Tackling OWASP Top 10 Vulnerabilities in Java Spring framework
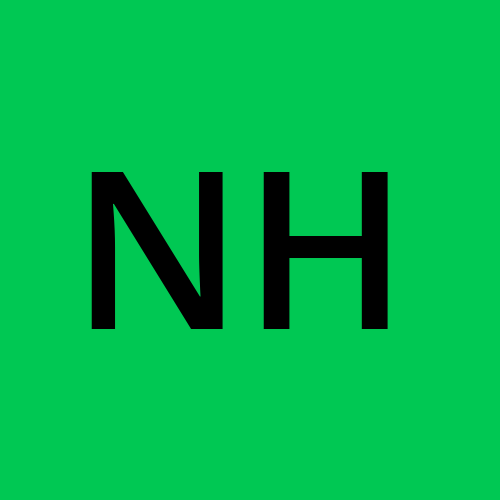
In the realm of web application security, the OWASP (Open Web Application Security Project) Top 10 list is a crucial resource. It identifies the most critical security risks to web applications. Understanding these vulnerabilities and knowing how to mitigate them is essential for developers. In this blog, we’ll explore each of the OWASP Top 10 vulnerabilities and demonstrate how to address them using Java Spring.
1. Injection
Overview
Injection flaws, such as SQL, NoSQL, OS, and LDAP injection, occur when untrusted data is sent to an interpreter as part of a command or query.
Example
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/user")
public User getUser(@RequestParam String username) {
String query = "SELECT * FROM users WHERE username = '" + username + "'";
return userRepository.findUserByQuery(query);
}
}
Mitigation
Use prepared statements and parameterized queries to avoid injection attacks.
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/user")
public User getUser(@RequestParam String username) {
return userRepository.findUserByUsername(username);
}
}
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.username = :username")
User findUserByUsername(@Param("username") String username);
}
2. Broken Authentication
Overview
Authentication mechanisms are often implemented incorrectly, allowing attackers to compromise passwords, keys, or session tokens.
Example
@RestController
public class AuthController {
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest loginRequest) {
// Basic login logic without security checks
return ResponseEntity.ok("Logged in");
}
}
Mitigation
Use Spring Security to handle authentication securely.
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
3. Sensitive Data Exposure
Overview
Sensitive data, such as financial, healthcare, or PII, should be protected both at rest and in transit.
Example
public class UserService {
public void saveUser(User user) {
// Save user data without encryption
}
}
Mitigation
Use encryption for sensitive data and HTTPS for secure data transmission.
public class UserService {
private static final String SECRET_KEY = "secret";
public void saveUser(User user) {
user.setPassword(encrypt(user.getPassword()));
// Save encrypted user data
}
private String encrypt(String data) {
// Encryption logic
}
}
Configure HTTPS in Spring Boot.
server:
port: 8443
ssl:
key-store: classpath:keystore.jks
key-store-password: changeit
key-password: changeit
4. XML External Entities (XXE)
Overview
Attackers can exploit vulnerable XML processors if they can upload XML or include hostile content in an XML document.
Example
public void parseXml(String xmlContent) {
// Parsing XML without disabling external entities
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.parse(new InputSource(new StringReader(xmlContent)));
}
Mitigation
Disable external entities in XML parsers.
public void parseXml(String xmlContent) throws Exception {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setFeature("http://apache.org/xml/features/disallow-doctype-decl", true);
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.parse(new InputSource(new StringReader(xmlContent)));
}
5. Broken Access Control
Overview
Restrictions on what authenticated users are allowed to do are often not properly enforced.
Example
@RestController
public class AdminController {
@GetMapping("/admin")
public String adminPage() {
return "Admin page";
}
}
Mitigation
Use annotations and security configuration to enforce access control.
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.formLogin()
.permitAll();
}
}
@RestController
public class AdminController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/admin")
public String adminPage() {
return "Admin page";
}
}
6. Security Misconfiguration
Overview
Security settings should be defined, implemented, and maintained as defaults are often insecure.
Example
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Mitigation
Ensure proper security configurations and regular updates.
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.csrf().disable()
.headers()
.frameOptions().deny()
.and()
.authorizeRequests()
.anyRequest().authenticated();
return http.build();
}
}
7. Cross-Site Scripting (XSS)
Overview
XSS flaws occur when an application includes untrusted data in a new web page without proper validation or escaping.
Example
@RestController
public class CommentController {
@PostMapping("/comment")
public ResponseEntity<String> postComment(@RequestBody String comment) {
return ResponseEntity.ok("Comment received: " + comment);
}
}
Mitigation
Use encoding libraries to sanitize user input.
@RestController
public class CommentController {
@PostMapping("/comment")
public ResponseEntity<String> postComment(@RequestBody String comment) {
String sanitizedComment = HtmlUtils.htmlEscape(comment);
return ResponseEntity.ok("Comment received: " + sanitizedComment);
}
}
8. Insecure Deserialization
Overview
Insecure deserialization often leads to remote code execution.
Example
public class UserController {
public User deserializeUser(String userData) throws IOException, ClassNotFoundException {
ByteArrayInputStream bis = new ByteArrayInputStream(Base64.getDecoder().decode(userData));
ObjectInputStream ois = new ObjectInputStream(bis);
return (User) ois.readObject();
}
}
Mitigation
Avoid Java serialization where possible. Use secure methods for data exchange.
public class UserController {
public User deserializeUser(String userData) throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.readValue(userData, User.class);
}
}
9. Using Components with Known Vulnerabilities
Overview
Vulnerable components, such as libraries and frameworks, can be exploited to attack applications.
Example
Using outdated libraries in pom.xml
.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>4.3.2.RELEASE</version>
</dependency>
Mitigation
Regularly update dependencies and use tools like OWASP Dependency-Check.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.9</version>
</dependency>
10. Insufficient Logging & Monitoring
Overview
Lack of logging and monitoring can delay the detection and response to security breaches.
Example
@RestController
public class LoginController {
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody LoginRequest request) {
// Login logic without logging
return ResponseEntity.ok("Logged in");
}
}
Mitigation
Implement comprehensive logging and monitoring.
@RestController
public class LoginController {
private static final Logger logger = LoggerFactory.getLogger(LoginController.class);
@PostMapping("/login")
public ResponseEntity<String> login(@RequestBody LoginRequest request) {
logger.info("Login attempt for user: {}", request.getUsername());
// Login logic
return ResponseEntity.ok("Logged in");
}
}
Conclusion
Securing web applications requires a thorough understanding of potential vulnerabilities and proactive measures to mitigate them. The OWASP Top 10 list provides an excellent framework for identifying and addressing the most critical security risks. By implementing these best practices in Java Spring applications, you can significantly reduce the risk of security breaches and ensure your applications remain robust and secure.
Here's a quick summary of the steps to mitigate each OWASP Top 10 vulnerability in Java Spring:
Injection: Use prepared statements and parameterized queries.
Broken Authentication: Implement secure authentication mechanisms using Spring Security.
Sensitive Data Exposure: Encrypt sensitive data and use HTTPS for secure data transmission.
XML External Entities (XXE): Disable external entities in XML parsers.
Broken Access Control: Enforce access control with annotations and security configurations.
Security Misconfiguration: Ensure proper security configurations and keep dependencies updated.
Cross-Site Scripting (XSS): Sanitize user inputs with encoding libraries.
Insecure Deserialization: Avoid Java serialization and use secure data exchange methods.
Using Components with Known Vulnerabilities: Regularly update dependencies and use vulnerability scanning tools.
Insufficient Logging & Monitoring: Implement comprehensive logging and monitoring mechanisms.
By addressing these vulnerabilities, developers can create more secure applications, protecting both their users and their data.
Practical Example of a Secure Spring Boot Application
Here is a comprehensive example of a secure Spring Boot application that addresses multiple OWASP Top 10 vulnerabilities:
@SpringBootApplication
public class SecureAppApplication {
public static void main(String[] args) {
SpringApplication.run(SecureAppApplication.class, args);
}
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.csrf().disable()
.authorizeRequests()
.antMatchers("/admin").hasRole("ADMIN")
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
return http.build();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Bean
public DataSource dataSource() {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/securedb");
config.setUsername("dbuser");
config.setPassword("dbpass");
config.addDataSourceProperty("cachePrepStmts", "true");
config.addDataSourceProperty("prepStmtCacheSize", "250");
config.addDataSourceProperty("prepStmtCacheSqlLimit", "2048");
return new HikariDataSource(config);
}
}
@RestController
public class SecureController {
private static final Logger logger = LoggerFactory.getLogger(SecureController.class);
@Autowired
private UserRepository userRepository;
@GetMapping("/user")
public User getUser(@RequestParam String username) {
return userRepository.findUserByUsername(username);
}
@PostMapping("/login")
public ResponseEntity<?> login(@RequestBody LoginRequest loginRequest) {
logger.info("Login attempt for user: {}", loginRequest.getUsername());
// Authenticate user logic
return ResponseEntity.ok("Logged in");
}
@PostMapping("/comment")
public ResponseEntity<String> postComment(@RequestBody String comment) {
String sanitizedComment = HtmlUtils.htmlEscape(comment);
return ResponseEntity.ok("Comment received: " + sanitizedComment);
}
@PostMapping("/user")
public ResponseEntity<?> createUser(@RequestBody User user) {
user.setPassword(new BCryptPasswordEncoder().encode(user.getPassword()));
userRepository.save(user);
return ResponseEntity.ok("User created");
}
}
Key Takeaways
Injection: Use parameterized queries.
Broken Authentication: Secure authentication using Spring Security.
Sensitive Data Exposure: Encrypt data and use HTTPS.
XML External Entities (XXE): Disable external entities.
Broken Access Control: Implement access control measures.
Security Misconfiguration: Properly configure security settings.
Cross-Site Scripting (XSS): Sanitize inputs.
Insecure Deserialization: Use secure deserialization methods.
Using Components with Known Vulnerabilities: Keep dependencies updated.
Insufficient Logging & Monitoring: Implement detailed logging and monitoring.
By incorporating these practices, you can significantly enhance the security of your Java Spring applications, ensuring they are resilient against the most common and critical security threats. Happy coding and stay secure!
Subscribe to my newsletter
Read articles from Nipun Hegde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
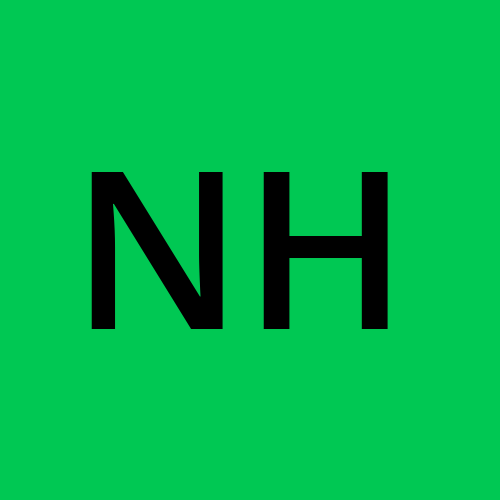
Nipun Hegde
Nipun Hegde
I'm a passionate software engineer always eager to learn and explore new technologies. Beyond coding, I'm deeply interested in DevOps and finance, constantly keeping up with the latest trends and innovations.I also enjoy sharing my knowledge through technical blogs, writing about the exciting things I learn.