Dreaming in OCaml: Exploring the Power of Dream Web Framework
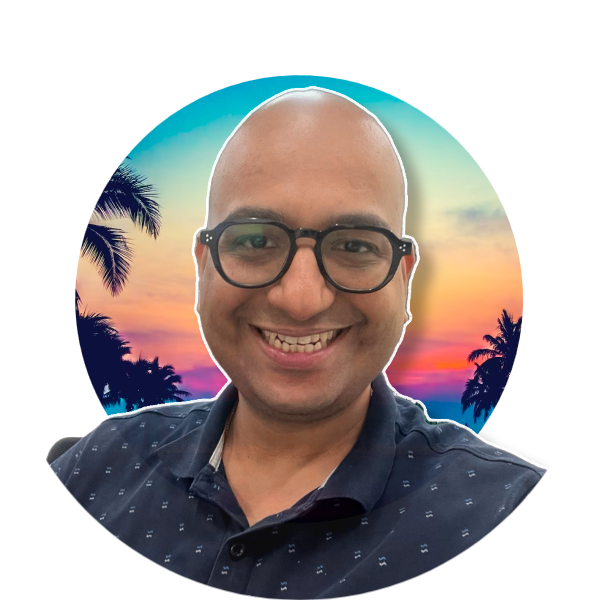
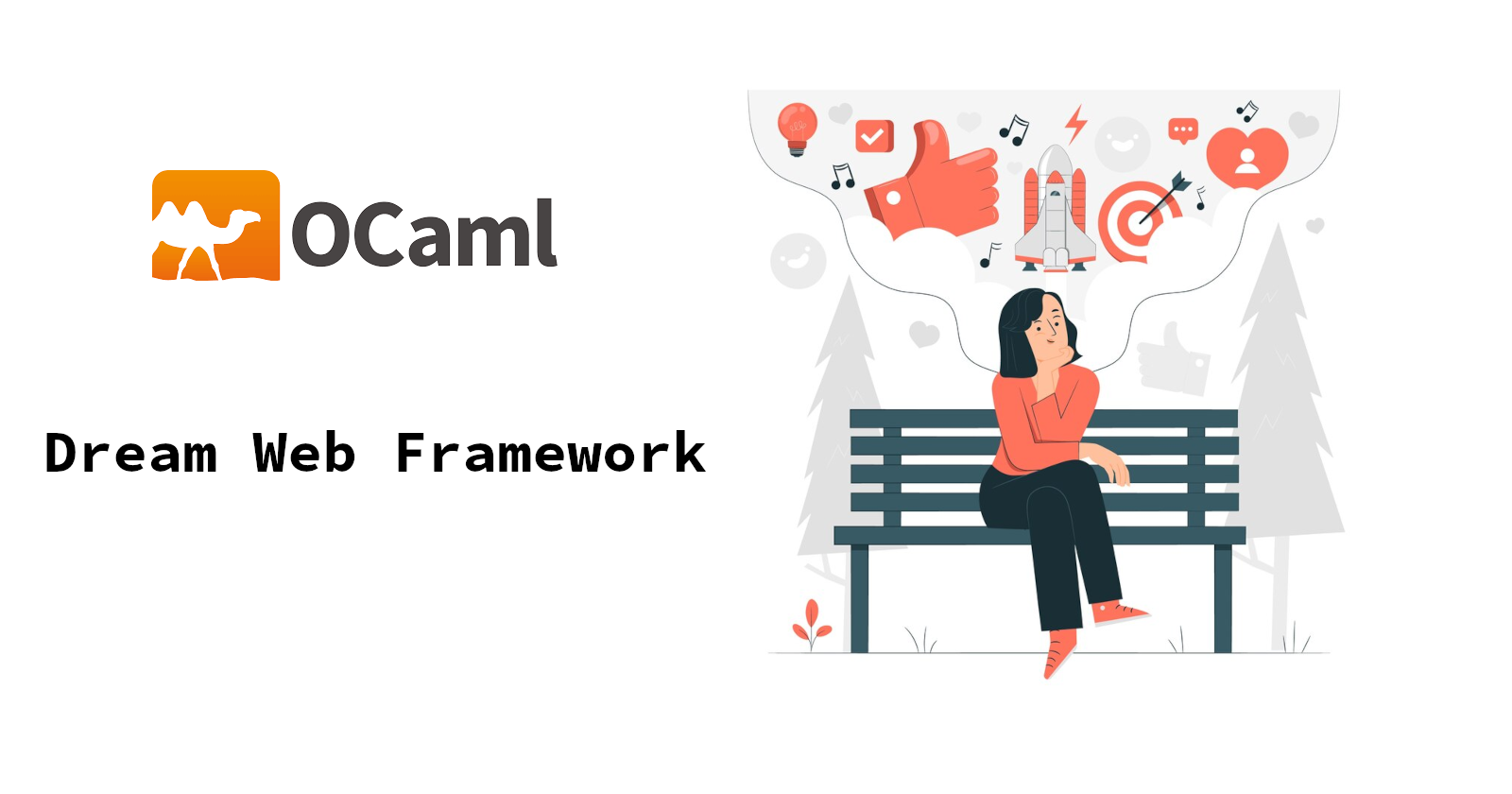
Dream is a lightweight, high-performance web framework for OCaml that aims to simplify web development while leveraging the language's strong type system and functional programming paradigm. Developed by Anton Bachin, Dream provides a cohesive set of tools for building web applications, APIs, and microservices. It combines simplicity with power, offering an intuitive API that allows developers to create robust web solutions quickly and efficiently.
Dream stands out for its minimalist approach, focusing on essential features without unnecessary complexity. It provides a solid foundation for web development in OCaml, including routing, request handling, templating, and WebSocket support. The framework's design emphasizes type safety, composability, and performance, making it an attractive choice for developers who value these qualities in their web projects.
Examples
- Basic "Hello, World!" server:
let () =
Dream.run
@@ Dream.logger
@@ Dream.router [
Dream.get "/"
(fun _ -> Dream.html "Hello, World!");
]
- Handling route parameters:
let greet who =
Dream.run
@@ Dream.logger
@@ Dream.router [
Dream.get "/hello/:name"
(fun request ->
let name = Dream.param request "name" in
Dream.html (Printf.sprintf "Hello, %s!" name));
]
let () = greet ()
- JSON API endpoint:
let json_handler _ =
let data = `Assoc [
("message", `String "Hello, JSON!");
("timestamp", `Int (int_of_float (Unix.time ())))
] in
Dream.json (Yojson.Safe.to_string data)
let () =
Dream.run
@@ Dream.logger
@@ Dream.router [
Dream.get "/api/hello" json_handler;
]
- WebSocket echo server:
let websocket_handler = Dream.websocket (fun ws ->
let rec loop () =
match%lwt Dream.receive ws with
| Some message ->
let%lwt () = Dream.send ws message in
loop ()
| None -> Lwt.return_unit
in
loop ())
let () =
Dream.run
@@ Dream.logger
@@ Dream.router [
Dream.get "/ws" websocket_handler;
]
Use cases
- RESTful APIs
Easily create and manage API endpoints
Leverage OCaml's type system for robust data handling
- Single Page Applications (SPAs)
Serve static files and handle API requests
Implement server-side rendering for improved performance
- Microservices
Build lightweight, focused services
Utilize Dream's performance for high-throughput scenarios
- Real-time Applications
Implement WebSocket support for live updates
Create chat applications or collaborative tools
- Content Management Systems (CMS)
Develop custom CMS backends
Integrate with databases and handle content delivery
Features
Lightweight and minimal core
Built-in routing with support for path parameters
Request and response handling with type-safe abstractions
WebSocket support
Static file serving
Sessions and cookies management
HTTPS and HTTP/2 support
Middleware system for easy extensibility
Integration with popular OCaml libraries (Lwt, Yojson, etc.)
Excellent performance and low memory footprint
Comprehensive documentation and examples
Take-away
Dream offers OCaml developers a modern, type-safe, and performant framework for web development. Its minimalist approach and intuitive API make it accessible to both beginners and experienced programmers. By leveraging OCaml's strengths, Dream provides a solid foundation for building robust web applications, APIs, and microservices. Whether you're working on a small project or a large-scale application, Dream's flexibility and performance make it a compelling choice for functional programmers venturing into web development. As the OCaml ecosystem continues to grow, Dream stands out as a promising tool for creating efficient and reliable web solutions.
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
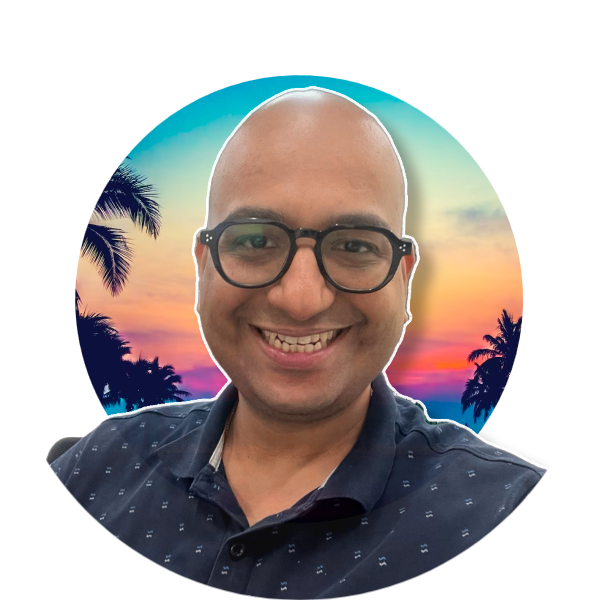
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.