Exploring Phoenix LiveView: Real-Time Web Development in Elixir
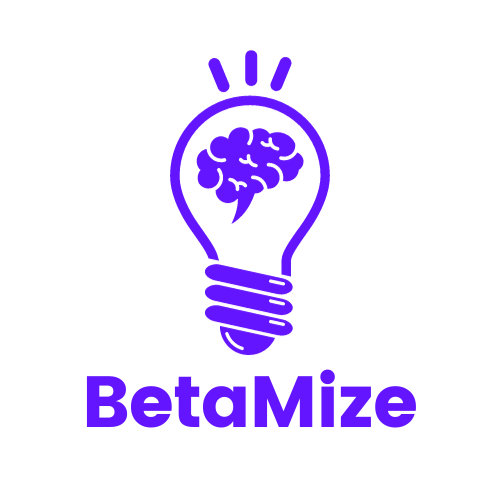
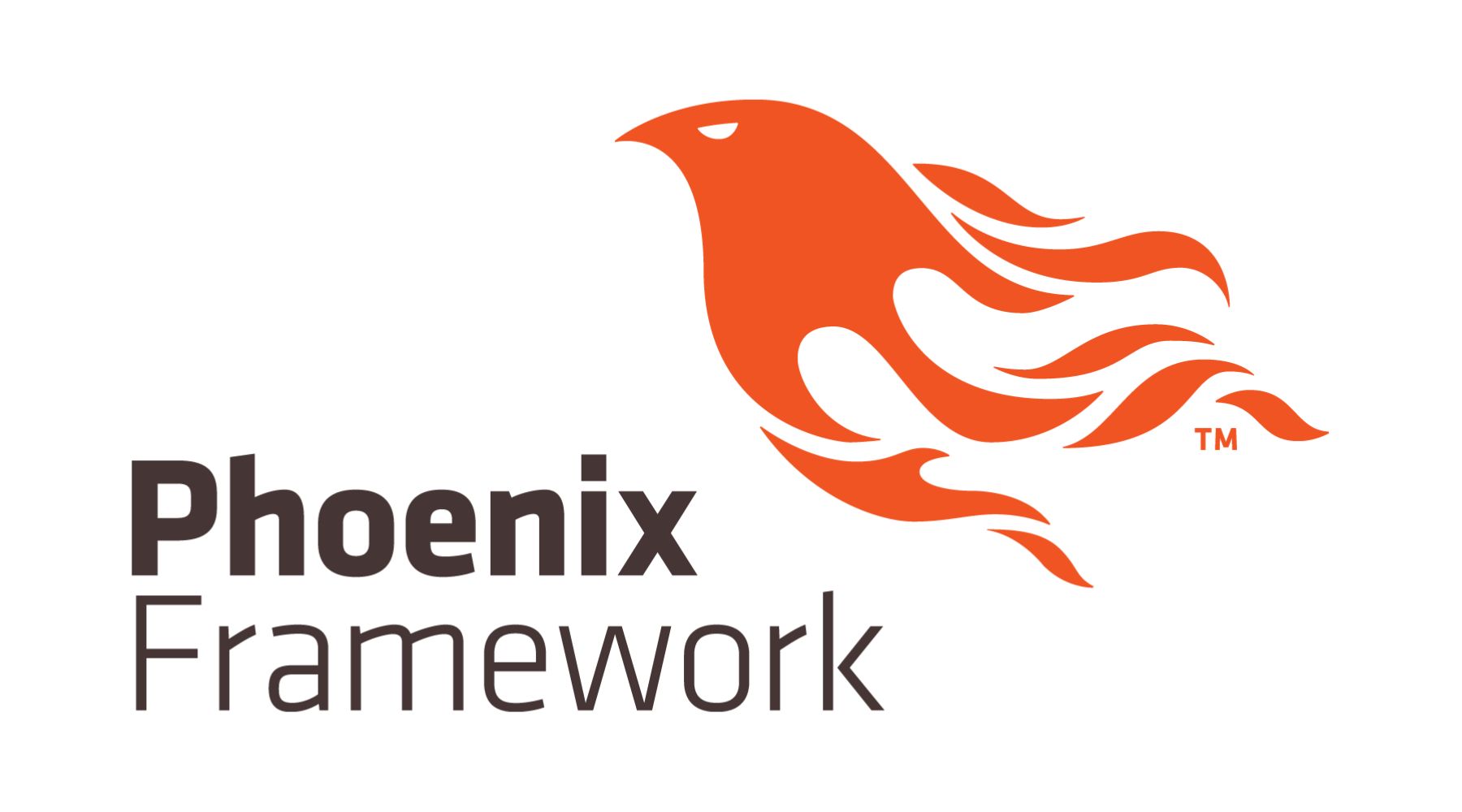
Phoenix LiveView is a groundbreaking library for Elixir that enables the creation of rich, real-time web interfaces without the need for complex JavaScript frameworks. Built on top of the Phoenix framework, LiveView leverages Elixir's strengths in concurrency and fault-tolerance to deliver highly interactive user experiences with minimal client-side code. In this article, we'll dive into what makes LiveView special, explore its key features, and provide practical examples to help you get started.
What is Phoenix LiveView?
Phoenix LiveView allows developers to build interactive web applications with real-time updates directly in Elixir. Traditionally, creating dynamic web interfaces required significant JavaScript, often leading to increased complexity and maintenance overhead. LiveView simplifies this by handling real-time communication between the server and client through WebSockets, allowing you to write reactive interfaces in a straightforward manner.
Key Features of Phoenix LiveView
1. Real-Time Updates
LiveView updates the user interface in real-time as data changes on the server. This is ideal for applications that require live updates, such as dashboards, notifications, and chat applications.
defmodule MyAppWeb.CounterLive do
use Phoenix.LiveView
def render(assigns) do
~L"""
<div>
<h1>Count: <%= @count %></h1>
<button phx-click="increment">Increment</button>
</div>
"""
end
def mount(_params, _session, socket) do
{:ok, assign(socket, :count, 0)}
end
def handle_event("increment", _value, socket) do
{:noreply, update(socket, :count, &(&1 + 1))}
end
end
In this example, a counter is incremented in real-time whenever the user clicks the button, without the need for page reloads.
2. Minimal JavaScript
LiveView minimizes the need for custom JavaScript by handling client-server interactions transparently. This reduces complexity and the risk of bugs, while keeping the codebase clean and maintainable.
3. Server-Side Logic
With LiveView, most of the application logic resides on the server. This ensures consistency and leverages Elixir's powerful concurrency model to handle multiple connections efficiently.
4. Seamless Integration with Phoenix
LiveView integrates seamlessly with the Phoenix framework, allowing you to reuse existing components and leverage Phoenix's features such as routing, templating, and data validation.
5. Fault-Tolerance
Built on the Erlang VM, LiveView benefits from Elixir's fault-tolerance and resilience. Processes can crash and restart without affecting the overall application stability.
Getting Started with Phoenix LiveView
To start using LiveView in your Phoenix project, follow these steps:
1. Install Phoenix LiveView
Add the following dependencies to your mix.exs
file:
defp deps do
[
{:phoenix_live_view, "~> 0.17.5"},
{:phoenix_live_dashboard, "~> 0.5"},
{:floki, ">= 0.30.0", only: :test}
]
end
Run mix deps.get
to install the dependencies.
2. Update Endpoint Configuration
Update your endpoint configuration in lib/my_app_web/endpoint.ex
to include the LiveView socket:
socket "/live", Phoenix.LiveView.Socket, websocket: [connect_info: [session: @session_options]]
3. Add LiveView JavaScript
Include the LiveView JavaScript in your assets/js/app.js
file:
import {Socket} from "phoenix"
import {LiveSocket} from "phoenix_live_view"
let csrfToken = document.querySelector("meta[name='csrf-token']").getAttribute("content")
let liveSocket = new LiveSocket("/live", Socket, {params: {_csrf_token: csrfToken}})
liveSocket.connect()
4. Create a LiveView Module
Create a LiveView module in lib/my_app_web/live/counter_live.ex
:
defmodule MyAppWeb.CounterLive do
use Phoenix.LiveView
def render(assigns) do
~L"""
<div>
<h1>Count: <%= @count %></h1>
<button phx-click="increment">Increment</button>
</div>
"""
end
def mount(_params, _session, socket) do
{:ok, assign(socket, :count, 0)}
end
def handle_event("increment", _value, socket) do
{:noreply, update(socket, :count, &(&1 + 1))}
end
end
5. Add Route
Add a route to your LiveView in lib/my_app_web/router.ex
:
live "/counter", MyAppWeb.CounterLive
Now, you can navigate to /counter
in your browser to see the LiveView in action.
Practical Examples
1. Live Search
Implementing live search with LiveView is straightforward. The user inputs a search term, and results update in real-time.
defmodule MyAppWeb.SearchLive do
use Phoenix.LiveView
def render(assigns) do
~L"""
<div>
<input type="text" phx-keyup="search" placeholder="Search..." value="<%= @query %>"/>
<ul>
<%= for result <- @results do %>
<li><%= result %></li>
<% end %>
</ul>
</div>
"""
end
def mount(_params, _session, socket) do
{:ok, assign(socket, query: "", results: [])}
end
def handle_event("search", %{"value" => query}, socket) do
results = search_database(query)
{:noreply, assign(socket, query: query, results: results)}
end
defp search_database(query) do
# Simulate database search
["result1", "result2", "result3"]
|> Enum.filter(&String.contains?(&1, query))
end
end
2. Live Notifications
LiveView can be used to push notifications to users in real-time.
defmodule MyAppWeb.NotificationLive do
use Phoenix.LiveView
def render(assigns) do
~L"""
<div id="notifications">
<%= for notification <- @notifications do %>
<div><%= notification %></div>
<% end %>
</div>
"""
end
def mount(_params, _session, socket) do
if connected?(socket), do: Process.send_after(self(), :notify, 5000)
{:ok, assign(socket, notifications: [])}
end
def handle_info(:notify, socket) do
notifications = ["New notification" | socket.assigns.notifications]
Process.send_after(self(), :notify, 5000)
{:noreply, assign(socket, notifications: notifications)}
end
end
Conclusion
Phoenix LiveView revolutionizes web development by enabling real-time, interactive user experiences without the complexity of JavaScript frameworks. By leveraging Elixir's strengths in concurrency and fault-tolerance, LiveView allows developers to build scalable and maintainable applications with ease.
Whether you're building a live chat application, real-time dashboards, or dynamic forms, LiveView provides the tools you need to create responsive and engaging web interfaces. Start exploring the power of Phoenix LiveView today and see how it can transform your web development projects.
Ready to build real-time applications with Elixir and Phoenix LiveView? Visit ElixirMasters for expert development services, developer hiring solutions, and consultancy. Elevate your project with the power of LiveView and Elixir!
Subscribe to my newsletter
Read articles from BetaMize directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
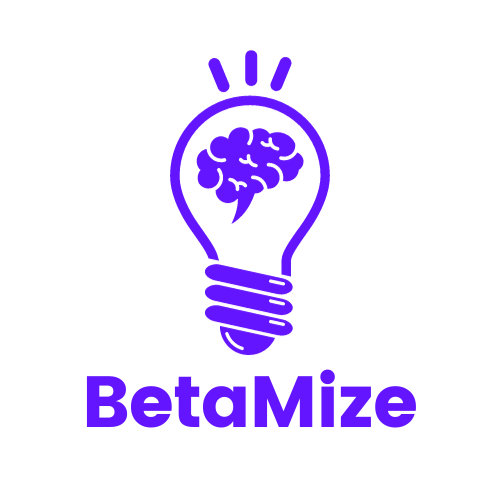