERVER'] = 'smtp.example.com' app.config['MAIL_PORT'] = 587 app.config['MAIL_USERNAME'] = 'your-email@example.com' app.config['MAIL_PASSWORD'] = 'your-password' app.config['MAIL_USE_TLS'] = True mail = Mail(app) @app.route('/send') def send_mail(): msg = Message('Hello', sender='your-email@example.com', recipients=['recipient@example.com']) msg.body = 'This is the email body' mail.send(msg) return "Mail sent!"
Flask-Login: It handles user session management. You can set it up like this:
from flask_login import LoginManager, UserMixin, login_user, login_required, logout_user login_manager = LoginManager() login_manager.init_app(app) class User(UserMixin): pass @login_manager.user_loader def load_user(user_id): user = User() user.id = user_id return user @app.route('/login') def login(): user = User() user.id = 'user_id' login_user(user) return "Logged in!" @app.route('/profile') @login_required def profile(): return "This is the profile page."
Flask-Migrate: It helps you handle database migrations. To use it, you first initialize it:
from flask_migrate import Migrate migrate = Migrate(app, db)
Then you can create and apply migrations using Flask commands:
flask db init flask db migrate -m "Initial migration." flask db upgrade
How to Integrate and Use Extensions
Integrating extensions is pretty simple. Just install them via pip, configure them in your app, and use their APIs. Here’s a general approach:
Install: Use pip to install the extension, like
pip install Flask-Mail
.Configure: Add the necessary configuration settings to your app.
Use: Call the extension’s methods where needed in your code.
By using blueprints and extensions, you can make your Flask app more modular and feature-rich, making it easier to develop and maintain.
Advanced Flask Features: Error Handling and Logging
When building Flask applications, handling errors and logging are crucial for maintaining a robust and debuggable system. Let's dive into these features with an easy-to-understand explanation and code examples.
Error Handling
Error handling in Flask allows you to gracefully manage and respond to errors that occur in your application. Instead of letting your app crash or display generic error messages, you can provide user-friendly responses and log the issues for further investigation.
Example: Handling 404 Errors
In Flask, you can handle specific HTTP errors by defining error handlers. For instance, if a user tries to access a page that doesn't exist, you might want to show a custom 404 error page.
Here’s how you can do it:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to the Home Page!"
@app.route('/about')
def about():
return "This is the About Page!"
# Custom 404 error handler
@app.errorhandler(404)
def page_not_found(e):
return render_template('404.html'), 404
if __name__ == '__main__':
app.run(debug=True)
In this code:
We define a route for the home page and about page.
The
@app.errorhandler(404)
decorator specifies that thepage_not_found
function will handle 404 errors.When a 404 error occurs, Flask will render the
404.html
template and return a 404 status code.
Logging
Logging helps you keep track of what happens in your application. It’s like keeping a diary of events, errors, and other useful information that can help in debugging and understanding application behavior.
Example: Basic Logging Setup
Here's how you can set up logging in your Flask application:
import logging
from flask import Flask
app = Flask(__name__)
# Set up basic logging
logging.basicConfig(filename='app.log', level=logging.DEBUG)
@app.route('/')
def home():
app.logger.info('Home page accessed')
return "Welcome to the Home Page!"
@app.route('/error')
def error():
app.logger.error('This is a test error')
return "This route causes an error!"
if __name__ == '__main__':
app.run(debug=True)
In this code:
We import the
logging
module and configure it to write logs toapp.log
with a level ofDEBUG
.app.logger.info
logs an informational message when the home page is accessed.app.logger.error
logs an error message when the/error
route is hit.
Summary
Error handling and logging are essential for building reliable Flask applications. Error handling helps you manage user errors gracefully, while logging provides valuable insights into your application's behavior. With these features, you can make your app more robust and easier to debug.
Feel free to tweak the error messages and logging levels based on your needs. And remember, good logging and error handling can make a huge difference in maintaining a healthy application!
Frequently Asked Question
What is Flask and why should I use it for web development?
Flask is a lightweight and flexible Python web framework that makes it easy to build web applications. It's known for its simplicity and allows developers to quickly get started with building APIs, web apps, or even complex websites. Flask provides essential tools and libraries while keeping the core framework simple, making it ideal for both beginners and experienced developers looking to create scalable web applications.
How do I structure a Flask project correctly?
Structuring a Flask project involves organizing your application into modules for better maintainability. A common approach is to create a main application package where you define your Flask app instance, and then separate concerns into modules like routes (for handling URLs), models (for database structures), and templates (for HTML rendering). This helps in keeping your codebase clean and makes it easier to add new features or scale your application.
What are the best security practices for Flask applications?
To ensure the security of your Flask application, always validate and sanitize user inputs to prevent SQL injection and XSS attacks. Use Flask extensions like Flask-WTF for secure form handling, and Flask-SQLAlchemy for database security. Implement strong password hashing with libraries like Werkzeug's
generate_password_hash
andcheck_password_hash
. Additionally, enable CSRF protection to prevent cross-site request forgery.How do I handle errors and exceptions in Flask?
Handling errors in Flask involves using error handlers and decorators. You can define custom error pages for HTTP errors using
@app.errorhandler
decorators, which allow you to return custom error responses or render specific error pages. For handling exceptions within your application, usetry-except
blocks to catch and manage exceptions gracefully, ensuring your application remains stable and informative to users.What are common database management mistakes in Flask?
Common mistakes in Flask database management include not using database migrations (like Flask-Migrate) to manage schema changes, inefficient query usage leading to performance bottlenecks, and not handling database connections properly (especially in multi-threaded environments). It's also important to avoid exposing sensitive database information in error messages and ensuring proper data validation to prevent data integrity issues.
How can I optimize the performance of my Flask application?
Optimizing Flask performance involves several strategies such as using caching mechanisms (like Flask-Cache or Redis) for frequently accessed data, optimizing database queries, minimizing unnecessary imports, and leveraging asynchronous tasks with Celery for background processing. Additionally, deploying your Flask app on a production server with optimized configurations (like Gunicorn or uWSGI) can significantly improve response times and scalability.
What testing tools are recommended for Flask applications?
For testing Flask applications, popular tools include pytest for unit testing, Flask-Testing for testing Flask extensions, and Selenium for end-to-end testing of web interfaces. You can also use libraries like Flask-DebugToolbar for debugging during development and Werkzeug's test client for simulating HTTP requests. These tools help ensure that your Flask application functions correctly across different scenarios and environments.
How do I deploy a Flask application?
Deploying a Flask application typically involves setting up a production-ready web server like Nginx or Apache to serve your Flask app via WSGI (Web Server Gateway Interface). You can use deployment tools like Docker for containerization, and platforms like Heroku, AWS Elastic Beanstalk, or DigitalOcean for cloud hosting. Ensure to configure environment variables securely and use HTTPS for secure communication.
Why is documentation important for a Flask project?
Documentation in a Flask project is crucial for developers, collaborators, and users to understand how the application works, its APIs, and configuration options. Good documentation improves maintainability, facilitates easier onboarding for new developers, and helps in troubleshooting and extending the application. It also serves as a reference guide for best practices, usage examples, and potential pitfalls.
Where can I find additional resources for learning Flask?
There are several resources available to learn Flask, including official documentation on Flask's website, tutorials on platforms like Real Python and Flask Mega-Tutorial by Miguel Grinberg, community forums like Stack Overflow and Reddit's r/flask subreddit, and Flask's official GitHub repository. Online courses on platforms like Udemy and Coursera also offer structured learning paths for beginners and advanced users alike.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
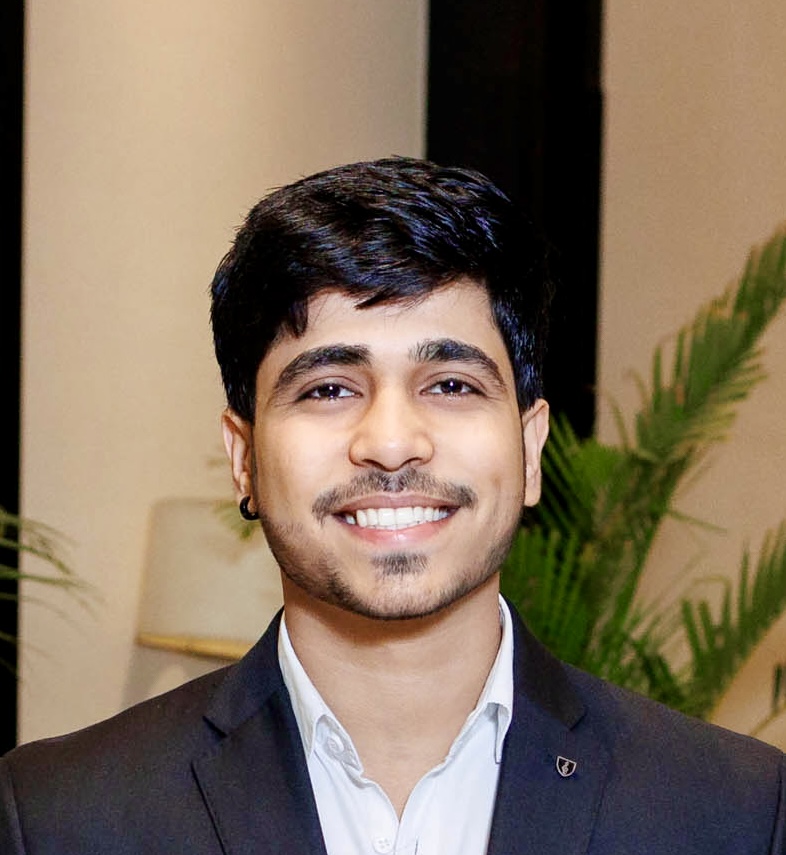
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.