Spring Beans 101: Basics for Building Your First Spring Application
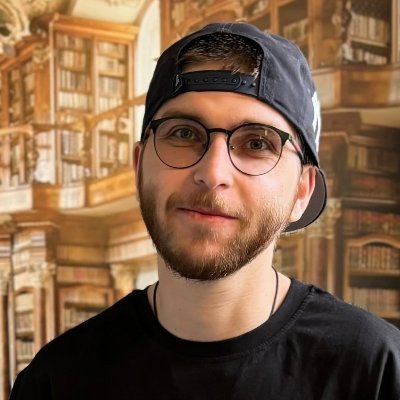
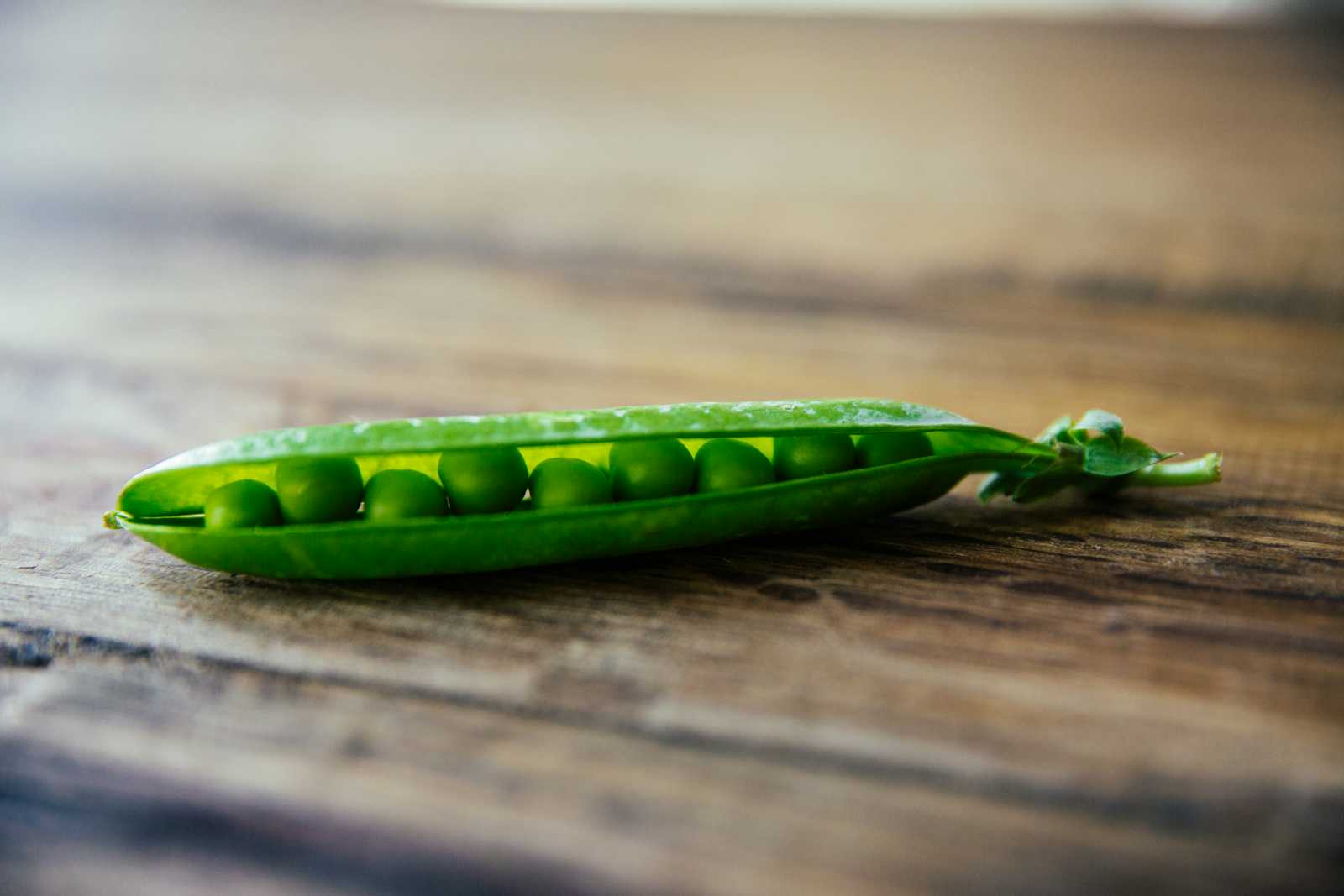
Ever built a Lego castle? You start with individual bricks, but those bricks come together to form something much cooler, right? Spring Beans are like those bricks for your Java application built with the Spring Framework.
In this post, we'll explain what Spring Beans are and why they're important for building well-organized Spring applications.
What are Spring Beans?
Imagine a toolbox filled with different tools – a hammer, screwdriver, wrench, etc. Each tool has a specific purpose, right? Spring Beans are like that. They're individual objects in your application that represent specific functionalities or data. These objects can be anything from a simple message printer to a complex database connection handler.
Why use Spring Beans?
Here's the cool part: you don't have to create and manage these Beans yourself. Spring takes care of it! Spring has a special component called the IoC Container (a fancy term for "Inversion of Control"). This container does several important things:
Creates your Beans based on your instructions. You define how you want your Beans to be created, and the IoC Container follows those instructions to instantiate them. This can include specifying the class to use, any initialization parameters, and even the scope of the Bean (like whether it should be a singleton or a prototype).
Manages their lifecycle (when they're created and destroyed). The IoC Container keeps track of when each Bean is needed and ensures they are created and destroyed at the right times. For example, a Bean might be created when the application starts up and destroyed when the application shuts down. This management helps to optimize resource usage and ensures that Beans are available when needed.
Wires them together – imagine connecting your Lego bricks! This means if one Bean needs something that another Bean provides, Spring handles the connection. For instance, if you have a Bean that handles database connections and another Bean that performs data operations, Spring will automatically inject the database connection Bean into the data operations Bean. This wiring is done through dependency injection, which can be configured via annotations or XML.
By using the IoC Container, you can focus on defining the behavior and relationships of your Beans without worrying about the underlying creation and management details. This leads to cleaner, more maintainable code and allows you to build complex applications more efficiently.
Benefits of using Spring Beans:
Clean and organized code: No more manual object creation and management, making your code easier to understand and maintain.
Loose coupling: Beans don't depend on how other Beans are implemented, just what functionality they provide. This makes your code more flexible and easier to test.
Reusability: Beans can be reused throughout your application, saving you time and effort.
Creating a Spring Bean
This code creates a Bean called messagePrinter
. You can then use this Bean in other parts of your application to print messages.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public MessagePrinter messagePrinter() {
return new MessagePrinter();
}
}
Conclusion
In conclusion, Spring Beans are fundamental building blocks for any Spring application, much like Lego bricks for a castle. They simplify the development process by allowing the Spring IoC Container to handle the creation, management, and wiring of these Beans. This results in cleaner, more organized code, promotes loose coupling, and enhances reusability. By leveraging Spring Beans, developers can focus on defining the behavior and relationships of their components, leading to more maintainable and efficient applications. Whether you're building a simple message printer or a complex system, understanding and utilizing Spring Beans is essential for any Java developer working with the Spring Framework.
Subscribe to my newsletter
Read articles from Christian Lehnert directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
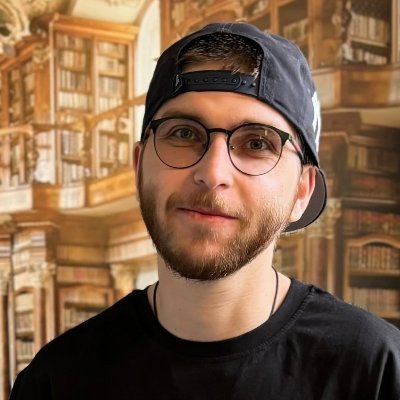
Christian Lehnert
Christian Lehnert
SR. Developer & CEO | Reduced Server Costs by 50% | 6+ Years Experience as Backend Developer | Expertise in Python, Java & Spring Boot | 30+ Certifications