Mastering Java: A Comprehensive Guide to Learning Java and Its Core Concepts

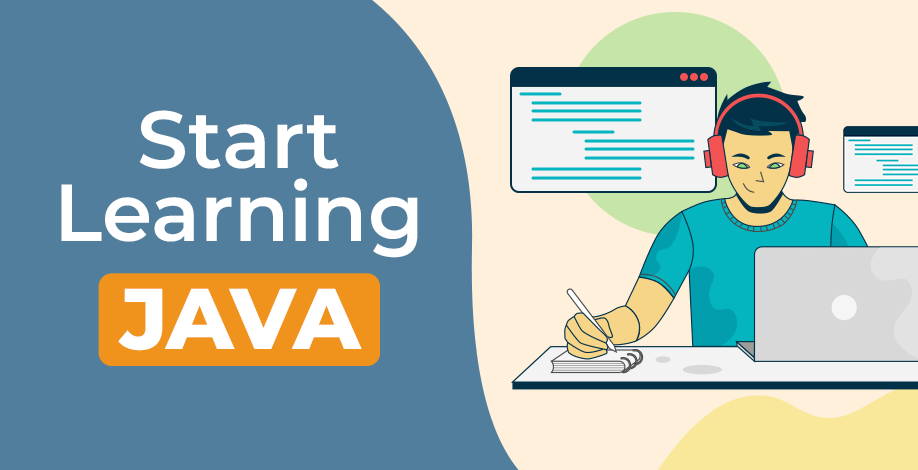
Java is one of the most popular programming languages in the world, known for its versatility, scalability, and robustness. Whether you're a beginner starting your programming journey or an experienced developer looking to expand your skill set, mastering Java is a valuable and rewarding pursuit. This comprehensive guide will take you through the essential steps to learn Java, its core pillars, and the distinctions between Core Java and Advanced Java. Let's dive into the world of Java!
1. Getting Started with Java
Understanding Java: Java is an object-oriented, class-based, and platform-independent programming language. It was developed by Sun Microsystems (now owned by Oracle) and released in 1995. Java's syntax is similar to C++, making it easy for developers familiar with C-style languages to learn.
Setting Up Your Environment: Before you start coding, you need to set up your development environment. Hereβs how you can get started:
Download and Install JDK:
- The Java Development Kit (JDK) includes tools required to develop and run Java programs. You can download it from the Oracle website or use an OpenJDK version.
Set Up Your IDE:
- Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, and NetBeans provide powerful features to simplify coding. Choose an IDE and install it on your machine.
Configure Environment Variables:
- Set up the
JAVA_HOME
environment variable and add the JDK bin directory to your system's PATH variable to enable Java commands in the terminal.
- Set up the
Verify Installation:
- Open a terminal or command prompt and type
java -version
andjavac -version
to verify that Java is installed correctly.
- Open a terminal or command prompt and type
2. Core Concepts of Java
Java's core concepts, often referred to as the "four pillars" of Java, are fundamental to understanding and mastering the language. These pillars are:
Abstraction:
- Abstraction is the concept of hiding the complex implementation details and showing only the essential features of an object. In Java, abstraction is achieved through abstract classes and interfaces. Abstract classes can have both abstract and concrete methods, while interfaces can have only abstract methods (Java 8 onwards allows default and static methods in interfaces).
Encapsulation:
- Encapsulation is the process of wrapping data (variables) and methods (functions) into a single unit called a class. It restricts direct access to some of the object's components and can be achieved through access modifiers (private, protected, public). Encapsulation ensures that the internal representation of an object is hidden from the outside, providing a clear interface for interaction.
Inheritance:
- Inheritance is the mechanism by which one class (child or subclass) can inherit the properties and methods of another class (parent or superclass). It promotes code reuse and establishes a hierarchical relationship between classes. Java supports single inheritance (a class can inherit from only one superclass) and interfaces for multiple inheritance-like behavior.
Polymorphism:
- Polymorphism allows objects to be treated as instances of their parent class rather than their actual class. The two types of polymorphism in Java are compile-time (method overloading) and runtime (method overriding). Method overloading allows multiple methods with the same name but different parameters, while method overriding allows a subclass to provide a specific implementation of a method already defined in its superclass.
3. Core Java
Core Java refers to the fundamental features and libraries of the Java programming language. It covers the basic building blocks required to build general-purpose applications. Here are some key areas of Core Java:
Basic Syntax and Structure:
- Understanding Java's syntax, data types, operators, control flow statements (if-else, switch, loops), and basic input/output operations.
Object-Oriented Programming (OOP):
- Learning how to define and use classes, objects, methods, constructors, and understand concepts like inheritance, polymorphism, abstraction, and encapsulation.
Exception Handling:
- Handling errors and exceptions using try-catch blocks, finally clause, and custom exceptions to ensure robust and error-free code.
Collections Framework:
- Using data structures like lists, sets, maps, and queues provided by the Java Collections Framework to store and manipulate groups of objects.
Multithreading and Concurrency:
- Creating and managing multiple threads to perform concurrent tasks, understanding synchronization, thread lifecycle, and concurrent collections.
File I/O and Serialization:
- Reading from and writing to files, handling byte and character streams, and serializing objects to persist their state.
Java Standard Library:
- Exploring the rich set of built-in libraries and APIs provided by Java, including utility classes, data structures, networking, and more.
4. Advanced Java
Advanced Java builds on the foundation of Core Java and focuses on developing web-based, network-centric, and enterprise-level applications. Here are some key areas of Advanced Java:
Java Database Connectivity (JDBC):
- Connecting to databases, executing SQL queries, and handling transactions using JDBC API to interact with relational databases.
Java Servlets and JavaServer Pages (JSP):
- Developing dynamic web applications using servlets and JSP. Servlets handle server-side logic, while JSP allows embedding Java code in HTML for dynamic content generation.
Java Enterprise Edition (Java EE):
- Building scalable, secure, and robust enterprise applications using Java EE technologies like Enterprise JavaBeans (EJB), Java Message Service (JMS), and Java Persistence API (JPA).
Web Frameworks:
- Leveraging popular web frameworks like Spring, Hibernate, and Struts to simplify development, improve productivity, and enhance application performance.
RESTful Web Services:
- Creating RESTful APIs to enable communication between different systems over HTTP using technologies like JAX-RS and Jersey.
Microservices:
- Designing and implementing microservices architecture using Java frameworks like Spring Boot and tools like Docker and Kubernetes for containerization and orchestration.
5. Learning Resources and Tips
Online Courses and Tutorials:
- Platforms like Coursera, Udemy, and Pluralsight offer comprehensive Java courses for all levels. YouTube channels and blogs also provide valuable tutorials and guides.
Books:
- "Effective Java" by Joshua Bloch, "Java: The Complete Reference" by Herbert Schildt, and "Head First Java" by Kathy Sierra and Bert Bates are excellent books for learning Java.
Practice and Projects:
- Practice coding regularly on platforms like LeetCode, HackerRank, and CodeWars. Work on real-world projects to apply your knowledge and gain practical experience.
Community and Forums:
- Join Java communities, forums, and groups like Stack Overflow, Reddit, and GitHub to connect with other developers, seek help, and share your knowledge.
Consistent Learning:
- Stay updated with the latest Java developments, new features, and best practices. Continuously improve your skills by learning new libraries, frameworks, and tools.
Conclusion
Learning Java is a journey that requires dedication, practice, and a solid understanding of its core concepts. By mastering Core Java and exploring Advanced Java, you can build powerful, scalable, and efficient applications. Whether you're aiming to develop web applications, enterprise solutions, or innovative software products, Java provides the tools and resources to achieve your goals. So, start your learning journey today and unlock the potential of one of the most influential programming languages in the world!
Subscribe to my newsletter
Read articles from Aniket Dhaygude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aniket Dhaygude
Aniket Dhaygude
π¨βπ» Freelance Developer | UI/UX Enthusiast | Tech Blogger Transforming ideas into engaging digital experiences. I specialize in front-end development, UI/UX design, and creating dynamic web apps. Let's build something amazing together! π