Jumpstarting Flask Applications
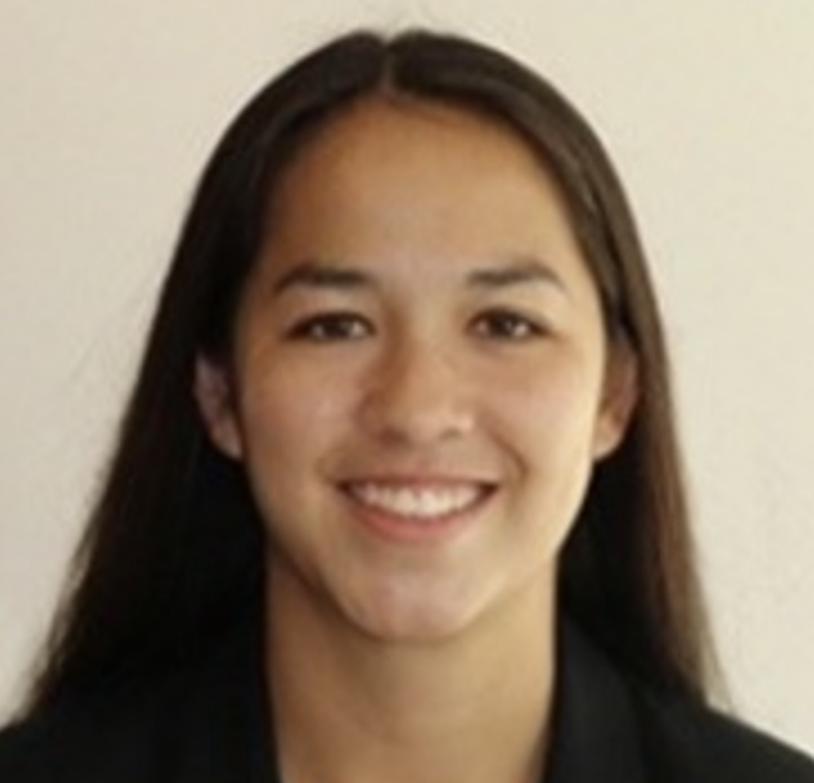
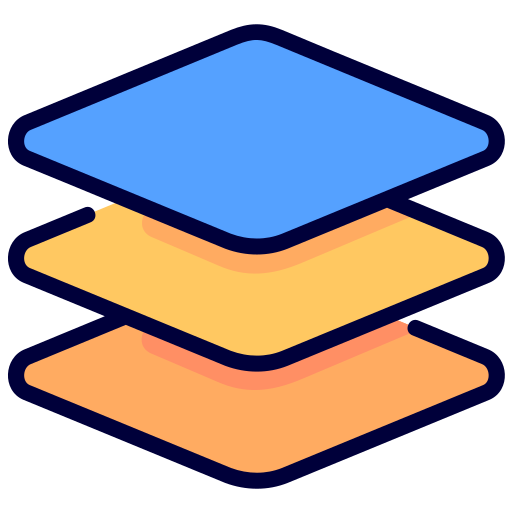
It's time to talk about Flask! No matter if you are early in your development career or a highly experienced programmer, chances are you have heard of Flask. It is one of the most popular web frameworks out there, and it is used by some of the biggest companies like Samsung, Netflix, Uber and Lyft!
Flask is popular for a number of reasons. It is a microframework and therefore highly configurable and easy to use when you want to get a project off the ground quickly. In fact, by the time you finish reading this blog, you will know how to jumpstart your own Flask application from scratch! Don't believe me? Keep on reading!
In the following sections, we will go through the basic steps of setting up a Flask application. Beginning with step one, let’s start with our environment. Flask is a microframework for Python, so we must first activate our virtual environment. Open up your shell from the directory in which you want to create your project. Type the command "pipenv install --python <version>" with whichever version of Python you would like to use (e.g. --python 3.8.13). Next, install Flask with the command "pipenv install flask".
After this, you should see your virtual environment has been created, along with a Pipfile containing the Python version and the Flask package. Type "pipenv shell" to enter your virtual environment. Because we are working on the back end server, we will create a "server" folder and build our application within it. Let's make an "app.py" file within this server folder and get to work. First, we need to import the Flask class from the package we previously installed and create an instance for our application:
# server/app.py
from flask import Flask
app = Flask(__name__)
The "__name__" argument will be discussed below, but the important takeaway is that our app is an instance of the Flask class. From here on out, any further configuration we perform will be on this new object (i.e. app).
As our code stands, there is nothing actually happening with app, so we need to include code to run it. Sounds easy enough, right? Well, it actually is:
# server/app.py
from flask import Flask
app = Flask(__name__)
app.run(port=5555, debug=True)
All we needed is one line of code to run our app! As you might have deduced, our program is running on port 5555 and we have set debug = True for development purposes. This might sound crazy but...this is technically all you need - we have ourselves a Flask application! Test it out by running "python app.py" from within the server directory. You should see we are up and running! Don't worry if there is a "Not Found" message in your browser. We have not yet defined any routes, so this is perfectly normal. The important piece is to confirm in the terminal that your development server is running.
One important note: we will likely export app as a module at some point in the development process, and we don't necessarily want our server to run if this is the case. To prevent this from happening and ensure that our app runs only when we execute our script directly, we can do the following:
# server/app.py
from flask import Flask
app = Flask(__name__)
if __name__ == "__main__":
app.run(port=5555, debug=True)
By including this conditional, we ensure our server runs only when our script is run directly, as we did above: "python app.py". If we imported app from a separate module "other.py" and ran "python other.py", then our server would not run. This is exactly the behavior we want.
Let's now go one step further and create our first route so we can look at something a bit more appealing than this "Not Found" error. Remember our overarching goal: we are developing a back end server that will be accessed by our front end application. Our server will eventually be wired to connect to a database, so we want to create routes that our front end can follow in order to access certain data to display.
Fortunately, Flask makes this quite easy. All we need to do is add an @app.route decorator, pass in the new route, and define a function that will manage the behavior of our application at that particular route. If, for example, we wanted to define our home route and simply show a welcome message to our users, we could do the following:
# server/app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def welcome_user():
return '<h1>Welcome to our Flask app!</h1>'
if __name__ == "__main__":
app.run(port=5555, debug=True)
Congratulations on creating your first route! As you can see, we've passed in "/" to our decorator, so whenever users navigate to this route, they will be greeted with a welcome message. Of course, this is a very simple example, but you can imagine how powerful this will eventually be. Once we connect to a database, we can include functionality to grab or make changes to our stored data. And data can also be returned in other formats (hint, hint...JSON!) that our front end applications can access and render to the user in all different ways!
So there you have it. When ready, try the steps above to get started with your own Flask application. Experiment with creating new routes and rendering different messages to your users. Once comfortable with that, your next step will be to incorporate SQLAlchemy into your application in order to build out your app's database functionality. Hopefully you are getting excited at this point, as you've taken your first steps toward building powerful Flask applications!
Subscribe to my newsletter
Read articles from Taylor Ng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
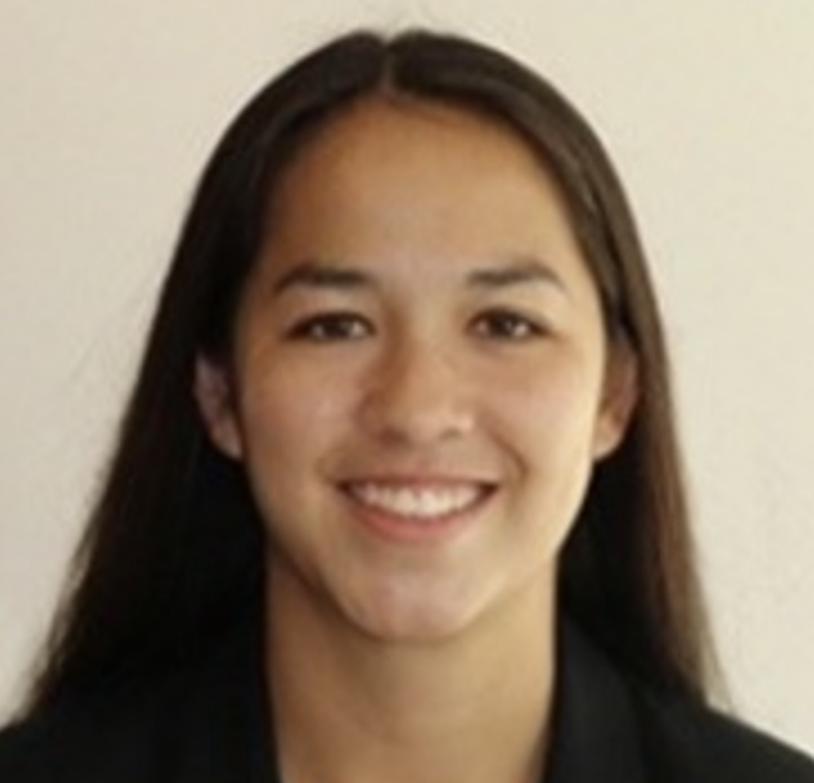