Promises Made Simple: A Comprehensive JavaScript Tutorial
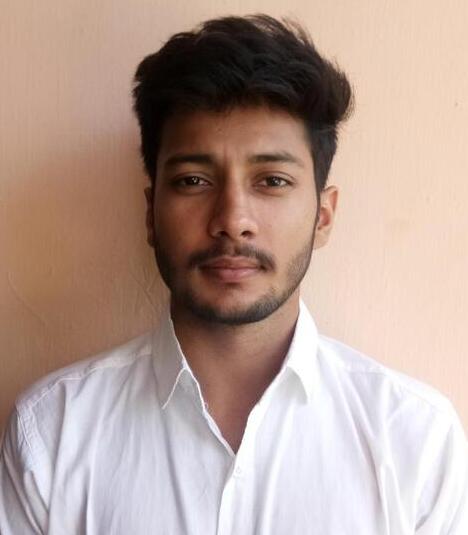
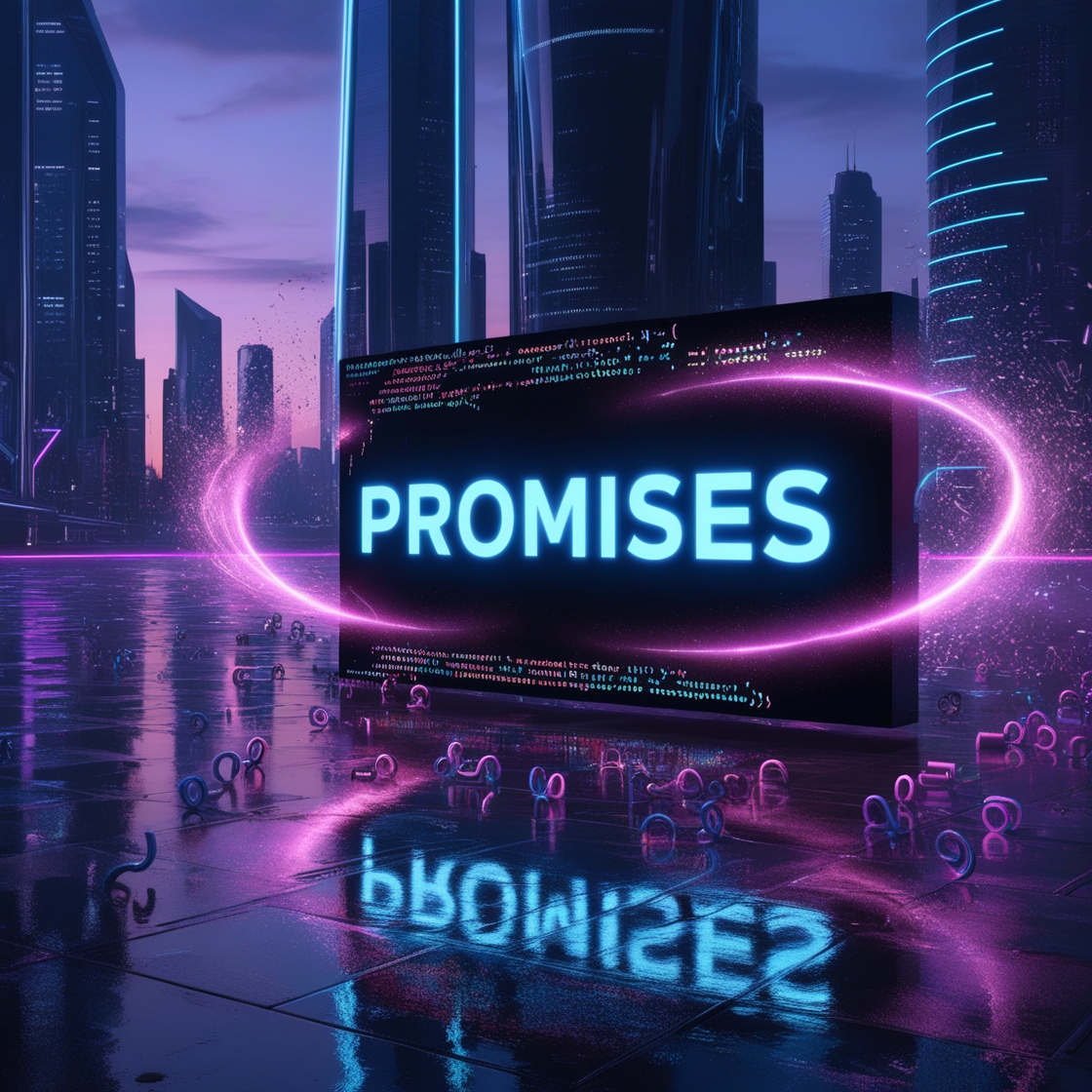
As a developer, you’re likely familiar with the phrase, “JavaScript is asynchronous by nature.” This characteristic allows JavaScript to perform multiple tasks without waiting for each one to complete before moving on to the next. However, managing these asynchronous tasks can sometimes be challenging. Enter Promises — one of the most powerful features in modern JavaScript for handling asynchronous operations.
What is a Promise?
A Promise in JavaScript is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Think of it as a placeholder for a value that will be available in the future. Promises provide a more elegant way to handle asynchronous code, replacing the old callback pattern with a more readable and maintainable approach.
A Promise can be in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully.
Rejected: The operation failed.
Creating a Promise
Creating a Promise is straightforward. Here’s a simple example:
const myPromise = new Promise((resolve, reject) => {
let condition = true; // this condition can be any asynchronous operation
if (condition) {
resolve("The promise was fulfilled!");
} else {
reject("The promise was rejected.");
}
});
In this example, myPromise
will be fulfilled if condition
is true and rejected if condition
is false.
Handling Promises
To handle the outcome of a Promise, you use the .then()
, .catch()
, and .finally()
methods.
.then(): This method takes two arguments, a callback for the fulfilled case and another for the rejected case. The second argument is optional.
myPromise.then((message) => {
console.log(message); // "The promise was fulfilled!"
}).catch((error) => {
console.log(error); // "The promise was rejected."
});
.catch(): This method is a shorthand for .then(null, rejection)
. It is used to handle errors.
myPromise.catch((error) => {
console.log(error); // "The promise was rejected."
});
.finally(): This method executes regardless of the promise being fulfilled or rejected, making it perfect for cleanup tasks.
myPromise.finally(() => {
console.log("Promise has been settled.");
});
Chaining Promises
One of the most powerful features of Promises is the ability to chain them, allowing you to handle a sequence of asynchronous operations.
const promise1 = new Promise((resolve) => {
setTimeout(() => resolve("First promise resolved!"), 1000);
});
promise1.then((result) => {
console.log(result); // "First promise resolved!"
return new Promise((resolve) => {
setTimeout(() => resolve("Second promise resolved!"), 1000);
});
}).then((result) => {
console.log(result); // "Second promise resolved!"
});
Promise.all and Promise.race
JavaScript also provides utility methods like Promise.all
and Promise.race
for working with multiple promises.
Promise.all(): This method takes an array of promises and returns a single promise that resolves when all of the input promises have resolved, or rejects if any of the input promises reject.
const promise2 = Promise.resolve("Promise 2 resolved"); const promise3 = Promise.resolve("Promise 3 resolved"); Promise.all([promise1, promise2, promise3]).then((values) => { console.log(values); // ["First promise resolved!", "Promise 2 resolved", "Promise 3 resolved"] });
Promise.race(): This method returns a promise that resolves or rejects as soon as one of the input promises resolves or rejects.
Promise.race([promise1, promise2, promise3]).then((value) => { console.log(value); // "First promise resolved!" (assuming promise1 resolves first) });
Real-World Application
Let’s consider a real-world example from my journey as a developer. Suppose you’re working on a meeting scheduling app where you need to fetch available time slots from different APIs. Using Promises, you can handle these asynchronous requests efficiently.
const fetchGoogleCalendar = new Promise((resolve) => { setTimeout(() => resolve("Google Calendar slots fetched"), 2000); }); const fetchOutlookCalendar = new Promise((resolve) => { setTimeout(() => resolve("Outlook Calendar slots fetched"), 3000); }); Promise.all([fetchGoogleCalendar, fetchOutlookCalendar]).then((values) => { console.log(values); // ["Google Calendar slots fetched", "Outlook Calendar slots fetched"] // Now you can process and merge these slots for the user. }).catch((error) => { console.log("Error fetching calendar slots:", error); });
This example demonstrates how Promises can streamline handling multiple asynchronous tasks, making your code cleaner and more maintainable.
Conclusion
Promises are a fundamental part of modern JavaScript, enabling developers to handle asynchronous operations more effectively. By understanding and utilizing Promises, you can write cleaner, more readable, and more maintainable code. As you continue your development journey, mastering Promises will undoubtedly enhance your ability to build robust and efficient applications.
Happy coding!
Subscribe to my newsletter
Read articles from Achutendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
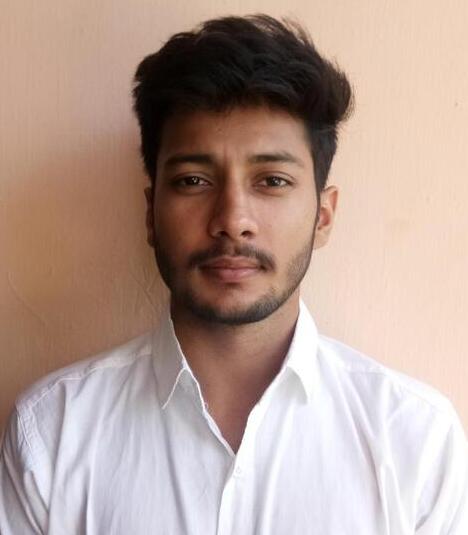
Achutendra Singh
Achutendra Singh
Full Stack Developer with a passion for continuous learning. Connect with me to explore the fascinating world of technology and beyond!