Coding Schrödinger's cat with Qiskit
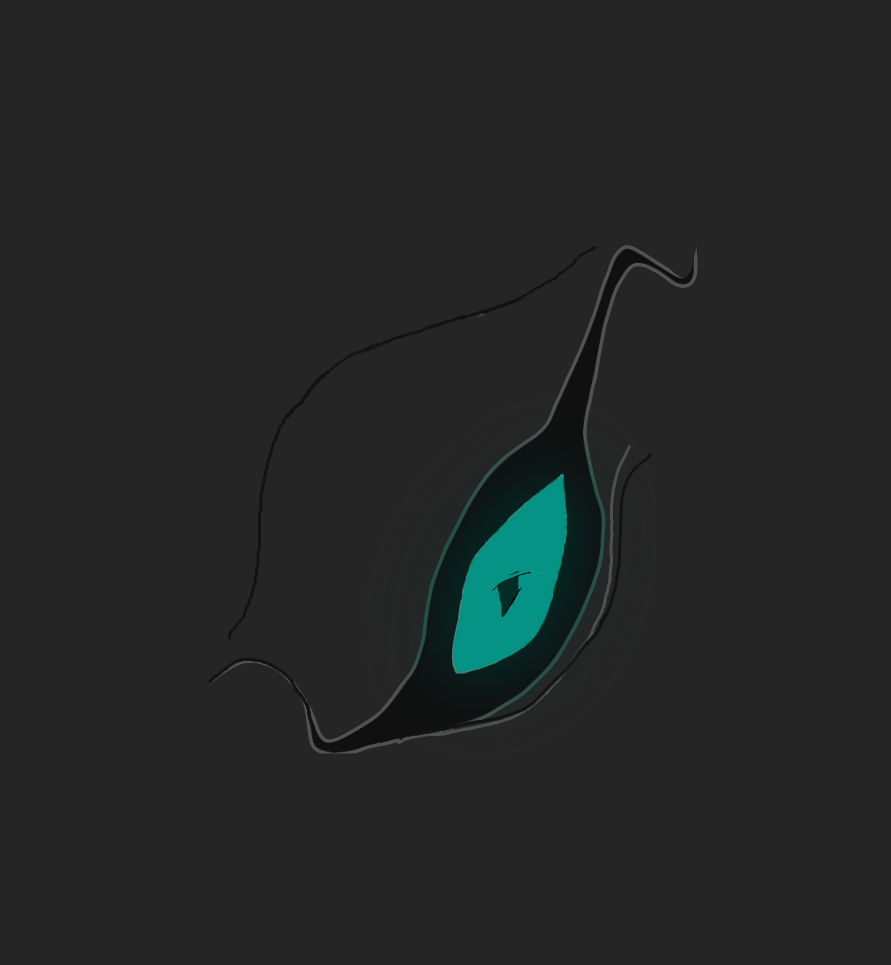
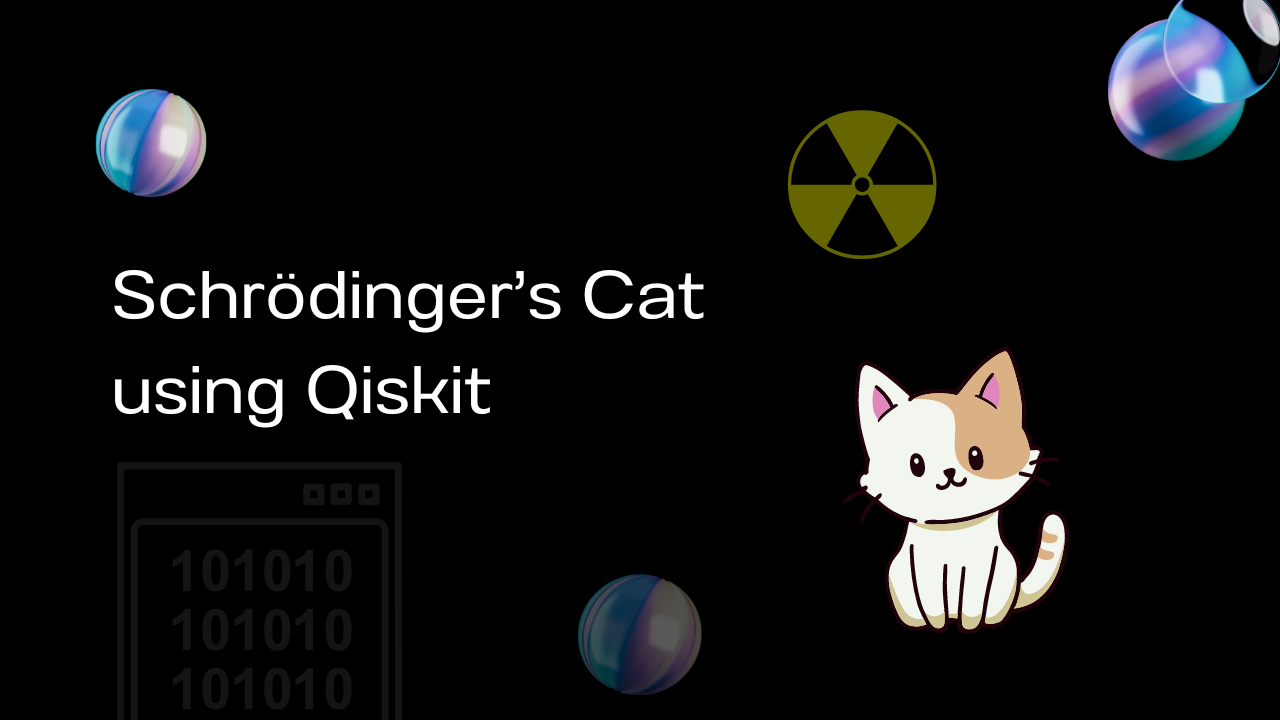
At least once in our journey as a science student, we have been mesmerized by the idea of Schrödinger's cat, and all the amazing concepts that quantum mechanics hold. Well!! what if we can code the concept, and no, not using a random number module, but implementing quantum superposition using a Hadamard gate, if you didn't get it, don't worry, let's dive into it.
Concept
In concept, we will use a qubit (a quantum version of a bit) resembling the radioactive atom, which can exist in a quantum superposition state. To achieve that we have to apply a Hadamard gate to it. A Hadamard gate is a quantum gate (an operation that can be performed in a qubit) that can be used to bring a qubit into a superposition state. Once we get our qubit ready, we will use another qubit to resemble the cat that entangles with the qubit 'atom'. Now we perform another gate called Controlled-Not such that: if atom == 1 then cat = !cat else (do nothing).
Now once we observe the system, i.e.: measure it in a classical bit, the system collapses to give either 'the cat is dead' or 'the cat is alive' outcome.
Implementation
from qiskit import QuantumCircuit, QuantumRegister, ClassicalRegister
from qiskit_aer import AerSimulator
backend = AerSimulator()
qr = QuantumRegister(1, name="atom")
qr_cat = QuantumRegister(1, name="cat")
deadoralive = ClassicalRegister(1, name="Dead or Alive")
qc = QuantumCircuit(qr, qr_cat, deadoralive)
qc.h(qr)
qc.cx(qr, qr_cat)
qc.measure(qr_cat, deadoralive)
For this article, we'll be using the Qiskit library. Qiskit is an open-source software development kit for working with quantum computers at the level of circuits, pulses, and algorithms.
In the first few lines, we have initialized the registers with 1 qubit each. Next, we initialize the quantum circuit with the registers.
In lines 10 & 11, we have applied the Hadamard gate and CNOT(controlled-not) gate as explained in the concept section. Next, we have to run the circuit, and we'll get the result
def observe():
result = backend.run(qc, shots=1).result()
return list(result.get_counts())[0]
if __name__ == '__main__':
while True:
input("\nPress enter to observe...")
observed = int(observe())
if observed:
print("The cat is dead!! Bury it")
else:
print("Voila!! The cat is alive")
Here, backend.run() runs the quantum circuit in a basic quantum simulator, and returns the result. In this case, this gives the measured state of the cat.
And finally, we can integrate it with a decent interface, expressing the results through custom strings
Summary
Although the concepts used here assume we know how a quantum circuit, or what a quantum gate is, or even the famous thought experiment of Schrödinger's cat. However, it is one of the fundamental understandings in the field of quantum computing. This can be extended to various ideas like 'What does observation mean?' or 'Who can be considered as observers?'. One such interesting theory suggests that once someone observes the states of the experiment, the observer becomes part of the entangled system, which can now coexist in the states of 'seen the cat dead' or 'seen the cat alive'.
Subscribe to my newsletter
Read articles from Ghostfreak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
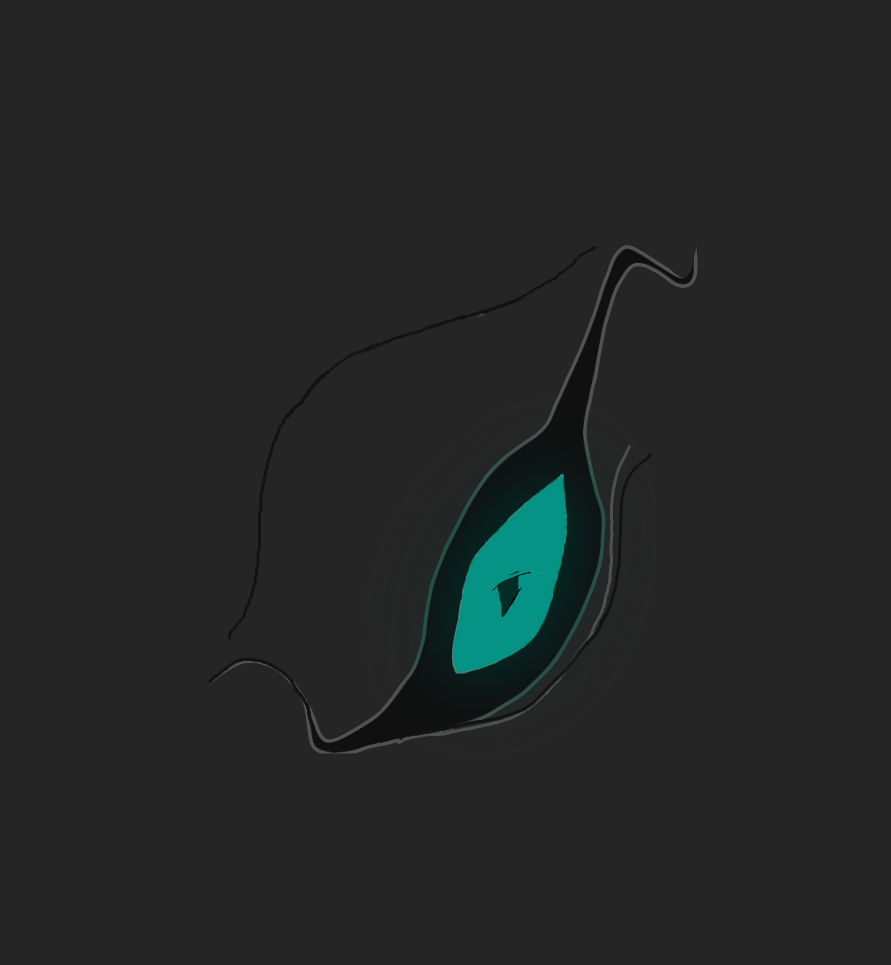
Ghostfreak
Ghostfreak
I am a Student who is trying to explore the tech world