✍️Day 18: Essential Python Libraries for DevOps
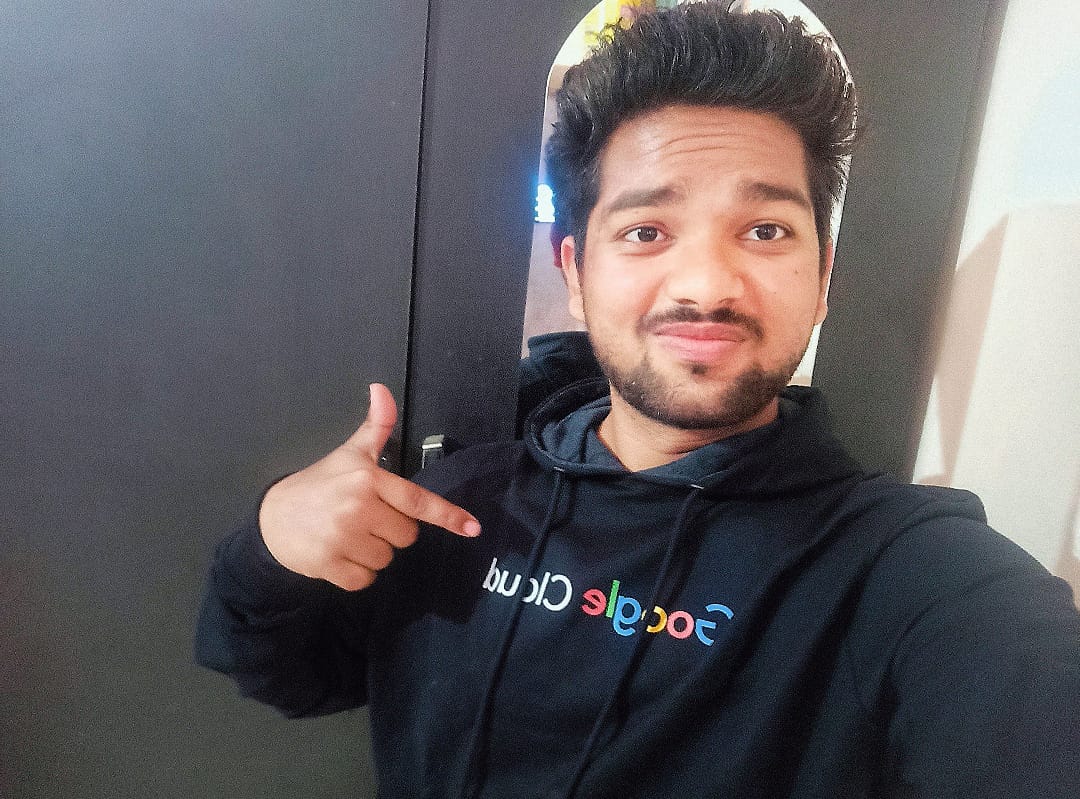
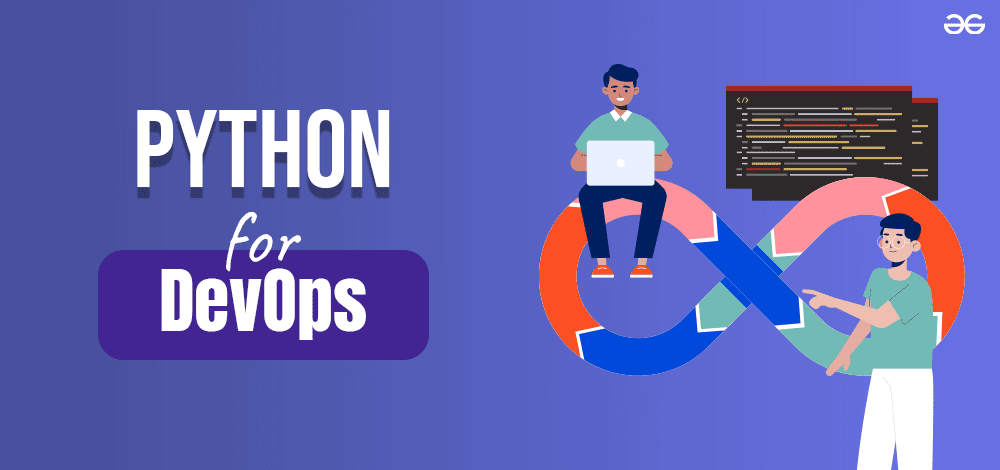
Hey everyone! Today, I delved into some essential Python libraries that every DevOps engineer should know about. These tools make automating tasks and managing systems much more efficient. Here’s a friendly breakdown of what I learned:
1. os and sys Libraries
These are like your Python toolkit for interacting with your computer’s operating system.
os: Think of this as your file manager in Python. With it, you can create new files, delete old ones, or navigate through directories—all from your code.
Example: Checking the current working directory.
import os print(os.getcwd()) # Prints the current directory
sys: This is like the command-line helper. It helps your script understand inputs you provide when you run it and manage system-specific details.
Example: Accessing inputs given when running the script.
import sys print(sys.argv) # Prints the list of arguments passed to the script
2. subprocess Library
Think of this as your Python script's way of running other programs or commands on your computer.
subprocess: This library allows your Python code to run shell commands (like
ls
ordir
) and get the results back.Example: Listing all files in a directory.
import subprocess result = subprocess.run(['ls', '-l'], capture_output=True, text=True) print(result.stdout) # Prints the result of the 'ls' command
3. requests Library
Imagine this library as your Python script’s way of browsing the web.
requests: It makes it easy to send HTTP requests (like visiting a website) and receive responses back. This is great for interacting with web services or APIs.
Example: Getting information from a website.
import requests response = requests.get('https://api.github.com') print(response.json()) # Prints the data retrieved from the website
4. PyYAML Library
YAML is a simple way to write and read configuration data. This library helps you handle YAML files easily.
PyYAML: This library makes it easy to read and write YAML files, which are often used for configuration settings.
Example: Reading configuration settings from a YAML file.
import yaml with open('config.yaml', 'r') as file: config = yaml.safe_load(file) print(config) # Prints the data loaded from the YAML file
5. docker-py Library
Docker helps package applications into containers. This library lets you control Docker directly from your Python script.
docker-py: This library is your Python bridge to Docker. You can use it to manage containers, images, and more without leaving your script.
Example: Listing all running Docker containers.
import docker client = docker.from_env() for container in client.containers.list(): print(container.name) # Prints the name of each running container
Conclusion
These libraries are indispensable for streamlining DevOps workflows, enhancing productivity, and ensuring robust automation. As we continue this 90 Days of DevOps journey, the knowledge gained today will serve as a solid foundation for more advanced topics in the coming days.
Happy coding! 🎉
Subscribe to my newsletter
Read articles from Ritesh Dolare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
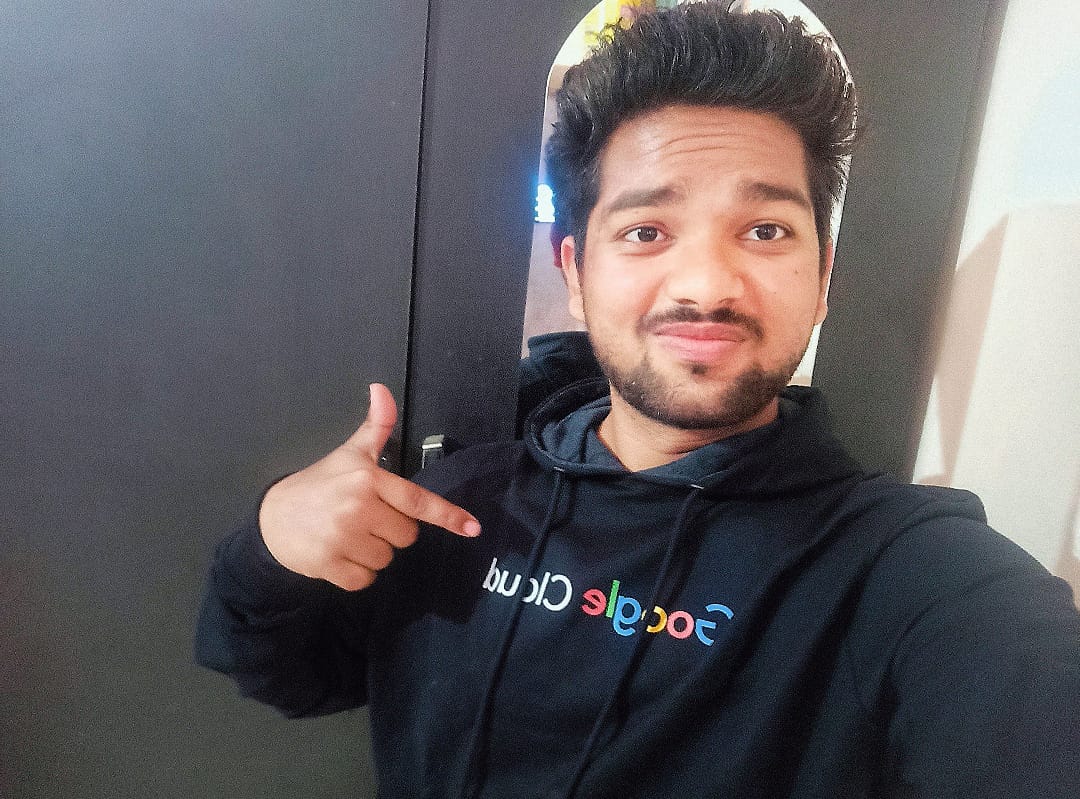
Ritesh Dolare
Ritesh Dolare
👋 Hi, I'm Ritesh Dolare, a DevOps enthusiast dedicated to mastering the art of DevOps.