Learn Node.js File System: Easy Step-by-Step Guide
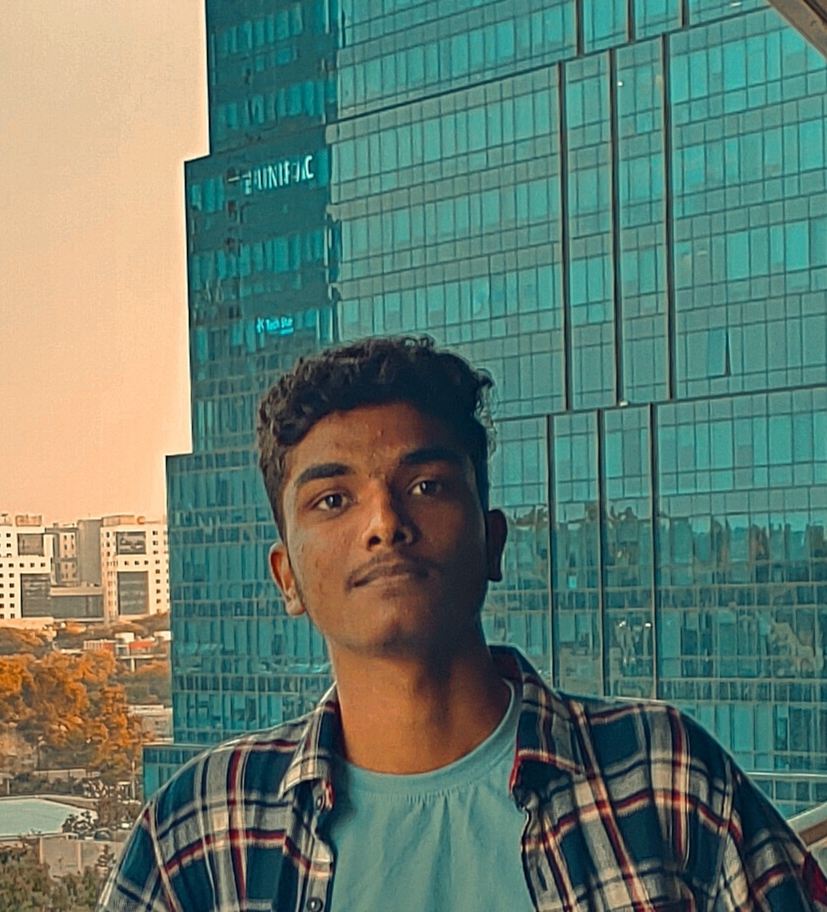
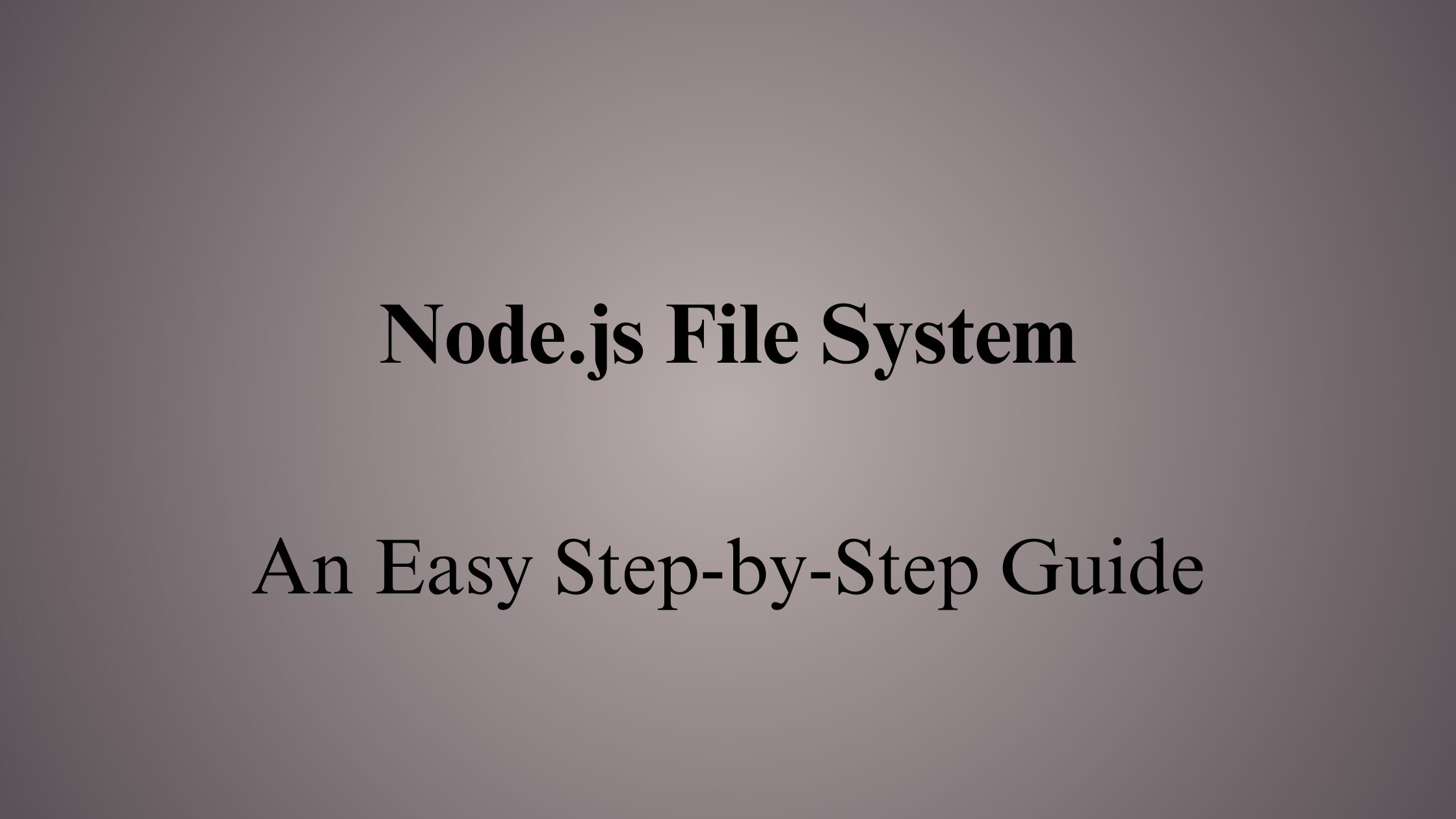
Node.js is known for its powerful capabilities, and one of its core modules is the File System (fs) module. This module allows you to interact with the file system in a way that is simple yet efficient. In this blog, we'll explore the basics of the Node.js File System module, complete with easy-to-understand examples.
Introduction to Node.js File System
The File System (fs) module in Node.js provides an API for interacting with the file system. You can use it to perform operations like reading, writing, updating, deleting, and renaming files and directories. The fs module is included with Node.js, so there's no need to install it separately.
To use the fs module, you first need to require it in your Node.js application:
const fs = require('fs');
Reading Files
Reading files is one of the most common tasks. You can read files in a synchronous or asynchronous manner.
Asynchronous Reading:
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
In this example, fs.readFile
reads the content of example.txt
. The second parameter, 'utf8'
, specifies the encoding. The callback function handles the file content or any errors that occur.
Synchronous Reading:
try {
const data = fs.readFileSync('example.txt', 'utf8');
console.log(data);
} catch (err) {
console.error(err);
}
Here, fs.readFileSync
reads the file content synchronously. If an error occurs, it is caught in the catch block.
Writing Files
Writing to files is equally straightforward and can also be done asynchronously or synchronously.
Asynchronous Writing:
const content = 'Hello, Node.js!';
fs.writeFile('example.txt', content, err => {
if (err) {
console.error(err);
return;
}
console.log('File written successfully!');
});
In this example, fs.writeFile
writes content
to example.txt
. If the file doesn't exist, it will be created.
Synchronous Writing:
const content = 'Hello, Node.js!';
try {
fs.writeFileSync('example.txt', content);
console.log('File written successfully!');
} catch (err) {
console.error(err);
}
Here, fs.writeFileSync
writes the content synchronously.
Appending to Files
To append data to an existing file, you can use fs.appendFile
or fs.appendFileSync
.
Asynchronous Appending:
const additionalContent = '\nAppending this text.';
fs.appendFile('example.txt', additionalContent, err => {
if (err) {
console.error(err);
return;
}
console.log('Content appended successfully!');
});
Synchronous Appending:
const additionalContent = '\nAppending this text.';
try {
fs.appendFileSync('example.txt', additionalContent);
console.log('Content appended successfully!');
} catch (err) {
console.error(err);
}
Deleting Files
You can delete files using fs.unlink
or fs.unlinkSync
.
Asynchronous Deleting:
fs.unlink('example.txt', err => {
if (err) {
console.error(err);
return;
}
console.log('File deleted successfully!');
});
Synchronous Deleting:
try {
fs.unlinkSync('example.txt');
console.log('File deleted successfully!');
} catch (err) {
console.error(err);
}
Renaming Files
Renaming files is also quite simple with fs.rename
or fs.renameSync
.
Asynchronous Renaming:
fs.rename('oldname.txt', 'newname.txt', err => {
if (err) {
console.error(err);
return;
}
console.log('File renamed successfully!');
});
Synchronous Renaming:
try {
fs.renameSync('oldname.txt', 'newname.txt');
console.log('File renamed successfully!');
} catch (err) {
console.error(err);
}
Conclusion
The Node.js File System module is a powerful tool for handling file operations in your applications. Whether you need to read, write, append, delete, or rename files, the fs module has got you covered. By understanding and utilizing these basic file operations, you can build efficient applications that interact seamlessly with the file system.
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
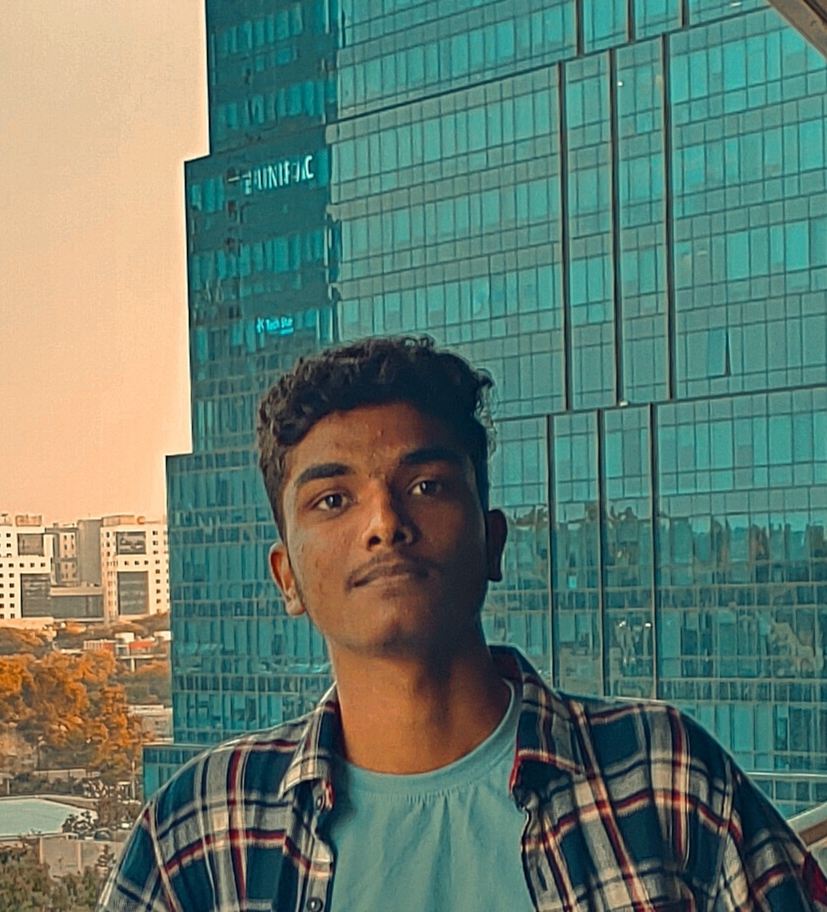
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.