Flutter Testing Tips
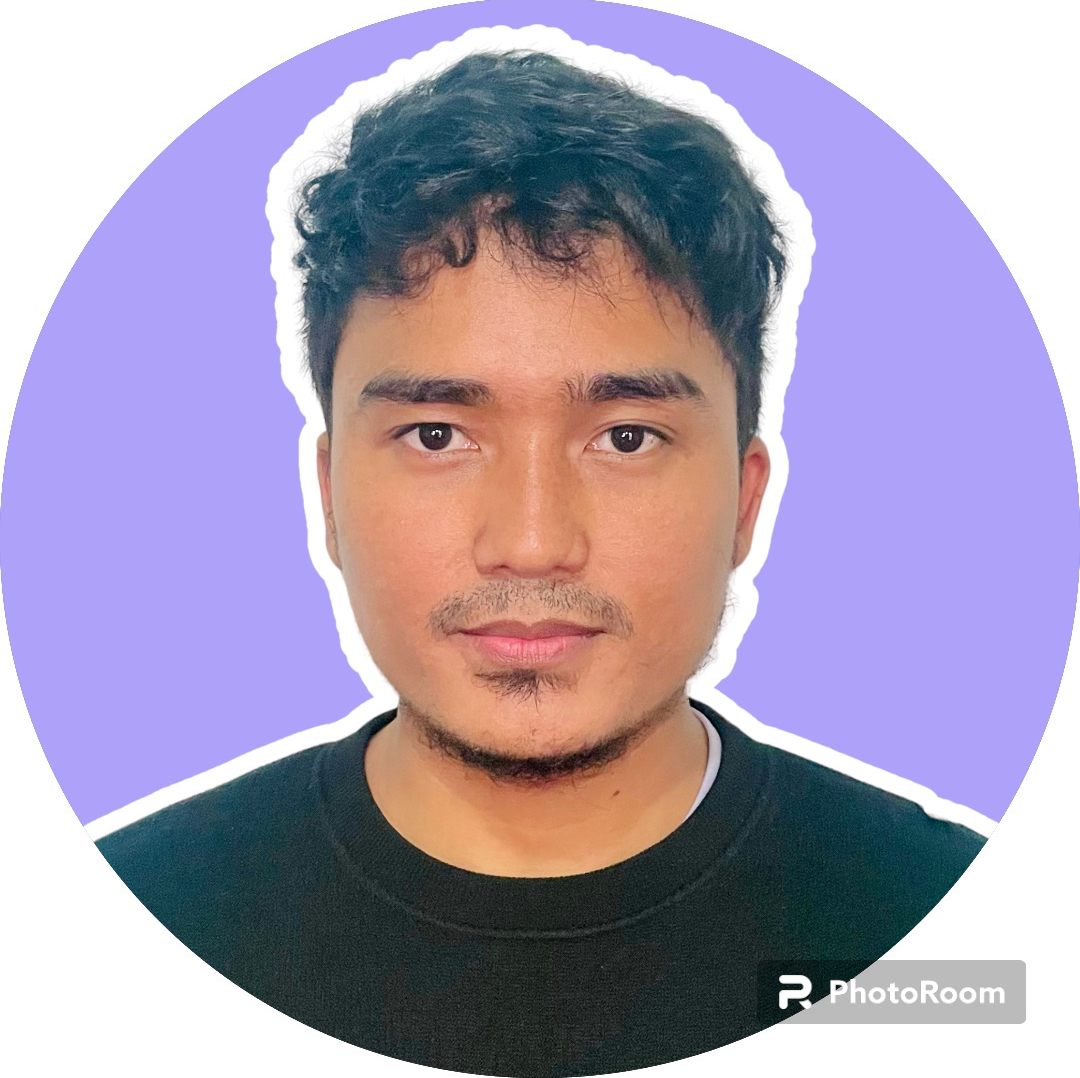
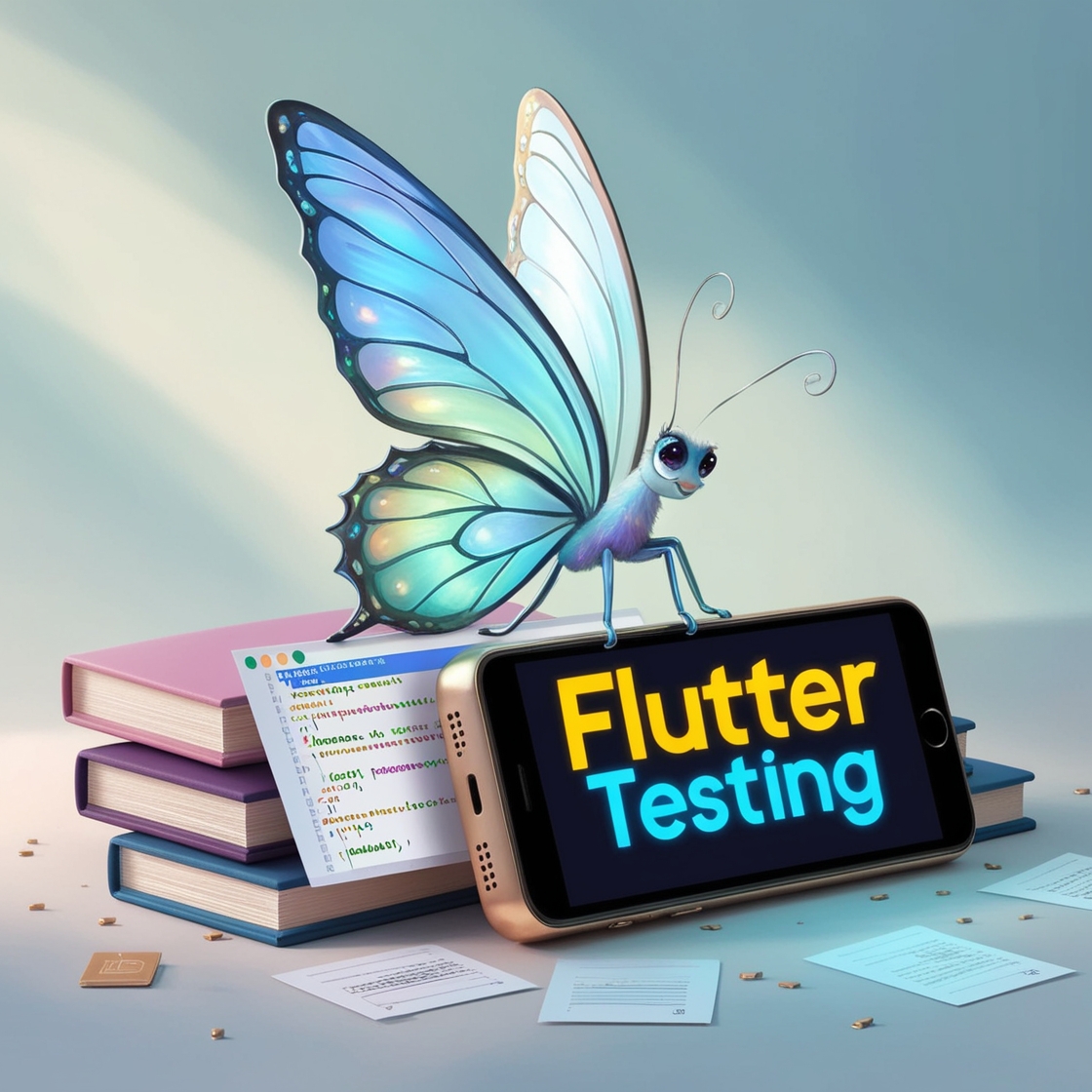
Streamlining Your Testing Workflow
Testing is an integral part of software development, and in Flutter, it's no different. Effective testing strategies ensure your app works as expected, catches bugs early, and makes maintenance easier. Here are some essential tips and techniques to help you make the most of Flutter's testing capabilities.
Using Test Tags in Flutter
What are Test Tags?
Ever wanted to filter tests so you only run the ones you need to? Test tags in Flutter make this possible, allowing you to target specific tests from the command line. This feature is especially useful when you have dozens or hundreds of tests.
How to Use Test Tags
Add Tags to Your Tests You can add one or more tags to your tests using the
tags
argument. Here's an example:dartCopy codevoid main() { testWidgets( 'Golden image test', (tester) async { await tester.pumpWidget(const MyApp()); await expectLater( find.byType(MyApp), matchesGoldenFile('golden_image.png'), ); }, tags: ['golden'], ); }
Configure Your Tags Create a
dart_test.yaml
file in your project with all the tags you intend to use:tags: golden:
Run Specific Tests Use the command line to run tests with specific tags:
flutter test --tags=golden
Tags are flexible and can be used to tag:
An entire test suite (using the
@Tags
annotation).A test group or an individual test (using the
tags
argument as shown above).
Developing a Comprehensive Testing Strategy
A well-rounded testing strategy combines various types of tests to maximize return on investment (ROI). In Flutter, you have several options:
Automated Testing (Part I)
Unit Testing:
Scenario: Testing individual methods or functions for correctness.
Example: Checking if a function returns the expected output given certain inputs.
Mocking Dependencies with Mocktail:
Scenario: Isolating the code under test by mocking dependencies.
Example: Using Mocktail to create mock instances of classes and verify interactions without relying on actual implementations.
// Example of using Mocktail class MockDependency extends Mock implements Dependency {} void main() { final mockDependency = MockDependency(); // Use mockDependency in your tests }
Test Matchers:
Scenario: Using matchers to assert conditions in tests.
Example: Using
expect
with matchers to validate test outcomes.expect(actual, equals(expected));
Testing with Given When Then:
Scenario: Structuring tests using the Given-When-Then format.
Example: Organizing tests to improve readability and maintainability.
test('Given some condition, When an action occurs, Then expect a result', () { // Given // When // Then });
Generating and Reporting Test Coverage Data:
Scenario: Measuring how much of your code is covered by tests and identifying areas that need more tests.
Example: Running tests with coverage options and generating coverage reports.
flutter test --coverage genhtml coverage/lcov.info -o coverage/html
Automated Testing (Part II)
Widget Testing:
Scenario: Testing the UI components in isolation.
Example: Ensuring a custom button widget behaves correctly and looks as expected.
Integration Testing:
Scenario: Testing how different parts of the app work together.
Example: Verifying that navigation between screens works as intended.
Robot Testing:
Scenario: Simulating user interactions programmatically.
Example: Automating complex user flows and interactions.
Golden Image Testing:
Scenario: Ensuring the visual appearance of widgets does not change unexpectedly.
Example: Comparing screenshots of widgets against a set of reference images to detect unintended changes.
Running Tests with GitHub Actions:
Scenario: Automating test runs as part of your CI/CD pipeline.
Example: Setting up GitHub Actions to run your tests on every push or pull request.
Conclusion
Incorporating effective testing strategies in your Flutter development process not only ensures your app's reliability but also enhances your productivity by catching issues early. Use test tags to streamline your workflow, and combine different types of tests for comprehensive coverage. Mock dependencies with Mocktail for isolated testing, use test matchers, follow the Given-When-Then format for structured testing, and generate test coverage data to ensure thorough testing. Implement various test scenarios including widget, integration, robot, and golden image tests, and automate your testing with GitHub Actions.
Happy testing!
Subscribe to my newsletter
Read articles from Nabraj Khadka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
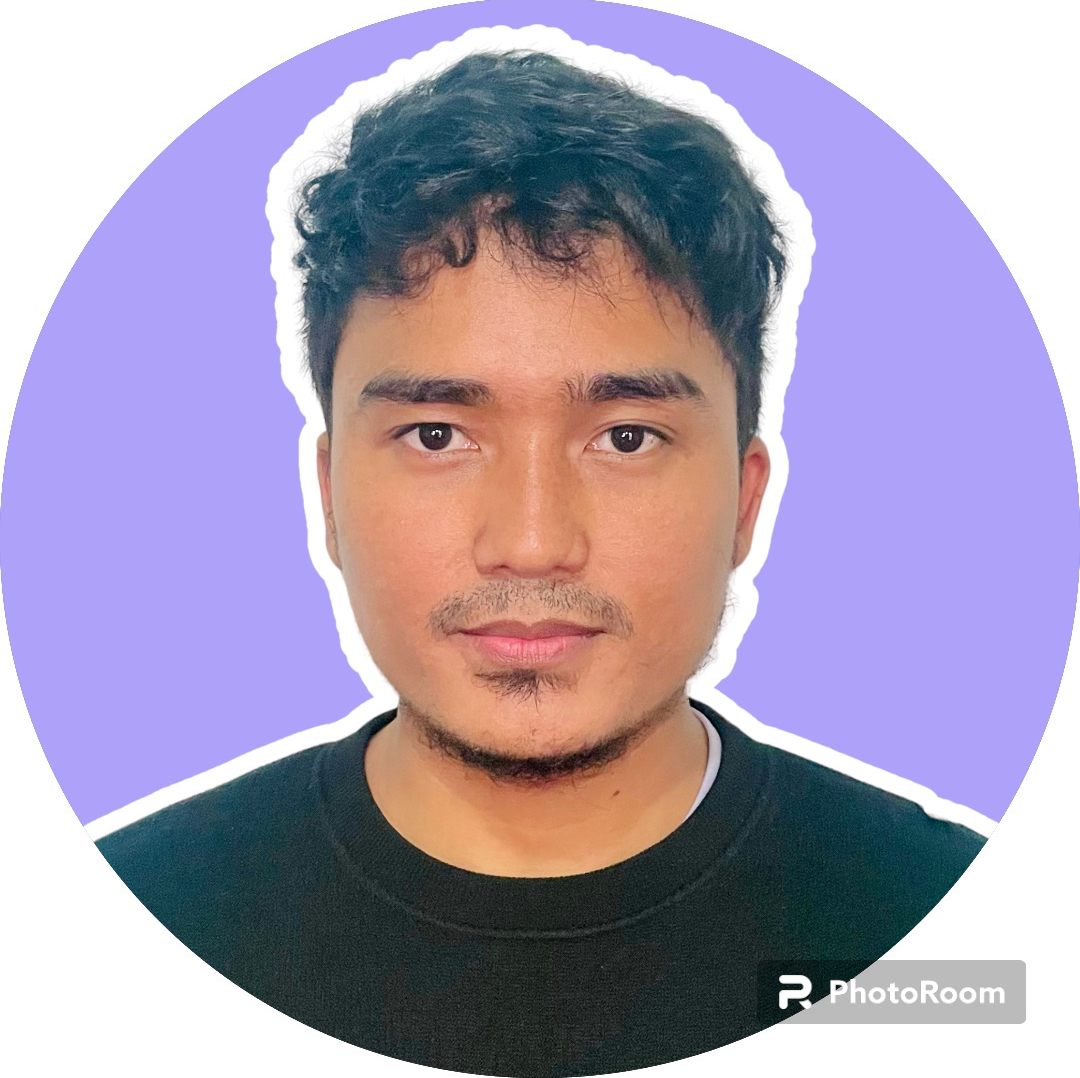
Nabraj Khadka
Nabraj Khadka
Experienced Flutter developer with 7+ years' expertise. Skilled in app creation, state management (e.g., Riverpod, Bloc, Getx), Firebase, Git, and project management. Proficient in layout design, APIs, firebase, cloud services, payment gateways Android SDK and bit of SwiftUI. Also versed in Java, Swift, Kotlin and PHP.