How to Store Files on AWS S3 from Your Next.js App Using App Router: A Comprehensive Guide
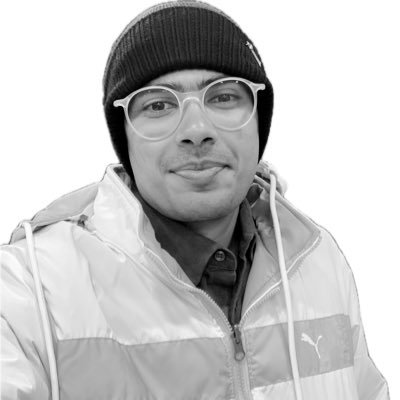
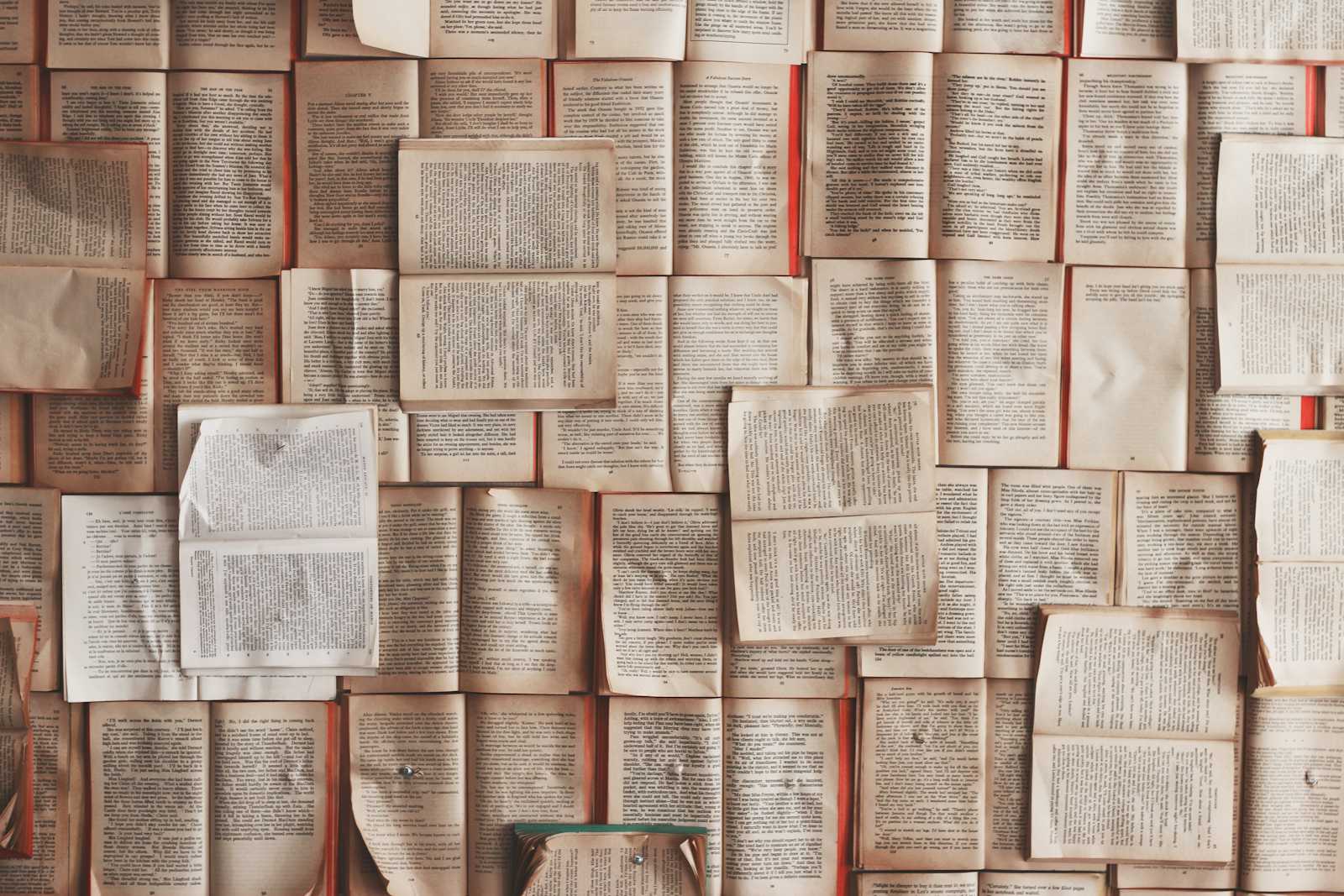
How to Store Files on AWS S3 from Your Next.js App Using App Router: A Comprehensive Guide 🌟
Storing files in the cloud is a common requirement for modern web applications. Amazon Web Services (AWS) Simple Storage Service (S3) is a powerful and scalable solution. In this blog post, we’ll walk through the steps to store files on AWS S3 from your Next.js app using the app router. 🚀
Why Use AWS S3 for File Storage? 🤔
AWS S3 offers numerous benefits:
Scalability: Automatically scales to handle high volumes of data. 📈
Durability: Provides 99.999999999% durability for your data. 💾
Security: Offers various security features including encryption and access control. 🔒
Cost-Effective: Pay only for what you use with flexible pricing options. 💸
Prerequisites 🛠️
Before we begin, ensure you have the following:
An AWS account.
Node.js and npm installed on your machine.
A Next.js application set up.
Step 1: Set Up AWS S3 🏗️
1. Create an S3 Bucket
Log in to the AWS Management Console.
Navigate to the S3 service.
Click on “Create bucket.”
Enter a unique bucket name and choose a region.
Configure the bucket settings as per your requirements and click “Create bucket.”
2. Configure Bucket Permissions
Select your bucket and go to the “Permissions” tab.
Edit the “Block public access” settings as needed.
Set bucket policies to control access permissions.
3. Create IAM User
Navigate to the IAM service in the AWS Management Console.
Click on “Users” and then “Add user.”
Enter a username and select “Programmatic access”.
Attach the “AmazonS3FullAccess” policy (or create a custom policy with restricted permissions).
Download the user’s access key ID and secret access key.
Step 2: Set Up Your Next.js App 🌐
1. Install AWS SDK
In your Next.js project directory, install the AWS SDK:
npm install aws-sdk
2. Create an API Route for File Upload
Create a new API route in your Next.js app to handle file uploads. In the app/api
directory, create a file named upload.js
:
// app/api/upload/route.js
import aws from 'aws-sdk';
aws.config.update({
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY,
region: process.env.AWS_REGION,
});
const s3 = new aws.S3();
export async function POST(request) {
const { file } = await request.json();
const params = {
Bucket: process.env.AWS_S3_BUCKET_NAME,
Key: file.name,
Body: Buffer.from(file.content, 'base64'),
ContentType: file.type,
ACL: 'public-read', // Adjust as needed
};
try {
const data = await s3.upload(params).promise();
return new Response(JSON.stringify({ url: data.Location }), { status: 200 });
} catch (error) {
console.error(error);
return new Response(JSON.stringify({ error: 'Error uploading file' }), { status: 500 });
}
}
3. Create a File Upload Form 📤
Create a file upload form in one of your Next.js pages:
// app/page.js
'use client';
import { useState } from 'react';
export default function Home() {
const [file, setFile] = useState(null);
const [message, setMessage] = useState('');
const handleFileChange = (event) => {
setFile(event.target.files[0]);
};
const handleUpload = async () => {
if (!file) return;
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onloadend = async () => {
const base64data = reader.result.split(',')[1];
const response = await fetch('/api/upload', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
file: {
name: file.name,
content: base64data,
type: file.type,
},
}),
});
const data = await response.json();
if (response.ok) {
setMessage(`File uploaded successfully: ${data.url}`);
} else {
setMessage(`Error uploading file: ${data.error}`);
}
};
};
return (
<div>
<h1>Upload File to AWS S3</h1>
<input type="file" onChange={handleFileChange} />
<button onClick={handleUpload}>Upload</button>
{message && <p>{message}</p>}
</div>
);
}
4. Configure Environment Variables 🔐
Create a .env.local
file in the root of your project and add your AWS credentials and bucket information:
AWS_ACCESS_KEY_ID=your-access-key-id
AWS_SECRET_ACCESS_KEY=your-secret-access-key
AWS_REGION=your-region
AWS_S3_BUCKET_NAME=your-bucket-name
Make sure to add .env.local
to your .gitignore
to keep your credentials secure.
By following these steps, you can successfully integrate AWS S3 with your Next.js app using the app router to store files in the cloud. As you continue to enhance your project, remember that focusing on core functionalities and user experience is key. 💡
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
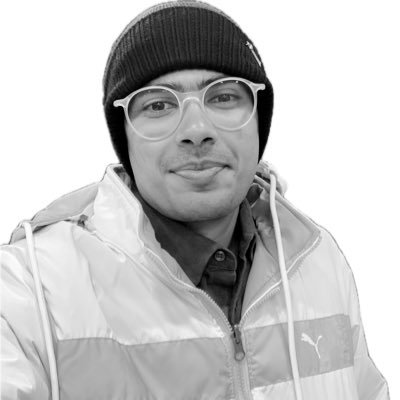
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on ⌨️ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix 👨🏻💻