How to Store API Keys in Flutter: --dart-define vs .env Files
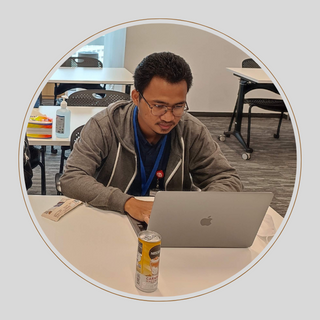
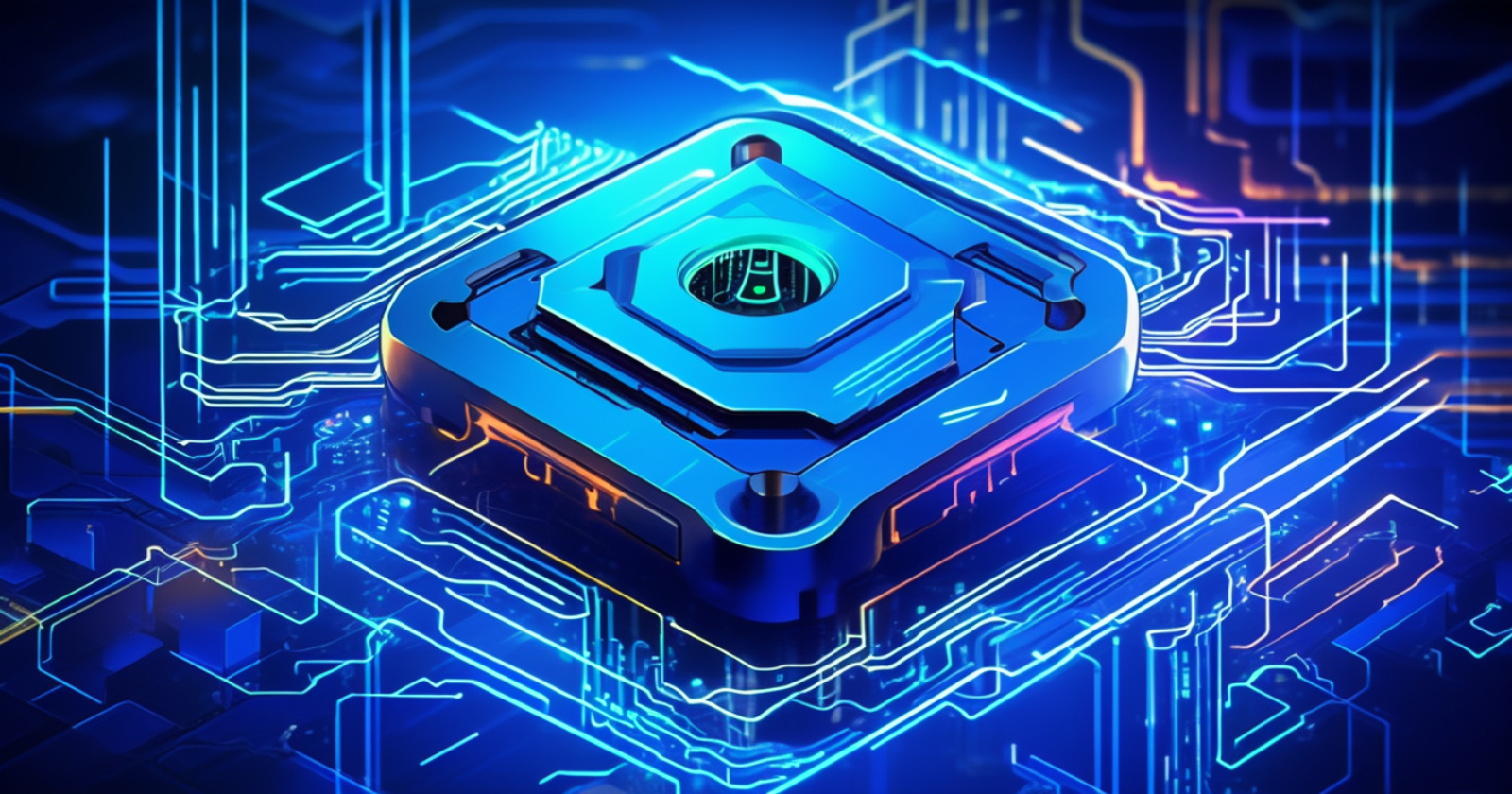
Handling sensitive data such as API keys securely is a crucial aspect of mobile application development. In Flutter, developers often face the challenge of deciding how to store and manage these keys securely. This article will explore two popular methods: using --dart-define
and .env
files, and also discuss the --dart-define-from-file
approach. We will examine the pros and cons of each method and provide best practices for ensuring security.
Why Security Matters
API keys are used to authenticate and authorize access to various services. If these keys are exposed, they can be exploited, leading to unauthorized access, data breaches, and potential financial loss. Therefore, securely managing API keys is essential to protect both the application and its users.
Method 1: Using --dart-define
The --dart-define
method allows developers to pass environment variables at build time. This approach helps keep sensitive information out of the source code and version control system.
How to Use --dart-define
Pass Variables at Build Time
When building the Flutter app, pass the API key as a parameter using the
--dart-define
flag:flutter build apk --dart-define=API_KEY=your_api_key
Access Variables in Code
Access the passed variables in your Flutter code using the
String.fromEnvironment
method:const String apiKey = String.fromEnvironment('API_KEY'); void main() { print('API Key: $apiKey'); runApp(MyApp()); }
Advantages of --dart-define
Security: API keys are not hardcoded in the source code or stored in plain text files.
Flexibility: Easily change environment variables for different build environments (development, staging, production).
Version Control: Keeps sensitive information out of version control systems.
Disadvantages of --dart-define
Build Complexity: Requires passing variables at build time, which can be cumbersome for multiple environments.
Manual Management: Developers need to remember to pass the variables each time they build the app.
Method 2: Using .env
Files
The .env
file method involves storing environment variables in a dedicated file and loading them at runtime. This approach is common in many development frameworks.
How to Use .env
Files
Install the
flutter_dotenv
PackageAdd the
flutter_dotenv
package to yourpubspec.yaml
file:dependencies: flutter: sdk: flutter flutter_dotenv: ^5.0.2
Create a
.env
FileCreate a
.env
file in the root directory of your project and add your API keys:API_KEY=your_api_key
Load Variables in Code
Load the
.env
file and access the variables in your Flutter code:import 'package:flutter/material.dart'; import 'package:flutter_dotenv/flutter_dotenv.dart'; void main() async { await dotenv.load(fileName: ".env"); String apiKey = dotenv.env['API_KEY'] ?? 'Unknown API Key'; print('API Key: $apiKey'); runApp(MyApp()); }
Advantages of .env
Files
Simplicity: Easy to manage and update environment variables.
Centralized Management: All environment variables are stored in a single file.
Disadvantages of .env
Files
Security Risks: If the
.env
file is not properly excluded from version control (using.gitignore
), it can expose sensitive information.Plain Text Storage: Storing API keys in plain text files can be a security risk if the file is compromised.
Method 3: Using --dart-define-from-file
The --dart-define-from-file
approach combines the security benefits of --dart-define
with the simplicity of managing variables in a file.
How to Use --dart-define-from-file
Create a JSON File
Create a JSON file (e.g.,
env.json
) in the root directory of your project with your environment variables:{ "API_KEY": "your_api_key" }
Build the App with
--dart-define-from-file
Pass the JSON file to the build command using the
--dart-define-from-file
flag:flutter build apk --dart-define-from-file=env.json
Access Variables in Code
Access the passed variables in your Flutter code using the
String.fromEnvironment
method:const String apiKey = String.fromEnvironment('API_KEY'); void main() { print('API Key: $apiKey'); runApp(MyApp()); }
Advantages of --dart-define-from-file
Security: Combines the security of
--dart-define
by keeping API keys out of source code.Ease of Management: Allows for centralized management of environment variables in a file.
Version Control: The JSON file can be excluded from version control for added security.
Disadvantages of --dart-define-from-file
Build Complexity: Requires passing the file at build time, which can be cumbersome for multiple environments.
Manual Management: Developers need to remember to pass the file each time they build the app.
Best Practices for Storing API Keys
1. Avoid Hardcoding API Keys
Never hardcode API keys directly in your source code. This practice can lead to accidental exposure and security vulnerabilities.
2. Use Environment Variables
Use environment variables to manage sensitive information. This approach keeps your API keys secure and separate from your source code.
3. Exclude Sensitive Files from Version Control
Ensure that files containing sensitive information, such as .env
files or JSON files used with --dart-define-from-file
, are excluded from version control using .gitignore
:
# .gitignore
.env
env.json
4. Use Secrets Management Services
For added security, consider using secrets management services like AWS Secrets Manager, Google Secret Manager, or HashiCorp Vault. These services provide secure storage and management of sensitive information.
5. Regularly Rotate API Keys
Regularly rotate your API keys to minimize the risk of them being compromised. Implement a process to update keys and redeploy your application as needed.
6. Monitor and Audit API Key Usage
Monitor and audit the usage of your API keys to detect any unauthorized access or unusual activity. This practice can help you identify potential security breaches early.
Conclusion
Storing and managing API keys securely is essential for protecting your Flutter applications and user data. The --dart-define
, .env
files, and --dart-define-from-file
methods each offer unique advantages and disadvantages. By understanding these methods and following best practices, you can ensure that your API keys are stored securely and your application remains protected.
Choose the method that best suits your needs and workflow, and always prioritize security when handling sensitive information. With the right approach, you can maintain the integrity and security of your Flutter applications while leveraging the power of APIs.
Subscribe to my newsletter
Read articles from Anaz S. Aji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
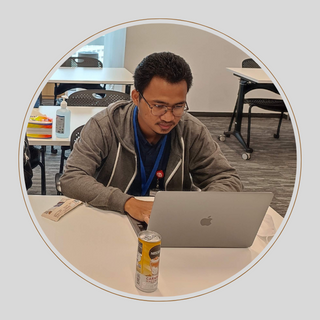
Anaz S. Aji
Anaz S. Aji
I am a dedicated Mobile Developer with a strong passion for exploring the latest advancements in technology and the dynamic world of cryptocurrency. My curiosity and enthusiasm drive me to continuously seek out and experiment with innovative solutions, ensuring that I stay at the forefront of industry trends and developments.